Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / CssStyleCollection.cs / 1 / CssStyleCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.IO; using System.Collections; using System.Collections.Specialized; using System.Reflection; using System.Web.UI; using System.Text; using System.Text.RegularExpressions; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class CssStyleCollection { private static readonly Regex _styleAttribRegex = new Regex( "\\G(\\s*(;\\s*)*" + // match leading semicolons and spaces "(?/// The ////// class contains HTML /// cascading-style sheets (CSS) inline style attributes. It automatically parses /// and exposes CSS properties through a dictionary pattern API. Each CSS key can be /// manipulated using a key/value indexed collection. /// [^:]+?)" + // match stylename - chars up to the semicolon "\\s*:\\s*" + // spaces, then the colon, then more spaces "(? [^;]*)" + // now match styleval ")*\\s*(;\\s*)*$", // match a trailing semicolon and trailing spaces RegexOptions.Singleline | RegexOptions.Multiline | RegexOptions.ExplicitCapture); private StateBag _state; private string _style; private IDictionary _table; private IDictionary _intTable; internal CssStyleCollection() : this(null) { } /* * Constructs an CssStyleCollection given a StateBag. */ internal CssStyleCollection(StateBag state) { _state = state; } /* * Automatically adds new keys. */ /// /// public string this[string key] { get { if (_table == null) ParseString(); string value = (string)_table[key]; if (value == null) { HtmlTextWriterStyle style = CssTextWriter.GetStyleKey(key); if (style != (HtmlTextWriterStyle)(-1)) { value = this[style]; } } return value; } set { Add(key, value); } } ////// Gets or sets a specified CSS value. /// ////// Gets or sets the specified known CSS value. /// public string this[HtmlTextWriterStyle key] { get { if (_intTable == null) { return null; } return (string)_intTable[(int)key]; } set { Add(key, value); } } /* * Returns a collection of keys. */ ////// public ICollection Keys { get { if (_table == null) ParseString(); if (_intTable != null) { // combine the keys into a single table. Note that to preserve existing // behavior, we convert enum values into strings to maintain a homogeneous collection. string[] keys = new string[_table.Count + _intTable.Count]; int i = 0; foreach (string s in _table.Keys) { keys[i] = s; i++; } foreach (HtmlTextWriterStyle style in _intTable.Keys) { keys[i] = CssTextWriter.GetStyleName(style); i++; } return keys; } return _table.Keys; } } ////// Gets a collection of keys to all the styles in the /// ///. /// /// public int Count { get { if (_table == null) ParseString(); return _table.Count + ((_intTable != null) ? _intTable.Count : 0); } } public string Value { get { if (_state == null) { if (_style == null) { _style = BuildString(); } return _style; } return(string)_state["style"]; } set { if (_state == null) { _style = value; } else { _state["style"] = value; } _table = null; } } ////// Gets the number of items in the ///. /// /// public void Add(string key, string value) { if (String.IsNullOrEmpty(key)) { throw new ArgumentNullException("key"); } if (_table == null) ParseString(); _table[key] = value; if (_intTable != null) { // Remove from the other table to avoid duplicates. HtmlTextWriterStyle style = CssTextWriter.GetStyleKey(key); if (style != (HtmlTextWriterStyle)(-1)) { _intTable.Remove(style); } } if (_state != null) { // keep style attribute synchronized _state["style"] = BuildString(); } _style = null; } public void Add(HtmlTextWriterStyle key, string value) { if (_intTable == null) { _intTable = new HybridDictionary(); } _intTable[(int)key] = value; string name = CssTextWriter.GetStyleName(key); if (name.Length != 0) { // Remove from the other table to avoid duplicates. if (_table == null) ParseString(); _table.Remove(name); } if (_state != null) { // keep style attribute synchronized _state["style"] = BuildString(); } _style = null; } ////// Adds a style to the CssStyleCollection. /// ////// public void Remove(string key) { if (_table == null) ParseString(); if (_table[key] != null) { _table.Remove(key); if (_state != null) { // keep style attribute synchronized _state["style"] = BuildString(); } _style = null; } } public void Remove(HtmlTextWriterStyle key) { if (_intTable == null) { return; } _intTable.Remove((int)key); if (_state != null) { // keep style attribute synchronized _state["style"] = BuildString(); } _style = null; } ////// Removes a style from the ///. /// /// public void Clear() { _table = null; _intTable = null; if (_state != null) { _state.Remove("style"); } _style = null; } /* BuildString * Form the style string from data contained in the * hash table */ private string BuildString() { // if the tables are null, there is nothing to build if (((_table == null) || (_table.Count == 0)) && ((_intTable == null) || (_intTable.Count == 0))) { return null; } StringWriter sw = new StringWriter(); CssTextWriter writer = new CssTextWriter(sw); Render(writer); return sw.ToString(); } /* ParseString * Parse the style string and fill the hash table with * corresponding values. */ private void ParseString() { // create a case-insensitive dictionary _table = new HybridDictionary(true); string s = (_state == null) ? _style : (string)_state["style"]; if (s != null) { Match match; if ((match = _styleAttribRegex.Match( s, 0)).Success) { CaptureCollection stylenames = match.Groups["stylename"].Captures; CaptureCollection stylevalues = match.Groups["styleval"].Captures; for (int i = 0; i < stylenames.Count; i++) { String styleName = stylenames[i].ToString(); String styleValue = stylevalues[i].ToString(); _table[styleName] = styleValue; } } } } ////// Removes all styles from the ///. /// /// Render out the attribute collection into a CSS TextWriter. This /// effectively renders the value of an inline style attribute. /// internal void Render(CssTextWriter writer) { if (_table != null && _table.Count > 0) { foreach (DictionaryEntry entry in _table) { writer.WriteAttribute((string)entry.Key, (string)entry.Value); } } if (_intTable != null && _intTable.Count > 0) { foreach (DictionaryEntry entry in _intTable) { writer.WriteAttribute((HtmlTextWriterStyle)entry.Key, (string)entry.Value); } } } ////// Render out the attribute collection into a CSS TextWriter. This /// effectively renders the value of an inline style attribute. /// Used by a Style object to render out its CSS attributes into an HtmlTextWriter. /// internal void Render(HtmlTextWriter writer) { if (_table != null && _table.Count > 0) { foreach (DictionaryEntry entry in _table) { writer.AddStyleAttribute((string)entry.Key, (string)entry.Value); } } if (_intTable != null && _intTable.Count > 0) { foreach (DictionaryEntry entry in _intTable) { writer.AddStyleAttribute((HtmlTextWriterStyle)entry.Key, (string)entry.Value); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
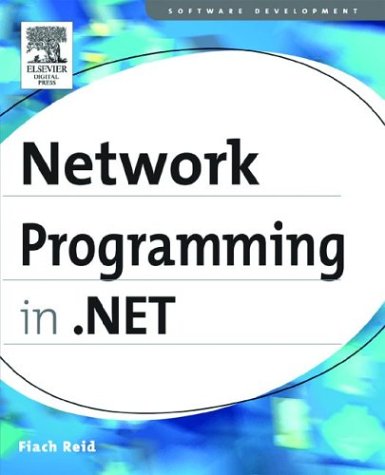
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentAutomationPeer.cs
- addressfiltermode.cs
- XmlSchemaAnnotated.cs
- HashRepartitionEnumerator.cs
- MimeObjectFactory.cs
- XamlPoint3DCollectionSerializer.cs
- TypeLoadException.cs
- QilInvokeLateBound.cs
- TrackingConditionCollection.cs
- SqlVisitor.cs
- InfoCardArgumentException.cs
- Literal.cs
- querybuilder.cs
- LongValidatorAttribute.cs
- FixedElement.cs
- TextEditorDragDrop.cs
- ResourceDisplayNameAttribute.cs
- PoisonMessageException.cs
- OutputCacheModule.cs
- SelectionPatternIdentifiers.cs
- BitmapEffectGeneralTransform.cs
- DataGridViewTextBoxColumn.cs
- NeutralResourcesLanguageAttribute.cs
- TreeView.cs
- ActivityExecutionWorkItem.cs
- ACE.cs
- XmlSchemaRedefine.cs
- _LoggingObject.cs
- TrustManagerMoreInformation.cs
- TimelineCollection.cs
- XmlUnspecifiedAttribute.cs
- RuntimeVariableList.cs
- RuleCache.cs
- ScriptingSectionGroup.cs
- BoundField.cs
- SelfIssuedAuthAsymmetricKey.cs
- BuildResult.cs
- Token.cs
- HttpConfigurationSystem.cs
- PageCodeDomTreeGenerator.cs
- GenericAuthenticationEventArgs.cs
- Translator.cs
- SqlTypesSchemaImporter.cs
- CollectionView.cs
- StatusBarDrawItemEvent.cs
- KeyFrames.cs
- WindowsListViewItemStartMenu.cs
- UserControl.cs
- InkPresenter.cs
- ActionFrame.cs
- FieldToken.cs
- LingerOption.cs
- EntityWrapper.cs
- IntAverageAggregationOperator.cs
- DataObjectCopyingEventArgs.cs
- SaveFileDialogDesigner.cs
- DesignOnlyAttribute.cs
- ErrorHandler.cs
- WebPartAuthorizationEventArgs.cs
- MimeParameter.cs
- CodeTypeReferenceCollection.cs
- XmlSignificantWhitespace.cs
- XmlSchemaComplexContent.cs
- DesignerTransactionCloseEvent.cs
- CompareValidator.cs
- Base64Stream.cs
- ChannelRequirements.cs
- WebPartZoneBase.cs
- LogicalTreeHelper.cs
- LineBreakRecord.cs
- RequestQueue.cs
- ChildrenQuery.cs
- Privilege.cs
- PriorityRange.cs
- RemotingService.cs
- BulletChrome.cs
- NamespaceListProperty.cs
- ExceptionHandlersDesigner.cs
- ResourceCollectionInfo.cs
- DataGridViewAdvancedBorderStyle.cs
- UpDownBase.cs
- SecurityTokenRequirement.cs
- HostedAspNetEnvironment.cs
- PersistChildrenAttribute.cs
- BevelBitmapEffect.cs
- DataColumnMapping.cs
- DecimalFormatter.cs
- CultureSpecificCharacterBufferRange.cs
- SiteMapProvider.cs
- NumberFunctions.cs
- DelegateSerializationHolder.cs
- RegexCharClass.cs
- QueryConverter.cs
- FileLoadException.cs
- SQLDateTime.cs
- XXXOnTypeBuilderInstantiation.cs
- AuthenticationSection.cs
- ListViewTableRow.cs
- AttributeConverter.cs
- FontFamilyIdentifier.cs