Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Objects / RelationshipWrapper.cs / 1 / RelationshipWrapper.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Data.Metadata.Edm; namespace System.Data.Objects { internal sealed class RelationshipWrapper : IEquatable{ internal readonly AssociationSet AssociationSet; internal readonly EntityKey Key0; internal readonly EntityKey Key1; internal RelationshipWrapper(AssociationSet extent, EntityKey key) { Debug.Assert(null != extent, "null RelationshipWrapper"); Debug.Assert(null != (object)key, "null key"); this.AssociationSet = extent; this.Key0 = key; this.Key1 = key; } internal RelationshipWrapper(RelationshipWrapper wrapper, int ordinal, EntityKey key) { Debug.Assert(null != wrapper, "null RelationshipWrapper"); Debug.Assert((uint)ordinal <= 1u, "ordinal out of range"); Debug.Assert(null != (object)key, "null key2"); this.AssociationSet = wrapper.AssociationSet; this.Key0 = (0 == ordinal) ? key : wrapper.Key0; this.Key1 = (0 == ordinal) ? wrapper.Key1 : key; } internal RelationshipWrapper(AssociationSet extent, KeyValuePair roleAndKey1, KeyValuePair roleAndKey2) : this(extent, roleAndKey1.Key, roleAndKey1.Value, roleAndKey2.Key, roleAndKey2.Value) { } internal RelationshipWrapper(AssociationSet extent, string role0, EntityKey key0, string role1, EntityKey key1) { Debug.Assert(null != extent, "null AssociationSet"); Debug.Assert(null != (object)key0, "null key0"); Debug.Assert(null != (object)key1, "null key1"); AssociationSet = extent; Debug.Assert(extent.ElementType.AssociationEndMembers.Count == 2, "only 2 ends are supported"); // this assert is explictly commented out to show that the two are similar but different // we should always use AssociationEndMembers, never CorrespondingAssociationEndMember //Debug.Assert(AssociationSet.AssociationSetEnds.Count == 2, "only 2 set ends supported"); //Debug.Assert(extent.ElementType.AssociationEndMembers[0] == AssociationSet.AssociationSetEnds[0].CorrespondingAssociationEndMember, "should be same end member"); //Debug.Assert(extent.ElementType.AssociationEndMembers[1] == AssociationSet.AssociationSetEnds[1].CorrespondingAssociationEndMember, "should be same end member"); if (extent.ElementType.AssociationEndMembers[0].Name == role0) { Debug.Assert(extent.ElementType.AssociationEndMembers[1].Name == role1, "a)roleAndKey1 Name differs"); Key0 = key0; Key1 = key1; } else { Debug.Assert(extent.ElementType.AssociationEndMembers[0].Name == role1, "b)roleAndKey1 Name differs"); Debug.Assert(extent.ElementType.AssociationEndMembers[1].Name == role0, "b)roleAndKey0 Name differs"); Key0 = key1; Key1 = key0; } } internal ReadOnlyMetadataCollection AssociationEndMembers { get { return this.AssociationSet.ElementType.AssociationEndMembers; } } internal AssociationEndMember GetAssociationEndMember(EntityKey key) { Debug.Assert(Key0 == key || Key1 == key, "didn't match a key"); return AssociationEndMembers[(Key0 != key) ? 1 : 0]; } internal EntityKey GetOtherEntityKey(EntityKey key) { return ((Key0 == key) ? Key1 : ((Key1 == key) ? Key0 : null)); } internal EntityKey GetEntityKey(int ordinal) { switch (ordinal) { case 0: return Key0; case 1: return Key1; default: throw EntityUtil.ArgumentOutOfRange("ordinal"); } } public override int GetHashCode() { return this.AssociationSet.Name.GetHashCode() ^ (this.Key0.GetHashCode() + this.Key1.GetHashCode()); } public override bool Equals(object obj) { return Equals(obj as RelationshipWrapper); } public bool Equals(RelationshipWrapper wrapper) { return (Object.ReferenceEquals(this, wrapper) || ((null != wrapper) && Object.ReferenceEquals(this.AssociationSet, wrapper.AssociationSet) && this.Key0.Equals(wrapper.Key0) && this.Key1.Equals(wrapper.Key1))); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Data.Metadata.Edm; namespace System.Data.Objects { internal sealed class RelationshipWrapper : IEquatable{ internal readonly AssociationSet AssociationSet; internal readonly EntityKey Key0; internal readonly EntityKey Key1; internal RelationshipWrapper(AssociationSet extent, EntityKey key) { Debug.Assert(null != extent, "null RelationshipWrapper"); Debug.Assert(null != (object)key, "null key"); this.AssociationSet = extent; this.Key0 = key; this.Key1 = key; } internal RelationshipWrapper(RelationshipWrapper wrapper, int ordinal, EntityKey key) { Debug.Assert(null != wrapper, "null RelationshipWrapper"); Debug.Assert((uint)ordinal <= 1u, "ordinal out of range"); Debug.Assert(null != (object)key, "null key2"); this.AssociationSet = wrapper.AssociationSet; this.Key0 = (0 == ordinal) ? key : wrapper.Key0; this.Key1 = (0 == ordinal) ? wrapper.Key1 : key; } internal RelationshipWrapper(AssociationSet extent, KeyValuePair roleAndKey1, KeyValuePair roleAndKey2) : this(extent, roleAndKey1.Key, roleAndKey1.Value, roleAndKey2.Key, roleAndKey2.Value) { } internal RelationshipWrapper(AssociationSet extent, string role0, EntityKey key0, string role1, EntityKey key1) { Debug.Assert(null != extent, "null AssociationSet"); Debug.Assert(null != (object)key0, "null key0"); Debug.Assert(null != (object)key1, "null key1"); AssociationSet = extent; Debug.Assert(extent.ElementType.AssociationEndMembers.Count == 2, "only 2 ends are supported"); // this assert is explictly commented out to show that the two are similar but different // we should always use AssociationEndMembers, never CorrespondingAssociationEndMember //Debug.Assert(AssociationSet.AssociationSetEnds.Count == 2, "only 2 set ends supported"); //Debug.Assert(extent.ElementType.AssociationEndMembers[0] == AssociationSet.AssociationSetEnds[0].CorrespondingAssociationEndMember, "should be same end member"); //Debug.Assert(extent.ElementType.AssociationEndMembers[1] == AssociationSet.AssociationSetEnds[1].CorrespondingAssociationEndMember, "should be same end member"); if (extent.ElementType.AssociationEndMembers[0].Name == role0) { Debug.Assert(extent.ElementType.AssociationEndMembers[1].Name == role1, "a)roleAndKey1 Name differs"); Key0 = key0; Key1 = key1; } else { Debug.Assert(extent.ElementType.AssociationEndMembers[0].Name == role1, "b)roleAndKey1 Name differs"); Debug.Assert(extent.ElementType.AssociationEndMembers[1].Name == role0, "b)roleAndKey0 Name differs"); Key0 = key1; Key1 = key0; } } internal ReadOnlyMetadataCollection AssociationEndMembers { get { return this.AssociationSet.ElementType.AssociationEndMembers; } } internal AssociationEndMember GetAssociationEndMember(EntityKey key) { Debug.Assert(Key0 == key || Key1 == key, "didn't match a key"); return AssociationEndMembers[(Key0 != key) ? 1 : 0]; } internal EntityKey GetOtherEntityKey(EntityKey key) { return ((Key0 == key) ? Key1 : ((Key1 == key) ? Key0 : null)); } internal EntityKey GetEntityKey(int ordinal) { switch (ordinal) { case 0: return Key0; case 1: return Key1; default: throw EntityUtil.ArgumentOutOfRange("ordinal"); } } public override int GetHashCode() { return this.AssociationSet.Name.GetHashCode() ^ (this.Key0.GetHashCode() + this.Key1.GetHashCode()); } public override bool Equals(object obj) { return Equals(obj as RelationshipWrapper); } public bool Equals(RelationshipWrapper wrapper) { return (Object.ReferenceEquals(this, wrapper) || ((null != wrapper) && Object.ReferenceEquals(this.AssociationSet, wrapper.AssociationSet) && this.Key0.Equals(wrapper.Key0) && this.Key1.Equals(wrapper.Key1))); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
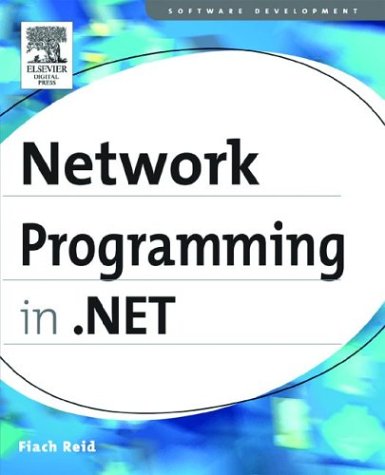
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Serializer.cs
- ImageFormatConverter.cs
- DataSourceView.cs
- VerticalAlignConverter.cs
- HtmlSelect.cs
- PropertyBuilder.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- DiscoveryVersion.cs
- JapaneseLunisolarCalendar.cs
- InternalsVisibleToAttribute.cs
- DataListItemEventArgs.cs
- Decoder.cs
- CellConstant.cs
- HttpCachePolicy.cs
- XmlDataSourceNodeDescriptor.cs
- Rect3D.cs
- ClassGenerator.cs
- Crc32Helper.cs
- SpeechDetectedEventArgs.cs
- XmlCharCheckingWriter.cs
- QueryActivatableWorkflowsCommand.cs
- FunctionImportElement.cs
- TextLineResult.cs
- WindowsRegion.cs
- XmlResolver.cs
- ResourceManager.cs
- QueryableDataSource.cs
- ClientSideQueueItem.cs
- InternalConfigHost.cs
- EventProviderWriter.cs
- LinkedList.cs
- QuestionEventArgs.cs
- ScrollBar.cs
- ReferenceConverter.cs
- EventTrigger.cs
- FrameworkContentElementAutomationPeer.cs
- GroupBoxRenderer.cs
- StyleBamlTreeBuilder.cs
- util.cs
- ResourceDescriptionAttribute.cs
- DragStartedEventArgs.cs
- CatalogPartChrome.cs
- CustomCategoryAttribute.cs
- NamespaceDisplay.xaml.cs
- ConfigsHelper.cs
- CriticalHandle.cs
- TreeView.cs
- StructuralCache.cs
- CollectionDataContractAttribute.cs
- IsolatedStorageFile.cs
- ComPlusSynchronizationContext.cs
- OdbcTransaction.cs
- SchemaImporterExtensionElement.cs
- MessageQueue.cs
- TransactionsSectionGroup.cs
- TextEditorCharacters.cs
- BatchStream.cs
- TypeSystem.cs
- DynamicDataRoute.cs
- ApplicationException.cs
- Int16Converter.cs
- TypeDescriptorFilterService.cs
- EdmType.cs
- FixedSOMPageConstructor.cs
- TextOnlyOutput.cs
- MgmtResManager.cs
- LayoutEngine.cs
- ToolStripSeparator.cs
- SeverityFilter.cs
- PlatformNotSupportedException.cs
- DES.cs
- MediaSystem.cs
- Validator.cs
- HostingEnvironmentSection.cs
- ReferencedAssembly.cs
- WindowsEditBoxRange.cs
- PageRequestManager.cs
- ResXDataNode.cs
- SettingsPropertyValueCollection.cs
- FileLogRecordHeader.cs
- TabPage.cs
- GridEntryCollection.cs
- EntityDataSourceColumn.cs
- XmlNavigatorStack.cs
- XmlNamespaceMapping.cs
- ClientTargetCollection.cs
- ErrorStyle.cs
- HTTPNotFoundHandler.cs
- StringValidatorAttribute.cs
- CSharpCodeProvider.cs
- ViewPort3D.cs
- HTMLTagNameToTypeMapper.cs
- RoutedEventArgs.cs
- LogArchiveSnapshot.cs
- AuthorizationRuleCollection.cs
- StorageScalarPropertyMapping.cs
- ScrollEventArgs.cs
- WebPartDescriptionCollection.cs
- ParserStreamGeometryContext.cs
- XmlNamespaceMapping.cs