Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Imaging / BitmapVisualManager.cs / 1305600 / BitmapVisualManager.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: BitmapVisualManager.cs // //----------------------------------------------------------------------------- using System; using System.Windows; using System.Threading; using System.Windows.Threading; using System.Diagnostics; using System.Collections; using System.Runtime.InteropServices; using System.IO; using MS.Internal; using System.Security; using System.Security.Permissions; using System.Windows.Media; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using MS.Win32.PresentationCore; namespace System.Windows.Media.Imaging { #region public class BitmapVisualManager ////// BitmapVisualManager holds state and context for a drawing a visual to an bitmap. /// internal class BitmapVisualManager : DispatcherObject { #region Constructors private BitmapVisualManager() { } ////// Create an BitmapVisualManager for drawing a visual to the bitmap. /// /// Where the resulting bitmap is rendered public BitmapVisualManager(RenderTargetBitmap bitmapTarget) { if (bitmapTarget == null) { throw new ArgumentNullException("bitmapTarget"); } if (bitmapTarget.IsFrozen) { throw new ArgumentException(SR.Get(SRID.Image_CantBeFrozen, null)); } _bitmapTarget = bitmapTarget; } #endregion #region Public methods ////// Render visual to printer. /// /// Root of the visual to render public void Render(Visual visual) { Render(visual, Matrix.Identity, Rect.Empty); } ////// Render visual to printer. /// /// Root of the visual to render /// World transform to apply to the root visual /// The window clip of the outermost window or Empty /// True if we are rendering the visual /// to apply an effect to it /// ////// Critical - Deals with bitmap handles /// TreatAsSafe - validates all parameters, uses safe wrappers /// [SecurityCritical,SecurityTreatAsSafe] internal void Render(Visual visual, Matrix worldTransform, Rect windowClip) { if (visual == null) { throw new ArgumentNullException("visual"); } // If the bitmapTarget we're writing to is frozen then we can't proceed. Note that // it's possible for the BitmapVisualManager to be constructed with a mutable BitmapImage // and for the app to later freeze it. Such an application is misbehaving if // they subsequently try to render to the BitmapImage. if (_bitmapTarget.IsFrozen) { throw new ArgumentException(SR.Get(SRID.Image_CantBeFrozen)); } int sizeX = _bitmapTarget.PixelWidth; int sizeY = _bitmapTarget.PixelHeight; double dpiX = _bitmapTarget.DpiX; double dpiY = _bitmapTarget.DpiY; Debug.Assert ((sizeX > 0) && (sizeY > 0)); Debug.Assert ((dpiX > 0) && (dpiY > 0)); // validate the data if ((sizeX <= 0) || (sizeY <= 0)) { return; // nothing to draw } if ((dpiX <= 0) || (dpiY <= 0)) { dpiX = 96; dpiY = 96; } SafeMILHandle renderTargetBitmap = _bitmapTarget.MILRenderTarget; Debug.Assert (renderTargetBitmap != null, "Render Target is null"); IntPtr pIRenderTargetBitmap = IntPtr.Zero; try { // // Allocate a fresh synchronous channel. // MediaContext mctx = MediaContext.CurrentMediaContext; DUCE.Channel channel = mctx.AllocateSyncChannel(); // // Acquire the target bitmap. // Guid iidRTB = MILGuidData.IID_IMILRenderTargetBitmap; HRESULT.Check(UnsafeNativeMethods.MILUnknown.QueryInterface( renderTargetBitmap, ref iidRTB, out pIRenderTargetBitmap)); // // Render the visual on the synchronous channel. // Renderer.Render( pIRenderTargetBitmap, channel, visual, sizeX, sizeY, dpiX, dpiY, worldTransform, windowClip); // // Release the synchronous channel. This way we can // re-use that channel later. // mctx.ReleaseSyncChannel(channel); } finally { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref pIRenderTargetBitmap); } _bitmapTarget.RenderTargetContentsChanged(); } #endregion #region Member Variables private RenderTargetBitmap _bitmapTarget = null; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: BitmapVisualManager.cs // //----------------------------------------------------------------------------- using System; using System.Windows; using System.Threading; using System.Windows.Threading; using System.Diagnostics; using System.Collections; using System.Runtime.InteropServices; using System.IO; using MS.Internal; using System.Security; using System.Security.Permissions; using System.Windows.Media; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using MS.Win32.PresentationCore; namespace System.Windows.Media.Imaging { #region public class BitmapVisualManager ////// BitmapVisualManager holds state and context for a drawing a visual to an bitmap. /// internal class BitmapVisualManager : DispatcherObject { #region Constructors private BitmapVisualManager() { } ////// Create an BitmapVisualManager for drawing a visual to the bitmap. /// /// Where the resulting bitmap is rendered public BitmapVisualManager(RenderTargetBitmap bitmapTarget) { if (bitmapTarget == null) { throw new ArgumentNullException("bitmapTarget"); } if (bitmapTarget.IsFrozen) { throw new ArgumentException(SR.Get(SRID.Image_CantBeFrozen, null)); } _bitmapTarget = bitmapTarget; } #endregion #region Public methods ////// Render visual to printer. /// /// Root of the visual to render public void Render(Visual visual) { Render(visual, Matrix.Identity, Rect.Empty); } ////// Render visual to printer. /// /// Root of the visual to render /// World transform to apply to the root visual /// The window clip of the outermost window or Empty /// True if we are rendering the visual /// to apply an effect to it /// ////// Critical - Deals with bitmap handles /// TreatAsSafe - validates all parameters, uses safe wrappers /// [SecurityCritical,SecurityTreatAsSafe] internal void Render(Visual visual, Matrix worldTransform, Rect windowClip) { if (visual == null) { throw new ArgumentNullException("visual"); } // If the bitmapTarget we're writing to is frozen then we can't proceed. Note that // it's possible for the BitmapVisualManager to be constructed with a mutable BitmapImage // and for the app to later freeze it. Such an application is misbehaving if // they subsequently try to render to the BitmapImage. if (_bitmapTarget.IsFrozen) { throw new ArgumentException(SR.Get(SRID.Image_CantBeFrozen)); } int sizeX = _bitmapTarget.PixelWidth; int sizeY = _bitmapTarget.PixelHeight; double dpiX = _bitmapTarget.DpiX; double dpiY = _bitmapTarget.DpiY; Debug.Assert ((sizeX > 0) && (sizeY > 0)); Debug.Assert ((dpiX > 0) && (dpiY > 0)); // validate the data if ((sizeX <= 0) || (sizeY <= 0)) { return; // nothing to draw } if ((dpiX <= 0) || (dpiY <= 0)) { dpiX = 96; dpiY = 96; } SafeMILHandle renderTargetBitmap = _bitmapTarget.MILRenderTarget; Debug.Assert (renderTargetBitmap != null, "Render Target is null"); IntPtr pIRenderTargetBitmap = IntPtr.Zero; try { // // Allocate a fresh synchronous channel. // MediaContext mctx = MediaContext.CurrentMediaContext; DUCE.Channel channel = mctx.AllocateSyncChannel(); // // Acquire the target bitmap. // Guid iidRTB = MILGuidData.IID_IMILRenderTargetBitmap; HRESULT.Check(UnsafeNativeMethods.MILUnknown.QueryInterface( renderTargetBitmap, ref iidRTB, out pIRenderTargetBitmap)); // // Render the visual on the synchronous channel. // Renderer.Render( pIRenderTargetBitmap, channel, visual, sizeX, sizeY, dpiX, dpiY, worldTransform, windowClip); // // Release the synchronous channel. This way we can // re-use that channel later. // mctx.ReleaseSyncChannel(channel); } finally { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref pIRenderTargetBitmap); } _bitmapTarget.RenderTargetContentsChanged(); } #endregion #region Member Variables private RenderTargetBitmap _bitmapTarget = null; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
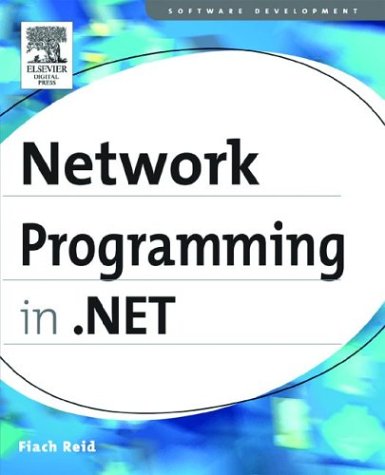
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RNGCryptoServiceProvider.cs
- DeleteStoreRequest.cs
- DataGridViewComboBoxCell.cs
- DataRowChangeEvent.cs
- RepeatButtonAutomationPeer.cs
- VisualTreeFlattener.cs
- OperandQuery.cs
- BinaryWriter.cs
- TransactedBatchingElement.cs
- SHA512Cng.cs
- Rotation3DAnimationUsingKeyFrames.cs
- HashStream.cs
- ButtonRenderer.cs
- metadatamappinghashervisitor.hashsourcebuilder.cs
- XmlNullResolver.cs
- ZipIOExtraFieldZip64Element.cs
- SafeEventLogWriteHandle.cs
- BinaryFormatter.cs
- RandomNumberGenerator.cs
- ReadOnlyMetadataCollection.cs
- DataRow.cs
- TableColumn.cs
- __TransparentProxy.cs
- remotingproxy.cs
- OleDbCommand.cs
- BindUriHelper.cs
- MaterializeFromAtom.cs
- ListChangedEventArgs.cs
- Number.cs
- SubMenuStyleCollection.cs
- OutputScopeManager.cs
- AnonymousIdentificationSection.cs
- TextChangedEventArgs.cs
- BindingSource.cs
- FileDialog_Vista_Interop.cs
- DataGridCell.cs
- WsdlParser.cs
- WmfPlaceableFileHeader.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- PackageRelationship.cs
- MetadataArtifactLoaderCompositeResource.cs
- AgileSafeNativeMemoryHandle.cs
- ScriptManagerProxy.cs
- ControlsConfig.cs
- CodePrimitiveExpression.cs
- ActiveDocumentEvent.cs
- DiscardableAttribute.cs
- OdbcReferenceCollection.cs
- ValueTable.cs
- IResourceProvider.cs
- XmlTextReaderImplHelpers.cs
- ExpressionHelper.cs
- ExtendedPropertyDescriptor.cs
- Compiler.cs
- Stackframe.cs
- ScriptRegistrationManager.cs
- CompiledIdentityConstraint.cs
- FileDialog.cs
- TabItem.cs
- AbstractExpressions.cs
- TimeStampChecker.cs
- ButtonAutomationPeer.cs
- cache.cs
- NavigatorInvalidBodyAccessException.cs
- ParameterReplacerVisitor.cs
- FlowDecision.cs
- TraceSection.cs
- graph.cs
- MediaContext.cs
- XPathException.cs
- DiscoveryInnerClientAdhoc11.cs
- ImageButton.cs
- Route.cs
- TypeSystemHelpers.cs
- PolicyException.cs
- VirtualPath.cs
- ConnectionPoint.cs
- ListSortDescription.cs
- StringAnimationUsingKeyFrames.cs
- CreateUserWizard.cs
- UserPreferenceChangedEventArgs.cs
- BamlLocalizabilityResolver.cs
- QueryConverter.cs
- Misc.cs
- EventEntry.cs
- EncoderParameter.cs
- Baml2006Reader.cs
- SystemException.cs
- ProtocolInformationWriter.cs
- RootBrowserWindow.cs
- PropertyInfoSet.cs
- PaintValueEventArgs.cs
- ApplicationSecurityManager.cs
- PageSetupDialog.cs
- EditModeSwitchButton.cs
- EditCommandColumn.cs
- TextPattern.cs
- StartUpEventArgs.cs
- ServiceBusyException.cs
- Collection.cs