Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / Design / UITypeEditor.cs / 1 / UITypeEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing.Design { using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Collections; using Microsoft.Win32; using System.ComponentModel.Design; using System.Drawing; using System.Collections.Generic; using System.Collections.ObjectModel; ////// /// [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class UITypeEditor { ///Provides a base class for editors /// that may provide users with a user interface to visually edit /// the values of the supported type or types. ////// /// In this static constructor we provide default UITypeEditors to /// the TypeDescriptor. /// static UITypeEditor() { Hashtable intrinsicEditors = new Hashtable(); // Our set of intrinsic editors. intrinsicEditors[typeof(DateTime)] = "System.ComponentModel.Design.DateTimeEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(Array)] = "System.ComponentModel.Design.ArrayEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(IList)] = "System.ComponentModel.Design.CollectionEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(ICollection)] = "System.ComponentModel.Design.CollectionEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(byte[])] = "System.ComponentModel.Design.BinaryEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(System.IO.Stream)] = "System.ComponentModel.Design.BinaryEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(string[])] = "System.Windows.Forms.Design.StringArrayEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(Collection)] = "System.Windows.Forms.Design.StringCollectionEditor, " + AssemblyRef.SystemDesign; // Add our intrinsic editors to TypeDescriptor. // TypeDescriptor.AddEditorTable(typeof(UITypeEditor), intrinsicEditors); } /// /// /// public UITypeEditor() { } ////// Initializes /// a new instance of the ///class. /// /// /// public virtual bool IsDropDownResizable { get { return false; } } ////// Determines if drop-down editors should be resizable by the user. /// ////// /// public object EditValue(IServiceProvider provider, object value) { return EditValue(null, provider, value); } ///Edits the specified value using the editor style /// provided by ///. /// /// public virtual object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { return value; } ///Edits the specified object's value using the editor style /// provided by ///. /// /// public UITypeEditorEditStyle GetEditStyle() { return GetEditStyle(null); } ////// Gets the ////// of the Edit method. /// /// /// public bool GetPaintValueSupported() { return GetPaintValueSupported(null); } ///Gets a value indicating whether this editor supports painting a representation /// of an object's value. ////// /// public virtual bool GetPaintValueSupported(ITypeDescriptorContext context) { return false; } ///Gets a value indicating whether the specified context supports painting a representation /// of an object's value. ////// /// public virtual UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.None; } ////// Gets the editing style of the Edit method. /// ////// /// public void PaintValue(object value, Graphics canvas, Rectangle rectangle) { PaintValue(new PaintValueEventArgs(null, value, canvas, rectangle)); } ///Paints a representative value of the specified object to the /// specified canvas. ////// /// [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] public virtual void PaintValue(PaintValueEventArgs e) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Paints a representative value of the specified object to the /// provided canvas. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing.Design { using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Collections; using Microsoft.Win32; using System.ComponentModel.Design; using System.Drawing; using System.Collections.Generic; using System.Collections.ObjectModel; ////// /// [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class UITypeEditor { ///Provides a base class for editors /// that may provide users with a user interface to visually edit /// the values of the supported type or types. ////// /// In this static constructor we provide default UITypeEditors to /// the TypeDescriptor. /// static UITypeEditor() { Hashtable intrinsicEditors = new Hashtable(); // Our set of intrinsic editors. intrinsicEditors[typeof(DateTime)] = "System.ComponentModel.Design.DateTimeEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(Array)] = "System.ComponentModel.Design.ArrayEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(IList)] = "System.ComponentModel.Design.CollectionEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(ICollection)] = "System.ComponentModel.Design.CollectionEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(byte[])] = "System.ComponentModel.Design.BinaryEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(System.IO.Stream)] = "System.ComponentModel.Design.BinaryEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(string[])] = "System.Windows.Forms.Design.StringArrayEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(Collection)] = "System.Windows.Forms.Design.StringCollectionEditor, " + AssemblyRef.SystemDesign; // Add our intrinsic editors to TypeDescriptor. // TypeDescriptor.AddEditorTable(typeof(UITypeEditor), intrinsicEditors); } /// /// /// public UITypeEditor() { } ////// Initializes /// a new instance of the ///class. /// /// /// public virtual bool IsDropDownResizable { get { return false; } } ////// Determines if drop-down editors should be resizable by the user. /// ////// /// public object EditValue(IServiceProvider provider, object value) { return EditValue(null, provider, value); } ///Edits the specified value using the editor style /// provided by ///. /// /// public virtual object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { return value; } ///Edits the specified object's value using the editor style /// provided by ///. /// /// public UITypeEditorEditStyle GetEditStyle() { return GetEditStyle(null); } ////// Gets the ////// of the Edit method. /// /// /// public bool GetPaintValueSupported() { return GetPaintValueSupported(null); } ///Gets a value indicating whether this editor supports painting a representation /// of an object's value. ////// /// public virtual bool GetPaintValueSupported(ITypeDescriptorContext context) { return false; } ///Gets a value indicating whether the specified context supports painting a representation /// of an object's value. ////// /// public virtual UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.None; } ////// Gets the editing style of the Edit method. /// ////// /// public void PaintValue(object value, Graphics canvas, Rectangle rectangle) { PaintValue(new PaintValueEventArgs(null, value, canvas, rectangle)); } ///Paints a representative value of the specified object to the /// specified canvas. ////// /// [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] public virtual void PaintValue(PaintValueEventArgs e) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Paints a representative value of the specified object to the /// provided canvas. ///
Link Menu
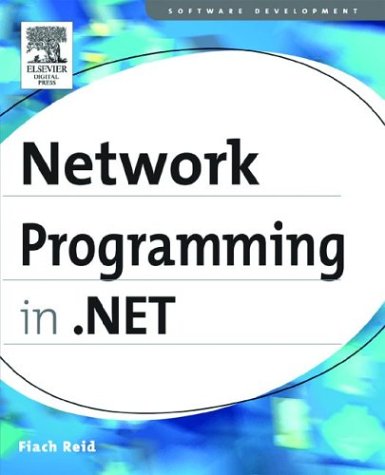
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DelegateTypeInfo.cs
- MobileCapabilities.cs
- UInt64Storage.cs
- BitmapScalingModeValidation.cs
- DocumentOutline.cs
- ServicesUtilities.cs
- RelationshipWrapper.cs
- SingleConverter.cs
- DataMemberFieldConverter.cs
- PackagePartCollection.cs
- SyndicationDeserializer.cs
- SessionIDManager.cs
- AttachedAnnotationChangedEventArgs.cs
- DocumentPaginator.cs
- LineBreakRecord.cs
- WebScriptServiceHost.cs
- OleCmdHelper.cs
- Environment.cs
- IsolatedStorage.cs
- PreApplicationStartMethodAttribute.cs
- OSFeature.cs
- WithStatement.cs
- Matrix3D.cs
- StyleHelper.cs
- ScriptingWebServicesSectionGroup.cs
- SocketAddress.cs
- ResXResourceWriter.cs
- TypeReference.cs
- ProxyGenerator.cs
- StringUtil.cs
- WsatServiceCertificate.cs
- NamespaceListProperty.cs
- DBDataPermissionAttribute.cs
- ExceptionAggregator.cs
- InstanceOwnerQueryResult.cs
- TextBlock.cs
- SelectionItemPattern.cs
- MsmqBindingBase.cs
- NavigationProgressEventArgs.cs
- StateChangeEvent.cs
- NamedPipeAppDomainProtocolHandler.cs
- ForceCopyBuildProvider.cs
- ObjectReaderCompiler.cs
- TemplateField.cs
- ScrollProperties.cs
- LogicalExpr.cs
- BuildProviderCollection.cs
- SQLResource.cs
- Atom10ItemFormatter.cs
- URIFormatException.cs
- ItemsControl.cs
- TableLayoutPanelCellPosition.cs
- DeviceContext2.cs
- DataViewManager.cs
- SimpleWebHandlerParser.cs
- GenericEnumConverter.cs
- ValidationSummary.cs
- TextBox.cs
- CharacterBuffer.cs
- ConfigurationSchemaErrors.cs
- TemplateColumn.cs
- WebPartActionVerb.cs
- TableRow.cs
- XmlRawWriterWrapper.cs
- ReadOnlyTernaryTree.cs
- MessageQueueAccessControlEntry.cs
- DropShadowEffect.cs
- DesignerAttribute.cs
- TreeViewImageKeyConverter.cs
- MenuItemBindingCollection.cs
- SchemaManager.cs
- SqlDataSourceCommandEventArgs.cs
- PropertyTabAttribute.cs
- objectresult_tresulttype.cs
- EntityDataSourceEntityTypeFilterItem.cs
- ControlPropertyNameConverter.cs
- DynamicMethod.cs
- DateTimeUtil.cs
- HttpCookiesSection.cs
- LoginDesigner.cs
- OpenFileDialog.cs
- IPPacketInformation.cs
- OrderedEnumerableRowCollection.cs
- DbSetClause.cs
- AnnotationMap.cs
- DataGridViewRowEventArgs.cs
- ConfigurationElementCollection.cs
- TTSVoice.cs
- SettingsSection.cs
- OdbcParameterCollection.cs
- HtmlInputButton.cs
- RegexCapture.cs
- RightsManagementInformation.cs
- HttpListenerRequest.cs
- OletxDependentTransaction.cs
- _SslSessionsCache.cs
- FontCollection.cs
- SystemParameters.cs
- SingleStorage.cs
- ButtonBase.cs