Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Generated / TextDecoration.cs / 1 / TextDecoration.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal.PresentationCore; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Converters; using MS.Internal.Collections; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows { sealed partial class TextDecoration : Animatable { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new TextDecoration Clone() { return (TextDecoration)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new TextDecoration CloneCurrentValue() { return (TextDecoration)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Pen - Pen. Default value is null. /// The pen used to draw the text decoration /// public Pen Pen { get { return (Pen) GetValue(PenProperty); } set { SetValueInternal(PenProperty, value); } } ////// PenOffset - double. Default value is 0.0. /// The offset of the text decoration to the location specified. /// public double PenOffset { get { return (double) GetValue(PenOffsetProperty); } set { SetValueInternal(PenOffsetProperty, value); } } ////// PenOffsetUnit - TextDecorationUnit. Default value is TextDecorationUnit.FontRecommended. /// The unit type we use to interpret the offset value. /// public TextDecorationUnit PenOffsetUnit { get { return (TextDecorationUnit) GetValue(PenOffsetUnitProperty); } set { SetValueInternal(PenOffsetUnitProperty, value); } } ////// PenThicknessUnit - TextDecorationUnit. Default value is TextDecorationUnit.FontRecommended. /// The unit type we use to interpret the thickness value. /// public TextDecorationUnit PenThicknessUnit { get { return (TextDecorationUnit) GetValue(PenThicknessUnitProperty); } set { SetValueInternal(PenThicknessUnitProperty, value); } } ////// Location - TextDecorationLocation. Default value is TextDecorationLocation.Underline. /// The Location of the text decorations /// public TextDecorationLocation Location { get { return (TextDecorationLocation) GetValue(LocationProperty); } set { SetValueInternal(LocationProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new TextDecoration(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the TextDecoration.Pen property. /// public static readonly DependencyProperty PenProperty; ////// The DependencyProperty for the TextDecoration.PenOffset property. /// public static readonly DependencyProperty PenOffsetProperty; ////// The DependencyProperty for the TextDecoration.PenOffsetUnit property. /// public static readonly DependencyProperty PenOffsetUnitProperty; ////// The DependencyProperty for the TextDecoration.PenThicknessUnit property. /// public static readonly DependencyProperty PenThicknessUnitProperty; ////// The DependencyProperty for the TextDecoration.Location property. /// public static readonly DependencyProperty LocationProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const double c_PenOffset = 0.0; internal const TextDecorationUnit c_PenOffsetUnit = TextDecorationUnit.FontRecommended; internal const TextDecorationUnit c_PenThicknessUnit = TextDecorationUnit.FontRecommended; internal const TextDecorationLocation c_Location = TextDecorationLocation.Underline; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static TextDecoration() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(TextDecoration); PenProperty = RegisterProperty("Pen", typeof(Pen), typeofThis, null, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); PenOffsetProperty = RegisterProperty("PenOffset", typeof(double), typeofThis, 0.0, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); PenOffsetUnitProperty = RegisterProperty("PenOffsetUnit", typeof(TextDecorationUnit), typeofThis, TextDecorationUnit.FontRecommended, null, new ValidateValueCallback(System.Windows.ValidateEnums.IsTextDecorationUnitValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); PenThicknessUnitProperty = RegisterProperty("PenThicknessUnit", typeof(TextDecorationUnit), typeofThis, TextDecorationUnit.FontRecommended, null, new ValidateValueCallback(System.Windows.ValidateEnums.IsTextDecorationUnitValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); LocationProperty = RegisterProperty("Location", typeof(TextDecorationLocation), typeofThis, TextDecorationLocation.Underline, null, new ValidateValueCallback(System.Windows.ValidateEnums.IsTextDecorationLocationValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal.PresentationCore; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Converters; using MS.Internal.Collections; using MS.Utility; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows { sealed partial class TextDecoration : Animatable { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new TextDecoration Clone() { return (TextDecoration)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new TextDecoration CloneCurrentValue() { return (TextDecoration)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Pen - Pen. Default value is null. /// The pen used to draw the text decoration /// public Pen Pen { get { return (Pen) GetValue(PenProperty); } set { SetValueInternal(PenProperty, value); } } ////// PenOffset - double. Default value is 0.0. /// The offset of the text decoration to the location specified. /// public double PenOffset { get { return (double) GetValue(PenOffsetProperty); } set { SetValueInternal(PenOffsetProperty, value); } } ////// PenOffsetUnit - TextDecorationUnit. Default value is TextDecorationUnit.FontRecommended. /// The unit type we use to interpret the offset value. /// public TextDecorationUnit PenOffsetUnit { get { return (TextDecorationUnit) GetValue(PenOffsetUnitProperty); } set { SetValueInternal(PenOffsetUnitProperty, value); } } ////// PenThicknessUnit - TextDecorationUnit. Default value is TextDecorationUnit.FontRecommended. /// The unit type we use to interpret the thickness value. /// public TextDecorationUnit PenThicknessUnit { get { return (TextDecorationUnit) GetValue(PenThicknessUnitProperty); } set { SetValueInternal(PenThicknessUnitProperty, value); } } ////// Location - TextDecorationLocation. Default value is TextDecorationLocation.Underline. /// The Location of the text decorations /// public TextDecorationLocation Location { get { return (TextDecorationLocation) GetValue(LocationProperty); } set { SetValueInternal(LocationProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new TextDecoration(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the TextDecoration.Pen property. /// public static readonly DependencyProperty PenProperty; ////// The DependencyProperty for the TextDecoration.PenOffset property. /// public static readonly DependencyProperty PenOffsetProperty; ////// The DependencyProperty for the TextDecoration.PenOffsetUnit property. /// public static readonly DependencyProperty PenOffsetUnitProperty; ////// The DependencyProperty for the TextDecoration.PenThicknessUnit property. /// public static readonly DependencyProperty PenThicknessUnitProperty; ////// The DependencyProperty for the TextDecoration.Location property. /// public static readonly DependencyProperty LocationProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const double c_PenOffset = 0.0; internal const TextDecorationUnit c_PenOffsetUnit = TextDecorationUnit.FontRecommended; internal const TextDecorationUnit c_PenThicknessUnit = TextDecorationUnit.FontRecommended; internal const TextDecorationLocation c_Location = TextDecorationLocation.Underline; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static TextDecoration() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(TextDecoration); PenProperty = RegisterProperty("Pen", typeof(Pen), typeofThis, null, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); PenOffsetProperty = RegisterProperty("PenOffset", typeof(double), typeofThis, 0.0, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); PenOffsetUnitProperty = RegisterProperty("PenOffsetUnit", typeof(TextDecorationUnit), typeofThis, TextDecorationUnit.FontRecommended, null, new ValidateValueCallback(System.Windows.ValidateEnums.IsTextDecorationUnitValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); PenThicknessUnitProperty = RegisterProperty("PenThicknessUnit", typeof(TextDecorationUnit), typeofThis, TextDecorationUnit.FontRecommended, null, new ValidateValueCallback(System.Windows.ValidateEnums.IsTextDecorationUnitValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); LocationProperty = RegisterProperty("Location", typeof(TextDecorationLocation), typeofThis, TextDecorationLocation.Underline, null, new ValidateValueCallback(System.Windows.ValidateEnums.IsTextDecorationLocationValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
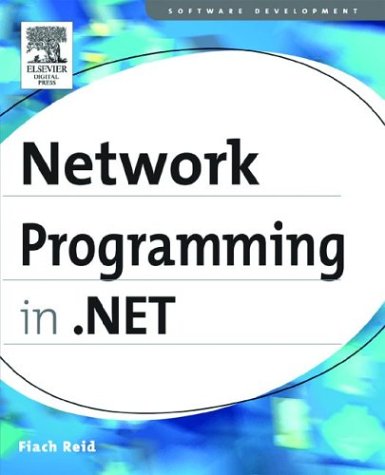
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Comparer.cs
- HttpCachePolicy.cs
- NameValuePermission.cs
- MappingException.cs
- ActivationArguments.cs
- UseAttributeSetsAction.cs
- XamlLoadErrorInfo.cs
- SerializationAttributes.cs
- ListViewItem.cs
- ContractNamespaceAttribute.cs
- AxHost.cs
- TabControlEvent.cs
- TextTreeTextElementNode.cs
- DataFormats.cs
- XmlSerializationWriter.cs
- GeneralTransform3DGroup.cs
- ThicknessAnimation.cs
- HttpFileCollection.cs
- XamlGridLengthSerializer.cs
- ComplexObject.cs
- EUCJPEncoding.cs
- IDataContractSurrogate.cs
- PathGeometry.cs
- FunctionParameter.cs
- SizeConverter.cs
- DataGridViewHeaderCell.cs
- ConsoleCancelEventArgs.cs
- _LocalDataStore.cs
- WebPartHelpVerb.cs
- SystemDiagnosticsSection.cs
- SimpleFieldTemplateFactory.cs
- ProfilePropertySettingsCollection.cs
- WpfWebRequestHelper.cs
- ZipIOExtraField.cs
- OleDbEnumerator.cs
- DataGridColumn.cs
- ExpressionBuilderContext.cs
- FormViewDeletedEventArgs.cs
- AnimationTimeline.cs
- TemplateBuilder.cs
- RuleSetDialog.cs
- MonitorWrapper.cs
- TextFormatterImp.cs
- SslStreamSecurityUpgradeProvider.cs
- OneWayChannelListener.cs
- X509SecurityTokenAuthenticator.cs
- WpfWebRequestHelper.cs
- NativeMethods.cs
- IdentitySection.cs
- MetadataCacheItem.cs
- UnauthorizedWebPart.cs
- CurrentChangedEventManager.cs
- Token.cs
- Point3DCollectionConverter.cs
- ObjectSecurity.cs
- HtmlInputHidden.cs
- ReaderWriterLock.cs
- Container.cs
- RefreshPropertiesAttribute.cs
- PasswordRecoveryDesigner.cs
- Icon.cs
- ViewgenContext.cs
- Panel.cs
- Latin1Encoding.cs
- ColorIndependentAnimationStorage.cs
- XPathDescendantIterator.cs
- ModuleConfigurationInfo.cs
- ServiceModelInstallComponent.cs
- ASCIIEncoding.cs
- GridViewDeletedEventArgs.cs
- XPathScanner.cs
- WindowsTitleBar.cs
- ObjectConverter.cs
- WindowsListViewGroup.cs
- HtmlInputRadioButton.cs
- DataObjectAttribute.cs
- ArgumentNullException.cs
- PriorityQueue.cs
- HtmlTable.cs
- OuterGlowBitmapEffect.cs
- ScrollableControl.cs
- ResourcePermissionBase.cs
- XmlRawWriter.cs
- XmlDataSourceView.cs
- LogConverter.cs
- XmlSignificantWhitespace.cs
- DispatcherHookEventArgs.cs
- FormsAuthenticationTicket.cs
- EpmSyndicationContentSerializer.cs
- DataGridViewComboBoxColumn.cs
- DataGridItemEventArgs.cs
- AttachedProperty.cs
- BodyGlyph.cs
- CaseInsensitiveHashCodeProvider.cs
- WorkflowRuntimeSection.cs
- CorrelationManager.cs
- SystemPens.cs
- BackgroundWorker.cs
- SafeCancelMibChangeNotify.cs
- ClusterUtils.cs