Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / Threading / PriorityRange.cs / 1305600 / PriorityRange.cs
using System; using MS.Internal.WindowsBase; namespace System.Windows.Threading { ////// Represents a range of priorities. /// internal struct PriorityRange { ////// The range of all possible priorities. /// public static readonly PriorityRange All = new PriorityRange(DispatcherPriority.Inactive, DispatcherPriority.Send, true); // NOTE: should be Priority ////// A range that includes no priorities. /// public static readonly PriorityRange None = new PriorityRange(DispatcherPriority.Invalid, DispatcherPriority.Invalid, true); // NOTE: should be Priority ////// Constructs an instance of the PriorityRange class. /// public PriorityRange(DispatcherPriority min, DispatcherPriority max) : this() // NOTE: should be Priority { Initialize(min, true, max, true); } ////// Constructs an instance of the PriorityRange class. /// public PriorityRange(DispatcherPriority min, bool isMinInclusive, DispatcherPriority max, bool isMaxInclusive) : this() // NOTE: should be Priority { Initialize(min, isMinInclusive, max, isMaxInclusive); } ////// The minimum priority of this range. /// public DispatcherPriority Min // NOTE: should be Priority { get { return _min; } } ////// The maximum priority of this range. /// public DispatcherPriority Max // NOTE: should be Priority { get { return _max; } } ////// Whether or not the minimum priority in included in this range. /// public bool IsMinInclusive { get { return _isMinInclusive; } } ////// Whether or not the maximum priority in included in this range. /// public bool IsMaxInclusive { get { return _isMaxInclusive; } } ////// Whether or not this priority range is valid. /// public bool IsValid { get { // return _min != null && _min.IsValid && _max != null && _max.IsValid; return (_min > DispatcherPriority.Invalid && _min <= DispatcherPriority.Send && _max > DispatcherPriority.Invalid && _max <= DispatcherPriority.Send); } } ////// Whether or not this priority range contains the specified /// priority. /// public bool Contains(DispatcherPriority priority) // NOTE: should be Priority { /* if (priority == null || !priority.IsValid) { return false; } */ if(priority <= DispatcherPriority.Invalid || priority > DispatcherPriority.Send) { return false; } if (!IsValid) { return false; } bool contains = false; if (_isMinInclusive) { contains = (priority >= _min); } else { contains = (priority > _min); } if (contains) { if (_isMaxInclusive) { contains = (priority <= _max); } else { contains = (priority < _max); } } return contains; } ////// Whether or not this priority range contains the specified /// priority range. /// public bool Contains(PriorityRange priorityRange) { if (!priorityRange.IsValid) { return false; } if (!IsValid) { return false; } bool contains = false; if (priorityRange._isMinInclusive) { contains = Contains(priorityRange.Min); } else { if(priorityRange.Min >= _min && priorityRange.Min < _max) { contains = true; } } if (contains) { if (priorityRange._isMaxInclusive) { contains = Contains(priorityRange.Max); } else { if(priorityRange.Max > _min && priorityRange.Max <= _max) { contains = true; } } } return contains; } ////// Equality method for two PriorityRange /// public override bool Equals(object o) { if(o is PriorityRange) { return Equals((PriorityRange) o); } else { return false; } } ////// Equality method for two PriorityRange /// public bool Equals(PriorityRange priorityRange) { return priorityRange._min == this._min && priorityRange._isMinInclusive == this._isMinInclusive && priorityRange._max == this._max && priorityRange._isMaxInclusive == this._isMaxInclusive; } ////// Equality operator /// public static bool operator== (PriorityRange priorityRange1, PriorityRange priorityRange2) { return priorityRange1.Equals(priorityRange2); } ////// Inequality operator /// public static bool operator!= (PriorityRange priorityRange1, PriorityRange priorityRange2) { return !(priorityRange1 == priorityRange2); } ////// Returns a reasonable hash code for this PriorityRange instance. /// public override int GetHashCode() { return base.GetHashCode(); } private void Initialize(DispatcherPriority min, bool isMinInclusive, DispatcherPriority max, bool isMaxInclusive) // NOTE: should be Priority { /* if(min == null) { throw new ArgumentNullException("min"); } if (!min.IsValid) { throw new ArgumentException("Invalid priority.", "min"); } */ if(min < DispatcherPriority.Invalid || min > DispatcherPriority.Send) { // If we move to a Priority class, this exception will have to change too. throw new System.ComponentModel.InvalidEnumArgumentException("min", (int)min, typeof(DispatcherPriority)); } if(min == DispatcherPriority.Inactive) { throw new ArgumentException(SR.Get(SRID.InvalidPriority), "min"); } /* if(max == null) { throw new ArgumentNullException("max"); } if (!max.IsValid) { throw new ArgumentException("Invalid priority.", "max"); } */ if(max < DispatcherPriority.Invalid || max > DispatcherPriority.Send) { // If we move to a Priority class, this exception will have to change too. throw new System.ComponentModel.InvalidEnumArgumentException("max", (int)max, typeof(DispatcherPriority)); } if(max == DispatcherPriority.Inactive) { throw new ArgumentException(SR.Get(SRID.InvalidPriority), "max"); } if (max < min) { throw new ArgumentException(SR.Get(SRID.InvalidPriorityRangeOrder)); } _min = min; _isMinInclusive = isMinInclusive; _max = max; _isMaxInclusive = isMaxInclusive; } // This is a constructor for our special static members. private PriorityRange(DispatcherPriority min, DispatcherPriority max, bool ignored) // NOTE: should be Priority { _min = min; _isMinInclusive = true; _max = max; _isMaxInclusive = true; } private DispatcherPriority _min; // NOTE: should be Priority private bool _isMinInclusive; private DispatcherPriority _max; // NOTE: should be Priority private bool _isMaxInclusive; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
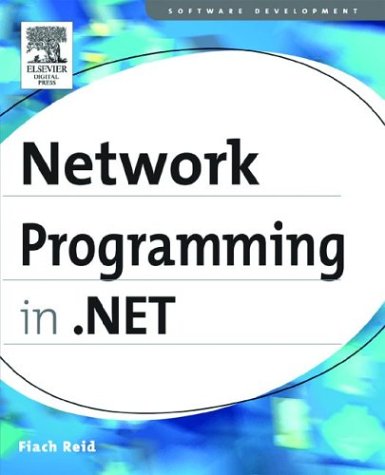
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResourceAttributes.cs
- IndicFontClient.cs
- TagPrefixInfo.cs
- AudioFileOut.cs
- SplineKeyFrames.cs
- PageAsyncTaskManager.cs
- PathData.cs
- CrossSiteScriptingValidation.cs
- ConfigurationConverterBase.cs
- ModuleBuilderData.cs
- StylusLogic.cs
- LoginNameDesigner.cs
- DoubleLinkListEnumerator.cs
- Expander.cs
- MethodBuilderInstantiation.cs
- CodeRemoveEventStatement.cs
- LookupNode.cs
- FileInfo.cs
- TypeContext.cs
- CoTaskMemHandle.cs
- ValidationErrorCollection.cs
- FormViewUpdatedEventArgs.cs
- Int64KeyFrameCollection.cs
- MaskInputRejectedEventArgs.cs
- ISessionStateStore.cs
- ListBox.cs
- GraphicsContext.cs
- XamlSerializationHelper.cs
- ClrProviderManifest.cs
- XmlDocument.cs
- SHA1.cs
- ErrorWebPart.cs
- XPathMultyIterator.cs
- BamlMapTable.cs
- SettingsBase.cs
- SmiMetaDataProperty.cs
- Opcode.cs
- GradientSpreadMethodValidation.cs
- SoapReflectionImporter.cs
- SetterBaseCollection.cs
- ParentControlDesigner.cs
- BamlLocalizer.cs
- TextParagraphProperties.cs
- ByteKeyFrameCollection.cs
- DragAssistanceManager.cs
- UnmanagedMarshal.cs
- FocusWithinProperty.cs
- Documentation.cs
- mda.cs
- LinkLabel.cs
- GetRecipientListRequest.cs
- CipherData.cs
- Canvas.cs
- input.cs
- XmlHierarchicalDataSourceView.cs
- DataServiceQueryOfT.cs
- DocumentPageViewAutomationPeer.cs
- GregorianCalendarHelper.cs
- IODescriptionAttribute.cs
- DataBindingCollection.cs
- PriorityRange.cs
- TextServicesCompartmentContext.cs
- WorkflowQueueInfo.cs
- SecurityTokenResolver.cs
- CalendarDateRangeChangingEventArgs.cs
- KeySplineConverter.cs
- XamlReader.cs
- DispatcherObject.cs
- RegistrationServices.cs
- EntityDataSourceQueryBuilder.cs
- ToolStripGripRenderEventArgs.cs
- QualificationDataAttribute.cs
- Polygon.cs
- WebPartMenu.cs
- CodeParameterDeclarationExpression.cs
- Convert.cs
- StatusBar.cs
- ViewSimplifier.cs
- SchemaTableOptionalColumn.cs
- COAUTHINFO.cs
- sqlser.cs
- Message.cs
- ParseHttpDate.cs
- PageDeviceFont.cs
- BinaryObjectWriter.cs
- RoleGroupCollection.cs
- DbUpdateCommandTree.cs
- SecurityHelper.cs
- TTSEvent.cs
- StrokeCollectionDefaultValueFactory.cs
- ClassValidator.cs
- ListSortDescription.cs
- NullableConverter.cs
- CodeTypeConstructor.cs
- DbProviderFactoriesConfigurationHandler.cs
- PathData.cs
- SimpleRecyclingCache.cs
- XPathNavigatorKeyComparer.cs
- HtmlCommandAdapter.cs
- CrossAppDomainChannel.cs