Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Security / Cryptography / CngKeyBlobFormat.cs / 1305376 / CngKeyBlobFormat.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics.Contracts; namespace System.Security.Cryptography { ////// Utility class to strongly type the format of key blobs used with CNG. Since all CNG APIs which /// require or return a key blob format take the name as a string, we use this string wrapper class to /// specifically mark which parameters and return values are expected to be key blob formats. We also /// provide a list of well known blob formats, which helps Intellisense users find a set of good blob /// formats to use. /// [Serializable] [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CngKeyBlobFormat : IEquatable{ private static CngKeyBlobFormat s_eccPrivate; private static CngKeyBlobFormat s_eccPublic; private static CngKeyBlobFormat s_genericPrivate; private static CngKeyBlobFormat s_genericPublic; private static CngKeyBlobFormat s_opaqueTransport; private static CngKeyBlobFormat s_pkcs8Private; private string m_format; public CngKeyBlobFormat(string format) { Contract.Ensures(!String.IsNullOrEmpty(m_format)); if (format == null) { throw new ArgumentNullException("format"); } if (format.Length == 0) { throw new ArgumentException(SR.GetString(SR.Cryptography_InvalidKeyBlobFormat, format), "format"); } m_format = format; } /// /// Name of the blob format /// public string Format { get { Contract.Ensures(!String.IsNullOrEmpty(Contract.Result())); return m_format; } } public static bool operator ==(CngKeyBlobFormat left, CngKeyBlobFormat right) { if (Object.ReferenceEquals(left, null)) { return Object.ReferenceEquals(right, null); } return left.Equals(right); } [Pure] public static bool operator !=(CngKeyBlobFormat left, CngKeyBlobFormat right) { if (Object.ReferenceEquals(left, null)) { return !Object.ReferenceEquals(right, null); } return !left.Equals(right); } public override bool Equals(object obj) { Contract.Assert(m_format != null); return Equals(obj as CngKeyBlobFormat); } public bool Equals(CngKeyBlobFormat other) { if (Object.ReferenceEquals(other, null)) { return false; } return m_format.Equals(other.Format); } public override int GetHashCode() { Contract.Assert(m_format != null); return m_format.GetHashCode(); } public override string ToString() { Contract.Assert(m_format != null); return m_format; } // // Well known key blob formats // public static CngKeyBlobFormat EccPrivateBlob { get { Contract.Ensures(Contract.Result () != null); if (s_eccPrivate == null) { s_eccPrivate = new CngKeyBlobFormat("ECCPRIVATEBLOB"); // BCRYPT_ECCPRIVATE_BLOB } return s_eccPrivate; } } public static CngKeyBlobFormat EccPublicBlob { get { Contract.Ensures(Contract.Result () != null); if (s_eccPublic == null) { s_eccPublic = new CngKeyBlobFormat("ECCPUBLICBLOB"); // BCRYPT_ECCPUBLIC_BLOB } return s_eccPublic; } } public static CngKeyBlobFormat GenericPrivateBlob { get { Contract.Ensures(Contract.Result () != null); if (s_genericPrivate == null) { s_genericPrivate = new CngKeyBlobFormat("PRIVATEBLOB"); // BCRYPT_PRIVATE_KEY_BLOB } return s_genericPrivate; } } public static CngKeyBlobFormat GenericPublicBlob { get { Contract.Ensures(Contract.Result () != null); if (s_genericPublic == null) { s_genericPublic = new CngKeyBlobFormat("PUBLICBLOB"); // BCRYPT_PUBLIC_KEY_BLOB } return s_genericPublic; } } public static CngKeyBlobFormat OpaqueTransportBlob { get { Contract.Ensures(Contract.Result () != null); if (s_opaqueTransport == null) { s_opaqueTransport = new CngKeyBlobFormat("OpaqueTransport"); // NCRYPT_OPAQUETRANSPORT_BLOB } return s_opaqueTransport; } } public static CngKeyBlobFormat Pkcs8PrivateBlob { get { Contract.Ensures(Contract.Result () != null); if (s_pkcs8Private == null) { s_pkcs8Private = new CngKeyBlobFormat("PKCS8_PRIVATEKEY"); // NCRYPT_PKCS8_PRIVATE_KEY_BLOB } return s_pkcs8Private; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics.Contracts; namespace System.Security.Cryptography { /// /// Utility class to strongly type the format of key blobs used with CNG. Since all CNG APIs which /// require or return a key blob format take the name as a string, we use this string wrapper class to /// specifically mark which parameters and return values are expected to be key blob formats. We also /// provide a list of well known blob formats, which helps Intellisense users find a set of good blob /// formats to use. /// [Serializable] [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CngKeyBlobFormat : IEquatable{ private static CngKeyBlobFormat s_eccPrivate; private static CngKeyBlobFormat s_eccPublic; private static CngKeyBlobFormat s_genericPrivate; private static CngKeyBlobFormat s_genericPublic; private static CngKeyBlobFormat s_opaqueTransport; private static CngKeyBlobFormat s_pkcs8Private; private string m_format; public CngKeyBlobFormat(string format) { Contract.Ensures(!String.IsNullOrEmpty(m_format)); if (format == null) { throw new ArgumentNullException("format"); } if (format.Length == 0) { throw new ArgumentException(SR.GetString(SR.Cryptography_InvalidKeyBlobFormat, format), "format"); } m_format = format; } /// /// Name of the blob format /// public string Format { get { Contract.Ensures(!String.IsNullOrEmpty(Contract.Result())); return m_format; } } public static bool operator ==(CngKeyBlobFormat left, CngKeyBlobFormat right) { if (Object.ReferenceEquals(left, null)) { return Object.ReferenceEquals(right, null); } return left.Equals(right); } [Pure] public static bool operator !=(CngKeyBlobFormat left, CngKeyBlobFormat right) { if (Object.ReferenceEquals(left, null)) { return !Object.ReferenceEquals(right, null); } return !left.Equals(right); } public override bool Equals(object obj) { Contract.Assert(m_format != null); return Equals(obj as CngKeyBlobFormat); } public bool Equals(CngKeyBlobFormat other) { if (Object.ReferenceEquals(other, null)) { return false; } return m_format.Equals(other.Format); } public override int GetHashCode() { Contract.Assert(m_format != null); return m_format.GetHashCode(); } public override string ToString() { Contract.Assert(m_format != null); return m_format; } // // Well known key blob formats // public static CngKeyBlobFormat EccPrivateBlob { get { Contract.Ensures(Contract.Result () != null); if (s_eccPrivate == null) { s_eccPrivate = new CngKeyBlobFormat("ECCPRIVATEBLOB"); // BCRYPT_ECCPRIVATE_BLOB } return s_eccPrivate; } } public static CngKeyBlobFormat EccPublicBlob { get { Contract.Ensures(Contract.Result () != null); if (s_eccPublic == null) { s_eccPublic = new CngKeyBlobFormat("ECCPUBLICBLOB"); // BCRYPT_ECCPUBLIC_BLOB } return s_eccPublic; } } public static CngKeyBlobFormat GenericPrivateBlob { get { Contract.Ensures(Contract.Result () != null); if (s_genericPrivate == null) { s_genericPrivate = new CngKeyBlobFormat("PRIVATEBLOB"); // BCRYPT_PRIVATE_KEY_BLOB } return s_genericPrivate; } } public static CngKeyBlobFormat GenericPublicBlob { get { Contract.Ensures(Contract.Result () != null); if (s_genericPublic == null) { s_genericPublic = new CngKeyBlobFormat("PUBLICBLOB"); // BCRYPT_PUBLIC_KEY_BLOB } return s_genericPublic; } } public static CngKeyBlobFormat OpaqueTransportBlob { get { Contract.Ensures(Contract.Result () != null); if (s_opaqueTransport == null) { s_opaqueTransport = new CngKeyBlobFormat("OpaqueTransport"); // NCRYPT_OPAQUETRANSPORT_BLOB } return s_opaqueTransport; } } public static CngKeyBlobFormat Pkcs8PrivateBlob { get { Contract.Ensures(Contract.Result () != null); if (s_pkcs8Private == null) { s_pkcs8Private = new CngKeyBlobFormat("PKCS8_PRIVATEKEY"); // NCRYPT_PKCS8_PRIVATE_KEY_BLOB } return s_pkcs8Private; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
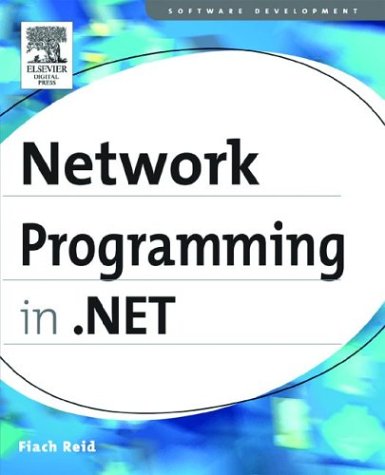
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Color.cs
- Queue.cs
- Validator.cs
- StorageEntityTypeMapping.cs
- ModuleBuilder.cs
- DefaultPropertyAttribute.cs
- storepermissionattribute.cs
- GenericAuthenticationEventArgs.cs
- SocketAddress.cs
- relpropertyhelper.cs
- WpfKnownMember.cs
- AppSettingsSection.cs
- CryptoConfig.cs
- TextProperties.cs
- ChannelTracker.cs
- AspNetPartialTrustHelpers.cs
- MaterialGroup.cs
- ChameleonKey.cs
- BinaryWriter.cs
- LoginUtil.cs
- VisualStateChangedEventArgs.cs
- Accessible.cs
- ObjectNotFoundException.cs
- CommandDesigner.cs
- ItemsControl.cs
- Win32KeyboardDevice.cs
- EncodingDataItem.cs
- FilteredXmlReader.cs
- CollectionExtensions.cs
- MatchingStyle.cs
- DesignerLoader.cs
- ScriptingWebServicesSectionGroup.cs
- BasePattern.cs
- TextBoxAutoCompleteSourceConverter.cs
- PkcsUtils.cs
- ToolboxItem.cs
- ResourceAssociationSetEnd.cs
- DataGridItem.cs
- FilterableAttribute.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- XslAstAnalyzer.cs
- WhitespaceRuleLookup.cs
- GridViewAutomationPeer.cs
- DataGridClipboardHelper.cs
- SQLInt64Storage.cs
- CollectionViewGroupRoot.cs
- IdentifierService.cs
- XPathBuilder.cs
- CompressedStack.cs
- DataServiceProviderWrapper.cs
- OdbcHandle.cs
- TextElementEnumerator.cs
- TypeDelegator.cs
- ErrorProvider.cs
- RoutedCommand.cs
- WebBrowser.cs
- CoTaskMemUnicodeSafeHandle.cs
- SEHException.cs
- OleDbStruct.cs
- Executor.cs
- METAHEADER.cs
- SamlAction.cs
- TileBrush.cs
- HMAC.cs
- WinFormsComponentEditor.cs
- DateTimeOffset.cs
- OperationDescriptionCollection.cs
- FunctionImportElement.cs
- Glyph.cs
- XmlSchemaType.cs
- IChannel.cs
- FileInfo.cs
- SqlServices.cs
- SecurityChannelFaultConverter.cs
- StringAnimationBase.cs
- pingexception.cs
- CodeMemberMethod.cs
- DefaultValueMapping.cs
- SmiContext.cs
- EntityViewContainer.cs
- XmlSchemaSubstitutionGroup.cs
- CreateUserErrorEventArgs.cs
- Transactions.cs
- InstanceDataCollection.cs
- DependencyPropertyHelper.cs
- PersonalizableAttribute.cs
- ScrollPatternIdentifiers.cs
- SafeLocalMemHandle.cs
- DbConnectionInternal.cs
- EntityCommandExecutionException.cs
- XPathNode.cs
- TypeTypeConverter.cs
- IteratorDescriptor.cs
- CryptoConfig.cs
- EntityReference.cs
- SBCSCodePageEncoding.cs
- ImageFormat.cs
- SocketException.cs
- relpropertyhelper.cs
- Events.cs