Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Metadata / MetadataArtifactLoaderComposite.cs / 2 / MetadataArtifactLoaderComposite.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Text; using System.Xml; using System.Data.Mapping; using System.IO; using System.Security; using System.Security.Permissions; using System.Collections.ObjectModel; namespace System.Data.Metadata.Edm { ////// This class represents a super-collection (a collection of collections) /// of artifact resources. Typically, this "meta-collection" would contain /// artifacts represented as individual files, directories (which are in /// turn collections of files), and embedded resources. /// ///This is the root class for access to all loader objects. internal class MetadataArtifactLoaderComposite : MetadataArtifactLoader, IEnumerable{ /// /// The list of loaders aggregated by the composite. /// private readonly ReadOnlyCollection_children; /// /// Constructor - loads all resources into the _children collection /// /// A list of collections to aggregate public MetadataArtifactLoaderComposite(Listchildren) { Debug.Assert(children != null); _children = new List (children).AsReadOnly(); } public override string Path { get { return string.Empty; } } public override void CollectFilePermissionPaths(List paths, DataSpace spaceToGet) { foreach (MetadataArtifactLoader loader in _children) { loader.CollectFilePermissionPaths(paths, spaceToGet); } } public override bool IsComposite { get { return true; } } /// /// Get the list of paths to all artifacts in the original, unexpanded form /// ///A List of strings identifying paths to all resources public override ListGetOriginalPaths() { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetOriginalPaths()); } return list; } /// /// Get paths to artifacts for a specific DataSpace, in the original, unexpanded /// form /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetOriginalPaths(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetOriginalPaths(spaceToGet)); } return list; } /// /// Get paths to artifacts for a specific DataSpace. /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetPaths(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetPaths(spaceToGet)); } return list; } /// /// Get paths to all artifacts /// ///A List of strings identifying paths to all resources public override ListGetPaths() { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.GetPaths()); } return list; } /// /// Aggregates all resource streams from the _children collection /// ///A List of XmlReader objects; cannot be null public override ListGetReaders(Dictionary sourceDictionary) { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.GetReaders(sourceDictionary)); } return list; } /// /// Get XmlReaders for a specific DataSpace. /// /// The DataSpace corresponding to the requested artifacts ///A List of XmlReader objects public override ListCreateReaders(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.CreateReaders(spaceToGet)); } return list; } #region IEnumerable Members public IEnumerator GetEnumerator() { return this._children.GetEnumerator(); } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return this._children.GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Text; using System.Xml; using System.Data.Mapping; using System.IO; using System.Security; using System.Security.Permissions; using System.Collections.ObjectModel; namespace System.Data.Metadata.Edm { ////// This class represents a super-collection (a collection of collections) /// of artifact resources. Typically, this "meta-collection" would contain /// artifacts represented as individual files, directories (which are in /// turn collections of files), and embedded resources. /// ///This is the root class for access to all loader objects. internal class MetadataArtifactLoaderComposite : MetadataArtifactLoader, IEnumerable{ /// /// The list of loaders aggregated by the composite. /// private readonly ReadOnlyCollection_children; /// /// Constructor - loads all resources into the _children collection /// /// A list of collections to aggregate public MetadataArtifactLoaderComposite(Listchildren) { Debug.Assert(children != null); _children = new List (children).AsReadOnly(); } public override string Path { get { return string.Empty; } } public override void CollectFilePermissionPaths(List paths, DataSpace spaceToGet) { foreach (MetadataArtifactLoader loader in _children) { loader.CollectFilePermissionPaths(paths, spaceToGet); } } public override bool IsComposite { get { return true; } } /// /// Get the list of paths to all artifacts in the original, unexpanded form /// ///A List of strings identifying paths to all resources public override ListGetOriginalPaths() { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetOriginalPaths()); } return list; } /// /// Get paths to artifacts for a specific DataSpace, in the original, unexpanded /// form /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetOriginalPaths(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetOriginalPaths(spaceToGet)); } return list; } /// /// Get paths to artifacts for a specific DataSpace. /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetPaths(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader loader in _children) { list.AddRange(loader.GetPaths(spaceToGet)); } return list; } /// /// Get paths to all artifacts /// ///A List of strings identifying paths to all resources public override ListGetPaths() { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.GetPaths()); } return list; } /// /// Aggregates all resource streams from the _children collection /// ///A List of XmlReader objects; cannot be null public override ListGetReaders(Dictionary sourceDictionary) { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.GetReaders(sourceDictionary)); } return list; } /// /// Get XmlReaders for a specific DataSpace. /// /// The DataSpace corresponding to the requested artifacts ///A List of XmlReader objects public override ListCreateReaders(DataSpace spaceToGet) { List list = new List (); foreach (MetadataArtifactLoader resource in _children) { list.AddRange(resource.CreateReaders(spaceToGet)); } return list; } #region IEnumerable Members public IEnumerator GetEnumerator() { return this._children.GetEnumerator(); } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return this._children.GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
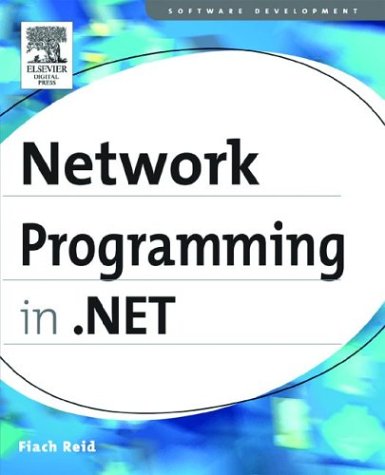
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SetterBase.cs
- InitializationEventAttribute.cs
- DragEventArgs.cs
- StylusPlugin.cs
- HotCommands.cs
- namescope.cs
- RoleService.cs
- AddressingProperty.cs
- LogicalExpr.cs
- ListSortDescriptionCollection.cs
- IInstanceTable.cs
- KeyEvent.cs
- RequestCachingSection.cs
- TimerEventSubscription.cs
- RenderCapability.cs
- ProjectionPlanCompiler.cs
- MSAAWinEventWrap.cs
- ObjectAnimationUsingKeyFrames.cs
- ArithmeticException.cs
- BulletedList.cs
- _UncName.cs
- TransformGroup.cs
- ItemMap.cs
- VectorKeyFrameCollection.cs
- ToolStripGrip.cs
- ControlUtil.cs
- DbTransaction.cs
- NumberSubstitution.cs
- HeaderedItemsControl.cs
- PropertyEmitterBase.cs
- AmbiguousMatchException.cs
- ImportOptions.cs
- RequestSecurityTokenForGetBrowserToken.cs
- EventEntry.cs
- WindowsListViewGroup.cs
- PagesSection.cs
- ObjectManager.cs
- HtmlInputRadioButton.cs
- CodeExporter.cs
- COM2Properties.cs
- _FtpControlStream.cs
- FileInfo.cs
- CharAnimationBase.cs
- FileDialog_Vista.cs
- ToolStripCustomTypeDescriptor.cs
- HtmlInputButton.cs
- XmlnsPrefixAttribute.cs
- ToolboxControl.cs
- EntityDataSourceChangedEventArgs.cs
- HttpPostLocalhostServerProtocol.cs
- XmlChildNodes.cs
- SystemWebExtensionsSectionGroup.cs
- PasswordTextNavigator.cs
- PageThemeBuildProvider.cs
- Pts.cs
- BooleanKeyFrameCollection.cs
- AsymmetricKeyExchangeFormatter.cs
- EntityConnectionStringBuilder.cs
- CodeTypeDeclarationCollection.cs
- NativeMethods.cs
- TransformationRules.cs
- OptimalTextSource.cs
- StrokeCollection.cs
- Animatable.cs
- BoundPropertyEntry.cs
- ScrollViewer.cs
- TextReturnReader.cs
- ConfigXmlSignificantWhitespace.cs
- GridViewItemAutomationPeer.cs
- InternalConfirm.cs
- PropertyIDSet.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- EmptyEnumerator.cs
- TraceInternal.cs
- X509SecurityTokenAuthenticator.cs
- Size3DValueSerializer.cs
- TreeViewImageIndexConverter.cs
- TdsParameterSetter.cs
- SelectionPattern.cs
- AnyAllSearchOperator.cs
- Hashtable.cs
- TextAutomationPeer.cs
- BinaryExpression.cs
- RoutedPropertyChangedEventArgs.cs
- ObjectPropertyMapping.cs
- WebPartAuthorizationEventArgs.cs
- Window.cs
- wgx_render.cs
- ZoneButton.cs
- FindRequestContext.cs
- Token.cs
- BackStopAuthenticationModule.cs
- ModelItemDictionary.cs
- FileClassifier.cs
- Membership.cs
- CreateRefExpr.cs
- SqlUtil.cs
- ConfigurationException.cs
- XmlSerializationReader.cs
- ProfessionalColors.cs