Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Core.Presentation / System / Activities / Core / Presentation / ConnectionPoint.cs / 1305376 / ConnectionPoint.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.Activities.Core.Presentation { using System.Windows; using System.Collections.Generic; using System.Windows.Shapes; using System.Diagnostics.CodeAnalysis; using System.Runtime; class ConnectionPoint : UIElement { //private UIElement visual; public static readonly DependencyProperty LocationProperty = DependencyProperty.Register("Location", typeof(Point), typeof(ConnectionPoint)); private ListattachedConnectors; private Point location; private UIElement parentDesigner; private ConnectionPointType pointType; // Size constants for the rectangle drawn internal const double DrawingSmallSide = 4; internal const double DrawingLargeSide = 10; // Size constants for the hit test area. internal const double HitTestSmallSide = 14; internal const double HitTestLargeSide = 20; public ConnectionPoint() { location = new Point(0, 0); pointType = ConnectionPointType.Default; attachedConnectors = new List (); this.parentDesigner = null; } public List AttachedConnectors { get { return this.attachedConnectors; } } public Point Location { get { return (Point)GetValue(ConnectionPoint.LocationProperty); } set { SetValue(ConnectionPoint.LocationProperty, value); } } // This is the vector from the point on the Edge to the topLeft of the rectangle being drawn. public Vector DrawingOffset { get { return GetOffset(DrawingSmallSide, DrawingLargeSide); } } // This is the vector from the point on the Edge to the topLeft of the rectangle being used for hit test. public Vector HitTestOffset { get { return GetOffset(HitTestSmallSide, HitTestLargeSide); } } // This is the size for the rectangle drawn (size is independent of coordinate system) public Size DrawingSize { get { return this.GetSize(ConnectionPoint.DrawingSmallSide, ConnectionPoint.DrawingLargeSide); } } // This is the size for the hit test area (size is independent of coordinate system) public Size HitTestSize { get { return this.GetSize(ConnectionPoint.HitTestSmallSide, ConnectionPoint.HitTestLargeSide); } } public UIElement ParentDesigner { get { return this.parentDesigner; } set { this.parentDesigner = value; } } public ConnectionPointType PointType { get { return this.pointType; } set { this.pointType = value; } } //Gets the list of Points representing the edge for the current ConnectionPoint with respect to the (0,0) of the FreeFormPanel. public List Edge { get { FrameworkElement parent = this.ParentDesigner as FrameworkElement; Fx.Assert(parent != null, "shape should be a FrameworkElement"); Point topLeft = FreeFormPanel.GetLocation(parent); topLeft.Offset(parent.Margin.Left, parent.Margin.Top); double parentWidth = parent.DesiredSize.Width - parent.Margin.Left - parent.Margin.Right; double parentHeight = parent.DesiredSize.Height - parent.Margin.Top - parent.Margin.Bottom; if (this.Location.X == topLeft.X) {//Left Edge return new List { topLeft, new Point(topLeft.X, topLeft.Y + parentHeight) }; } else if (this.Location.X == topLeft.X + parentWidth) {//Right edge return new List { new Point(topLeft.X + parentWidth, topLeft.Y), new Point(topLeft.X + parentWidth, topLeft.Y + parentHeight) }; } else if (this.Location.Y == topLeft.Y) {//Top Edge return new List { topLeft, new Point(topLeft.X + parentWidth, topLeft.Y) }; } else if (this.Location.Y == topLeft.Y + parentHeight) {//Bottom edge return new List { new Point(topLeft.X, topLeft.Y + parentHeight), new Point(topLeft.X + parentWidth, topLeft.Y + parentHeight) }; } return null; } } public EdgeLocation EdgeLocation { get; set; } // This is the vector from the point on the Edge to the topLeft of a rectangle with a particular (small, large) pair. Vector GetOffset(double small, double large) { return this.EdgeToDrawnMidPointOffset() + this.MidPointToTopLeftOffset(small, large); } // This is the vector from the point on the Edge to the topLeft of the "drawn" rectangle. Vector EdgeToDrawnMidPointOffset() { double small = ConnectionPoint.DrawingSmallSide; switch (this.EdgeLocation) { case EdgeLocation.Left: return new Vector(-small / 2, 0); case EdgeLocation.Right: return new Vector(small / 2, 0); case EdgeLocation.Top: return new Vector(0, -small / 2); case EdgeLocation.Bottom: return new Vector(0, small / 2); } Fx.Assert("There is no other possibilities for EdgeDirections"); // To please compiler return new Vector(); } // This is the vector from the midpoint of the rectangle to the topLeft of the rectangle with a particular (small, large) pair. Vector MidPointToTopLeftOffset(double small, double large) { Size rectSize = GetSize(small, large); return new Vector(-rectSize.Width / 2, -rectSize.Height / 2); } // This is the size for the rectangle with a particular (small, large) pair Size GetSize(double small, double large) { if (this.EdgeLocation == EdgeLocation.Left || this.EdgeLocation == EdgeLocation.Right) { return new Size(small, large); } else { return new Size(large, small); } } } enum EdgeLocation { Left, Right, Top, Bottom } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
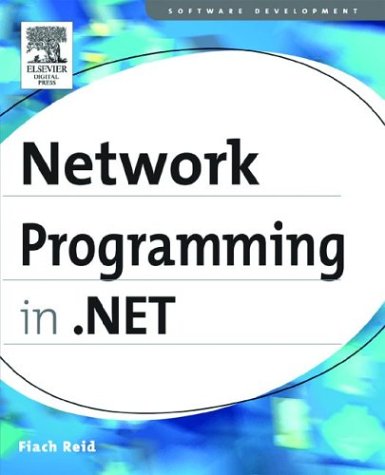
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GregorianCalendar.cs
- FileLogRecordStream.cs
- AddInAdapter.cs
- TaskForm.cs
- ScriptMethodAttribute.cs
- Imaging.cs
- ToolStripItem.cs
- StreamHelper.cs
- SchemaCompiler.cs
- AssemblyBuilderData.cs
- ApplicationBuildProvider.cs
- MaterialGroup.cs
- ViewStateException.cs
- PkcsUtils.cs
- SocketException.cs
- WebConfigurationFileMap.cs
- StringCollection.cs
- EDesignUtil.cs
- SqlDataSourceSummaryPanel.cs
- WebPageTraceListener.cs
- XmlSchemaComplexType.cs
- TrueReadOnlyCollection.cs
- ColorPalette.cs
- FormParameter.cs
- PrivateFontCollection.cs
- TableCell.cs
- CursorInteropHelper.cs
- RbTree.cs
- SqlDataAdapter.cs
- SecurityUniqueId.cs
- DecimalConverter.cs
- ViewStateModeByIdAttribute.cs
- MyContact.cs
- Base64Stream.cs
- WindowsUpDown.cs
- SystemNetworkInterface.cs
- XslCompiledTransform.cs
- ResXResourceSet.cs
- DbConvert.cs
- SQLCharsStorage.cs
- DecimalStorage.cs
- AuthorizationRuleCollection.cs
- URLString.cs
- EventSource.cs
- Nodes.cs
- HideDisabledControlAdapter.cs
- RepeatInfo.cs
- FileUtil.cs
- DetailsViewModeEventArgs.cs
- ListParagraph.cs
- ItemList.cs
- WhitespaceSignificantCollectionAttribute.cs
- EntityTransaction.cs
- CompiledQuery.cs
- PersonalizationStateInfoCollection.cs
- VerbConverter.cs
- DES.cs
- FillRuleValidation.cs
- RepeaterItemEventArgs.cs
- UserUseLicenseDictionaryLoader.cs
- JsonEncodingStreamWrapper.cs
- XmlFileEditor.cs
- TextDecoration.cs
- DynamicMetaObject.cs
- AuthorizationRuleCollection.cs
- HtmlInputImage.cs
- ElementFactory.cs
- AssertUtility.cs
- DesignerExtenders.cs
- Scene3D.cs
- PolicyUtility.cs
- EmbossBitmapEffect.cs
- SelectedDatesCollection.cs
- ZipIORawDataFileBlock.cs
- LassoSelectionBehavior.cs
- UIPropertyMetadata.cs
- SharedHttpTransportManager.cs
- UnknownWrapper.cs
- DebugControllerThread.cs
- ProgressBarHighlightConverter.cs
- VerticalAlignConverter.cs
- FactoryRecord.cs
- ItemList.cs
- ContainerParagraph.cs
- Material.cs
- Subset.cs
- StylusLogic.cs
- FrameworkObject.cs
- NavigatorInput.cs
- Rotation3D.cs
- FigureHelper.cs
- SizeAnimationUsingKeyFrames.cs
- CmsInterop.cs
- FormViewDeletedEventArgs.cs
- SByteStorage.cs
- PathNode.cs
- AssemblyBuilderData.cs
- GetPageNumberCompletedEventArgs.cs
- Queue.cs
- MexHttpsBindingElement.cs