Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / Util / TaskForm.cs / 1 / TaskForm.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.Util { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Drawing; using System.Windows.Forms; using System.Windows.Forms.Design; ////// Represents a wizard used to guide users through configuration processes. /// internal abstract class TaskForm : TaskFormBase { private System.Windows.Forms.Button _okButton; private System.Windows.Forms.Label _dummyLabel1; private System.Windows.Forms.Button _cancelButton; private System.Windows.Forms.TableLayoutPanel _dialogButtonsTableLayoutPanel; ////// Creates a new TaskForm with a given service provider. /// public TaskForm(IServiceProvider serviceProvider) : base(serviceProvider) { InitializeComponent(); InitializeUI(); } protected Button OKButton { get { return _okButton; } } #region Designer generated code ////// Required method for Designer support - do not modify /// the contents of this method with the code editor. /// private void InitializeComponent() { this._dialogButtonsTableLayoutPanel = new System.Windows.Forms.TableLayoutPanel(); this._okButton = new System.Windows.Forms.Button(); this._cancelButton = new System.Windows.Forms.Button(); this._dummyLabel1 = new System.Windows.Forms.Label(); this._dialogButtonsTableLayoutPanel.SuspendLayout(); this.SuspendLayout(); // // _dialogButtonsTableLayoutPanel // this._dialogButtonsTableLayoutPanel.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Bottom | System.Windows.Forms.AnchorStyles.Right))); this._dialogButtonsTableLayoutPanel.AutoSize = true; this._dialogButtonsTableLayoutPanel.ColumnCount = 3; this._dialogButtonsTableLayoutPanel.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Percent, 50F)); this._dialogButtonsTableLayoutPanel.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Absolute, 6F)); this._dialogButtonsTableLayoutPanel.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Percent, 50F)); this._dialogButtonsTableLayoutPanel.Controls.Add(this._okButton); this._dialogButtonsTableLayoutPanel.Controls.Add(this._dummyLabel1); this._dialogButtonsTableLayoutPanel.Controls.Add(this._cancelButton); this._dialogButtonsTableLayoutPanel.Location = new System.Drawing.Point(404, 381); this._dialogButtonsTableLayoutPanel.Name = "_dialogButtonsTableLayoutPanel"; this._dialogButtonsTableLayoutPanel.RowStyles.Add(new System.Windows.Forms.RowStyle()); this._dialogButtonsTableLayoutPanel.Size = new System.Drawing.Size(156, 23); this._dialogButtonsTableLayoutPanel.TabIndex = 100; // // _okButton // this._okButton.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Left | System.Windows.Forms.AnchorStyles.Right))); this._okButton.AutoSize = true; this._okButton.DialogResult = System.Windows.Forms.DialogResult.OK; this._okButton.Enabled = false; this._okButton.Location = new System.Drawing.Point(0, 0); this._okButton.Margin = new System.Windows.Forms.Padding(0); this._okButton.MinimumSize = new System.Drawing.Size(75, 23); this._okButton.Name = "_okButton"; this._okButton.TabIndex = 50; this._okButton.Click += new System.EventHandler(this.OnOKButtonClick); // // _dummyLabel1 // this._dummyLabel1.Location = new System.Drawing.Point(75, 0); this._dummyLabel1.Margin = new System.Windows.Forms.Padding(0); this._dummyLabel1.Name = "_dummyLabel1"; this._dummyLabel1.Size = new System.Drawing.Size(6, 0); this._dummyLabel1.TabIndex = 20; // // _cancelButton // this._cancelButton.Anchor = ((System.Windows.Forms.AnchorStyles)((System.Windows.Forms.AnchorStyles.Left | System.Windows.Forms.AnchorStyles.Right))); this._cancelButton.AutoSize = true; this._cancelButton.DialogResult = System.Windows.Forms.DialogResult.Cancel; this._cancelButton.Location = new System.Drawing.Point(81, 0); this._cancelButton.Margin = new System.Windows.Forms.Padding(0); this._cancelButton.MinimumSize = new System.Drawing.Size(75, 23); this._cancelButton.Name = "_cancelButton"; this._cancelButton.TabIndex = 70; this._cancelButton.Click += new System.EventHandler(this.OnCancelButtonClick); // // TaskForm // this.AcceptButton = this._okButton; this.FormBorderStyle = FormBorderStyle.FixedDialog; this.CancelButton = this._cancelButton; this.Controls.Add(this._dialogButtonsTableLayoutPanel); this._dialogButtonsTableLayoutPanel.ResumeLayout(false); this._dialogButtonsTableLayoutPanel.PerformLayout(); this.ResumeLayout(false); this.PerformLayout(); } #endregion ////// Called after InitializeComponent to perform additional actions that /// are not supported by the designer. /// private void InitializeUI() { _cancelButton.Text = SR.GetString(SR.Wizard_CancelButton); _okButton.Text = SR.GetString(SR.OKCaption); } ////// Click event handler for the Cancel button. /// protected virtual void OnCancelButtonClick(object sender, System.EventArgs e) { DialogResult = DialogResult.Cancel; Close(); } ////// Click event handler for the OK button. /// protected virtual void OnOKButtonClick(object sender, System.EventArgs e) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
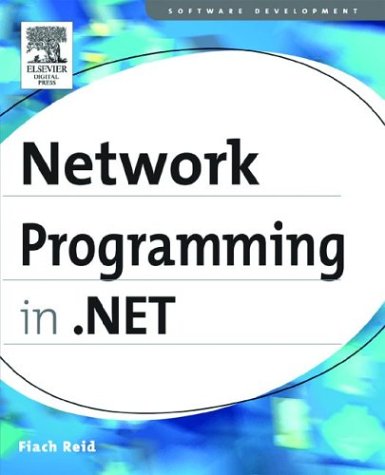
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlBulkCopyColumnMapping.cs
- UserControlCodeDomTreeGenerator.cs
- UserMapPath.cs
- WebPartUtil.cs
- KerberosSecurityTokenProvider.cs
- SharedStatics.cs
- LockedHandleGlyph.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- SizeChangedInfo.cs
- DataGridViewRowConverter.cs
- CustomValidator.cs
- ItemList.cs
- PropVariant.cs
- InvalidDocumentContentsException.cs
- Decorator.cs
- DeviceContext.cs
- AdRotator.cs
- TypeConverter.cs
- DtdParser.cs
- HostingEnvironmentException.cs
- TraceListeners.cs
- XmlQueryRuntime.cs
- SafeRightsManagementEnvironmentHandle.cs
- RegisteredExpandoAttribute.cs
- DocumentSchemaValidator.cs
- _ProxyChain.cs
- ModelItemKeyValuePair.cs
- ObjectManager.cs
- ElementHostAutomationPeer.cs
- DbParameterHelper.cs
- ConfigurationValidatorBase.cs
- EmptyEnumerator.cs
- StringCollection.cs
- StaticSiteMapProvider.cs
- SystemEvents.cs
- MimeTypeMapper.cs
- XmlDataDocument.cs
- MessageSmuggler.cs
- InternalSafeNativeMethods.cs
- ObjectSet.cs
- ItemCollection.cs
- PrivilegeNotHeldException.cs
- PipelineModuleStepContainer.cs
- SinglePageViewer.cs
- TreeNodeSelectionProcessor.cs
- AudioSignalProblemOccurredEventArgs.cs
- DiscardableAttribute.cs
- NumberSubstitution.cs
- BaseAddressElementCollection.cs
- PackagePart.cs
- SqlConnectionHelper.cs
- GPPOINT.cs
- Rotation3D.cs
- OrderedDictionaryStateHelper.cs
- ElementHostAutomationPeer.cs
- XmlTextWriter.cs
- XPathQilFactory.cs
- ManagementClass.cs
- EdmEntityTypeAttribute.cs
- PageParser.cs
- TrackingQuery.cs
- HandleRef.cs
- TypeDependencyAttribute.cs
- SerializationEventsCache.cs
- MatrixAnimationUsingKeyFrames.cs
- InputScopeNameConverter.cs
- QilInvokeLateBound.cs
- BamlLocalizationDictionary.cs
- DesignerActionGlyph.cs
- SoapSchemaExporter.cs
- AudioSignalProblemOccurredEventArgs.cs
- Listen.cs
- TreeBuilderXamlTranslator.cs
- Token.cs
- PanelDesigner.cs
- BinaryMessageEncoder.cs
- SoapServerMessage.cs
- UserControl.cs
- AutoResetEvent.cs
- TreeViewEvent.cs
- XmlSchemaExternal.cs
- ErrorTableItemStyle.cs
- RequestTimeoutManager.cs
- EntityWithKeyStrategy.cs
- SqlInfoMessageEvent.cs
- Registry.cs
- PropertyInfoSet.cs
- SqlServices.cs
- DataGridViewTextBoxColumn.cs
- ObjectStateEntry.cs
- WaitHandleCannotBeOpenedException.cs
- ModifierKeysValueSerializer.cs
- _AutoWebProxyScriptHelper.cs
- RowBinding.cs
- EmptyEnumerator.cs
- SecurityElement.cs
- UriTemplateLiteralQueryValue.cs
- StylusSystemGestureEventArgs.cs
- MessageVersionConverter.cs
- XsltLibrary.cs