Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / _LocalDataStore.cs / 1 / _LocalDataStore.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: LocalDataStore ** ** ** Purpose: Class that stores local data. This class is used in cooperation ** with the _LocalDataStoreMgr class. ** ** =============================================================================*/ namespace System { using System; using System.Threading; using System.Runtime.CompilerServices; // This class will not be marked serializable internal class LocalDataStore { /*========================================================================= ** DON'T CHANGE THESE UNLESS YOU MODIFY LocalDataStoreBaseObject in vm\object.h =========================================================================*/ private Object[] m_DataTable; private LocalDataStoreMgr m_Manager; #pragma warning disable 414 // Field is not used from managed. private int DONT_USE_InternalStore = 0; // pointer #pragma warning restore 414 /*========================================================================= ** Initialize the data store. =========================================================================*/ public LocalDataStore(LocalDataStoreMgr mgr, int InitialCapacity) { if (null == mgr) throw new ArgumentNullException("mgr"); // Store the manager of the local data store. m_Manager = mgr; // Allocate the array that will contain the data. m_DataTable = new Object[InitialCapacity]; } /*========================================================================= ** Retrieves the value from the specified slot. =========================================================================*/ public Object GetData(LocalDataStoreSlot slot) { Object o = null; // Validate the slot. m_Manager.ValidateSlot(slot); // Cache the slot index to avoid synchronization issues. int slotIdx = slot.Slot; if (slotIdx >= 0) { // Delay expansion of m_DataTable if we can if (slotIdx >= m_DataTable.Length) return null; // Retrieve the data from the given slot. o = m_DataTable[slotIdx]; } // Check if the slot has become invalid. if (!slot.IsValid()) throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_SlotHasBeenFreed")); return o; } /*========================================================================== ** Sets the data in the specified slot. =========================================================================*/ public void SetData(LocalDataStoreSlot slot, Object data) { // Validate the slot. m_Manager.ValidateSlot(slot); // I can't think of a way to avoid the ---- described in the // LocalDataStoreSlot finalizer method without a lock. bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(m_Manager, ref tookLock); if (!slot.IsValid()) throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_SlotHasBeenFreed")); // Do the actual set operation. SetDataInternal(slot.Slot, data, true /*bAlloc*/ ); } finally { if (tookLock) Monitor.Exit(m_Manager); } } /*========================================================================= ** This method does the actual work of setting the data. =========================================================================*/ internal void SetDataInternal(int slot, Object data, bool bAlloc) { // We try to delay allocate the dataTable (in cases like the manager clearing a // just-freed slot in all stores if (slot >= m_DataTable.Length) { if (!bAlloc) return; SetCapacity(m_Manager.GetSlotTableLength()); } // Set the data on the given slot. m_DataTable[slot] = data; } /*========================================================================== ** Method used to set the capacity of the local data store. =========================================================================*/ private void SetCapacity(int capacity) { // Validate that the specified capacity is larger than the current one. if (capacity < m_DataTable.Length) throw new ArgumentException(Environment.GetResourceString("Argument_ALSInvalidCapacity")); // Allocate the new data table. Object[] NewDataTable = new Object[capacity]; // Copy all the objects into the new table. Array.Copy(m_DataTable, NewDataTable, m_DataTable.Length); // Save the new table. m_DataTable = NewDataTable; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: LocalDataStore ** ** ** Purpose: Class that stores local data. This class is used in cooperation ** with the _LocalDataStoreMgr class. ** ** =============================================================================*/ namespace System { using System; using System.Threading; using System.Runtime.CompilerServices; // This class will not be marked serializable internal class LocalDataStore { /*========================================================================= ** DON'T CHANGE THESE UNLESS YOU MODIFY LocalDataStoreBaseObject in vm\object.h =========================================================================*/ private Object[] m_DataTable; private LocalDataStoreMgr m_Manager; #pragma warning disable 414 // Field is not used from managed. private int DONT_USE_InternalStore = 0; // pointer #pragma warning restore 414 /*========================================================================= ** Initialize the data store. =========================================================================*/ public LocalDataStore(LocalDataStoreMgr mgr, int InitialCapacity) { if (null == mgr) throw new ArgumentNullException("mgr"); // Store the manager of the local data store. m_Manager = mgr; // Allocate the array that will contain the data. m_DataTable = new Object[InitialCapacity]; } /*========================================================================= ** Retrieves the value from the specified slot. =========================================================================*/ public Object GetData(LocalDataStoreSlot slot) { Object o = null; // Validate the slot. m_Manager.ValidateSlot(slot); // Cache the slot index to avoid synchronization issues. int slotIdx = slot.Slot; if (slotIdx >= 0) { // Delay expansion of m_DataTable if we can if (slotIdx >= m_DataTable.Length) return null; // Retrieve the data from the given slot. o = m_DataTable[slotIdx]; } // Check if the slot has become invalid. if (!slot.IsValid()) throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_SlotHasBeenFreed")); return o; } /*========================================================================== ** Sets the data in the specified slot. =========================================================================*/ public void SetData(LocalDataStoreSlot slot, Object data) { // Validate the slot. m_Manager.ValidateSlot(slot); // I can't think of a way to avoid the ---- described in the // LocalDataStoreSlot finalizer method without a lock. bool tookLock = false; RuntimeHelpers.PrepareConstrainedRegions(); try { Monitor.ReliableEnter(m_Manager, ref tookLock); if (!slot.IsValid()) throw new InvalidOperationException(Environment.GetResourceString("InvalidOperation_SlotHasBeenFreed")); // Do the actual set operation. SetDataInternal(slot.Slot, data, true /*bAlloc*/ ); } finally { if (tookLock) Monitor.Exit(m_Manager); } } /*========================================================================= ** This method does the actual work of setting the data. =========================================================================*/ internal void SetDataInternal(int slot, Object data, bool bAlloc) { // We try to delay allocate the dataTable (in cases like the manager clearing a // just-freed slot in all stores if (slot >= m_DataTable.Length) { if (!bAlloc) return; SetCapacity(m_Manager.GetSlotTableLength()); } // Set the data on the given slot. m_DataTable[slot] = data; } /*========================================================================== ** Method used to set the capacity of the local data store. =========================================================================*/ private void SetCapacity(int capacity) { // Validate that the specified capacity is larger than the current one. if (capacity < m_DataTable.Length) throw new ArgumentException(Environment.GetResourceString("Argument_ALSInvalidCapacity")); // Allocate the new data table. Object[] NewDataTable = new Object[capacity]; // Copy all the objects into the new table. Array.Copy(m_DataTable, NewDataTable, m_DataTable.Length); // Save the new table. m_DataTable = NewDataTable; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
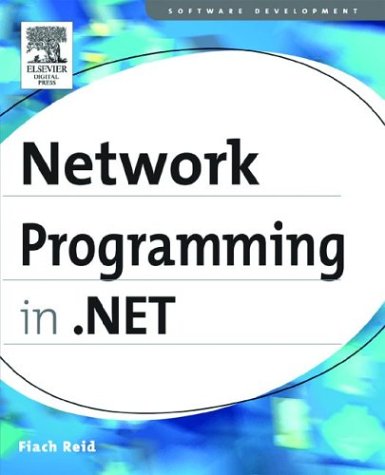
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- wmiprovider.cs
- PersonalizableAttribute.cs
- SelectedDatesCollection.cs
- HMACMD5.cs
- EnumValAlphaComparer.cs
- TreeWalkHelper.cs
- BeginStoryboard.cs
- MaskInputRejectedEventArgs.cs
- SystemColors.cs
- UpdateCompiler.cs
- ProxyFragment.cs
- PropertyDescriptor.cs
- TextFragmentEngine.cs
- WebPartTransformerCollection.cs
- VisualStyleElement.cs
- ApplicationDirectory.cs
- SQLInt16.cs
- AnnotationDocumentPaginator.cs
- StyleSelector.cs
- ActivationArguments.cs
- RadioButtonList.cs
- DataFormats.cs
- LocalizeDesigner.cs
- SymbolType.cs
- dbdatarecord.cs
- ByteStreamMessageUtility.cs
- LocatorManager.cs
- CategoryList.cs
- EditorZone.cs
- SqlTypeSystemProvider.cs
- TreeViewHitTestInfo.cs
- XmlTextReaderImplHelpers.cs
- BaseDataBoundControl.cs
- ReferenceConverter.cs
- DataObjectEventArgs.cs
- SchemaObjectWriter.cs
- CreatingCookieEventArgs.cs
- BindingExpressionUncommonField.cs
- WindowVisualStateTracker.cs
- Graphics.cs
- SafeMILHandle.cs
- ResXResourceSet.cs
- TypeForwardedToAttribute.cs
- GuidelineCollection.cs
- StringDictionaryWithComparer.cs
- SpeakProgressEventArgs.cs
- AssertFilter.cs
- Permission.cs
- TemplateBamlTreeBuilder.cs
- PointAnimationBase.cs
- ObjectStateManager.cs
- DataSourceXmlSerializationAttribute.cs
- SpeechUI.cs
- TableCellCollection.cs
- ItemsChangedEventArgs.cs
- Activator.cs
- XmlHierarchicalEnumerable.cs
- URL.cs
- SemaphoreSecurity.cs
- TopClause.cs
- WsdlInspector.cs
- ZipIOModeEnforcingStream.cs
- DocumentScope.cs
- GetPageNumberCompletedEventArgs.cs
- CursorInteropHelper.cs
- PeerNodeTraceRecord.cs
- WrappedIUnknown.cs
- SecondaryViewProvider.cs
- ListDesigner.cs
- UserControl.cs
- CommandTreeTypeHelper.cs
- InsufficientMemoryException.cs
- OleDbMetaDataFactory.cs
- ClosableStream.cs
- HttpSessionStateWrapper.cs
- WebPartVerb.cs
- SqlTrackingQuery.cs
- EventLogPermissionEntry.cs
- EntryPointNotFoundException.cs
- BuildProviderUtils.cs
- WebPart.cs
- MimeObjectFactory.cs
- TextFormatter.cs
- ScrollBar.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- Math.cs
- CustomAttributeFormatException.cs
- Style.cs
- BaseDataBoundControlDesigner.cs
- BuildProviderAppliesToAttribute.cs
- behaviorssection.cs
- ParseHttpDate.cs
- PageParserFilter.cs
- CodeExpressionStatement.cs
- SectionUpdates.cs
- Literal.cs
- TreeViewDataItemAutomationPeer.cs
- SystemParameters.cs
- CatalogPart.cs
- CommentGlyph.cs