Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Objects / DataClasses / ComplexObject.cs / 3 / ComplexObject.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data; using System.Diagnostics; using System.Reflection; using System.ComponentModel; using System.Runtime.Serialization; namespace System.Data.Objects.DataClasses { ////// This is the interface that represent the minimum interface required /// to be an entity in ADO.NET. /// [DataContract(IsReference = true)] [Serializable] public abstract class ComplexObject : StructuralObject { // The following fields are serialized. Adding or removing a serialized field is considered // a breaking change. This includes changing the field type or field name of existing // serialized fields. If you need to make this kind of change, it may be possible, but it // will require some custom serialization/deserialization code. private StructuralObject _parent; // Object that contains this ComplexObject (can be Entity or ComplexObject) private string _parentPropertyName; // Property name for this type on the containing object ////// Associate the ComplexType with an Entity or another ComplexObject /// Parent may be an Entity or ComplexObject /// /// Object to be added to. /// The property on the parent that reference the complex type. internal void AttachToParent( StructuralObject parent, string parentPropertyName) { Debug.Assert(null != parent, "Attempt to attach to a null parent"); Debug.Assert(null != parentPropertyName, "Must provide parentPropertyName in order to attach"); if (_parent != null) { throw EntityUtil.ComplexObjectAlreadyAttachedToParent(); } Debug.Assert(_parentPropertyName == null); _parent = parent; _parentPropertyName = parentPropertyName; } ////// Removes this instance from the parent it was attached to. /// Parent may be an Entity or ComplexObject /// internal void DetachFromParent() { // We will null out _parent and _parentPropertyName anyway, so if they are already null // it is an unexpected condition, but should not cause a failure in released code Debug.Assert(_parent != null, "Attempt to detach from a null _parent"); Debug.Assert(_parentPropertyName != null, "Null _parentPropertyName on a non-null _parent"); _parent = null; _parentPropertyName = null; } ////// Reports that a change is about to occur to one of the properties of this instance /// to the containing object and then continues default change /// reporting behavior. /// protected sealed override void ReportPropertyChanging( string property) { EntityUtil.CheckStringArgument(property, "property"); base.ReportPropertyChanging(property); // Since we are a ComplexObject, all changes (scalar or complex) are considered complex property changes ReportComplexPropertyChanging(null, this, property); } ////// Reports a change to one of the properties of this instance /// to the containing object and then continues default change /// reporting behavior. /// protected sealed override void ReportPropertyChanged( string property) { EntityUtil.CheckStringArgument(property, "property"); // Since we are a ComplexObject, all changes (scalar or complex) are considered complex property changes ReportComplexPropertyChanged(null, this, property); base.ReportPropertyChanged(property); } internal sealed override bool IsChangeTracked { get { return _parent == null ? false : _parent.IsChangeTracked; } } ////// This method is used to report all changes on this ComplexObject to its parent entity or ComplexObject /// /// /// Should be null in this method override. /// This is only relevant in Entity's implementation of this method, so it is unused here /// Instead of passing the most-derived property name up the hierarchy, we will always pass the current _parentPropertyName /// Once this gets up to the Entity, it will actually use the value that was passed in /// /// /// The instance of the object on which the property is changing. /// /// /// The name of the changing property on complexObject. /// internal sealed override void ReportComplexPropertyChanging( string entityMemberName, ComplexObject complexObject, string complexMemberName) { // entityMemberName is unused here because we just keep passing the current parent name up the hierarchy // This value is only used in the EntityObject override of this method Debug.Assert(complexObject != null, "invalid complexObject"); Debug.Assert(!String.IsNullOrEmpty(complexMemberName), "invalid complexMemberName"); if (null != _parent) { _parent.ReportComplexPropertyChanging(_parentPropertyName, complexObject, complexMemberName); } } ////// This method is used to report all changes on this ComplexObject to its parent entity or ComplexObject /// /// /// Should be null in this method override. /// This is only relevant in Entity's implementation of this method, so it is unused here /// Instead of passing the most-derived property name up the hierarchy, we will always pass the current _parentPropertyName /// Once this gets up to the Entity, it will actually use the value that was passed in. /// /// /// The instance of the object on which the property is changing. /// /// /// The name of the changing property on complexObject. /// internal sealed override void ReportComplexPropertyChanged( string entityMemberName, ComplexObject complexObject, string complexMemberName) { // entityMemberName is unused here because we just keep passing the current parent name up the hierarchy // This value is only used in the EntityObject override of this method Debug.Assert(complexObject != null, "invalid complexObject"); Debug.Assert(!String.IsNullOrEmpty(complexMemberName), "invalid complexMemberName"); if (null != _parent) { _parent.ReportComplexPropertyChanged(_parentPropertyName, complexObject, complexMemberName); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data; using System.Diagnostics; using System.Reflection; using System.ComponentModel; using System.Runtime.Serialization; namespace System.Data.Objects.DataClasses { ////// This is the interface that represent the minimum interface required /// to be an entity in ADO.NET. /// [DataContract(IsReference = true)] [Serializable] public abstract class ComplexObject : StructuralObject { // The following fields are serialized. Adding or removing a serialized field is considered // a breaking change. This includes changing the field type or field name of existing // serialized fields. If you need to make this kind of change, it may be possible, but it // will require some custom serialization/deserialization code. private StructuralObject _parent; // Object that contains this ComplexObject (can be Entity or ComplexObject) private string _parentPropertyName; // Property name for this type on the containing object ////// Associate the ComplexType with an Entity or another ComplexObject /// Parent may be an Entity or ComplexObject /// /// Object to be added to. /// The property on the parent that reference the complex type. internal void AttachToParent( StructuralObject parent, string parentPropertyName) { Debug.Assert(null != parent, "Attempt to attach to a null parent"); Debug.Assert(null != parentPropertyName, "Must provide parentPropertyName in order to attach"); if (_parent != null) { throw EntityUtil.ComplexObjectAlreadyAttachedToParent(); } Debug.Assert(_parentPropertyName == null); _parent = parent; _parentPropertyName = parentPropertyName; } ////// Removes this instance from the parent it was attached to. /// Parent may be an Entity or ComplexObject /// internal void DetachFromParent() { // We will null out _parent and _parentPropertyName anyway, so if they are already null // it is an unexpected condition, but should not cause a failure in released code Debug.Assert(_parent != null, "Attempt to detach from a null _parent"); Debug.Assert(_parentPropertyName != null, "Null _parentPropertyName on a non-null _parent"); _parent = null; _parentPropertyName = null; } ////// Reports that a change is about to occur to one of the properties of this instance /// to the containing object and then continues default change /// reporting behavior. /// protected sealed override void ReportPropertyChanging( string property) { EntityUtil.CheckStringArgument(property, "property"); base.ReportPropertyChanging(property); // Since we are a ComplexObject, all changes (scalar or complex) are considered complex property changes ReportComplexPropertyChanging(null, this, property); } ////// Reports a change to one of the properties of this instance /// to the containing object and then continues default change /// reporting behavior. /// protected sealed override void ReportPropertyChanged( string property) { EntityUtil.CheckStringArgument(property, "property"); // Since we are a ComplexObject, all changes (scalar or complex) are considered complex property changes ReportComplexPropertyChanged(null, this, property); base.ReportPropertyChanged(property); } internal sealed override bool IsChangeTracked { get { return _parent == null ? false : _parent.IsChangeTracked; } } ////// This method is used to report all changes on this ComplexObject to its parent entity or ComplexObject /// /// /// Should be null in this method override. /// This is only relevant in Entity's implementation of this method, so it is unused here /// Instead of passing the most-derived property name up the hierarchy, we will always pass the current _parentPropertyName /// Once this gets up to the Entity, it will actually use the value that was passed in /// /// /// The instance of the object on which the property is changing. /// /// /// The name of the changing property on complexObject. /// internal sealed override void ReportComplexPropertyChanging( string entityMemberName, ComplexObject complexObject, string complexMemberName) { // entityMemberName is unused here because we just keep passing the current parent name up the hierarchy // This value is only used in the EntityObject override of this method Debug.Assert(complexObject != null, "invalid complexObject"); Debug.Assert(!String.IsNullOrEmpty(complexMemberName), "invalid complexMemberName"); if (null != _parent) { _parent.ReportComplexPropertyChanging(_parentPropertyName, complexObject, complexMemberName); } } ////// This method is used to report all changes on this ComplexObject to its parent entity or ComplexObject /// /// /// Should be null in this method override. /// This is only relevant in Entity's implementation of this method, so it is unused here /// Instead of passing the most-derived property name up the hierarchy, we will always pass the current _parentPropertyName /// Once this gets up to the Entity, it will actually use the value that was passed in. /// /// /// The instance of the object on which the property is changing. /// /// /// The name of the changing property on complexObject. /// internal sealed override void ReportComplexPropertyChanged( string entityMemberName, ComplexObject complexObject, string complexMemberName) { // entityMemberName is unused here because we just keep passing the current parent name up the hierarchy // This value is only used in the EntityObject override of this method Debug.Assert(complexObject != null, "invalid complexObject"); Debug.Assert(!String.IsNullOrEmpty(complexMemberName), "invalid complexMemberName"); if (null != _parent) { _parent.ReportComplexPropertyChanged(_parentPropertyName, complexObject, complexMemberName); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
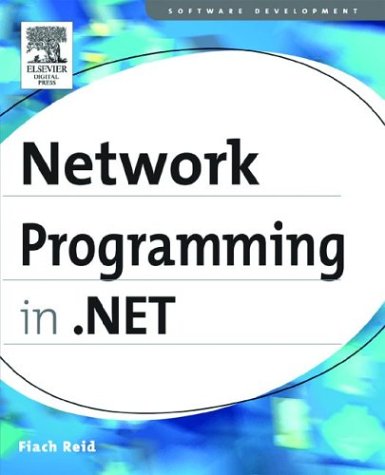
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PersonalizationDictionary.cs
- XmlSchemaInfo.cs
- ToolboxItemSnapLineBehavior.cs
- PrintingPermissionAttribute.cs
- ServiceControllerDesigner.cs
- RegexGroupCollection.cs
- BulletedList.cs
- SafeLibraryHandle.cs
- WebPartVerbCollection.cs
- PointCollectionConverter.cs
- PaintEvent.cs
- BroadcastEventHelper.cs
- GenericArgumentsUpdater.cs
- FileNameEditor.cs
- XsdCachingReader.cs
- UnmanagedMemoryStream.cs
- ColorConverter.cs
- SerialStream.cs
- ToolboxComponentsCreatingEventArgs.cs
- EventMetadata.cs
- StringUtil.cs
- XmlFormatReaderGenerator.cs
- SQLBinaryStorage.cs
- ControlParameter.cs
- RequiredFieldValidator.cs
- Property.cs
- _UriTypeConverter.cs
- IArgumentProvider.cs
- DocumentReference.cs
- Console.cs
- ActivityExecutorSurrogate.cs
- SqlDataSourceTableQuery.cs
- entitydatasourceentitysetnameconverter.cs
- PageStatePersister.cs
- AspCompat.cs
- WindowsListViewItemCheckBox.cs
- WebPartTransformerCollection.cs
- ObjectDataSourceView.cs
- DataGridViewHitTestInfo.cs
- DetailsViewPagerRow.cs
- OdbcErrorCollection.cs
- Process.cs
- RelationshipEndCollection.cs
- ListViewSelectEventArgs.cs
- StateMachine.cs
- WebPartMenuStyle.cs
- ResourceDisplayNameAttribute.cs
- LeftCellWrapper.cs
- SamlConstants.cs
- DoubleStorage.cs
- RequestSecurityToken.cs
- PropertyRef.cs
- SqlFormatter.cs
- SqlXmlStorage.cs
- MimeFormImporter.cs
- CurrentChangingEventManager.cs
- CodeDOMProvider.cs
- CheckBox.cs
- PartitionerStatic.cs
- AssemblyName.cs
- QuaternionRotation3D.cs
- LassoHelper.cs
- DbDataAdapter.cs
- LockCookie.cs
- RC2.cs
- SimpleRecyclingCache.cs
- FilteredReadOnlyMetadataCollection.cs
- BufferedGraphicsManager.cs
- RightsManagementInformation.cs
- filewebrequest.cs
- ArgumentNullException.cs
- DataGridViewCellStyleConverter.cs
- ViewEvent.cs
- VirtualDirectoryMapping.cs
- ImageProxy.cs
- DemultiplexingClientMessageFormatter.cs
- Positioning.cs
- PairComparer.cs
- CornerRadiusConverter.cs
- Oci.cs
- ChildTable.cs
- Thumb.cs
- SchemaImporterExtensionElementCollection.cs
- HitTestDrawingContextWalker.cs
- FamilyMap.cs
- COM2Enum.cs
- TemplateNameScope.cs
- DesignerAttribute.cs
- GenericsInstances.cs
- SchemaNamespaceManager.cs
- QilInvoke.cs
- FontFamilyConverter.cs
- OciEnlistContext.cs
- Roles.cs
- ContextBase.cs
- InheritedPropertyChangedEventArgs.cs
- GroupDescription.cs
- Compiler.cs
- NullableLongAverageAggregationOperator.cs
- XmlSchemaNotation.cs