Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media3D / Point3D.cs / 1 / Point3D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 3D point implementation. // // See spec at http://avalon/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/02/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Media.Media3D; using System; namespace System.Windows.Media.Media3D { ////// Point3D - 3D point representation. /// Defaults to (0,0,0). /// public partial struct Point3D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor that sets point's initial values. /// /// Value of the X coordinate of the new point. /// Value of the Y coordinate of the new point. /// Value of the Z coordinate of the new point. public Point3D(double x, double y, double z) { _x = x; _y = y; _z = z; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Offset - update point position by adding offsetX to X, offsetY to Y, and offsetZ to Z. /// /// Offset in the X direction. /// Offset in the Y direction. /// Offset in the Z direction. public void Offset(double offsetX, double offsetY, double offsetZ) { _x += offsetX; _y += offsetY; _z += offsetZ; } ////// Point3D + Vector3D addition. /// /// Point being added. /// Vector being added. ///Result of addition. public static Point3D operator +(Point3D point, Vector3D vector) { return new Point3D(point._x + vector._x, point._y + vector._y, point._z + vector._z); } ////// Point3D + Vector3D addition. /// /// Point being added. /// Vector being added. ///Result of addition. public static Point3D Add(Point3D point, Vector3D vector) { return new Point3D(point._x + vector._x, point._y + vector._y, point._z + vector._z); } ////// Point3D - Vector3D subtraction. /// /// Point from which vector is being subtracted. /// Vector being subtracted from the point. ///Result of subtraction. public static Point3D operator -(Point3D point, Vector3D vector) { return new Point3D(point._x - vector._x, point._y - vector._y, point._z - vector._z); } ////// Point3D - Vector3D subtraction. /// /// Point from which vector is being subtracted. /// Vector being subtracted from the point. ///Result of subtraction. public static Point3D Subtract(Point3D point, Vector3D vector) { return new Point3D(point._x - vector._x, point._y - vector._y, point._z - vector._z); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Vector3D operator -(Point3D point1, Point3D point2) { return new Vector3D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Vector3D Subtract(Point3D point1, Point3D point2) { Vector3D v = new Vector3D(); Subtract(ref point1, ref point2, out v); return v; } ////// Faster internal version of Subtract that avoids copies /// /// p1 and p2 to a passed by ref for perf and ARE NOT MODIFIED /// internal static void Subtract(ref Point3D p1, ref Point3D p2, out Vector3D result) { result._x = p1._x - p2._x; result._y = p1._y - p2._y; result._z = p1._z - p2._z; } ////// Point3D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point3D operator *(Point3D point, Matrix3D matrix) { return matrix.Transform(point); } ////// Point3D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point3D Multiply(Point3D point, Matrix3D matrix) { return matrix.Transform(point); } ////// Explicit conversion to Vector3D. /// /// Given point. ///Vector representing the point. public static explicit operator Vector3D(Point3D point) { return new Vector3D(point._x, point._y, point._z); } ////// Explicit conversion to Point4D. /// /// Given point. ///4D point representing the 3D point. public static explicit operator Point4D(Point3D point) { return new Point4D(point._x, point._y, point._z, 1.0); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 3D point implementation. // // See spec at http://avalon/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/02/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Media.Media3D; using System; namespace System.Windows.Media.Media3D { ////// Point3D - 3D point representation. /// Defaults to (0,0,0). /// public partial struct Point3D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor that sets point's initial values. /// /// Value of the X coordinate of the new point. /// Value of the Y coordinate of the new point. /// Value of the Z coordinate of the new point. public Point3D(double x, double y, double z) { _x = x; _y = y; _z = z; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Offset - update point position by adding offsetX to X, offsetY to Y, and offsetZ to Z. /// /// Offset in the X direction. /// Offset in the Y direction. /// Offset in the Z direction. public void Offset(double offsetX, double offsetY, double offsetZ) { _x += offsetX; _y += offsetY; _z += offsetZ; } ////// Point3D + Vector3D addition. /// /// Point being added. /// Vector being added. ///Result of addition. public static Point3D operator +(Point3D point, Vector3D vector) { return new Point3D(point._x + vector._x, point._y + vector._y, point._z + vector._z); } ////// Point3D + Vector3D addition. /// /// Point being added. /// Vector being added. ///Result of addition. public static Point3D Add(Point3D point, Vector3D vector) { return new Point3D(point._x + vector._x, point._y + vector._y, point._z + vector._z); } ////// Point3D - Vector3D subtraction. /// /// Point from which vector is being subtracted. /// Vector being subtracted from the point. ///Result of subtraction. public static Point3D operator -(Point3D point, Vector3D vector) { return new Point3D(point._x - vector._x, point._y - vector._y, point._z - vector._z); } ////// Point3D - Vector3D subtraction. /// /// Point from which vector is being subtracted. /// Vector being subtracted from the point. ///Result of subtraction. public static Point3D Subtract(Point3D point, Vector3D vector) { return new Point3D(point._x - vector._x, point._y - vector._y, point._z - vector._z); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Vector3D operator -(Point3D point1, Point3D point2) { return new Vector3D(point1._x - point2._x, point1._y - point2._y, point1._z - point2._z); } ////// Subtraction. /// /// Point from which we are subtracting the second point. /// Point being subtracted. ///Vector between the two points. public static Vector3D Subtract(Point3D point1, Point3D point2) { Vector3D v = new Vector3D(); Subtract(ref point1, ref point2, out v); return v; } ////// Faster internal version of Subtract that avoids copies /// /// p1 and p2 to a passed by ref for perf and ARE NOT MODIFIED /// internal static void Subtract(ref Point3D p1, ref Point3D p2, out Vector3D result) { result._x = p1._x - p2._x; result._y = p1._y - p2._y; result._z = p1._z - p2._z; } ////// Point3D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point3D operator *(Point3D point, Matrix3D matrix) { return matrix.Transform(point); } ////// Point3D * Matrix3D multiplication. /// /// Point being transformed. /// Transformation matrix applied to the point. ///Result of the transformation matrix applied to the point. public static Point3D Multiply(Point3D point, Matrix3D matrix) { return matrix.Transform(point); } ////// Explicit conversion to Vector3D. /// /// Given point. ///Vector representing the point. public static explicit operator Vector3D(Point3D point) { return new Vector3D(point._x, point._y, point._z); } ////// Explicit conversion to Point4D. /// /// Given point. ///4D point representing the 3D point. public static explicit operator Point4D(Point3D point) { return new Point4D(point._x, point._y, point._z, 1.0); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
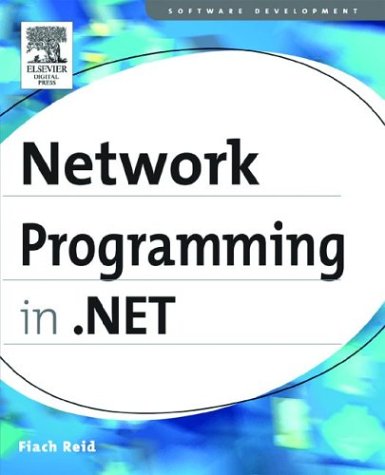
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BrowserDefinition.cs
- CustomPeerResolverService.cs
- SplineQuaternionKeyFrame.cs
- IdentityModelStringsVersion1.cs
- CodeMethodReturnStatement.cs
- AspNetSynchronizationContext.cs
- MetadataUtilsSmi.cs
- MainMenu.cs
- Native.cs
- BrowserInteropHelper.cs
- StreamInfo.cs
- ContextStack.cs
- _SpnDictionary.cs
- ReadOnlyDataSourceView.cs
- XmlSchemaAppInfo.cs
- RelationshipConstraintValidator.cs
- ObjectDataSourceView.cs
- GridSplitterAutomationPeer.cs
- HandledEventArgs.cs
- StreamHelper.cs
- Internal.cs
- DefaultWorkflowLoaderService.cs
- DataServiceProviderWrapper.cs
- ProtectedConfigurationSection.cs
- OrCondition.cs
- PageContentAsyncResult.cs
- PseudoWebRequest.cs
- EventListenerClientSide.cs
- HtmlHistory.cs
- MILUtilities.cs
- AspNetSynchronizationContext.cs
- Positioning.cs
- DBSqlParser.cs
- UIElementParagraph.cs
- EmptyImpersonationContext.cs
- AlternationConverter.cs
- ContentTypeSettingDispatchMessageFormatter.cs
- SecurityMode.cs
- DataControlFieldCell.cs
- NavigationPropertyEmitter.cs
- MessageDecoder.cs
- ISO2022Encoding.cs
- ComponentDispatcherThread.cs
- ExtractedStateEntry.cs
- CommandManager.cs
- DesignerProperties.cs
- LinearGradientBrush.cs
- SByteConverter.cs
- UnaryNode.cs
- ErrorHandler.cs
- SmtpFailedRecipientException.cs
- DataGridViewColumnHeaderCell.cs
- Pkcs7Signer.cs
- BuildManager.cs
- Geometry3D.cs
- EntityDataReader.cs
- TextEditorCopyPaste.cs
- ConnectionsZone.cs
- FilteredDataSetHelper.cs
- TransactionValidationBehavior.cs
- ListSourceHelper.cs
- ProjectionPruner.cs
- ContainerControl.cs
- AssemblyInfo.cs
- AdornedElementPlaceholder.cs
- ListDictionaryInternal.cs
- RolePrincipal.cs
- BlockCollection.cs
- TypeUtil.cs
- DecoratedNameAttribute.cs
- EntityContainerRelationshipSetEnd.cs
- NameTable.cs
- TypeBuilder.cs
- ApplicationTrust.cs
- ParamArrayAttribute.cs
- WhitespaceRuleReader.cs
- SqlTopReducer.cs
- PagesSection.cs
- ToolStripDropDownItem.cs
- ObjectDataSourceView.cs
- SqlIdentifier.cs
- WFItemsToSpacerVisibility.cs
- TableLayoutPanel.cs
- ScriptingProfileServiceSection.cs
- ObjectTag.cs
- ToolboxItemAttribute.cs
- SqlConnectionPoolGroupProviderInfo.cs
- BitStack.cs
- Maps.cs
- Debug.cs
- PagerSettings.cs
- TextAutomationPeer.cs
- SqlMethodAttribute.cs
- ManagementBaseObject.cs
- ResourceExpression.cs
- _BasicClient.cs
- OwnerDrawPropertyBag.cs
- ScrollChrome.cs
- TimeManager.cs
- URI.cs