Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / Internal / CommandTreeTypeHelper.cs / 4 / CommandTreeTypeHelper.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; using System.Data.Common; // for TypeHelpers namespace System.Data.Common.CommandTrees.Internal { internal class CommandTreeTypeHelper { private DbCommandTree _owner; private TypeUsage _boolType; ////// Constructs a new CommandTreeTypeHelper that is associated with the specified DbCommandTree /// /// The DbCommandTree that specifies the metadata collection and dataspace against which this TypeHelper should operate internal CommandTreeTypeHelper(DbCommandTree owner) { _owner = owner; } internal static void CheckReadOnly(GlobalItem item, string varName) { EntityUtil.CheckArgumentNull(item, varName); if (!(item.IsReadOnly)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_MetadataNotReadOnly, varName); } } internal static void CheckReadOnly(TypeUsage item, string varName) { EntityUtil.CheckArgumentNull(item, varName); if (!(item.IsReadOnly)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_MetadataNotReadOnly, varName); } } internal static void CheckReadOnly(EntitySetBase item, string varName) { EntityUtil.CheckArgumentNull(item, varName); if (!(item.IsReadOnly)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_MetadataNotReadOnly, varName); } } internal static void CheckType(EdmType type) { EntityUtil.CheckArgumentNull(type, "type"); CheckReadOnly(type, "type"); } ////// Ensures that the specified type is non-null, associated with the correct metadata workspace/dataspace, and is not NullType. /// /// The type usage instance to verify. ///If the specified type metadata is null ///If the specified type metadata belongs to a metadata workspace other than the workspace of the command tree ///If the specified type metadata belongs to a dataspace other than the dataspace of the command tree internal void CheckType(TypeUsage type) { CheckType(type, "type"); } internal void CheckType(TypeUsage type, string varName) { EntityUtil.CheckArgumentNull(type, varName); CheckReadOnly(type, varName); if (TypeSemantics.IsNullType(type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_NullTypeInvalid, varName); } if (null == type.EdmType) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_TypeUsageNullEdmTypeInvalid, "type"); } if (!CheckWorkspaceAndDataSpace(type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_TypeUsageIncorrectSpace, "type"); } } ////// Ensures that the specified type is non-null, associated with the correct metadata workspace/dataspace, /// is not NullType, and is polymorphic. /// /// The type usage instance to verify. ///If the specified type metadata is null ///If the specified type metadata belongs to a metadata workspace other than the workspace of the command tree ///If the specified type metadata belongs to a dataspace other than the dataspace of the command tree ///If the specified type metadata does not represent a polymorphic type internal void CheckPolymorphicType(TypeUsage type) { this.CheckType(type); if (!TypeSemantics.IsPolymorphicType(type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_PolymorphicTypeRequired(TypeHelpers.GetFullName(type)), "type"); } } ////// Verifies that the specified member is valid - non-null, from the same metadata workspace and data space as the command tree, etc /// /// The member to verify /// The name of the variable to which this member instance is being assigned internal void CheckMember(EdmMember memberMeta, string varName) { EntityUtil.CheckArgumentNull(memberMeta, varName); CheckReadOnly(memberMeta.DeclaringType, varName); if (null == memberMeta.Name) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EdmMemberNameNull, varName); } if (TypeSemantics.IsNullOrNullType(memberMeta.TypeUsage)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EdmMemberTypeNull, varName); } if (TypeSemantics.IsNullOrNullType(memberMeta.DeclaringType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EdmMemberDeclaringTypeNull, varName); } if(!CheckWorkspaceAndDataSpace(memberMeta.TypeUsage) || !CheckWorkspaceAndDataSpace(memberMeta.DeclaringType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EdmMemberIncorrectSpace, varName); } } internal void CheckParameter(FunctionParameter paramMeta, string varName) { EntityUtil.CheckArgumentNull(paramMeta, varName); CheckReadOnly(paramMeta.DeclaringFunction, varName); if (null == paramMeta.Name) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionParameterNameNull, varName); } if (TypeSemantics.IsNullOrNullType(paramMeta.TypeUsage)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionParameterTypeNull, varName); } // Verify that the parameter is from the same workspace as the DbCommandTree if (!CheckWorkspaceAndDataSpace(paramMeta.TypeUsage)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionParameterIncorrectSpace, varName); } } ////// Verifies that the specified function metadata is valid - non-null and either created by this command tree (if a LambdaFunction) or from the same metadata collection and data space as the command tree (for ordinary function metadata) /// /// The function metadata to verify internal void CheckFunction(EdmFunction function) { EntityUtil.CheckArgumentNull(function, "function"); CheckReadOnly(function, "function"); if (null == function.Name) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionNameNull, "function"); } if (!CheckWorkspaceAndDataSpace(function)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionIncorrectSpace, "function"); } // Composable functions must have a return parameter. if (function.IsComposableAttribute && null == function.ReturnParameter) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionReturnParameterNull, "function"); } // Verify that the function ReturnType - if present - is from the DbCommandTree's metadata collection and dataspace // A return parameter is not required for non-composable functions. if (function.ReturnParameter != null) { if (TypeSemantics.IsNullOrNullType(function.ReturnParameter.TypeUsage)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionParameterTypeNull, "function.ReturnParameter"); } if (!CheckWorkspaceAndDataSpace(function.ReturnParameter.TypeUsage)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionParameterIncorrectSpace, "function.ReturnParameter"); } } // Verify that the function parameters collection is non-null and, // if non-empty, contains valid IParameterMetadata instances. IListfunctionParams = function.Parameters; if (null == functionParams) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionParametersNull, "function"); } for (int idx = 0; idx < functionParams.Count; idx++) { this.CheckParameter(functionParams[idx], CommandTreeUtils.FormatIndex("function.Parameters", idx)); } } /// /// Verifies that the specified EntitySet is valid with respect to the command tree /// /// The EntitySet to verify /// The variable name to use if an exception should be thrown internal void CheckEntitySet(EntitySetBase entitySet, string varName) { EntityUtil.CheckArgumentNull(entitySet, varName); CheckReadOnly(entitySet, varName); if (null == entitySet.Name) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EntitySetNameNull, varName); } // // Verify the Extent's Container // if (null == entitySet.EntityContainer) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EntitySetEntityContainerNull, varName); } if(!CheckWorkspaceAndDataSpace(entitySet.EntityContainer)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EntitySetIncorrectSpace, varName); } // // Verify the Extent's Entity Type // if (null == entitySet.ElementType) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EntitySetElementTypeNull, varName); } if(!CheckWorkspaceAndDataSpace(entitySet.ElementType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EntitySetIncorrectSpace, varName); } } private bool CheckWorkspaceAndDataSpace(TypeUsage type) { return CheckWorkspaceAndDataSpace(type.EdmType); } private bool CheckWorkspaceAndDataSpace(GlobalItem item) { // Since the set of primitive types and canonical functions are shared, we don't need to check for them. // Additionally, any non-canonical function in the C-Space must be a cached store function, which will // also not be present in the workspace. if (BuiltInTypeKind.PrimitiveType == item.BuiltInTypeKind || (BuiltInTypeKind.EdmFunction == item.BuiltInTypeKind && DataSpace.CSpace == item.DataSpace)) { return true; } GlobalItem newGlobalItem = null; if((item.DataSpace == DataSpace.SSpace || item.DataSpace == DataSpace.CSpace) && _owner.MetadataWorkspace.TryGetItem(item.Identity, item.DataSpace, out newGlobalItem) && object.ReferenceEquals(item, newGlobalItem)) { return true; } if (Helper.IsRowType(item)) { foreach (EdmProperty prop in ((RowType)item).Properties) { if (!CheckWorkspaceAndDataSpace(prop.TypeUsage)) { return false; } } return true; } if (Helper.IsCollectionType(item)) { return CheckWorkspaceAndDataSpace(((CollectionType)item).TypeUsage); } if (Helper.IsRefType(item)) { return CheckWorkspaceAndDataSpace(((RefType)item).ElementType); } return false; } /// /// Verifies that the specified EntitySet is valid with respect to the command tree /// /// The EntitySet to verify internal void CheckEntitySet(EntitySetBase entitySet) { CheckEntitySet(entitySet, "entitySet"); } internal static TypeUsage CreateCollectionOfRowResultType(List> columns) { TypeUsage retUsage = TypeUsage.Create( TypeHelpers.CreateCollectionType( TypeUsage.Create( TypeHelpers.CreateRowType(columns) ) ) ); return retUsage; } internal static TypeUsage CreateCollectionResultType(EdmType type) { TypeUsage retUsage = TypeUsage.Create( TypeHelpers.CreateCollectionType( TypeUsage.Create(type) ) ); return retUsage; } internal static TypeUsage CreateCollectionResultType(TypeUsage type) { TypeUsage retUsage = TypeUsage.Create(TypeHelpers.CreateCollectionType(type)); return retUsage; } internal TypeUsage CreateBooleanResultType() { if (null == _boolType) { _boolType = _owner.MetadataWorkspace.GetCanonicalModelTypeUsage(PrimitiveTypeKind.Boolean); } return _boolType; } internal static TypeUsage CreateResultType(EdmType resultType) { return TypeUsage.Create(resultType); } internal static TypeUsage CreateReferenceResultType(EntityTypeBase referencedEntityType) { return TypeUsage.Create(TypeHelpers.CreateReferenceType(referencedEntityType)); } /// /// Requires: non-null expression /// Determines whether the expression is a constant negative integer value. Always returns /// false for non-constant, non-integer expression instances. /// internal static bool IsConstantNegativeInteger(DbExpression expression) { return (expression.ExpressionKind == DbExpressionKind.Constant && TypeSemantics.IsIntegerNumericType(expression.ResultType) && Convert.ToInt64(((DbConstantExpression)expression).Value, CultureInfo.InvariantCulture) < 0); } internal static TypeUsage SetResultAsNullable(TypeUsage typeUsage) { TypeUsage newTypeUsage = typeUsage; if (!TypeSemantics.IsNullable(typeUsage)) { newTypeUsage = newTypeUsage.ShallowCopy(new FacetValues { Nullable = true }); } return newTypeUsage; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; using System.Data.Common; // for TypeHelpers namespace System.Data.Common.CommandTrees.Internal { internal class CommandTreeTypeHelper { private DbCommandTree _owner; private TypeUsage _boolType; ////// Constructs a new CommandTreeTypeHelper that is associated with the specified DbCommandTree /// /// The DbCommandTree that specifies the metadata collection and dataspace against which this TypeHelper should operate internal CommandTreeTypeHelper(DbCommandTree owner) { _owner = owner; } internal static void CheckReadOnly(GlobalItem item, string varName) { EntityUtil.CheckArgumentNull(item, varName); if (!(item.IsReadOnly)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_MetadataNotReadOnly, varName); } } internal static void CheckReadOnly(TypeUsage item, string varName) { EntityUtil.CheckArgumentNull(item, varName); if (!(item.IsReadOnly)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_MetadataNotReadOnly, varName); } } internal static void CheckReadOnly(EntitySetBase item, string varName) { EntityUtil.CheckArgumentNull(item, varName); if (!(item.IsReadOnly)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_MetadataNotReadOnly, varName); } } internal static void CheckType(EdmType type) { EntityUtil.CheckArgumentNull(type, "type"); CheckReadOnly(type, "type"); } ////// Ensures that the specified type is non-null, associated with the correct metadata workspace/dataspace, and is not NullType. /// /// The type usage instance to verify. ///If the specified type metadata is null ///If the specified type metadata belongs to a metadata workspace other than the workspace of the command tree ///If the specified type metadata belongs to a dataspace other than the dataspace of the command tree internal void CheckType(TypeUsage type) { CheckType(type, "type"); } internal void CheckType(TypeUsage type, string varName) { EntityUtil.CheckArgumentNull(type, varName); CheckReadOnly(type, varName); if (TypeSemantics.IsNullType(type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_NullTypeInvalid, varName); } if (null == type.EdmType) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_TypeUsageNullEdmTypeInvalid, "type"); } if (!CheckWorkspaceAndDataSpace(type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_TypeUsageIncorrectSpace, "type"); } } ////// Ensures that the specified type is non-null, associated with the correct metadata workspace/dataspace, /// is not NullType, and is polymorphic. /// /// The type usage instance to verify. ///If the specified type metadata is null ///If the specified type metadata belongs to a metadata workspace other than the workspace of the command tree ///If the specified type metadata belongs to a dataspace other than the dataspace of the command tree ///If the specified type metadata does not represent a polymorphic type internal void CheckPolymorphicType(TypeUsage type) { this.CheckType(type); if (!TypeSemantics.IsPolymorphicType(type)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_General_PolymorphicTypeRequired(TypeHelpers.GetFullName(type)), "type"); } } ////// Verifies that the specified member is valid - non-null, from the same metadata workspace and data space as the command tree, etc /// /// The member to verify /// The name of the variable to which this member instance is being assigned internal void CheckMember(EdmMember memberMeta, string varName) { EntityUtil.CheckArgumentNull(memberMeta, varName); CheckReadOnly(memberMeta.DeclaringType, varName); if (null == memberMeta.Name) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EdmMemberNameNull, varName); } if (TypeSemantics.IsNullOrNullType(memberMeta.TypeUsage)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EdmMemberTypeNull, varName); } if (TypeSemantics.IsNullOrNullType(memberMeta.DeclaringType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EdmMemberDeclaringTypeNull, varName); } if(!CheckWorkspaceAndDataSpace(memberMeta.TypeUsage) || !CheckWorkspaceAndDataSpace(memberMeta.DeclaringType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EdmMemberIncorrectSpace, varName); } } internal void CheckParameter(FunctionParameter paramMeta, string varName) { EntityUtil.CheckArgumentNull(paramMeta, varName); CheckReadOnly(paramMeta.DeclaringFunction, varName); if (null == paramMeta.Name) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionParameterNameNull, varName); } if (TypeSemantics.IsNullOrNullType(paramMeta.TypeUsage)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionParameterTypeNull, varName); } // Verify that the parameter is from the same workspace as the DbCommandTree if (!CheckWorkspaceAndDataSpace(paramMeta.TypeUsage)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionParameterIncorrectSpace, varName); } } ////// Verifies that the specified function metadata is valid - non-null and either created by this command tree (if a LambdaFunction) or from the same metadata collection and data space as the command tree (for ordinary function metadata) /// /// The function metadata to verify internal void CheckFunction(EdmFunction function) { EntityUtil.CheckArgumentNull(function, "function"); CheckReadOnly(function, "function"); if (null == function.Name) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionNameNull, "function"); } if (!CheckWorkspaceAndDataSpace(function)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionIncorrectSpace, "function"); } // Composable functions must have a return parameter. if (function.IsComposableAttribute && null == function.ReturnParameter) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionReturnParameterNull, "function"); } // Verify that the function ReturnType - if present - is from the DbCommandTree's metadata collection and dataspace // A return parameter is not required for non-composable functions. if (function.ReturnParameter != null) { if (TypeSemantics.IsNullOrNullType(function.ReturnParameter.TypeUsage)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionParameterTypeNull, "function.ReturnParameter"); } if (!CheckWorkspaceAndDataSpace(function.ReturnParameter.TypeUsage)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionParameterIncorrectSpace, "function.ReturnParameter"); } } // Verify that the function parameters collection is non-null and, // if non-empty, contains valid IParameterMetadata instances. IListfunctionParams = function.Parameters; if (null == functionParams) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_FunctionParametersNull, "function"); } for (int idx = 0; idx < functionParams.Count; idx++) { this.CheckParameter(functionParams[idx], CommandTreeUtils.FormatIndex("function.Parameters", idx)); } } /// /// Verifies that the specified EntitySet is valid with respect to the command tree /// /// The EntitySet to verify /// The variable name to use if an exception should be thrown internal void CheckEntitySet(EntitySetBase entitySet, string varName) { EntityUtil.CheckArgumentNull(entitySet, varName); CheckReadOnly(entitySet, varName); if (null == entitySet.Name) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EntitySetNameNull, varName); } // // Verify the Extent's Container // if (null == entitySet.EntityContainer) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EntitySetEntityContainerNull, varName); } if(!CheckWorkspaceAndDataSpace(entitySet.EntityContainer)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EntitySetIncorrectSpace, varName); } // // Verify the Extent's Entity Type // if (null == entitySet.ElementType) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EntitySetElementTypeNull, varName); } if(!CheckWorkspaceAndDataSpace(entitySet.ElementType)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Metadata_EntitySetIncorrectSpace, varName); } } private bool CheckWorkspaceAndDataSpace(TypeUsage type) { return CheckWorkspaceAndDataSpace(type.EdmType); } private bool CheckWorkspaceAndDataSpace(GlobalItem item) { // Since the set of primitive types and canonical functions are shared, we don't need to check for them. // Additionally, any non-canonical function in the C-Space must be a cached store function, which will // also not be present in the workspace. if (BuiltInTypeKind.PrimitiveType == item.BuiltInTypeKind || (BuiltInTypeKind.EdmFunction == item.BuiltInTypeKind && DataSpace.CSpace == item.DataSpace)) { return true; } GlobalItem newGlobalItem = null; if((item.DataSpace == DataSpace.SSpace || item.DataSpace == DataSpace.CSpace) && _owner.MetadataWorkspace.TryGetItem(item.Identity, item.DataSpace, out newGlobalItem) && object.ReferenceEquals(item, newGlobalItem)) { return true; } if (Helper.IsRowType(item)) { foreach (EdmProperty prop in ((RowType)item).Properties) { if (!CheckWorkspaceAndDataSpace(prop.TypeUsage)) { return false; } } return true; } if (Helper.IsCollectionType(item)) { return CheckWorkspaceAndDataSpace(((CollectionType)item).TypeUsage); } if (Helper.IsRefType(item)) { return CheckWorkspaceAndDataSpace(((RefType)item).ElementType); } return false; } /// /// Verifies that the specified EntitySet is valid with respect to the command tree /// /// The EntitySet to verify internal void CheckEntitySet(EntitySetBase entitySet) { CheckEntitySet(entitySet, "entitySet"); } internal static TypeUsage CreateCollectionOfRowResultType(List> columns) { TypeUsage retUsage = TypeUsage.Create( TypeHelpers.CreateCollectionType( TypeUsage.Create( TypeHelpers.CreateRowType(columns) ) ) ); return retUsage; } internal static TypeUsage CreateCollectionResultType(EdmType type) { TypeUsage retUsage = TypeUsage.Create( TypeHelpers.CreateCollectionType( TypeUsage.Create(type) ) ); return retUsage; } internal static TypeUsage CreateCollectionResultType(TypeUsage type) { TypeUsage retUsage = TypeUsage.Create(TypeHelpers.CreateCollectionType(type)); return retUsage; } internal TypeUsage CreateBooleanResultType() { if (null == _boolType) { _boolType = _owner.MetadataWorkspace.GetCanonicalModelTypeUsage(PrimitiveTypeKind.Boolean); } return _boolType; } internal static TypeUsage CreateResultType(EdmType resultType) { return TypeUsage.Create(resultType); } internal static TypeUsage CreateReferenceResultType(EntityTypeBase referencedEntityType) { return TypeUsage.Create(TypeHelpers.CreateReferenceType(referencedEntityType)); } /// /// Requires: non-null expression /// Determines whether the expression is a constant negative integer value. Always returns /// false for non-constant, non-integer expression instances. /// internal static bool IsConstantNegativeInteger(DbExpression expression) { return (expression.ExpressionKind == DbExpressionKind.Constant && TypeSemantics.IsIntegerNumericType(expression.ResultType) && Convert.ToInt64(((DbConstantExpression)expression).Value, CultureInfo.InvariantCulture) < 0); } internal static TypeUsage SetResultAsNullable(TypeUsage typeUsage) { TypeUsage newTypeUsage = typeUsage; if (!TypeSemantics.IsNullable(typeUsage)) { newTypeUsage = newTypeUsage.ShallowCopy(new FacetValues { Nullable = true }); } return newTypeUsage; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
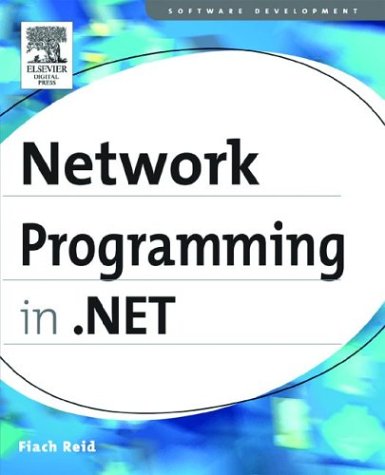
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DoubleLinkList.cs
- Preprocessor.cs
- ClientSession.cs
- DefaultSection.cs
- DoubleKeyFrameCollection.cs
- ConsoleTraceListener.cs
- StructuredTypeInfo.cs
- PackageRelationshipCollection.cs
- Figure.cs
- ConfigurationCollectionAttribute.cs
- AuthenticationModuleElementCollection.cs
- CheckedPointers.cs
- CompoundFileIOPermission.cs
- TemplateControlCodeDomTreeGenerator.cs
- DirectoryInfo.cs
- RemotingAttributes.cs
- NameValueFileSectionHandler.cs
- MDIControlStrip.cs
- ExpressionBuilderContext.cs
- MessageQueueInstaller.cs
- ItemCollection.cs
- QuaternionAnimationBase.cs
- OraclePermissionAttribute.cs
- URLString.cs
- ConsumerConnectionPointCollection.cs
- XNameTypeConverter.cs
- ReadContentAsBinaryHelper.cs
- AspProxy.cs
- FlatButtonAppearance.cs
- XmlEncodedRawTextWriter.cs
- Geometry3D.cs
- Types.cs
- dataSvcMapFileLoader.cs
- QueryReaderSettings.cs
- DirectoryInfo.cs
- EntityDataSourceWizardForm.cs
- ListViewSelectEventArgs.cs
- RequestBringIntoViewEventArgs.cs
- GridViewItemAutomationPeer.cs
- DataControlButton.cs
- ConfigurationPropertyCollection.cs
- HtmlInputReset.cs
- QuaternionKeyFrameCollection.cs
- ProtocolsSection.cs
- ObjectDisposedException.cs
- XPathSelfQuery.cs
- _ShellExpression.cs
- SqlBooleanizer.cs
- NegotiateStream.cs
- OrderToken.cs
- TextLine.cs
- ApplicationSecurityInfo.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- GPPOINT.cs
- HyperLinkStyle.cs
- SiteMapSection.cs
- ViewCellRelation.cs
- TimeSpan.cs
- ToolStripPanelRenderEventArgs.cs
- BackStopAuthenticationModule.cs
- DataServiceHost.cs
- SafeEventLogWriteHandle.cs
- CompletedAsyncResult.cs
- Processor.cs
- _SingleItemRequestCache.cs
- util.cs
- SiteMapNodeItem.cs
- MsmqIntegrationOutputChannel.cs
- DynamicFilter.cs
- FormViewDesigner.cs
- EventDrivenDesigner.cs
- EntityDataSourceConfigureObjectContextPanel.cs
- SqlAliaser.cs
- EmptyControlCollection.cs
- RegexRunner.cs
- ValidationError.cs
- SqlTriggerAttribute.cs
- InputReferenceExpression.cs
- GridViewColumnCollection.cs
- UiaCoreApi.cs
- SafeNativeMethods.cs
- LocationReference.cs
- SqlRowUpdatingEvent.cs
- QueryCacheEntry.cs
- NetworkAddressChange.cs
- MimeXmlImporter.cs
- XPathChildIterator.cs
- Zone.cs
- InvalidCastException.cs
- CodeTypeDelegate.cs
- ListBox.cs
- ColumnWidthChangedEvent.cs
- GraphicsState.cs
- CannotUnloadAppDomainException.cs
- CapacityStreamGeometryContext.cs
- DataGridViewElement.cs
- EnumCodeDomSerializer.cs
- OleDbReferenceCollection.cs
- storepermission.cs
- SimpleBitVector32.cs