Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / FontEmbeddingManager.cs / 1 / FontEmbeddingManager.cs
//---------------------------------------------------------------------------- // //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: The FontEmbeddingManager class handles physical and composite font embedding. // // See spec at http://avalon/text/DesignDocsAndSpecs/Font%20embedding%20APIs.htm // // // History: // 01/27/2004 : mleonov - Created // //--------------------------------------------------------------------------- using System; using System.Text; using System.IO; using System.Globalization; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using MS.Internal.FontCache; using MS.Internal.FontFace; using MS.Internal.Shaping; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // Allow suppression of presharp warnings #pragma warning disable 1634, 1691 namespace System.Windows.Media { ////// The FontEmbeddingManager class handles physical and composite font embedding. /// public class FontEmbeddingManager { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates a new instance of font usage manager. /// public FontEmbeddingManager() { _collectedGlyphTypefaces = new Dictionary>(_uriComparer); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods /// /// Collects information about glyph typeface and index used by a glyph run. /// /// Glyph run to obtain typeface and index information from. public void RecordUsage(GlyphRun glyphRun) { if (glyphRun == null) throw new ArgumentNullException("glyphRun"); // Suppress PRESharp parameter validation warning about glyphRun.GlyphTypeface because // GlyphRun.GlyphTypeface property cannot be null. #pragma warning suppress 56506 Uri glyphTypeface = glyphRun.GlyphTypeface.FontUri; DictionaryglyphSet; if (_collectedGlyphTypefaces.ContainsKey(glyphTypeface)) glyphSet = _collectedGlyphTypefaces[glyphTypeface]; else glyphSet = _collectedGlyphTypefaces[glyphTypeface] = new Dictionary (); foreach(ushort glyphIndex in glyphRun.GlyphIndices) { glyphSet[glyphIndex] = true; } } /// /// Returns the collection of glyph typefaces used by the previously added glyph runs. /// ///The collection of glyph typefaces used by the previously added glyph runs. [CLSCompliant(false)] public ICollectionGlyphTypefaceUris { get { return _collectedGlyphTypefaces.Keys; } } /// /// Obtain the list of glyphs used by the glyph typeface specified by a Uri. /// /// Specifies the Uri of a glyph typeface to obtain usage data for. ///A collection of glyph indices recorded previously. ////// Glyph typeface Uri does not point to a previously recorded glyph typeface. /// [CLSCompliant(false)] public ICollectionGetUsedGlyphs(Uri glyphTypeface) { Dictionary glyphsUsed = _collectedGlyphTypefaces[glyphTypeface]; if (glyphsUsed == null) { throw new ArgumentException(SR.Get(SRID.GlyphTypefaceNotRecorded), "glyphTypeface"); } return glyphsUsed.Keys; } #endregion Public Methods private class UriComparer : IEqualityComparer { #region IEqualityComparer Members public bool Equals(Uri x, Uri y) { // We don't use Uri.Equals because it doesn't compare Fragment parts, // and we use Fragment part to store font face index. return String.Equals(x.ToString(), y.ToString(), StringComparison.OrdinalIgnoreCase); } public int GetHashCode(Uri obj) { return obj.GetHashCode(); } #endregion } //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields /// /// bool values in the dictionary don't matter, /// we'll switch to Set class when it becomes available. /// private Dictionary> _collectedGlyphTypefaces; private static UriComparer _uriComparer = new UriComparer(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // // Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: The FontEmbeddingManager class handles physical and composite font embedding. // // See spec at http://avalon/text/DesignDocsAndSpecs/Font%20embedding%20APIs.htm // // // History: // 01/27/2004 : mleonov - Created // //--------------------------------------------------------------------------- using System; using System.Text; using System.IO; using System.Globalization; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using MS.Internal.FontCache; using MS.Internal.FontFace; using MS.Internal.Shaping; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // Allow suppression of presharp warnings #pragma warning disable 1634, 1691 namespace System.Windows.Media { ////// The FontEmbeddingManager class handles physical and composite font embedding. /// public class FontEmbeddingManager { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates a new instance of font usage manager. /// public FontEmbeddingManager() { _collectedGlyphTypefaces = new Dictionary>(_uriComparer); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods /// /// Collects information about glyph typeface and index used by a glyph run. /// /// Glyph run to obtain typeface and index information from. public void RecordUsage(GlyphRun glyphRun) { if (glyphRun == null) throw new ArgumentNullException("glyphRun"); // Suppress PRESharp parameter validation warning about glyphRun.GlyphTypeface because // GlyphRun.GlyphTypeface property cannot be null. #pragma warning suppress 56506 Uri glyphTypeface = glyphRun.GlyphTypeface.FontUri; DictionaryglyphSet; if (_collectedGlyphTypefaces.ContainsKey(glyphTypeface)) glyphSet = _collectedGlyphTypefaces[glyphTypeface]; else glyphSet = _collectedGlyphTypefaces[glyphTypeface] = new Dictionary (); foreach(ushort glyphIndex in glyphRun.GlyphIndices) { glyphSet[glyphIndex] = true; } } /// /// Returns the collection of glyph typefaces used by the previously added glyph runs. /// ///The collection of glyph typefaces used by the previously added glyph runs. [CLSCompliant(false)] public ICollectionGlyphTypefaceUris { get { return _collectedGlyphTypefaces.Keys; } } /// /// Obtain the list of glyphs used by the glyph typeface specified by a Uri. /// /// Specifies the Uri of a glyph typeface to obtain usage data for. ///A collection of glyph indices recorded previously. ////// Glyph typeface Uri does not point to a previously recorded glyph typeface. /// [CLSCompliant(false)] public ICollectionGetUsedGlyphs(Uri glyphTypeface) { Dictionary glyphsUsed = _collectedGlyphTypefaces[glyphTypeface]; if (glyphsUsed == null) { throw new ArgumentException(SR.Get(SRID.GlyphTypefaceNotRecorded), "glyphTypeface"); } return glyphsUsed.Keys; } #endregion Public Methods private class UriComparer : IEqualityComparer { #region IEqualityComparer Members public bool Equals(Uri x, Uri y) { // We don't use Uri.Equals because it doesn't compare Fragment parts, // and we use Fragment part to store font face index. return String.Equals(x.ToString(), y.ToString(), StringComparison.OrdinalIgnoreCase); } public int GetHashCode(Uri obj) { return obj.GetHashCode(); } #endregion } //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields /// /// bool values in the dictionary don't matter, /// we'll switch to Set class when it becomes available. /// private Dictionary> _collectedGlyphTypefaces; private static UriComparer _uriComparer = new UriComparer(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
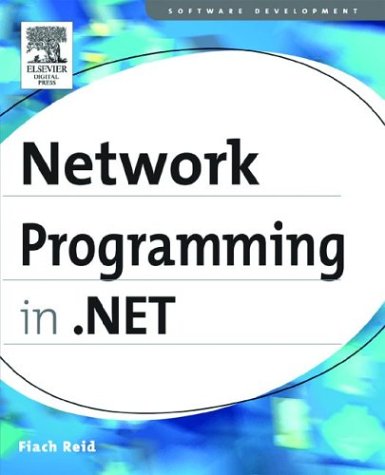
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormsAuthenticationUser.cs
- ActivityBuilderXamlWriter.cs
- CopyAction.cs
- PointLightBase.cs
- CompiledQueryCacheKey.cs
- CredentialManagerDialog.cs
- DesignerDataTable.cs
- ContractMapping.cs
- Array.cs
- DrawingBrush.cs
- BufferedGraphics.cs
- PackageFilter.cs
- ObjectRef.cs
- AtomServiceDocumentSerializer.cs
- UnderstoodHeaders.cs
- ZipIOLocalFileBlock.cs
- BufferedStream.cs
- TextRunCacheImp.cs
- ConnectionsZoneAutoFormat.cs
- AccessDataSourceDesigner.cs
- DocumentSignatureManager.cs
- TypeBinaryExpression.cs
- PaintValueEventArgs.cs
- SchemaSetCompiler.cs
- MediaElementAutomationPeer.cs
- GeneralTransform3DTo2D.cs
- InvokeProviderWrapper.cs
- PrintPageEvent.cs
- ManualResetEventSlim.cs
- PropertyContainer.cs
- UndoUnit.cs
- Membership.cs
- KeyBinding.cs
- InterleavedZipPartStream.cs
- PublishLicense.cs
- TextParaClient.cs
- WebPartChrome.cs
- oledbmetadatacolumnnames.cs
- CancellationToken.cs
- ImplicitInputBrush.cs
- AssociationType.cs
- SecurityChannelFactory.cs
- GridView.cs
- AlphabeticalEnumConverter.cs
- FixedSOMTextRun.cs
- RegexStringValidatorAttribute.cs
- Tuple.cs
- DbConnectionPoolGroup.cs
- ObjectMemberMapping.cs
- TraceUtility.cs
- OpenFileDialog.cs
- AstTree.cs
- NoResizeSelectionBorderGlyph.cs
- GenericUriParser.cs
- XPathDocumentNavigator.cs
- TableLayoutPanel.cs
- DBSqlParser.cs
- GetLastErrorDetailsRequest.cs
- SubpageParaClient.cs
- Point3D.cs
- XmlSchemaAll.cs
- SQLChars.cs
- DetailsViewRowCollection.cs
- HwndKeyboardInputProvider.cs
- TextEvent.cs
- NonBatchDirectoryCompiler.cs
- TaskFileService.cs
- AuthenticatingEventArgs.cs
- ContentPlaceHolder.cs
- QuaternionAnimationBase.cs
- TextEncodedRawTextWriter.cs
- SessionStateSection.cs
- ResourceProperty.cs
- RankException.cs
- ViewValidator.cs
- GroupBox.cs
- DataService.cs
- XmlTextAttribute.cs
- WorkflowApplicationEventArgs.cs
- RequestCachePolicy.cs
- StatusStrip.cs
- ObjectSecurity.cs
- ContentPlaceHolder.cs
- BitmapData.cs
- GroupAggregateExpr.cs
- LinearQuaternionKeyFrame.cs
- ButtonBaseAutomationPeer.cs
- DirectoryObjectSecurity.cs
- TextLineBreak.cs
- FontStyle.cs
- PropertyIDSet.cs
- TemplateControl.cs
- IMembershipProvider.cs
- BoundField.cs
- EdgeModeValidation.cs
- SignatureToken.cs
- mda.cs
- PageHandlerFactory.cs
- XmlUnspecifiedAttribute.cs
- SectionVisual.cs