Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Net / System / Net / Configuration / HttpWebRequestElement.cs / 1 / HttpWebRequestElement.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Configuration { using System; using System.Configuration; using System.Reflection; using System.Security.Permissions; public sealed class HttpWebRequestElement : ConfigurationElement { public HttpWebRequestElement() { this.properties.Add(this.maximumResponseHeadersLength); this.properties.Add(this.maximumErrorResponseLength); this.properties.Add(this.maximumUnauthorizedUploadLength); this.properties.Add(this.useUnsafeHeaderParsing); } protected override void PostDeserialize() { // Perf optimization. If the configuration is coming from machine.config // It is safe and we don't need to check for permissions. if (EvaluationContext.IsMachineLevel) return; PropertyInformation[] protectedProperties = { ElementInformation.Properties[ConfigurationStrings.MaximumResponseHeadersLength], ElementInformation.Properties[ConfigurationStrings.MaximumErrorResponseLength] }; foreach (PropertyInformation property in protectedProperties) if (property.ValueOrigin == PropertyValueOrigin.SetHere) { try { ExceptionHelper.WebPermissionUnrestricted.Demand(); } catch (Exception exception) { throw new ConfigurationErrorsException( SR.GetString(SR.net_config_property_permission, property.Name), exception); } } } protected override ConfigurationPropertyCollection Properties { get { return this.properties; } } [ConfigurationProperty(ConfigurationStrings.maximumUnauthorizedUploadLength, DefaultValue=(int)(-1))] public int MaximumUnauthorizedUploadLength { get { return (int)this[this.maximumUnauthorizedUploadLength]; } set { this[this.maximumUnauthorizedUploadLength] = value; } } [ConfigurationProperty(ConfigurationStrings.MaximumErrorResponseLength, DefaultValue=(int)(64))] public int MaximumErrorResponseLength { get { return (int)this[this.maximumErrorResponseLength]; } set { this[this.maximumErrorResponseLength] = value; } } [ConfigurationProperty(ConfigurationStrings.MaximumResponseHeadersLength, DefaultValue= 64)] public int MaximumResponseHeadersLength { get { return (int)this[this.maximumResponseHeadersLength]; } set { this[this.maximumResponseHeadersLength] = value; } } [ConfigurationProperty(ConfigurationStrings.UseUnsafeHeaderParsing, DefaultValue= false)] public bool UseUnsafeHeaderParsing { get { return (bool) this[this.useUnsafeHeaderParsing]; } set { this[this.useUnsafeHeaderParsing] = value; } } ConfigurationPropertyCollection properties = new ConfigurationPropertyCollection(); readonly ConfigurationProperty maximumResponseHeadersLength = new ConfigurationProperty(ConfigurationStrings.MaximumResponseHeadersLength, typeof(int), 64, ConfigurationPropertyOptions.None); readonly ConfigurationProperty maximumErrorResponseLength = new ConfigurationProperty(ConfigurationStrings.MaximumErrorResponseLength, typeof(int), 64, ConfigurationPropertyOptions.None); readonly ConfigurationProperty maximumUnauthorizedUploadLength = new ConfigurationProperty(ConfigurationStrings.maximumUnauthorizedUploadLength, typeof(int), -1, ConfigurationPropertyOptions.None); readonly ConfigurationProperty useUnsafeHeaderParsing = new ConfigurationProperty(ConfigurationStrings.UseUnsafeHeaderParsing, typeof(bool), false, ConfigurationPropertyOptions.None); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Configuration { using System; using System.Configuration; using System.Reflection; using System.Security.Permissions; public sealed class HttpWebRequestElement : ConfigurationElement { public HttpWebRequestElement() { this.properties.Add(this.maximumResponseHeadersLength); this.properties.Add(this.maximumErrorResponseLength); this.properties.Add(this.maximumUnauthorizedUploadLength); this.properties.Add(this.useUnsafeHeaderParsing); } protected override void PostDeserialize() { // Perf optimization. If the configuration is coming from machine.config // It is safe and we don't need to check for permissions. if (EvaluationContext.IsMachineLevel) return; PropertyInformation[] protectedProperties = { ElementInformation.Properties[ConfigurationStrings.MaximumResponseHeadersLength], ElementInformation.Properties[ConfigurationStrings.MaximumErrorResponseLength] }; foreach (PropertyInformation property in protectedProperties) if (property.ValueOrigin == PropertyValueOrigin.SetHere) { try { ExceptionHelper.WebPermissionUnrestricted.Demand(); } catch (Exception exception) { throw new ConfigurationErrorsException( SR.GetString(SR.net_config_property_permission, property.Name), exception); } } } protected override ConfigurationPropertyCollection Properties { get { return this.properties; } } [ConfigurationProperty(ConfigurationStrings.maximumUnauthorizedUploadLength, DefaultValue=(int)(-1))] public int MaximumUnauthorizedUploadLength { get { return (int)this[this.maximumUnauthorizedUploadLength]; } set { this[this.maximumUnauthorizedUploadLength] = value; } } [ConfigurationProperty(ConfigurationStrings.MaximumErrorResponseLength, DefaultValue=(int)(64))] public int MaximumErrorResponseLength { get { return (int)this[this.maximumErrorResponseLength]; } set { this[this.maximumErrorResponseLength] = value; } } [ConfigurationProperty(ConfigurationStrings.MaximumResponseHeadersLength, DefaultValue= 64)] public int MaximumResponseHeadersLength { get { return (int)this[this.maximumResponseHeadersLength]; } set { this[this.maximumResponseHeadersLength] = value; } } [ConfigurationProperty(ConfigurationStrings.UseUnsafeHeaderParsing, DefaultValue= false)] public bool UseUnsafeHeaderParsing { get { return (bool) this[this.useUnsafeHeaderParsing]; } set { this[this.useUnsafeHeaderParsing] = value; } } ConfigurationPropertyCollection properties = new ConfigurationPropertyCollection(); readonly ConfigurationProperty maximumResponseHeadersLength = new ConfigurationProperty(ConfigurationStrings.MaximumResponseHeadersLength, typeof(int), 64, ConfigurationPropertyOptions.None); readonly ConfigurationProperty maximumErrorResponseLength = new ConfigurationProperty(ConfigurationStrings.MaximumErrorResponseLength, typeof(int), 64, ConfigurationPropertyOptions.None); readonly ConfigurationProperty maximumUnauthorizedUploadLength = new ConfigurationProperty(ConfigurationStrings.maximumUnauthorizedUploadLength, typeof(int), -1, ConfigurationPropertyOptions.None); readonly ConfigurationProperty useUnsafeHeaderParsing = new ConfigurationProperty(ConfigurationStrings.UseUnsafeHeaderParsing, typeof(bool), false, ConfigurationPropertyOptions.None); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
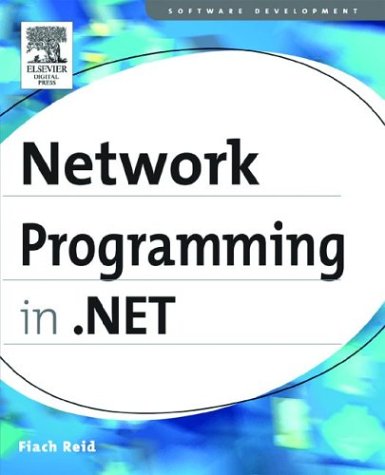
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataBindingCollection.cs
- TTSVoice.cs
- DPTypeDescriptorContext.cs
- CompilerWrapper.cs
- SynchronizationHandlesCodeDomSerializer.cs
- QueryOperationResponseOfT.cs
- OleDbParameter.cs
- PrintPageEvent.cs
- GlobalizationSection.cs
- XsdDataContractExporter.cs
- PartitionerStatic.cs
- Duration.cs
- UnsafeNativeMethods.cs
- Quaternion.cs
- TrackingSection.cs
- CompiledScopeCriteria.cs
- DbProviderFactories.cs
- Error.cs
- ObjectAnimationBase.cs
- SerialReceived.cs
- BrowserCapabilitiesCodeGenerator.cs
- StreamSecurityUpgradeInitiatorBase.cs
- ComponentCommands.cs
- ErrorFormatter.cs
- ResourceDescriptionAttribute.cs
- NullableDecimalSumAggregationOperator.cs
- Wizard.cs
- ControlIdConverter.cs
- IsolatedStorageFile.cs
- BitmapPalette.cs
- DesignerCategoryAttribute.cs
- CorruptingExceptionCommon.cs
- HandlerFactoryCache.cs
- UnsafeNativeMethods.cs
- InternalBufferOverflowException.cs
- XmlNullResolver.cs
- LongValidator.cs
- ASCIIEncoding.cs
- BufferModesCollection.cs
- CacheHelper.cs
- HeaderCollection.cs
- BaseComponentEditor.cs
- CannotUnloadAppDomainException.cs
- ColorMap.cs
- ResourceType.cs
- SearchForVirtualItemEventArgs.cs
- StyleXamlParser.cs
- ButtonPopupAdapter.cs
- StructuredTypeEmitter.cs
- EncodingInfo.cs
- Function.cs
- TrackingAnnotationCollection.cs
- OverrideMode.cs
- MenuItemBindingCollection.cs
- SortAction.cs
- UpDownBase.cs
- RowToParametersTransformer.cs
- UnsafeNativeMethods.cs
- _TransmitFileOverlappedAsyncResult.cs
- DataGridViewRowHeaderCell.cs
- SqlExpressionNullability.cs
- ErasingStroke.cs
- StreamWriter.cs
- OleDbRowUpdatingEvent.cs
- PopOutPanel.cs
- GridViewPageEventArgs.cs
- XmlSchemaSimpleTypeUnion.cs
- DocumentApplicationDocumentViewer.cs
- SerializationObjectManager.cs
- DispatcherExceptionFilterEventArgs.cs
- EntityViewGenerationAttribute.cs
- EncodingInfo.cs
- Convert.cs
- ConstructorArgumentAttribute.cs
- BrowserPolicyValidator.cs
- SafeNativeMethodsCLR.cs
- ConnectionsZone.cs
- InstanceKeyCollisionException.cs
- HttpWrapper.cs
- xmlglyphRunInfo.cs
- OdbcConnectionStringbuilder.cs
- RegistryPermission.cs
- entitydatasourceentitysetnameconverter.cs
- BaseDataBoundControl.cs
- ClientRuntimeConfig.cs
- InputScopeNameConverter.cs
- ComponentDispatcherThread.cs
- MembershipPasswordException.cs
- XmlQueryCardinality.cs
- FileCodeGroup.cs
- WizardSideBarListControlItem.cs
- TextRunProperties.cs
- InputLangChangeRequestEvent.cs
- _Events.cs
- Tokenizer.cs
- XComponentModel.cs
- OutArgument.cs
- Base64WriteStateInfo.cs
- PerformanceCounterPermission.cs
- DataFieldConverter.cs