Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / WebParts / ProxyWebPart.cs / 1 / ProxyWebPart.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Security.Permissions; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.Util; [ ToolboxItem(false) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class ProxyWebPart : WebPart { private string _originalID; private string _originalTypeName; private string _originalPath; private string _genericWebPartID; protected ProxyWebPart(WebPart webPart) { if (webPart == null) { throw new ArgumentNullException("webPart"); } GenericWebPart genericWebPart = webPart as GenericWebPart; if (genericWebPart != null) { Control childControl = genericWebPart.ChildControl; if (childControl == null) { throw new ArgumentException(SR.GetString( SR.PropertyCannotBeNull, "ChildControl"), "webPart"); } _originalID = childControl.ID; if (String.IsNullOrEmpty(_originalID)) { throw new ArgumentException(SR.GetString( SR.PropertyCannotBeNullOrEmptyString, "ChildControl.ID"), "webPart"); } Type originalType; UserControl childUserControl = childControl as UserControl; if (childUserControl != null) { originalType = typeof(UserControl); _originalPath = childUserControl.AppRelativeVirtualPath; } else { originalType = childControl.GetType(); } _originalTypeName = WebPartUtil.SerializeType(originalType); _genericWebPartID = genericWebPart.ID; if (String.IsNullOrEmpty(_genericWebPartID)) { throw new ArgumentException(SR.GetString( SR.PropertyCannotBeNullOrEmptyString, "ID"), "webPart"); } ID = _genericWebPartID; } else { _originalID = webPart.ID; if (String.IsNullOrEmpty(_originalID)) { throw new ArgumentException(SR.GetString( SR.PropertyCannotBeNullOrEmptyString, "ID"), "webPart"); } _originalTypeName = WebPartUtil.SerializeType(webPart.GetType()); ID = _originalID; } } protected ProxyWebPart(string originalID, string originalTypeName, string originalPath, string genericWebPartID) { if (String.IsNullOrEmpty(originalID)) { throw ExceptionUtil.ParameterNullOrEmpty("originalID"); } if (String.IsNullOrEmpty(originalTypeName)) { throw ExceptionUtil.ParameterNullOrEmpty("originalTypeName"); } // If you specify a path, this must be a GenericWebPart so genericWebPartID should not be null if (!String.IsNullOrEmpty(originalPath) && String.IsNullOrEmpty(genericWebPartID)) { throw ExceptionUtil.ParameterNullOrEmpty("genericWebPartID"); } _originalID = originalID; _originalTypeName = originalTypeName; _originalPath = originalPath; _genericWebPartID = genericWebPartID; if (!String.IsNullOrEmpty(genericWebPartID)) { ID = _genericWebPartID; } else { ID = _originalID; } } public string GenericWebPartID { get { return (_genericWebPartID != null) ? _genericWebPartID : String.Empty; } } // Seal the ID property so we can set it in the constructor without an FxCop violation. public sealed override string ID { get { return base.ID; } set { base.ID = value; } } public string OriginalID { get { return (_originalID != null) ? _originalID : String.Empty; } } public string OriginalTypeName { get { return (_originalTypeName != null) ? _originalTypeName : String.Empty; } } public string OriginalPath { get { return (_originalPath != null) ? _originalPath : String.Empty; } } // Accept any ControlState, but do nothing with it, since it is actually the ControlState // for the WebPart we are replacing. protected internal override void LoadControlState(object savedState) { } // Accept any ViewState, but do nothing with it, since it is actually the ViewState // for the WebPart we are replacing. protected override void LoadViewState(object savedState) { } // Do not save any ControlState, since the ProxyWebPart itself does not need to save state // between requests. protected internal override object SaveControlState() { // Call base in case it has some side-effects that the control relies on base.SaveControlState(); return null; } // Do not save any ViewState, since the ProxyWebPart itself does not need to save state // between requests. protected override object SaveViewState() { // Call base in case it has some side-effects that the control relies on base.SaveViewState(); return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Security.Permissions; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.Util; [ ToolboxItem(false) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class ProxyWebPart : WebPart { private string _originalID; private string _originalTypeName; private string _originalPath; private string _genericWebPartID; protected ProxyWebPart(WebPart webPart) { if (webPart == null) { throw new ArgumentNullException("webPart"); } GenericWebPart genericWebPart = webPart as GenericWebPart; if (genericWebPart != null) { Control childControl = genericWebPart.ChildControl; if (childControl == null) { throw new ArgumentException(SR.GetString( SR.PropertyCannotBeNull, "ChildControl"), "webPart"); } _originalID = childControl.ID; if (String.IsNullOrEmpty(_originalID)) { throw new ArgumentException(SR.GetString( SR.PropertyCannotBeNullOrEmptyString, "ChildControl.ID"), "webPart"); } Type originalType; UserControl childUserControl = childControl as UserControl; if (childUserControl != null) { originalType = typeof(UserControl); _originalPath = childUserControl.AppRelativeVirtualPath; } else { originalType = childControl.GetType(); } _originalTypeName = WebPartUtil.SerializeType(originalType); _genericWebPartID = genericWebPart.ID; if (String.IsNullOrEmpty(_genericWebPartID)) { throw new ArgumentException(SR.GetString( SR.PropertyCannotBeNullOrEmptyString, "ID"), "webPart"); } ID = _genericWebPartID; } else { _originalID = webPart.ID; if (String.IsNullOrEmpty(_originalID)) { throw new ArgumentException(SR.GetString( SR.PropertyCannotBeNullOrEmptyString, "ID"), "webPart"); } _originalTypeName = WebPartUtil.SerializeType(webPart.GetType()); ID = _originalID; } } protected ProxyWebPart(string originalID, string originalTypeName, string originalPath, string genericWebPartID) { if (String.IsNullOrEmpty(originalID)) { throw ExceptionUtil.ParameterNullOrEmpty("originalID"); } if (String.IsNullOrEmpty(originalTypeName)) { throw ExceptionUtil.ParameterNullOrEmpty("originalTypeName"); } // If you specify a path, this must be a GenericWebPart so genericWebPartID should not be null if (!String.IsNullOrEmpty(originalPath) && String.IsNullOrEmpty(genericWebPartID)) { throw ExceptionUtil.ParameterNullOrEmpty("genericWebPartID"); } _originalID = originalID; _originalTypeName = originalTypeName; _originalPath = originalPath; _genericWebPartID = genericWebPartID; if (!String.IsNullOrEmpty(genericWebPartID)) { ID = _genericWebPartID; } else { ID = _originalID; } } public string GenericWebPartID { get { return (_genericWebPartID != null) ? _genericWebPartID : String.Empty; } } // Seal the ID property so we can set it in the constructor without an FxCop violation. public sealed override string ID { get { return base.ID; } set { base.ID = value; } } public string OriginalID { get { return (_originalID != null) ? _originalID : String.Empty; } } public string OriginalTypeName { get { return (_originalTypeName != null) ? _originalTypeName : String.Empty; } } public string OriginalPath { get { return (_originalPath != null) ? _originalPath : String.Empty; } } // Accept any ControlState, but do nothing with it, since it is actually the ControlState // for the WebPart we are replacing. protected internal override void LoadControlState(object savedState) { } // Accept any ViewState, but do nothing with it, since it is actually the ViewState // for the WebPart we are replacing. protected override void LoadViewState(object savedState) { } // Do not save any ControlState, since the ProxyWebPart itself does not need to save state // between requests. protected internal override object SaveControlState() { // Call base in case it has some side-effects that the control relies on base.SaveControlState(); return null; } // Do not save any ViewState, since the ProxyWebPart itself does not need to save state // between requests. protected override object SaveViewState() { // Call base in case it has some side-effects that the control relies on base.SaveViewState(); return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
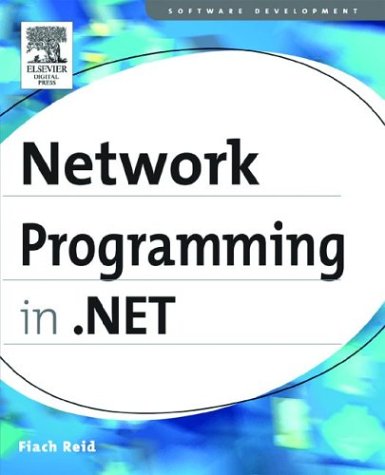
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringSource.cs
- QilInvokeEarlyBound.cs
- ComPlusThreadInitializer.cs
- WebPartTransformerAttribute.cs
- HttpCachePolicyElement.cs
- ExclusiveTcpListener.cs
- WebResourceAttribute.cs
- DateTimeSerializationSection.cs
- Rect3D.cs
- MemoryMappedViewStream.cs
- FillBehavior.cs
- AggregateNode.cs
- SizeKeyFrameCollection.cs
- ProcessModuleCollection.cs
- NetNamedPipeSecurity.cs
- XmlTextReaderImpl.cs
- HttpAsyncResult.cs
- DataControlFieldHeaderCell.cs
- VirtualPathUtility.cs
- RuleProcessor.cs
- ExpandCollapsePattern.cs
- SymbolEqualComparer.cs
- TableRowsCollectionEditor.cs
- DebugView.cs
- MessageSmuggler.cs
- SpeakCompletedEventArgs.cs
- IResourceProvider.cs
- IsolatedStorageFile.cs
- EpmContentDeSerializerBase.cs
- DesignerValidatorAdapter.cs
- DummyDataSource.cs
- TemporaryBitmapFile.cs
- MaterialGroup.cs
- UTF7Encoding.cs
- DropSourceBehavior.cs
- LocalFileSettingsProvider.cs
- ListDictionary.cs
- OpenFileDialog.cs
- ObjectDataSourceFilteringEventArgs.cs
- QfeChecker.cs
- DesignerCommandAdapter.cs
- DbModificationClause.cs
- HtmlTableCell.cs
- DataGridViewToolTip.cs
- CorrelationActionMessageFilter.cs
- CodeRegionDirective.cs
- LayoutInformation.cs
- ReflectTypeDescriptionProvider.cs
- Popup.cs
- NullableBoolConverter.cs
- ActivityTypeResolver.xaml.cs
- WeakReference.cs
- LazyLoadBehavior.cs
- PathData.cs
- UITypeEditor.cs
- ClipboardData.cs
- XamlPointCollectionSerializer.cs
- XmlSchemaImporter.cs
- MergePropertyDescriptor.cs
- _UncName.cs
- StrongNameUtility.cs
- RegistrationServices.cs
- DiscoveryClientRequestChannel.cs
- FormClosingEvent.cs
- CorrelationKeyCalculator.cs
- _IPv4Address.cs
- ParentControlDesigner.cs
- DataControlField.cs
- RegionData.cs
- StateMachineExecutionState.cs
- RelationshipEntry.cs
- WebPartZoneAutoFormat.cs
- ToggleProviderWrapper.cs
- Byte.cs
- XmlNamespaceManager.cs
- AccessibleObject.cs
- PersistenceTypeAttribute.cs
- HttpInputStream.cs
- ValidationResult.cs
- WebPartExportVerb.cs
- NullableDecimalMinMaxAggregationOperator.cs
- HashCodeCombiner.cs
- NullableIntAverageAggregationOperator.cs
- PointAnimationUsingPath.cs
- CodeSnippetTypeMember.cs
- ObjectDataSourceStatusEventArgs.cs
- ChannelPool.cs
- KnownBoxes.cs
- ConsumerConnectionPointCollection.cs
- Internal.cs
- ScrollBarRenderer.cs
- DelegateHelpers.cs
- Utils.cs
- DATA_BLOB.cs
- LookupBindingPropertiesAttribute.cs
- InputProviderSite.cs
- IdentifierService.cs
- ThrowHelper.cs
- StrongNameKeyPair.cs
- XmlDocumentFieldSchema.cs