Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / Primitives / RangeBase.cs / 1305600 / RangeBase.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Windows.Threading; using System.Windows.Automation.Peers; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows; using System.Windows.Input; using System.Windows.Media; using MS.Internal; using MS.Utility; namespace System.Windows.Controls.Primitives { ////// The RangeBase class is the base class from which all "range-like" /// controls derive. It defines the relevant events and properties, as /// well as providing handlers for the relevant input events. /// ///[DefaultEvent("ValueChanged"), DefaultProperty("Value")] public abstract class RangeBase : Control { #region Constructors /// /// This is the static constructor for the RangeBase class. It /// hooks the changed notifications needed for visual state changes. /// static RangeBase() { IsEnabledProperty.OverrideMetadata(typeof(RangeBase), new UIPropertyMetadata(new PropertyChangedCallback(OnVisualStatePropertyChanged))); IsMouseOverPropertyKey.OverrideMetadata(typeof(RangeBase), new UIPropertyMetadata(new PropertyChangedCallback(OnVisualStatePropertyChanged))); } ////// Default RangeBase constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// protected RangeBase() { } #endregion Constructors #region Events ////// Event correspond to Value changed event /// public static readonly RoutedEvent ValueChangedEvent = EventManager.RegisterRoutedEvent("ValueChanged", RoutingStrategy.Bubble, typeof(RoutedPropertyChangedEventHandler), typeof(RangeBase)); /// /// Add / Remove ValueChangedEvent handler /// [Category("Behavior")] public event RoutedPropertyChangedEventHandlerValueChanged { add { AddHandler(ValueChangedEvent, value); } remove { RemoveHandler(ValueChangedEvent, value); } } #endregion Events #region Properties /// /// The DependencyProperty for the Minimum property. /// Flags: none /// Default Value: 0 /// public static readonly DependencyProperty MinimumProperty = DependencyProperty.Register( "Minimum", typeof(double), typeof(RangeBase), new FrameworkPropertyMetadata( 0.0d, new PropertyChangedCallback(OnMinimumChanged)), new ValidateValueCallback(IsValidDoubleValue)); ////// Minimum restricts the minimum value of the Value property /// [Bindable(true), Category("Behavior")] public double Minimum { get { return (double) GetValue(MinimumProperty); } set { SetValue(MinimumProperty, value); } } ////// Called when MinimumProperty is changed on "d." /// private static void OnMinimumChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { RangeBase ctrl = (RangeBase) d; RangeBaseAutomationPeer peer = UIElementAutomationPeer.FromElement(ctrl) as RangeBaseAutomationPeer; if (peer != null) { peer.RaiseMinimumPropertyChangedEvent((double)e.OldValue, (double)e.NewValue); } ctrl.CoerceValue(MaximumProperty); ctrl.CoerceValue(ValueProperty); ctrl.OnMinimumChanged((double)e.OldValue, (double)e.NewValue); } ////// This method is invoked when the Minimum property changes. /// /// The old value of the Minimum property. /// The new value of the Minimum property. protected virtual void OnMinimumChanged(double oldMinimum, double newMinimum) { } ////// The DependencyProperty for the Maximum property. /// Flags: none /// Default Value: 1 /// public static readonly DependencyProperty MaximumProperty = DependencyProperty.Register( "Maximum", typeof(double), typeof(RangeBase), new FrameworkPropertyMetadata( 1.0d, new PropertyChangedCallback(OnMaximumChanged), new CoerceValueCallback(CoerceMaximum)), new ValidateValueCallback(IsValidDoubleValue)); private static object CoerceMaximum(DependencyObject d, object value) { RangeBase ctrl = (RangeBase) d; double min = ctrl.Minimum; if ((double) value < min) { return min; } return value; } ////// Maximum restricts the maximum value of the Value property /// [Bindable(true), Category("Behavior")] public double Maximum { get { return (double) GetValue(MaximumProperty); } set { SetValue(MaximumProperty, value); } } ////// Called when MaximumProperty is changed on "d." /// private static void OnMaximumChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { RangeBase ctrl = (RangeBase) d; RangeBaseAutomationPeer peer = UIElementAutomationPeer.FromElement(ctrl) as RangeBaseAutomationPeer; if (peer != null) { peer.RaiseMaximumPropertyChangedEvent((double)e.OldValue, (double)e.NewValue); } ctrl.CoerceValue(ValueProperty); ctrl.OnMaximumChanged((double) e.OldValue, (double) e.NewValue); } ////// This method is invoked when the Maximum property changes. /// /// The old value of the Maximum property. /// The new value of the Maximum property. protected virtual void OnMaximumChanged(double oldMaximum, double newMaximum) { } ////// The DependencyProperty for the Value property. /// Flags: None /// Default Value: 0 /// public static readonly DependencyProperty ValueProperty = DependencyProperty.Register( "Value", typeof(double), typeof(RangeBase), new FrameworkPropertyMetadata( 0.0d, FrameworkPropertyMetadataOptions.BindsTwoWayByDefault | FrameworkPropertyMetadataOptions.Journal, new PropertyChangedCallback(OnValueChanged), new CoerceValueCallback(ConstrainToRange)), new ValidateValueCallback(IsValidDoubleValue)); // made this internal because Slider wants to leverage it internal static object ConstrainToRange(DependencyObject d, object value) { RangeBase ctrl = (RangeBase) d; double min = ctrl.Minimum; double v = (double) value; if (v < min) { return min; } double max = ctrl.Maximum; if (v > max) { return max; } return value; } ////// Value property /// [Bindable(true), Category("Behavior")] public double Value { get { return (double) GetValue(ValueProperty); } set { SetValue(ValueProperty, value); } } ////// Called when ValueID is changed on "d." /// private static void OnValueChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { RangeBase ctrl = (RangeBase)d; RangeBaseAutomationPeer peer = UIElementAutomationPeer.FromElement(ctrl) as RangeBaseAutomationPeer; if (peer != null) { peer.RaiseValuePropertyChangedEvent((double)e.OldValue, (double)e.NewValue); } ctrl.OnValueChanged((double) e.OldValue, (double) e.NewValue); } ////// This method is invoked when the Value property changes. /// /// The old value of the Value property. /// The new value of the Value property. protected virtual void OnValueChanged(double oldValue, double newValue) { RoutedPropertyChangedEventArgsargs = new RoutedPropertyChangedEventArgs (oldValue, newValue); args.RoutedEvent=RangeBase.ValueChangedEvent; RaiseEvent(args); } /// /// Validate input value in RangeBase (Minimum, Maximum, and Value). /// /// ///Returns False if value is NaN or NegativeInfinity or PositiveInfinity. Otherwise, returns True. private static bool IsValidDoubleValue(object value) { double d = (double)value; return !(DoubleUtil.IsNaN(d) || double.IsInfinity(d)); } ////// Validate input value in RangeBase (SmallChange and LargeChange). /// /// ///Returns False if value is NaN or NegativeInfinity or PositiveInfinity or negative. Otherwise, returns True. private static bool IsValidChange(object value) { double d = (double)value; return IsValidDoubleValue(value) && d >= 0.0; } #region LargeChange Property ////// The DependencyProperty for the LargeChange property. /// public static readonly DependencyProperty LargeChangeProperty = DependencyProperty.Register("LargeChange", typeof(double), typeof(RangeBase), new FrameworkPropertyMetadata(1.0), new ValidateValueCallback(IsValidChange)); ////// LargeChange property /// [Bindable(true), Category("Behavior")] public double LargeChange { get { return (double)GetValue(LargeChangeProperty); } set { SetValue(LargeChangeProperty, value); } } #endregion #region SmallChange Property ////// The DependencyProperty for the SmallChange property. /// public static readonly DependencyProperty SmallChangeProperty = DependencyProperty.Register("SmallChange", typeof(double), typeof(RangeBase), new FrameworkPropertyMetadata(0.1), new ValidateValueCallback(IsValidChange)); ////// SmallChange property /// [Bindable(true), Category("Behavior")] public double SmallChange { get { return (double)GetValue(SmallChangeProperty); } set { SetValue(SmallChangeProperty, value); } } #endregion #endregion #region Method Overrides internal override void ChangeVisualState(bool useTransitions) { if (!IsEnabled) { VisualStates.GoToState(this, useTransitions, VisualStates.StateDisabled, VisualStates.StateNormal); } else if (IsMouseOver) { VisualStates.GoToState(this, useTransitions, VisualStates.StateMouseOver, VisualStates.StateNormal); } else { VisualStateManager.GoToState(this, VisualStates.StateNormal, useTransitions); } if (IsKeyboardFocused) { VisualStates.GoToState(this, useTransitions, VisualStates.StateFocused, VisualStates.StateUnfocused); } else { VisualStateManager.GoToState(this, VisualStates.StateUnfocused, useTransitions); } base.ChangeVisualState(useTransitions); } ////// Gives a string representation of this object. /// public override string ToString() { string typeText = this.GetType().ToString(); double min = double.NaN; double max = double.NaN; double val = double.NaN; bool valuesDefined = false; // Accessing RangeBase properties may be thread sensitive if (CheckAccess()) { min = Minimum; max = Maximum; val = Value; valuesDefined = true; } else { //Not on dispatcher, try posting to the dispatcher with 20ms timeout Dispatcher.Invoke(DispatcherPriority.Send, new TimeSpan(0, 0, 0, 0, 20), new DispatcherOperationCallback(delegate(object o) { min = Minimum; max = Maximum; val = Value; valuesDefined = true; return null; }), null); } // If min, max, value are defined if (valuesDefined) { return SR.Get(SRID.ToStringFormatString_RangeBase, typeText, min, max, val); } // Not able to access the dispatcher return typeText; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
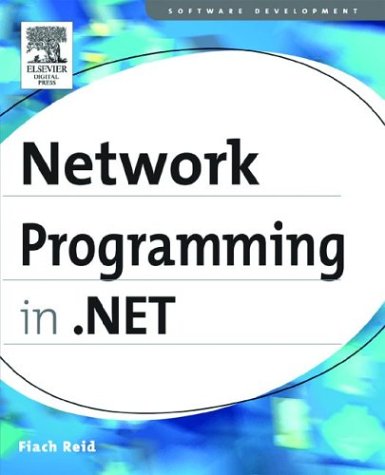
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SwitchCase.cs
- ResourceManagerWrapper.cs
- EntityViewGenerationConstants.cs
- RouteParametersHelper.cs
- ObjectViewQueryResultData.cs
- UpWmlPageAdapter.cs
- OleDbException.cs
- XmlSchemaElement.cs
- PageSettings.cs
- TypeToken.cs
- safesecurityhelperavalon.cs
- DirectoryObjectSecurity.cs
- ErrorHandler.cs
- RelationshipType.cs
- OleDbDataAdapter.cs
- CounterSample.cs
- UnsafeCollabNativeMethods.cs
- MemberAssignmentAnalysis.cs
- HuffCodec.cs
- InvalidateEvent.cs
- DataServices.cs
- DiagnosticsConfigurationHandler.cs
- SynchronizedCollection.cs
- MemberAccessException.cs
- AddInProcess.cs
- Deflater.cs
- MarkupProperty.cs
- WorkflowFileItem.cs
- grammarelement.cs
- WsdlParser.cs
- Span.cs
- UnsafeNativeMethods.cs
- ContentValidator.cs
- BezierSegment.cs
- DataGridPagerStyle.cs
- FontCollection.cs
- CharEntityEncoderFallback.cs
- Random.cs
- XpsResourceDictionary.cs
- SystemIPInterfaceProperties.cs
- WebPartManagerInternals.cs
- ECDsaCng.cs
- ToolStripContentPanelRenderEventArgs.cs
- FloaterParagraph.cs
- ModelPropertyImpl.cs
- ChtmlCommandAdapter.cs
- WebPartCatalogAddVerb.cs
- ExpressionPrefixAttribute.cs
- PrintPreviewControl.cs
- FamilyTypeface.cs
- SegmentInfo.cs
- COM2ColorConverter.cs
- StructuredTypeEmitter.cs
- ThreadStaticAttribute.cs
- Grant.cs
- CssClassPropertyAttribute.cs
- BrushProxy.cs
- ParserContext.cs
- Container.cs
- BindingExpressionUncommonField.cs
- StubHelpers.cs
- ItemsChangedEventArgs.cs
- CqlBlock.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- HtmlControlPersistable.cs
- SqlCacheDependency.cs
- SQLInt32Storage.cs
- DescendantQuery.cs
- SafeMemoryMappedFileHandle.cs
- XmlDictionaryReaderQuotas.cs
- SystemFonts.cs
- StructuredTypeEmitter.cs
- SqlDataSourceSummaryPanel.cs
- TemplateBindingExpressionConverter.cs
- WebServiceClientProxyGenerator.cs
- ToolboxBitmapAttribute.cs
- ConnectionProviderAttribute.cs
- RichTextBoxAutomationPeer.cs
- SizeKeyFrameCollection.cs
- DesignSurfaceEvent.cs
- PropertyNames.cs
- QilPatternVisitor.cs
- BinHexDecoder.cs
- AstNode.cs
- BamlLocalizationDictionary.cs
- LayoutEvent.cs
- TogglePatternIdentifiers.cs
- AsymmetricCryptoHandle.cs
- DataSourceHelper.cs
- ControlBindingsConverter.cs
- StylusEventArgs.cs
- TextTrailingWordEllipsis.cs
- CannotUnloadAppDomainException.cs
- PipeException.cs
- RSAOAEPKeyExchangeFormatter.cs
- RadialGradientBrush.cs
- Stream.cs
- InvalidateEvent.cs
- EventsTab.cs
- PropVariant.cs