Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Shared / MS / Internal / SequentialUshortCollection.cs / 1305600 / SequentialUshortCollection.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: A class that implements ICollectionfor a sequence of numbers [0..n-1]. // // // History: // 03/21/2005 : MLeonov - Created it. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal { internal class SequentialUshortCollection : ICollection { public SequentialUshortCollection(ushort count) { _count = count; } #region ICollection Members public void Add(ushort item) { throw new NotSupportedException(); } public void Clear() { throw new NotSupportedException(); } public bool Contains(ushort item) { return item < _count; } public void CopyTo(ushort[] array, int arrayIndex) { if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException(SR.Get(SRID.Collection_BadRank)); } // The extra "arrayIndex >= array.Length" check in because even if _collection.Count // is 0 the index is not allowed to be equal or greater than the length // (from the MSDN ICollection docs) if (arrayIndex < 0 || arrayIndex >= array.Length || (arrayIndex + Count) > array.Length) { throw new ArgumentOutOfRangeException("arrayIndex"); } for (ushort i = 0; i < _count; ++i) array[arrayIndex + i] = i; } public int Count { get { return _count; } } public bool IsReadOnly { get { return true; } } public bool Remove(ushort item) { throw new NotSupportedException(); } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { for (ushort i = 0; i < _count; ++i) yield return i; } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion private ushort _count; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: A class that implements ICollection for a sequence of numbers [0..n-1]. // // // History: // 03/21/2005 : MLeonov - Created it. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal { internal class SequentialUshortCollection : ICollection { public SequentialUshortCollection(ushort count) { _count = count; } #region ICollection Members public void Add(ushort item) { throw new NotSupportedException(); } public void Clear() { throw new NotSupportedException(); } public bool Contains(ushort item) { return item < _count; } public void CopyTo(ushort[] array, int arrayIndex) { if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException(SR.Get(SRID.Collection_BadRank)); } // The extra "arrayIndex >= array.Length" check in because even if _collection.Count // is 0 the index is not allowed to be equal or greater than the length // (from the MSDN ICollection docs) if (arrayIndex < 0 || arrayIndex >= array.Length || (arrayIndex + Count) > array.Length) { throw new ArgumentOutOfRangeException("arrayIndex"); } for (ushort i = 0; i < _count; ++i) array[arrayIndex + i] = i; } public int Count { get { return _count; } } public bool IsReadOnly { get { return true; } } public bool Remove(ushort item) { throw new NotSupportedException(); } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { for (ushort i = 0; i < _count; ++i) yield return i; } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion private ushort _count; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
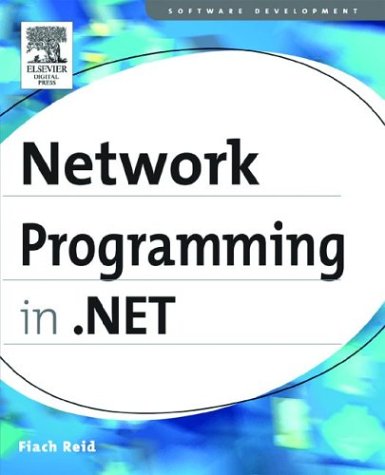
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AssociationTypeEmitter.cs
- ListItemConverter.cs
- HashCodeCombiner.cs
- XsltConvert.cs
- EdmConstants.cs
- CompressionTransform.cs
- LayoutSettings.cs
- DataGridTableCollection.cs
- DecodeHelper.cs
- LineVisual.cs
- UrlPath.cs
- ThemeDirectoryCompiler.cs
- SystemIPv4InterfaceProperties.cs
- MasterPageBuildProvider.cs
- SetStateEventArgs.cs
- EventWaitHandleSecurity.cs
- MouseDevice.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- XhtmlBasicImageAdapter.cs
- SchemaNamespaceManager.cs
- SchemaEntity.cs
- EventListenerClientSide.cs
- TextTreeInsertElementUndoUnit.cs
- WaitForChangedResult.cs
- NullReferenceException.cs
- WS2007FederationHttpBinding.cs
- TimeStampChecker.cs
- _ListenerResponseStream.cs
- XmlSerializationGeneratedCode.cs
- SoundPlayerAction.cs
- StringDictionary.cs
- LicenseContext.cs
- Math.cs
- CompilationUtil.cs
- LineServices.cs
- FragmentQuery.cs
- Decorator.cs
- AbandonedMutexException.cs
- DeclarativeCatalogPart.cs
- CompiledXpathExpr.cs
- CodeTypeDeclarationCollection.cs
- StreamingContext.cs
- ProfilePropertyNameValidator.cs
- WorkflowMarkupSerializationProvider.cs
- WebPermission.cs
- ConfigurationManagerInternalFactory.cs
- arclist.cs
- PolicyManager.cs
- PointAnimationUsingPath.cs
- OracleSqlParser.cs
- TableLayoutSettingsTypeConverter.cs
- _NetworkingPerfCounters.cs
- BitStream.cs
- BrowserCapabilitiesFactoryBase.cs
- dbdatarecord.cs
- InlineObject.cs
- ReadOnlyCollectionBase.cs
- EventHandlersDesigner.cs
- TeredoHelper.cs
- DecoderBestFitFallback.cs
- PartialCachingAttribute.cs
- SafeJobHandle.cs
- StorageBasedPackageProperties.cs
- TabPanel.cs
- ListDataBindEventArgs.cs
- ResourceType.cs
- SynchronizingStream.cs
- Screen.cs
- XmlPropertyBag.cs
- DocumentPageTextView.cs
- DrawingContextDrawingContextWalker.cs
- HtmlHead.cs
- NavigationProperty.cs
- securitycriticaldataClass.cs
- BeginEvent.cs
- InstanceNotReadyException.cs
- SmtpNtlmAuthenticationModule.cs
- AsyncSerializedWorker.cs
- StreamMarshaler.cs
- LoadMessageLogger.cs
- MeasureData.cs
- XdrBuilder.cs
- AssemblyFilter.cs
- CustomPopupPlacement.cs
- ServiceDescription.cs
- BaseValidatorDesigner.cs
- Margins.cs
- CodeTypeMemberCollection.cs
- Operators.cs
- XmlQueryRuntime.cs
- TitleStyle.cs
- WebPartCatalogCloseVerb.cs
- WindowsHyperlink.cs
- Validator.cs
- EntityException.cs
- StubHelpers.cs
- TextHidden.cs
- LicenseException.cs
- RedirectionProxy.cs
- FixedFlowMap.cs