Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Imaging / BitmapPalettes.cs / 1305600 / BitmapPalettes.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All rights reserved. // // File: BitmapPalettes.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; namespace System.Windows.Media.Imaging { #region BitmapPalettes ////// Pre-defined palette types /// static public class BitmapPalettes { ////// BlackAndWhite /// static public Imaging.BitmapPalette BlackAndWhite { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedBW, false); } } ////// BlackAndWhiteTransparent /// static public Imaging.BitmapPalette BlackAndWhiteTransparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedBW, true); } } ////// Halftone8 /// static public Imaging.BitmapPalette Halftone8 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone8, false); } } ////// Halftone8Transparent /// static public Imaging.BitmapPalette Halftone8Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone8, true); } } ////// Halftone27 /// static public Imaging.BitmapPalette Halftone27 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone27, false); } } ////// Halftone27Transparent /// static public Imaging.BitmapPalette Halftone27Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone27, true); } } ////// Halftone64 /// static public Imaging.BitmapPalette Halftone64 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone64, false); } } ////// Halftone64Transparent /// static public Imaging.BitmapPalette Halftone64Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone64, true); } } ////// Halftone125 /// static public Imaging.BitmapPalette Halftone125 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone125, false); } } ////// Halftone125Transparent /// static public Imaging.BitmapPalette Halftone125Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone125, true); } } ////// Halftone216 /// static public Imaging.BitmapPalette Halftone216 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone216, false); } } ////// Halftone216Transparent /// static public Imaging.BitmapPalette Halftone216Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone216, true); } } ////// Halftone252 /// static public Imaging.BitmapPalette Halftone252 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone252, false); } } ////// Halftone252Transparent /// static public Imaging.BitmapPalette Halftone252Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone252, true); } } ////// Halftone256 /// static public Imaging.BitmapPalette Halftone256 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone256, false); } } ////// Halftone256Transparent /// static public Imaging.BitmapPalette Halftone256Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone256, true); } } ////// Gray4 /// static public Imaging.BitmapPalette Gray4 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedGray4, false); } } ////// Gray4Transparent /// static public Imaging.BitmapPalette Gray4Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedGray4, true); } } ////// Gray16 /// static public Imaging.BitmapPalette Gray16 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedGray16, false); } } ////// Gray16Transparent /// static public Imaging.BitmapPalette Gray16Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedGray16, true); } } ////// Gray256 /// static public Imaging.BitmapPalette Gray256 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedGray256, false); } } ////// Gray256Transparent /// static public Imaging.BitmapPalette Gray256Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedGray256, true); } } ////// WebPalette /// static public Imaging.BitmapPalette WebPalette { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone216, false); } } ////// WebpaletteTransparent /// static public Imaging.BitmapPalette WebPaletteTransparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone216, true); } } static internal Imaging.BitmapPalette FromMILPaletteType(WICPaletteType type, bool hasAlpha) { int key = (int)type; Debug.Assert(key < c_maxPalettes); Imaging.BitmapPalette palette; Imaging.BitmapPalette[] palettes; if (hasAlpha) { palettes = transparentPalettes; } else { palettes = opaquePalettes; } palette = palettes[key]; if (palette == null) { lock (palettes) { // palettes might have changed while waiting for the lock. // Need to check again. palette = palettes[key]; if (palette == null) { palette = new Imaging.BitmapPalette(type, hasAlpha); palettes[key] = palette; } } } return palette; } static private Imaging.BitmapPalette[] transparentPalettes { get { if (s_transparentPalettes == null) { s_transparentPalettes = new Imaging.BitmapPalette[c_maxPalettes]; } return s_transparentPalettes; } } static private Imaging.BitmapPalette[] opaquePalettes { get { if (s_opaquePalettes == null) { s_opaquePalettes = new Imaging.BitmapPalette[c_maxPalettes]; } return s_opaquePalettes; } } static private Imaging.BitmapPalette[] s_transparentPalettes; static private Imaging.BitmapPalette[] s_opaquePalettes; private const int c_maxPalettes = 64; } #endregion // Imaging.BitmapPalettes } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All rights reserved. // // File: BitmapPalettes.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; namespace System.Windows.Media.Imaging { #region BitmapPalettes ////// Pre-defined palette types /// static public class BitmapPalettes { ////// BlackAndWhite /// static public Imaging.BitmapPalette BlackAndWhite { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedBW, false); } } ////// BlackAndWhiteTransparent /// static public Imaging.BitmapPalette BlackAndWhiteTransparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedBW, true); } } ////// Halftone8 /// static public Imaging.BitmapPalette Halftone8 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone8, false); } } ////// Halftone8Transparent /// static public Imaging.BitmapPalette Halftone8Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone8, true); } } ////// Halftone27 /// static public Imaging.BitmapPalette Halftone27 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone27, false); } } ////// Halftone27Transparent /// static public Imaging.BitmapPalette Halftone27Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone27, true); } } ////// Halftone64 /// static public Imaging.BitmapPalette Halftone64 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone64, false); } } ////// Halftone64Transparent /// static public Imaging.BitmapPalette Halftone64Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone64, true); } } ////// Halftone125 /// static public Imaging.BitmapPalette Halftone125 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone125, false); } } ////// Halftone125Transparent /// static public Imaging.BitmapPalette Halftone125Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone125, true); } } ////// Halftone216 /// static public Imaging.BitmapPalette Halftone216 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone216, false); } } ////// Halftone216Transparent /// static public Imaging.BitmapPalette Halftone216Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone216, true); } } ////// Halftone252 /// static public Imaging.BitmapPalette Halftone252 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone252, false); } } ////// Halftone252Transparent /// static public Imaging.BitmapPalette Halftone252Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone252, true); } } ////// Halftone256 /// static public Imaging.BitmapPalette Halftone256 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone256, false); } } ////// Halftone256Transparent /// static public Imaging.BitmapPalette Halftone256Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone256, true); } } ////// Gray4 /// static public Imaging.BitmapPalette Gray4 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedGray4, false); } } ////// Gray4Transparent /// static public Imaging.BitmapPalette Gray4Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedGray4, true); } } ////// Gray16 /// static public Imaging.BitmapPalette Gray16 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedGray16, false); } } ////// Gray16Transparent /// static public Imaging.BitmapPalette Gray16Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedGray16, true); } } ////// Gray256 /// static public Imaging.BitmapPalette Gray256 { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedGray256, false); } } ////// Gray256Transparent /// static public Imaging.BitmapPalette Gray256Transparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedGray256, true); } } ////// WebPalette /// static public Imaging.BitmapPalette WebPalette { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone216, false); } } ////// WebpaletteTransparent /// static public Imaging.BitmapPalette WebPaletteTransparent { get { return BitmapPalettes.FromMILPaletteType(WICPaletteType.WICPaletteTypeFixedHalftone216, true); } } static internal Imaging.BitmapPalette FromMILPaletteType(WICPaletteType type, bool hasAlpha) { int key = (int)type; Debug.Assert(key < c_maxPalettes); Imaging.BitmapPalette palette; Imaging.BitmapPalette[] palettes; if (hasAlpha) { palettes = transparentPalettes; } else { palettes = opaquePalettes; } palette = palettes[key]; if (palette == null) { lock (palettes) { // palettes might have changed while waiting for the lock. // Need to check again. palette = palettes[key]; if (palette == null) { palette = new Imaging.BitmapPalette(type, hasAlpha); palettes[key] = palette; } } } return palette; } static private Imaging.BitmapPalette[] transparentPalettes { get { if (s_transparentPalettes == null) { s_transparentPalettes = new Imaging.BitmapPalette[c_maxPalettes]; } return s_transparentPalettes; } } static private Imaging.BitmapPalette[] opaquePalettes { get { if (s_opaquePalettes == null) { s_opaquePalettes = new Imaging.BitmapPalette[c_maxPalettes]; } return s_opaquePalettes; } } static private Imaging.BitmapPalette[] s_transparentPalettes; static private Imaging.BitmapPalette[] s_opaquePalettes; private const int c_maxPalettes = 64; } #endregion // Imaging.BitmapPalettes } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
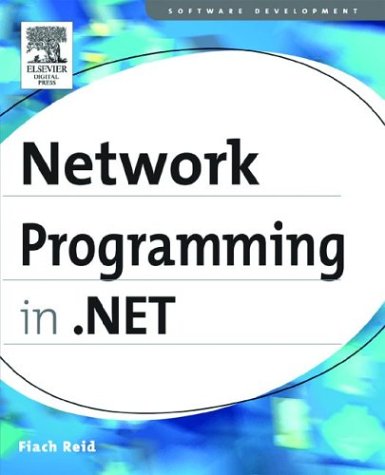
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RadioButton.cs
- Substitution.cs
- UmAlQuraCalendar.cs
- ViewLoader.cs
- UnsafeNativeMethodsTablet.cs
- ComplexTypeEmitter.cs
- Trace.cs
- XmlWrappingWriter.cs
- StringSource.cs
- StoragePropertyMapping.cs
- Model3DGroup.cs
- SequentialUshortCollection.cs
- InputLanguageManager.cs
- OdbcDataAdapter.cs
- CodeDirectionExpression.cs
- RC2.cs
- OptionalColumn.cs
- FacetChecker.cs
- FileStream.cs
- PeerEndPoint.cs
- activationcontext.cs
- CalendarDataBindingHandler.cs
- ConnectionManagementElement.cs
- SerializationSectionGroup.cs
- SpeechUI.cs
- DataBoundControl.cs
- WebPartHeaderCloseVerb.cs
- UrlPropertyAttribute.cs
- OperationCanceledException.cs
- XslException.cs
- Win32.cs
- StrokeCollectionConverter.cs
- FlowLayoutPanelDesigner.cs
- PathSegmentCollection.cs
- FormatterConverter.cs
- IssuanceLicense.cs
- DispatchProxy.cs
- PageSetupDialog.cs
- Model3D.cs
- EventToken.cs
- TreeBuilderXamlTranslator.cs
- AccessDataSource.cs
- srgsitem.cs
- StackSpiller.cs
- XmlParser.cs
- ExeConfigurationFileMap.cs
- LinkedList.cs
- PropertyDescriptorGridEntry.cs
- HttpHostedTransportConfiguration.cs
- XamlRtfConverter.cs
- Light.cs
- TypefaceMetricsCache.cs
- VoiceObjectToken.cs
- TextParagraphCache.cs
- _IPv4Address.cs
- OneWayElement.cs
- MasterPage.cs
- SqlBulkCopyColumnMappingCollection.cs
- Asn1Utilities.cs
- ObfuscateAssemblyAttribute.cs
- SecUtil.cs
- HMACSHA512.cs
- EnumUnknown.cs
- ExpressionBinding.cs
- HttpCookiesSection.cs
- ChannelRequirements.cs
- InheritanceService.cs
- MonitoringDescriptionAttribute.cs
- BigIntegerStorage.cs
- Mappings.cs
- TextEditorDragDrop.cs
- AdornerHitTestResult.cs
- RootProfilePropertySettingsCollection.cs
- AtomParser.cs
- WinEventHandler.cs
- NonSerializedAttribute.cs
- NamespaceEmitter.cs
- OutputCacheSection.cs
- Tablet.cs
- MultiBindingExpression.cs
- Delegate.cs
- ActionMessageFilterTable.cs
- SendMailErrorEventArgs.cs
- LinearKeyFrames.cs
- StringCollection.cs
- StylusButtonEventArgs.cs
- WinFormsComponentEditor.cs
- FirstMatchCodeGroup.cs
- EncodingNLS.cs
- MenuBase.cs
- ProcessModuleDesigner.cs
- DataGridViewSelectedRowCollection.cs
- BindingManagerDataErrorEventArgs.cs
- ColumnHeaderConverter.cs
- UserControlBuildProvider.cs
- CookielessHelper.cs
- TextCharacters.cs
- ObjectViewFactory.cs
- ProfileModule.cs
- EventlogProvider.cs