Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / Behavior / Glyph.cs / 1 / Glyph.cs
namespace System.Windows.Forms.Design.Behavior { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Windows.Forms.Design; ////// /// A Glyph represents a single UI entity managed by an Adorner. A Glyph /// does not have an HWnd - and is rendered on the BehaviorService's /// AdornerWindow control. Each Glyph can have a Behavior associated with /// it - the idea here is that a successfully Hit-Tested Glyph has the /// opportunity to 'push' a new/different Behavior onto the BehaviorService's /// BehaviorStack. Note that all Glyphs really do is paint and hit test. /// public abstract class Glyph { private Behavior behavior;//the Behaivor associated with the Glyph - can be null. ////// /// Glyph's default constructor takes a Behavior. /// protected Glyph(Behavior behavior) { this.behavior = behavior; } ////// /// This read-only property will return the Behavior associated with /// this Glyph. The Behavior can be null. /// public virtual Behavior Behavior{ get { return behavior; } } ////// /// This read-only property will return the Bounds associated with /// this Glyph. The Bounds can be empty. /// public virtual Rectangle Bounds { get { return Rectangle.Empty; } } ////// /// Abstract method that forces Glyph implementations to provide /// hit test logic. Given any point - if the Glyph has decided to /// be involved with that location, the Glyph will need to return /// a valid Cursor. Otherwise, returning null will cause the /// the BehaviorService to simply ignore it. /// public abstract Cursor GetHitTest(Point p); ////// /// Abstract method that forces Glyph implementations to provide /// paint logic. The PaintEventArgs object passed into this method /// contains the Graphics object related to the BehaviorService's /// AdornerWindow. /// public abstract void Paint(PaintEventArgs pe); ////// /// This method is called by inheriting classes to change the /// Behavior object associated with the Glyph. /// [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate")] protected void SetBehavior(Behavior behavior) { this.behavior = behavior; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
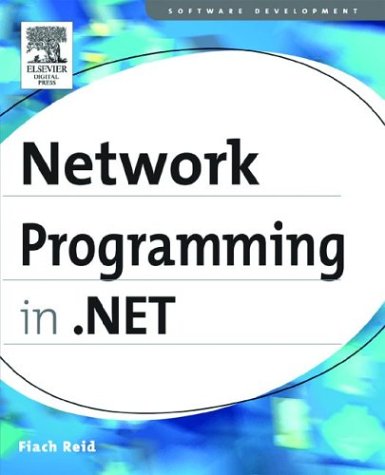
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FileLogRecordStream.cs
- CAGDesigner.cs
- ThrowHelper.cs
- ProfilePropertySettingsCollection.cs
- NetworkStream.cs
- NumericUpDown.cs
- PermissionListSet.cs
- Grant.cs
- DataViewManager.cs
- XslException.cs
- BaseParaClient.cs
- MimeParameterWriter.cs
- counter.cs
- StylusPointPropertyId.cs
- DataBoundLiteralControl.cs
- SharedTcpTransportManager.cs
- HTMLTextWriter.cs
- TaskExceptionHolder.cs
- XmlSchemaAnyAttribute.cs
- DispatcherExceptionEventArgs.cs
- LinkedDataMemberFieldEditor.cs
- TextEffect.cs
- CompensatableSequenceActivity.cs
- DesignerSerializerAttribute.cs
- QueryResponse.cs
- ByteStack.cs
- Style.cs
- VectorCollection.cs
- COAUTHINFO.cs
- Type.cs
- LoginCancelEventArgs.cs
- GenericEnumConverter.cs
- ProfileSettings.cs
- NavigatorOutput.cs
- EmptyElement.cs
- TextTreeUndoUnit.cs
- BindingListCollectionView.cs
- ClientBuildManagerCallback.cs
- TimeSpanStorage.cs
- KeyPullup.cs
- WindowsEditBox.cs
- DataGridViewBand.cs
- Activator.cs
- DynamicControlParameter.cs
- QueryExpr.cs
- SqlAggregateChecker.cs
- ComponentConverter.cs
- KerberosRequestorSecurityToken.cs
- Models.cs
- IsolatedStorageException.cs
- DataTablePropertyDescriptor.cs
- PaperSize.cs
- CryptoHelper.cs
- WebBrowserNavigatingEventHandler.cs
- OracleException.cs
- NamedPipeHostedTransportConfiguration.cs
- JsonClassDataContract.cs
- CompositionTarget.cs
- ResourceAssociationSetEnd.cs
- ServicePointManagerElement.cs
- COM2Properties.cs
- Normalization.cs
- ExtendedPropertyCollection.cs
- FormViewDeleteEventArgs.cs
- SqlCacheDependencyDatabaseCollection.cs
- ImportContext.cs
- TextBoxBase.cs
- Highlights.cs
- ModuleBuilderData.cs
- FileIOPermission.cs
- DoubleLinkList.cs
- EnumMember.cs
- PolyLineSegmentFigureLogic.cs
- LocalizabilityAttribute.cs
- XmlSchemaExporter.cs
- LayoutDump.cs
- StateItem.cs
- RectangleGeometry.cs
- StackOverflowException.cs
- DesignSurfaceManager.cs
- ButtonField.cs
- PositiveTimeSpanValidator.cs
- Native.cs
- SerialPort.cs
- EventLogPermission.cs
- Connector.cs
- TreeNodeSelectionProcessor.cs
- XmlNamespaceDeclarationsAttribute.cs
- RangeValueProviderWrapper.cs
- SyndicationSerializer.cs
- TextTreeRootNode.cs
- ConvertTextFrag.cs
- LogExtentCollection.cs
- ItemDragEvent.cs
- SubMenuStyleCollection.cs
- ADMembershipUser.cs
- IncrementalHitTester.cs
- PropertyTab.cs
- Point.cs
- Substitution.cs