Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.WorkflowServices / System / Workflow / Activities / ChannelToken.cs / 1305376 / ChannelToken.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.Workflow.Activities { using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; using System.Diagnostics.CodeAnalysis; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Dispatcher; using System.Workflow.ComponentModel; using System.Workflow.ComponentModel.Design; using System.Workflow.ComponentModel.Serialization; using System.Xml; [DesignerSerializer(typeof(DependencyObjectCodeDomSerializer), typeof(CodeDomSerializer))] [TypeConverter(typeof(ChannelTokenTypeConverter))] public sealed class ChannelToken : DependencyObject, IPropertyValueProvider { [SuppressMessage("Microsoft.Security", "CA2104:DoNotDeclareReadOnlyMutableReferenceTypes")] internal static readonly DependencyProperty EndpointNameProperty = DependencyProperty.Register("EndpointName", typeof(string), typeof(ChannelToken), new PropertyMetadata(null)); [SuppressMessage("Microsoft.Security", "CA2104:DoNotDeclareReadOnlyMutableReferenceTypes")] internal static readonly DependencyProperty NameProperty = DependencyProperty.Register("Name", typeof(string), typeof(ChannelToken), new PropertyMetadata(null, DependencyPropertyOptions.Metadata, new Attribute[] { new BrowsableAttribute(false) })); [SuppressMessage("Microsoft.Security", "CA2104:DoNotDeclareReadOnlyMutableReferenceTypes")] internal static readonly DependencyProperty OwnerActivityNameProperty = DependencyProperty.Register("OwnerActivityName", typeof(string), typeof(ChannelToken), new PropertyMetadata(null, DependencyPropertyOptions.Metadata, new Attribute[] { new TypeConverterAttribute(typeof(PropertyValueProviderTypeConverter)) })); public ChannelToken() { } internal ChannelToken(string name) { this.Name = name; } [DefaultValue(null)] [SR2Description(SR2DescriptionAttribute.ChannelToken_EndpointName_Description)] public string EndpointName { get { return (string) GetValue(EndpointNameProperty); } set { SetValue(EndpointNameProperty, value); } } [Browsable(false)] [DefaultValue(null)] [SR2Description(SR2DescriptionAttribute.ChannelToken_Name_Description)] public string Name { get { return (string) GetValue(NameProperty); } set { SetValue(NameProperty, value); } } [DefaultValue(null)] [TypeConverter(typeof(PropertyValueProviderTypeConverter))] [SR2Description(SR2DescriptionAttribute.ChannelToken_OwnerActivityName_Description)] public string OwnerActivityName { get { return (string) GetValue(OwnerActivityNameProperty); } set { SetValue(OwnerActivityNameProperty, value); } } ICollection IPropertyValueProvider.GetPropertyValues(ITypeDescriptorContext context) { StringCollection names = new StringCollection(); if (string.Equals(context.PropertyDescriptor.Name, "OwnerActivityName", StringComparison.Ordinal)) { ISelectionService selectionService = context.GetService(typeof(ISelectionService)) as ISelectionService; if (selectionService != null && selectionService.SelectionCount == 1 && selectionService.PrimarySelection is Activity) { // add empty string as an option // names.Add(string.Empty); Activity currentActivity = selectionService.PrimarySelection as Activity; foreach (Activity activity in GetEnclosingCompositeActivities(currentActivity)) { string activityId = activity.QualifiedName; if (!names.Contains(activityId)) { names.Add(activityId); } } } } return names; } internal static LogicalChannel GetLogicalChannel(Activity activity, ChannelToken endpoint, Type contractType) { if (activity == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("activity"); } if (endpoint == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("endpoint"); } if (contractType == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("contractType"); } return GetLogicalChannel(activity, endpoint.Name, endpoint.OwnerActivityName, contractType); } internal static LogicalChannel GetLogicalChannel(Activity activity, string name, string ownerActivityName, Type contractType) { if (activity == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("activity"); } if (string.IsNullOrEmpty(name)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("name", SR2.GetString(SR2.Error_ArgumentValueNullOrEmptyString)); } if (contractType == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("contractType"); } Activity contextActivity = activity.ContextActivity; Activity owner = null; if (contextActivity == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new InvalidOperationException(SR2.GetString(SR2.Error_ContextOwnerActivityMissing))); } if (string.IsNullOrEmpty(ownerActivityName)) { owner = contextActivity.RootActivity; } else { while (contextActivity != null) { owner = contextActivity.GetActivityByName(ownerActivityName, true); if (owner != null) { break; } contextActivity = contextActivity.Parent; if (contextActivity != null) { contextActivity = contextActivity.ContextActivity; } } } if (owner == null && !string.IsNullOrEmpty(ownerActivityName)) { owner = Helpers.ParseActivityForBind(activity, ownerActivityName); } if (owner == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new InvalidOperationException(SR2.GetString(SR2.Error_ContextOwnerActivityMissing))); } LogicalChannel logicalChannel = null; LogicalChannelCollection collection = owner.GetValue(LogicalChannelCollection.LogicalChannelCollectionProperty) as LogicalChannelCollection; if (collection == null) { collection = new LogicalChannelCollection(); owner.SetValue(LogicalChannelCollection.LogicalChannelCollectionProperty, collection); logicalChannel = new LogicalChannel(name, contractType); collection.Add(logicalChannel); } else if (!collection.Contains(name)) { logicalChannel = new LogicalChannel(name, contractType); collection.Add(logicalChannel); } else { logicalChannel = collection[name]; } if (logicalChannel.ContractType != contractType) { logicalChannel = null; } return logicalChannel; } internal static LogicalChannel Register(Activity activity, ChannelToken endpoint, Type contractType) { if (activity == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("activity"); } if (endpoint == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("endpoint"); } if (contractType == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("contractType"); } LogicalChannel logicalChannel = GetLogicalChannel(activity, endpoint, contractType); if (logicalChannel == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new InvalidOperationException(SR2.GetString(SR2.Error_FailedToRegisterChannel, endpoint.Name))); } return logicalChannel; } private static IEnumerable GetEnclosingCompositeActivities(Activity startActivity) { Activity currentActivity = null; StackactivityStack = new Stack (); activityStack.Push(startActivity); while ((currentActivity = activityStack.Pop()) != null) { if (currentActivity.Enabled) { yield return currentActivity; } activityStack.Push(currentActivity.Parent); } yield break; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
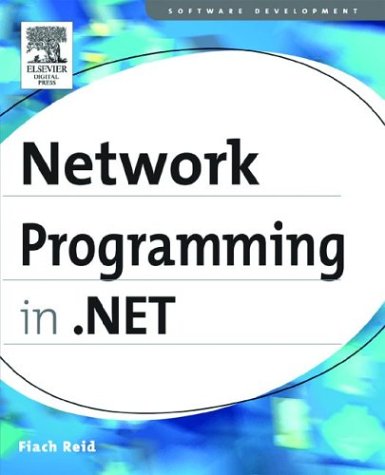
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventSetterHandlerConverter.cs
- InputQueue.cs
- XmlILStorageConverter.cs
- SingletonChannelAcceptor.cs
- SqlDelegatedTransaction.cs
- SamlAssertion.cs
- PropertyDescriptorGridEntry.cs
- ObjectQuery.cs
- FocusTracker.cs
- TdsEnums.cs
- DependencySource.cs
- TimeSpanValidator.cs
- Latin1Encoding.cs
- ActivityXRefPropertyEditor.cs
- FormatterConverter.cs
- HtmlElementErrorEventArgs.cs
- Bold.cs
- WorkflowViewElement.cs
- DataGridViewAutoSizeModeEventArgs.cs
- QilSortKey.cs
- DataFormat.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- Assembly.cs
- TranslateTransform3D.cs
- CodeEventReferenceExpression.cs
- QueueException.cs
- Wildcard.cs
- Internal.cs
- RectKeyFrameCollection.cs
- SuppressMessageAttribute.cs
- Base64Encoder.cs
- XPathNavigatorReader.cs
- ExternalException.cs
- QilBinary.cs
- TableRow.cs
- WmfPlaceableFileHeader.cs
- SupportingTokenChannel.cs
- XPathNavigator.cs
- WebCategoryAttribute.cs
- KeyedCollection.cs
- SecurityState.cs
- BaseProcessor.cs
- XPathQilFactory.cs
- ExpressionBindingCollection.cs
- SevenBitStream.cs
- InteropBitmapSource.cs
- X500Name.cs
- DaylightTime.cs
- DataGridViewColumnHeaderCell.cs
- SystemThemeKey.cs
- XPathArrayIterator.cs
- DbConnectionStringCommon.cs
- DrawingContextDrawingContextWalker.cs
- PlainXmlDeserializer.cs
- EnumerableRowCollection.cs
- LicenseManager.cs
- RootProjectionNode.cs
- PropertyManager.cs
- ColorMap.cs
- GeometryHitTestParameters.cs
- SafeFileMapViewHandle.cs
- WebConfigurationHostFileChange.cs
- ParameterCollection.cs
- SymmetricKey.cs
- SqlSelectClauseBuilder.cs
- UndoUnit.cs
- CodeComment.cs
- BitStream.cs
- Point3D.cs
- UnmanagedBitmapWrapper.cs
- GCHandleCookieTable.cs
- XhtmlBasicValidatorAdapter.cs
- EnterpriseServicesHelper.cs
- DataColumnPropertyDescriptor.cs
- TextTreeTextBlock.cs
- PartManifestEntry.cs
- TextInfo.cs
- AttributeAction.cs
- GPRECT.cs
- invalidudtexception.cs
- IProvider.cs
- CancellationTokenSource.cs
- XmlElementList.cs
- _AutoWebProxyScriptEngine.cs
- MaterializeFromAtom.cs
- ChildDocumentBlock.cs
- WindowsRichEdit.cs
- BoundColumn.cs
- ExtensionWindowHeader.cs
- ReturnValue.cs
- AsnEncodedData.cs
- DocumentOutline.cs
- ReachDocumentReferenceCollectionSerializerAsync.cs
- DataServiceQueryOfT.cs
- _StreamFramer.cs
- BufferedStream2.cs
- ReadingWritingEntityEventArgs.cs
- SchemaTypeEmitter.cs
- ParameterCollection.cs
- ConfigurationManager.cs