Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SrgsCompiler / grammarelement.cs / 1 / grammarelement.cs
//// Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: // // History: // 6/1/2004 [....] Converted from the managed code // 10/1/2004 [....] Added Custom grammar support //---------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Globalization; using System.Speech.Internal.SrgsParser; namespace System.Speech.Internal.SrgsCompiler { internal class GrammarElement : ParseElement, IGrammar { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors #if !NO_STG internal GrammarElement (Backend backend, CustomGrammar cg) : base (null) { _cg = cg; #else internal GrammarElement (Backend backend) : base (null) { #endif _backend = backend; } #endregion //******************************************************************* // // Internal Method // //******************************************************************** #region Internal Method string IGrammar.Root { set { _sRoot = value; } get { return _sRoot; } } IRule IGrammar.CreateRule (string id, RulePublic publicRule, RuleDynamic dynamic, bool hasScript) { SPCFGRULEATTRIBUTES dwRuleAttributes = 0; // Determine rule attributes to apply based on RuleScope, IsDynamic, and IsRootRule. // IsRootRule RuleScope IsDynamic Rule Attributes // --------------------------------------------------------------------- // true * true Root | Active | TopLevel | Export | Dynamic // true * false Root | Active | TopLevel | Export // false internal true TopLevel | Export | Dynamic // false internal false TopLevel | Export // false private true Dynamic // false private false 0 if (id == _sRoot) { dwRuleAttributes |= SPCFGRULEATTRIBUTES.SPRAF_Root | SPCFGRULEATTRIBUTES.SPRAF_Active | SPCFGRULEATTRIBUTES.SPRAF_TopLevel; _hasRoot = true; } if (publicRule == RulePublic.True) { dwRuleAttributes |= SPCFGRULEATTRIBUTES.SPRAF_TopLevel | SPCFGRULEATTRIBUTES.SPRAF_Export; } if (dynamic == RuleDynamic.True) { // BackEnd supports exported dynamic rules for SRGS grammars. dwRuleAttributes |= SPCFGRULEATTRIBUTES.SPRAF_Dynamic; } // Create rule with specified attributes Rule rule = GetRule (id, dwRuleAttributes); #if !NO_STG // Add this rule to the list of rules of the STG list if (publicRule == RulePublic.True || id == _sRoot || hasScript) { _cg._rules.Add (rule); } #endif return (IRule) rule; } void IElement.PostParse (IElement parent) { if (_sRoot != null && !_hasRoot) { // "Root rule ""%s"" is undefined." XmlParser.ThrowSrgsException (SRID.RootNotDefined, _sRoot); } if (_undefRules.Count > 0) { // "Root rule ""%s"" is undefined." Rule rule = _undefRules [0]; XmlParser.ThrowSrgsException (SRID.UndefRuleRef, rule.Name); } #if !NO_STG // SAPI semantics only for .Net Semantics bool containsCode = ((IGrammar) this).CodeBehind.Count > 0 || ((IGrammar) this).ImportNamespaces.Count > 0 || ((IGrammar) this).AssemblyReferences.Count > 0 || CustomGrammar._scriptRefs.Count > 0; if (containsCode && ((IGrammar) this).TagFormat != System.Speech.Recognition.SrgsGrammar.SrgsTagFormat.KeyValuePairs) { XmlParser.ThrowSrgsException (SRID.InvalidSemanticProcessingType); } #endif } #if !NO_STG internal void AddScript (string name, string code) { foreach (Rule rule in _cg._rules) { if (rule.Name == name) { rule.Script.Append (code); break; } } } #endif #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties /// |summary| /// Base URI of grammar (xml:base) /// |/summary| /// |remarks| /// Uri IGrammar.XmlBase { set { if (value != null) { _backend.SetBasePath (value.ToString ()); } } } /// |summary| /// GrammarElement language (xml:lang) /// |/summary| CultureInfo IGrammar.Culture { set { Helpers.ThrowIfNull (value, "value"); _backend.LangId = value.LCID; } } /// |summary| /// GrammarElement mode. voice or dtmf /// |/summary| GrammarType IGrammar.Mode { set { _backend.GrammarMode = value; } } /// |summary| /// GrammarElement mode. voice or dtmf /// |/summary| AlphabetType IGrammar.PhoneticAlphabet { set { _backend.Alphabet = value; } } /// |summary| /// Tag format (srgs:tag-format) /// |/summary| System.Speech.Recognition.SrgsGrammar.SrgsTagFormat IGrammar.TagFormat { get { return System.Speech.Recognition.SrgsGrammar.SrgsDocument.GrammarOptions2TagFormat (_backend.GrammarOptions); } set { _backend.GrammarOptions = System.Speech.Recognition.SrgsGrammar.SrgsDocument.TagFormat2GrammarOptions (value); } } /// |summary| /// Tag format (srgs:tag-format) /// |/summary| CollectionIGrammar.GlobalTags { get { return _backend.GlobalTags; } set { _backend.GlobalTags = value; } } internal List UndefRules { get { return _undefRules; } } internal Backend Backend { get { return _backend; } } #if !NO_STG /// |summary| /// language /// |/summary| string IGrammar.Language { set { _cg._language = value; } get { return _cg._language; } } /// |summary| /// namespace /// |/summary| string IGrammar.Namespace { set { _cg._namespace = value; } get { return _cg._namespace; } } /// |summary| /// CodeBehind /// |/summary| Collection IGrammar.CodeBehind { set { _cg._codebehind = value; } get { return _cg._codebehind; } } /// |summary| /// Add #line statements to the inline scripts if set /// |/summary| bool IGrammar.Debug { set { _cg._fDebugScript = value; } } /// |summary| /// ImportNameSpaces /// |/summary| Collection IGrammar.ImportNamespaces { set { _cg._importNamespaces = value; } get { return _cg._importNamespaces; } } /// |summary| /// ImportNameSpaces /// |/summary| Collection IGrammar.AssemblyReferences { set { _cg._assemblyReferences = value; } get { return _cg._assemblyReferences; } } internal CustomGrammar CustomGrammar { get { return _cg; } } #endif #endregion //******************************************************************* // // Private Methods // //******************************************************************** #region Private Methods /// /// Create a new rule with the specified name and attribute, and return the initial state. /// Verify if Rule is unique. A Rule may already have been created as a placeholder during RuleRef. /// /// Rule name /// Rule attributes ///private Rule GetRule (string sRuleId, SPCFGRULEATTRIBUTES dwAttributes) { System.Diagnostics.Debug.Assert (!string.IsNullOrEmpty (sRuleId)); // Check if RuleID is unique. Rule rule = _backend.FindRule (sRuleId); if (rule != null) { // Rule already defined. Check if it is a placeholder. int iRule = _undefRules.IndexOf (rule); if (iRule != -1) { // This is a UndefinedRule created as a placeholder for a RuleRef. // - Update placeholder rule with correct attributes. _backend.SetRuleAttributes (rule, dwAttributes); // - Remove this now defined rule from UndefinedRules. // Swap top element with this rule and pop the top element. _undefRules.RemoveAt (iRule); } else { // Multiple definitions of the same Rule. XmlParser.ThrowSrgsException (SRID.RuleRedefinition, sRuleId); // "Redefinition of rule ""%s""." } } else { // Rule not yet defined. Create a new rule and return the InitalState. rule = _backend.CreateRule (sRuleId, dwAttributes); } return rule; } #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region Private Fields private Backend _backend; // Collection of referenced, but undefined, rules private List _undefRules = new List (); // Collection of defined rules private List _rules = new List (); #if !NO_STG private CustomGrammar _cg; #endif private string _sRoot; private bool _hasRoot; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
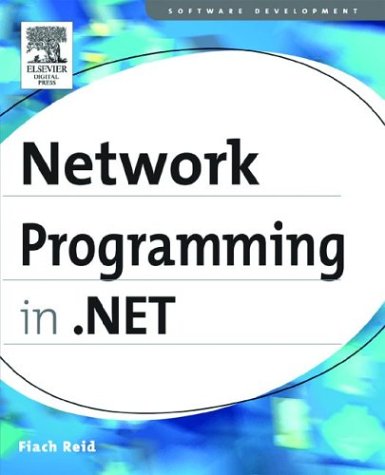
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SelectionBorderGlyph.cs
- ModelVisual3D.cs
- DbCommandDefinition.cs
- ContentPosition.cs
- WebPartMenuStyle.cs
- RectangleConverter.cs
- RegexWriter.cs
- DbLambda.cs
- HwndMouseInputProvider.cs
- PointLightBase.cs
- SyndicationFeed.cs
- DatatypeImplementation.cs
- EventProxy.cs
- Simplifier.cs
- securitymgrsite.cs
- Tag.cs
- WebPartMenuStyle.cs
- SupportingTokenSecurityTokenResolver.cs
- ParameterCollection.cs
- SoapClientMessage.cs
- TreePrinter.cs
- Exception.cs
- RtType.cs
- DateTimeOffsetStorage.cs
- XmlSchemaObject.cs
- JournalEntryListConverter.cs
- HwndHostAutomationPeer.cs
- ParsedAttributeCollection.cs
- ServiceReflector.cs
- GenericTypeParameterBuilder.cs
- ErrorFormatterPage.cs
- TextServicesProperty.cs
- Button.cs
- SqlReferenceCollection.cs
- QuadTree.cs
- EntityDataSourceMemberPath.cs
- TextServicesContext.cs
- Query.cs
- basecomparevalidator.cs
- PerformanceCounterPermissionEntry.cs
- PresentationTraceSources.cs
- Double.cs
- MD5HashHelper.cs
- COM2ExtendedTypeConverter.cs
- BaseWebProxyFinder.cs
- FileDialog.cs
- WebPartCollection.cs
- FacetEnabledSchemaElement.cs
- Matrix3D.cs
- EventItfInfo.cs
- PropertyMetadata.cs
- Ray3DHitTestResult.cs
- ResourcesBuildProvider.cs
- DocumentPageTextView.cs
- invalidudtexception.cs
- ManualResetEvent.cs
- SamlAssertion.cs
- SystemIPInterfaceProperties.cs
- MediaElement.cs
- ReturnEventArgs.cs
- RtType.cs
- COM2PropertyDescriptor.cs
- ActivationWorker.cs
- ProviderManager.cs
- LayoutInformation.cs
- PostBackOptions.cs
- AutoGeneratedFieldProperties.cs
- CodeTryCatchFinallyStatement.cs
- PathFigureCollectionConverter.cs
- FlowDocumentView.cs
- SafeArchiveContext.cs
- Converter.cs
- PaperSource.cs
- StateChangeEvent.cs
- DataTableClearEvent.cs
- BitmapEncoder.cs
- TheQuery.cs
- NamedPermissionSet.cs
- ListDataBindEventArgs.cs
- GridViewHeaderRowPresenter.cs
- MouseButtonEventArgs.cs
- EdmError.cs
- PropertyEmitter.cs
- DataSourceGeneratorException.cs
- ZipIOLocalFileHeader.cs
- NativeMethods.cs
- SqlUDTStorage.cs
- SymmetricCryptoHandle.cs
- AnnotationHighlightLayer.cs
- Validator.cs
- MiniLockedBorderGlyph.cs
- FrameworkEventSource.cs
- ItemsPresenter.cs
- OdbcCommand.cs
- DocumentViewerHelper.cs
- SqlCacheDependency.cs
- ComboBoxRenderer.cs
- DetailsView.cs
- HtmlEmptyTagControlBuilder.cs
- WebPartVerb.cs