Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / Interop / ComponentDispatcherThread.cs / 1305600 / ComponentDispatcherThread.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using MS.Win32; using System.Globalization; using System.Security; using System.Security.Permissions; using MS.Internal.WindowsBase; namespace System.Windows.Interop { ////// This is a class used to implement per-thread instance of the ComponentDispatcher. /// internal class ComponentDispatcherThread { ////// Returns true if one or more components has gone modal. /// Although once one component is modal a 2nd shouldn't. /// public bool IsThreadModal { get { return (_modalCount > 0); } } ////// Returns "current" message. More exactly the last MSG Raised. /// ////// Critical: This is blocked off as defense in depth to avoid leaking message information /// public MSG CurrentKeyboardMessage { [SecurityCritical] get { return _currentKeyboardMSG; } [SecurityCritical] set { _currentKeyboardMSG = value; } } ////// A component calls this to go modal. Current thread wide only. /// ////// Critical: This is blocked off as defense in depth /// [SecurityCritical] public void PushModal() { _modalCount += 1; if(1 == _modalCount) { if(null != _enterThreadModal) _enterThreadModal(null, EventArgs.Empty); } } ////// A component calls this to end being modal. /// ////// Critical: This is blocked off as defense in depth to avoid tampering with input /// [SecurityCritical] public void PopModal() { _modalCount -= 1; if(0 == _modalCount) { if(null != _leaveThreadModal) _leaveThreadModal(null, EventArgs.Empty); } if(_modalCount < 0) _modalCount = 0; // Throwing is also good } ////// The message loop pumper calls this when it is time to do idle processing. /// ////// Critical: This is blocked off as defense in depth /// [SecurityCritical] public void RaiseIdle() { if(null != _threadIdle) _threadIdle(null, EventArgs.Empty); } ////// The message loop pumper calls this for every keyboard message. /// ////// Critical: This is blocked off as defense in depth to prevent message leakage /// [SecurityCritical] public bool RaiseThreadMessage(ref MSG msg) { bool handled = false; if (null != _threadFilterMessage) _threadFilterMessage(ref msg, ref handled); if (handled) return handled; if (null != _threadPreprocessMessage) _threadPreprocessMessage(ref msg, ref handled); return handled; } ////// Components register delegates with this event to handle /// thread idle processing. /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event EventHandler ThreadIdle { [SecurityCritical] add { _threadIdle += value; } [SecurityCritical] remove { _threadIdle -= value; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [method:SecurityCritical] private event EventHandler _threadIdle; ////// Components register delegates with this event to handle /// Keyboard Messages (first chance processing). /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event ThreadMessageEventHandler ThreadFilterMessage { [SecurityCritical] add { _threadFilterMessage += value; } [SecurityCritical] remove { _threadFilterMessage -= value; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [method:SecurityCritical] private event ThreadMessageEventHandler _threadFilterMessage; ////// Components register delegates with this event to handle /// Keyboard Messages (second chance processing). /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event ThreadMessageEventHandler ThreadPreprocessMessage { [SecurityCritical] add { _threadPreprocessMessage += value; } [SecurityCritical] remove { _threadPreprocessMessage -= value; } } ////// Adds the specified handler to the front of the invocation list /// of the PreprocessMessage event. /// /// /// Critical: This is blocked off as defense in depth and is used /// to transmit input related information /// [SecurityCritical] public void AddThreadPreprocessMessageHandlerFirst(ThreadMessageEventHandler handler) { _threadPreprocessMessage = (ThreadMessageEventHandler)Delegate.Combine(handler, _threadPreprocessMessage); } ////// Removes the first occurance of the specified handler from the /// invocation list of the PreprocessMessage event. /// /// /// Critical: This is blocked off as defense in depth and is used /// to transmit input related information /// [SecurityCritical] public void RemoveThreadPreprocessMessageHandlerFirst(ThreadMessageEventHandler handler) { if (_threadPreprocessMessage != null) { ThreadMessageEventHandler newHandler = null; foreach (ThreadMessageEventHandler testHandler in _threadPreprocessMessage.GetInvocationList()) { if (testHandler == handler) { // This is the handler to remove. We should not check // for any more occurances. handler = null; } else { newHandler += testHandler; } } _threadPreprocessMessage = newHandler; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [method:SecurityCritical] private event ThreadMessageEventHandler _threadPreprocessMessage; ////// Components register delegates with this event to handle /// a component on this thread has "gone modal", when previously none were. /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event EventHandler EnterThreadModal { [SecurityCritical] add { _enterThreadModal += value; } [SecurityCritical] remove { _enterThreadModal -= value; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [method:SecurityCritical] private event EventHandler _enterThreadModal; ////// Components register delegates with this event to handle /// all components on this thread are done being modal. /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event EventHandler LeaveThreadModal { [SecurityCritical] add { _leaveThreadModal += value; } [SecurityCritical] remove { _leaveThreadModal -= value; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [method:SecurityCritical] private event EventHandler _leaveThreadModal; private int _modalCount; ////// Critical: This holds the last message that was recieved /// [SecurityCritical] private MSG _currentKeyboardMSG; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using MS.Win32; using System.Globalization; using System.Security; using System.Security.Permissions; using MS.Internal.WindowsBase; namespace System.Windows.Interop { ////// This is a class used to implement per-thread instance of the ComponentDispatcher. /// internal class ComponentDispatcherThread { ////// Returns true if one or more components has gone modal. /// Although once one component is modal a 2nd shouldn't. /// public bool IsThreadModal { get { return (_modalCount > 0); } } ////// Returns "current" message. More exactly the last MSG Raised. /// ////// Critical: This is blocked off as defense in depth to avoid leaking message information /// public MSG CurrentKeyboardMessage { [SecurityCritical] get { return _currentKeyboardMSG; } [SecurityCritical] set { _currentKeyboardMSG = value; } } ////// A component calls this to go modal. Current thread wide only. /// ////// Critical: This is blocked off as defense in depth /// [SecurityCritical] public void PushModal() { _modalCount += 1; if(1 == _modalCount) { if(null != _enterThreadModal) _enterThreadModal(null, EventArgs.Empty); } } ////// A component calls this to end being modal. /// ////// Critical: This is blocked off as defense in depth to avoid tampering with input /// [SecurityCritical] public void PopModal() { _modalCount -= 1; if(0 == _modalCount) { if(null != _leaveThreadModal) _leaveThreadModal(null, EventArgs.Empty); } if(_modalCount < 0) _modalCount = 0; // Throwing is also good } ////// The message loop pumper calls this when it is time to do idle processing. /// ////// Critical: This is blocked off as defense in depth /// [SecurityCritical] public void RaiseIdle() { if(null != _threadIdle) _threadIdle(null, EventArgs.Empty); } ////// The message loop pumper calls this for every keyboard message. /// ////// Critical: This is blocked off as defense in depth to prevent message leakage /// [SecurityCritical] public bool RaiseThreadMessage(ref MSG msg) { bool handled = false; if (null != _threadFilterMessage) _threadFilterMessage(ref msg, ref handled); if (handled) return handled; if (null != _threadPreprocessMessage) _threadPreprocessMessage(ref msg, ref handled); return handled; } ////// Components register delegates with this event to handle /// thread idle processing. /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event EventHandler ThreadIdle { [SecurityCritical] add { _threadIdle += value; } [SecurityCritical] remove { _threadIdle -= value; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [method:SecurityCritical] private event EventHandler _threadIdle; ////// Components register delegates with this event to handle /// Keyboard Messages (first chance processing). /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event ThreadMessageEventHandler ThreadFilterMessage { [SecurityCritical] add { _threadFilterMessage += value; } [SecurityCritical] remove { _threadFilterMessage -= value; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [method:SecurityCritical] private event ThreadMessageEventHandler _threadFilterMessage; ////// Components register delegates with this event to handle /// Keyboard Messages (second chance processing). /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event ThreadMessageEventHandler ThreadPreprocessMessage { [SecurityCritical] add { _threadPreprocessMessage += value; } [SecurityCritical] remove { _threadPreprocessMessage -= value; } } ////// Adds the specified handler to the front of the invocation list /// of the PreprocessMessage event. /// /// /// Critical: This is blocked off as defense in depth and is used /// to transmit input related information /// [SecurityCritical] public void AddThreadPreprocessMessageHandlerFirst(ThreadMessageEventHandler handler) { _threadPreprocessMessage = (ThreadMessageEventHandler)Delegate.Combine(handler, _threadPreprocessMessage); } ////// Removes the first occurance of the specified handler from the /// invocation list of the PreprocessMessage event. /// /// /// Critical: This is blocked off as defense in depth and is used /// to transmit input related information /// [SecurityCritical] public void RemoveThreadPreprocessMessageHandlerFirst(ThreadMessageEventHandler handler) { if (_threadPreprocessMessage != null) { ThreadMessageEventHandler newHandler = null; foreach (ThreadMessageEventHandler testHandler in _threadPreprocessMessage.GetInvocationList()) { if (testHandler == handler) { // This is the handler to remove. We should not check // for any more occurances. handler = null; } else { newHandler += testHandler; } } _threadPreprocessMessage = newHandler; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [method:SecurityCritical] private event ThreadMessageEventHandler _threadPreprocessMessage; ////// Components register delegates with this event to handle /// a component on this thread has "gone modal", when previously none were. /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event EventHandler EnterThreadModal { [SecurityCritical] add { _enterThreadModal += value; } [SecurityCritical] remove { _enterThreadModal -= value; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [method:SecurityCritical] private event EventHandler _enterThreadModal; ////// Components register delegates with this event to handle /// all components on this thread are done being modal. /// ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// public event EventHandler LeaveThreadModal { [SecurityCritical] add { _leaveThreadModal += value; } [SecurityCritical] remove { _leaveThreadModal -= value; } } ////// Critical: This is blocked off as defense in depth and is used to transmit input related information /// [method:SecurityCritical] private event EventHandler _leaveThreadModal; private int _modalCount; ////// Critical: This holds the last message that was recieved /// [SecurityCritical] private MSG _currentKeyboardMSG; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
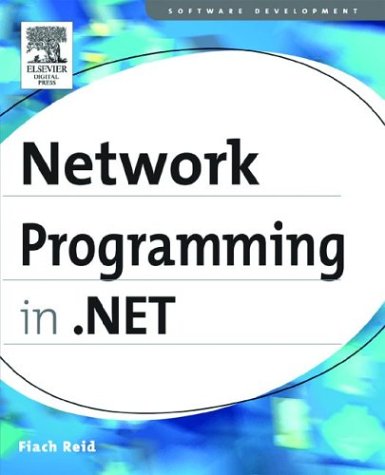
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlFacetAttribute.cs
- TdsParser.cs
- DetailsViewPagerRow.cs
- Int64KeyFrameCollection.cs
- BuildProviderCollection.cs
- RegexWriter.cs
- PerformanceCounterNameAttribute.cs
- Tokenizer.cs
- Bitmap.cs
- ObjectList.cs
- SyntaxCheck.cs
- SchemaNotation.cs
- NativeObjectSecurity.cs
- DataTableReaderListener.cs
- COM2Properties.cs
- oledbmetadatacollectionnames.cs
- SoapIncludeAttribute.cs
- RegisterInfo.cs
- DispatcherExceptionEventArgs.cs
- Baml6Assembly.cs
- TransformerInfoCollection.cs
- ArrangedElement.cs
- UnsettableComboBox.cs
- RotateTransform3D.cs
- ObjectPersistData.cs
- odbcmetadatacolumnnames.cs
- SchemaCreator.cs
- SQlBooleanStorage.cs
- GridProviderWrapper.cs
- HttpProfileGroupBase.cs
- SubMenuStyleCollection.cs
- UnsafeNativeMethods.cs
- WorkflowInstance.cs
- SafeUserTokenHandle.cs
- LayoutUtils.cs
- DecoderReplacementFallback.cs
- SQLInt64Storage.cs
- LongValidatorAttribute.cs
- ToolStripItemImageRenderEventArgs.cs
- LocatorBase.cs
- ToolStripScrollButton.cs
- PointConverter.cs
- XmlFormatExtensionPointAttribute.cs
- ToolStripHighContrastRenderer.cs
- CodeSnippetTypeMember.cs
- InvariantComparer.cs
- PowerModeChangedEventArgs.cs
- ISAPIWorkerRequest.cs
- SessionPageStatePersister.cs
- DBSqlParserColumnCollection.cs
- StatusBarItem.cs
- SiteMapDataSource.cs
- Storyboard.cs
- VisualBasicSettingsConverter.cs
- SafeNativeMemoryHandle.cs
- ErrorEventArgs.cs
- SubpageParaClient.cs
- ChannelToken.cs
- RoleServiceManager.cs
- IisTraceListener.cs
- DataPagerFieldCommandEventArgs.cs
- WebDisplayNameAttribute.cs
- FileRegion.cs
- InputScopeManager.cs
- ConnectionStringSettingsCollection.cs
- ImageSourceConverter.cs
- EventLevel.cs
- Image.cs
- TableLayoutSettings.cs
- NetMsmqBinding.cs
- XmlSequenceWriter.cs
- OperatingSystem.cs
- SerializableTypeCodeDomSerializer.cs
- SchemaImporterExtensionElementCollection.cs
- SignalGate.cs
- RoutingSection.cs
- LocatorPart.cs
- DataGridViewCellValidatingEventArgs.cs
- WebPermission.cs
- Transform3DGroup.cs
- RoleGroup.cs
- AutomationAttributeInfo.cs
- ListControl.cs
- SatelliteContractVersionAttribute.cs
- dataobject.cs
- JsonObjectDataContract.cs
- SafeFindHandle.cs
- RegistrationServices.cs
- HotSpotCollection.cs
- CompleteWizardStep.cs
- FontStretches.cs
- Aes.cs
- AudioDeviceOut.cs
- Timer.cs
- OperationFormatUse.cs
- DataPagerFieldItem.cs
- VectorCollectionConverter.cs
- ButtonRenderer.cs
- HitTestParameters.cs
- SystemIcmpV6Statistics.cs