Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / UIAutomation / UIAutomationProvider / System / Windows / Automation / Provider / AutomationInteropProvider.cs / 1 / AutomationInteropProvider.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Provides functionality that Win32/Avalon servers need (non-Avalon specific) // // History: // 06/02/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 using System; using System.Windows.Automation; using MS.Internal.Automation; namespace System.Windows.Automation.Provider { ////// Class containing methods used by Win32 Automation implementations /// #if (INTERNAL_COMPILE) internal static class AutomationInteropProvider #else public static class AutomationInteropProvider #endif { //----------------------------------------------------- // // Public Constants & readonly Fields // //----------------------------------------------------- #region Public Constants & readonly Fields ///WM_GETOBJECT lParam value indicating that server should return a reference to the root RawElementProvider public const int RootObjectId = -25; ///Maximum number of events to send before batching public const int InvalidateLimit = 20; ///When returned as the first element of IRawElementProviderFragment.GetRuntimeId(), indicates /// that the ID is partial and should be appended to the ID provided by the base provider. Typically /// only used by Win32 proxies public const int AppendRuntimeId = 3; #endregion Public Constants & readonly Fields //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Servers that are slotting into the HWND tree can use this to get a base implementation. /// /// HWND that server is slotting in over ///base raw element for specified window public static IRawElementProviderSimple HostProviderFromHandle ( IntPtr hwnd ) { ValidateArgument(hwnd != IntPtr.Zero, SRID.HwndMustBeNonNULL); return UiaCoreProviderApi.UiaHostProviderFromHwnd(hwnd); } ////// Server uses this to return an element in response to WM_GETOBJECT. /// /// hwnd from the WM_GETOBJECT message /// wParam from the WM_GETOBJECT message /// lParam from the WM_GETOBJECT message /// element to return ///Server should return the return value as the lresult return value to the WM_GETOBJECT windows message public static IntPtr ReturnRawElementProvider (IntPtr hwnd, IntPtr wParam, IntPtr lParam, IRawElementProviderSimple el ) { ValidateArgument( hwnd != IntPtr.Zero, SRID.HwndMustBeNonNULL ); ValidateArgumentNonNull(el, "el" ); return UiaCoreProviderApi.UiaReturnRawElementProvider(hwnd, wParam, lParam, el); } ////// Called by a server to determine if there are any listeners for events. /// public static bool ClientsAreListening { get { return UiaCoreProviderApi.UiaClientsAreListening(); } } ////// Called by a server to notify the UIAccess server of a AutomationPropertyChangedEvent event. /// /// The actual server-side element associated with this event. /// Contains information about the property that changed. public static void RaiseAutomationPropertyChangedEvent(IRawElementProviderSimple element, AutomationPropertyChangedEventArgs e) { ValidateArgumentNonNull(element, "element"); ValidateArgumentNonNull(e, "e"); // PRESHARP will flag this as warning 56506/6506:Parameter 'e' to this public method must be validated: A null-dereference can occur here. // False positive, e is checked, see above #pragma warning suppress 6506 UiaCoreProviderApi.UiaRaiseAutomationPropertyChangedEvent(element, e.Property.Id, e.OldValue, e.NewValue); } ////// Called to notify listeners of a pattern or custom event. This could could be called by a server implementation or by a proxy's event /// translator. /// /// An AutomationEvent representing this event. /// The actual server-side element associated with this event. /// Contains information about the event (may be null). public static void RaiseAutomationEvent(AutomationEvent eventId, IRawElementProviderSimple provider, AutomationEventArgs e) { ValidateArgumentNonNull(eventId, "eventId"); ValidateArgumentNonNull(provider, "provider"); ValidateArgumentNonNull(e, "e"); // PRESHARP will flag this as warning 56506/6506:Parameter 'e' to this public method must be validated: A null-dereference can occur here. // False positive, e is checked, see above #pragma warning suppress 6506 if (e.EventId == AutomationElementIdentifiers.AsyncContentLoadedEvent) { AsyncContentLoadedEventArgs asyncArgs = e as AsyncContentLoadedEventArgs; if(asyncArgs == null) ThrowInvalidArgument("e"); UiaCoreProviderApi.UiaRaiseAsyncContentLoadedEvent(provider, asyncArgs.AsyncContentLoadedState, asyncArgs.PercentComplete); return; } // PRESHARP will flag this as warning 56506/6506:Parameter 'e' to this public method must be validated: A null-dereference can occur here. // False positive, e is checked, see above #pragma warning suppress 6506 if (e.EventId == WindowPatternIdentifiers.WindowClosedEvent && !(e is WindowClosedEventArgs)) ThrowInvalidArgument("e"); // fire to all clients // PRESHARP will flag this as warning 56506/6506:Parameter 'eventId' to this public method must be validated: A null-dereference can occur here. // False positive, eventId is checked, see above #pragma warning suppress 6506 UiaCoreProviderApi.UiaRaiseAutomationEvent(provider, eventId.Id); } ////// Called by a server to notify the UIAccess server of a tree change event. /// /// The actual server-side element associated with this event. /// Contains information about the event. public static void RaiseStructureChangedEvent(IRawElementProviderSimple provider, StructureChangedEventArgs e) { ValidateArgumentNonNull(provider, "provider"); ValidateArgumentNonNull(e, "e"); // PRESHARP will flag this as warning 56506/6506:Parameter 'e' to this public method must be validated: A null-dereference can occur here. // False positive, e is checked, see above #pragma warning suppress 6506 UiaCoreProviderApi.UiaRaiseStructureChangedEvent(provider, e.StructureChangeType, e.GetRuntimeId()); } #endregion Public Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods // Check that specified argument is non-null, if so, throw exception private static void ValidateArgumentNonNull(object obj, string argName) { if (obj == null) { throw new ArgumentNullException(argName); } } // Throw an argument Exception with a generic error private static void ThrowInvalidArgument(string argName) { throw new ArgumentException(SR.Get(SRID.GenericInvalidArgument, argName)); } // Check that specified condition is true; if not, throw exception private static void ValidateArgument(bool cond, string reason) { if (!cond) { throw new ArgumentException(SR.Get(reason)); } } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Provides functionality that Win32/Avalon servers need (non-Avalon specific) // // History: // 06/02/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 using System; using System.Windows.Automation; using MS.Internal.Automation; namespace System.Windows.Automation.Provider { ////// Class containing methods used by Win32 Automation implementations /// #if (INTERNAL_COMPILE) internal static class AutomationInteropProvider #else public static class AutomationInteropProvider #endif { //----------------------------------------------------- // // Public Constants & readonly Fields // //----------------------------------------------------- #region Public Constants & readonly Fields ///WM_GETOBJECT lParam value indicating that server should return a reference to the root RawElementProvider public const int RootObjectId = -25; ///Maximum number of events to send before batching public const int InvalidateLimit = 20; ///When returned as the first element of IRawElementProviderFragment.GetRuntimeId(), indicates /// that the ID is partial and should be appended to the ID provided by the base provider. Typically /// only used by Win32 proxies public const int AppendRuntimeId = 3; #endregion Public Constants & readonly Fields //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Servers that are slotting into the HWND tree can use this to get a base implementation. /// /// HWND that server is slotting in over ///base raw element for specified window public static IRawElementProviderSimple HostProviderFromHandle ( IntPtr hwnd ) { ValidateArgument(hwnd != IntPtr.Zero, SRID.HwndMustBeNonNULL); return UiaCoreProviderApi.UiaHostProviderFromHwnd(hwnd); } ////// Server uses this to return an element in response to WM_GETOBJECT. /// /// hwnd from the WM_GETOBJECT message /// wParam from the WM_GETOBJECT message /// lParam from the WM_GETOBJECT message /// element to return ///Server should return the return value as the lresult return value to the WM_GETOBJECT windows message public static IntPtr ReturnRawElementProvider (IntPtr hwnd, IntPtr wParam, IntPtr lParam, IRawElementProviderSimple el ) { ValidateArgument( hwnd != IntPtr.Zero, SRID.HwndMustBeNonNULL ); ValidateArgumentNonNull(el, "el" ); return UiaCoreProviderApi.UiaReturnRawElementProvider(hwnd, wParam, lParam, el); } ////// Called by a server to determine if there are any listeners for events. /// public static bool ClientsAreListening { get { return UiaCoreProviderApi.UiaClientsAreListening(); } } ////// Called by a server to notify the UIAccess server of a AutomationPropertyChangedEvent event. /// /// The actual server-side element associated with this event. /// Contains information about the property that changed. public static void RaiseAutomationPropertyChangedEvent(IRawElementProviderSimple element, AutomationPropertyChangedEventArgs e) { ValidateArgumentNonNull(element, "element"); ValidateArgumentNonNull(e, "e"); // PRESHARP will flag this as warning 56506/6506:Parameter 'e' to this public method must be validated: A null-dereference can occur here. // False positive, e is checked, see above #pragma warning suppress 6506 UiaCoreProviderApi.UiaRaiseAutomationPropertyChangedEvent(element, e.Property.Id, e.OldValue, e.NewValue); } ////// Called to notify listeners of a pattern or custom event. This could could be called by a server implementation or by a proxy's event /// translator. /// /// An AutomationEvent representing this event. /// The actual server-side element associated with this event. /// Contains information about the event (may be null). public static void RaiseAutomationEvent(AutomationEvent eventId, IRawElementProviderSimple provider, AutomationEventArgs e) { ValidateArgumentNonNull(eventId, "eventId"); ValidateArgumentNonNull(provider, "provider"); ValidateArgumentNonNull(e, "e"); // PRESHARP will flag this as warning 56506/6506:Parameter 'e' to this public method must be validated: A null-dereference can occur here. // False positive, e is checked, see above #pragma warning suppress 6506 if (e.EventId == AutomationElementIdentifiers.AsyncContentLoadedEvent) { AsyncContentLoadedEventArgs asyncArgs = e as AsyncContentLoadedEventArgs; if(asyncArgs == null) ThrowInvalidArgument("e"); UiaCoreProviderApi.UiaRaiseAsyncContentLoadedEvent(provider, asyncArgs.AsyncContentLoadedState, asyncArgs.PercentComplete); return; } // PRESHARP will flag this as warning 56506/6506:Parameter 'e' to this public method must be validated: A null-dereference can occur here. // False positive, e is checked, see above #pragma warning suppress 6506 if (e.EventId == WindowPatternIdentifiers.WindowClosedEvent && !(e is WindowClosedEventArgs)) ThrowInvalidArgument("e"); // fire to all clients // PRESHARP will flag this as warning 56506/6506:Parameter 'eventId' to this public method must be validated: A null-dereference can occur here. // False positive, eventId is checked, see above #pragma warning suppress 6506 UiaCoreProviderApi.UiaRaiseAutomationEvent(provider, eventId.Id); } ////// Called by a server to notify the UIAccess server of a tree change event. /// /// The actual server-side element associated with this event. /// Contains information about the event. public static void RaiseStructureChangedEvent(IRawElementProviderSimple provider, StructureChangedEventArgs e) { ValidateArgumentNonNull(provider, "provider"); ValidateArgumentNonNull(e, "e"); // PRESHARP will flag this as warning 56506/6506:Parameter 'e' to this public method must be validated: A null-dereference can occur here. // False positive, e is checked, see above #pragma warning suppress 6506 UiaCoreProviderApi.UiaRaiseStructureChangedEvent(provider, e.StructureChangeType, e.GetRuntimeId()); } #endregion Public Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods // Check that specified argument is non-null, if so, throw exception private static void ValidateArgumentNonNull(object obj, string argName) { if (obj == null) { throw new ArgumentNullException(argName); } } // Throw an argument Exception with a generic error private static void ThrowInvalidArgument(string argName) { throw new ArgumentException(SR.Get(SRID.GenericInvalidArgument, argName)); } // Check that specified condition is true; if not, throw exception private static void ValidateArgument(bool cond, string reason) { if (!cond) { throw new ArgumentException(SR.Get(reason)); } } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
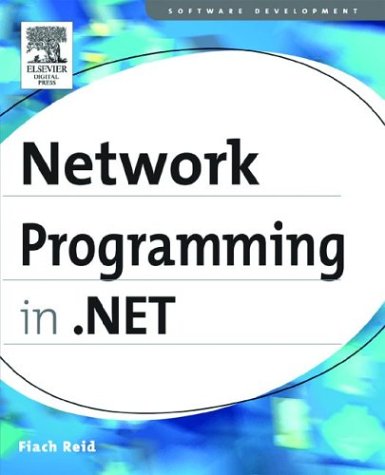
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HostExecutionContextManager.cs
- Registry.cs
- SchemaCollectionCompiler.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- XmlDigitalSignatureProcessor.cs
- OleDbCommand.cs
- ConnectionManager.cs
- DataPointer.cs
- MULTI_QI.cs
- DesignerWidgets.cs
- DataGridViewCellValidatingEventArgs.cs
- Type.cs
- DropDownList.cs
- DataServiceQueryOfT.cs
- UserControl.cs
- ToolStripStatusLabel.cs
- HttpCacheVary.cs
- UnsafeNativeMethods.cs
- EventBindingService.cs
- WebUtility.cs
- QuaternionRotation3D.cs
- BasicHttpSecurityMode.cs
- BaseValidator.cs
- BaseValidatorDesigner.cs
- ArcSegment.cs
- RegexTree.cs
- PngBitmapDecoder.cs
- ResourcePool.cs
- ACE.cs
- PipelineModuleStepContainer.cs
- HttpConfigurationContext.cs
- ColorAnimationBase.cs
- PathFigureCollectionConverter.cs
- XamlClipboardData.cs
- Size3D.cs
- JsonServiceDocumentSerializer.cs
- DeviceFilterDictionary.cs
- xdrvalidator.cs
- XmlDownloadManager.cs
- SimpleModelProvider.cs
- ReflectionHelper.cs
- FormViewPagerRow.cs
- DrawingState.cs
- ExtentCqlBlock.cs
- GridViewCellAutomationPeer.cs
- FaultConverter.cs
- BitConverter.cs
- SoapObjectWriter.cs
- FormViewDesigner.cs
- ListenerBinder.cs
- DocumentApplicationState.cs
- PerfCounterSection.cs
- TaskFormBase.cs
- XappLauncher.cs
- SynchronizedKeyedCollection.cs
- DoubleLinkList.cs
- ButtonBase.cs
- PenThreadPool.cs
- WebReferencesBuildProvider.cs
- DesignTimeValidationFeature.cs
- RuleAction.cs
- DbConnectionOptions.cs
- EventlogProvider.cs
- QueryGenerator.cs
- GAC.cs
- RecordsAffectedEventArgs.cs
- ObjectListItemCollection.cs
- VisualStyleElement.cs
- Rect.cs
- MissingFieldException.cs
- NetDispatcherFaultException.cs
- ListenerUnsafeNativeMethods.cs
- PersonalizableTypeEntry.cs
- SortableBindingList.cs
- InheritanceContextHelper.cs
- ShaderEffect.cs
- SystemNetworkInterface.cs
- DllNotFoundException.cs
- FeatureSupport.cs
- mil_sdk_version.cs
- SqlConnectionHelper.cs
- _ScatterGatherBuffers.cs
- HGlobalSafeHandle.cs
- PtsCache.cs
- ContextBase.cs
- SQLDoubleStorage.cs
- ScriptMethodAttribute.cs
- SmtpLoginAuthenticationModule.cs
- DataControlFieldCollection.cs
- ProgressiveCrcCalculatingStream.cs
- DynamicMethod.cs
- SignatureGenerator.cs
- StringConverter.cs
- DbDataReader.cs
- RelationshipEndCollection.cs
- Rfc2898DeriveBytes.cs
- FixedSOMTextRun.cs
- WebReference.cs
- ServiceActivationException.cs
- StatusBarAutomationPeer.cs