Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Animation / KeySplineConverter.cs / 1 / KeySplineConverter.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001-2003 // // File: KeySplineConverter.cs //----------------------------------------------------------------------------- // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using MS.Internal; using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Windows.Media.Animation; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// PointConverter - Converter class for converting instances of other types to Point instances /// ///public class KeySplineConverter : TypeConverter { /// /// CanConvertFrom - Returns whether or not this class can convert from a given type /// ///public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptor, Type destinationType) { if (destinationType == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible ///public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } /// /// ConvertFrom /// public override object ConvertFrom( ITypeDescriptorContext context, CultureInfo cultureInfo, object value) { string stringValue = value as string; if (value == null) { throw new NotSupportedException(SR.Get(SRID.Converter_ConvertFromNotSupported)); } TokenizerHelper th = new TokenizerHelper(stringValue, cultureInfo); return new KeySpline( Convert.ToDouble(th.NextTokenRequired(), cultureInfo), Convert.ToDouble(th.NextTokenRequired(), cultureInfo), Convert.ToDouble(th.NextTokenRequired(), cultureInfo), Convert.ToDouble(th.NextTokenRequired(), cultureInfo)); } ////// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs), null is a valid value /// value to convert from /// Type to convert to ///converted value ////// /// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for KeySpline, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext context, CultureInfo cultureInfo, object value, Type destinationType) { KeySpline keySpline = value as KeySpline; if (keySpline != null && destinationType != null) { if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(KeySpline).GetConstructor(new Type[] { typeof(double), typeof(double), typeof(double), typeof(double) }); return new InstanceDescriptor(ci, new object[] { keySpline.ControlPoint1.X, keySpline.ControlPoint1.Y, keySpline.ControlPoint2.X, keySpline.ControlPoint2.Y }); } else if (destinationType == typeof(string)) { #pragma warning disable 56506 // Suppress presharp warning: Parameter 'cultureInfo.TextInfo' to this public method must be validated: A null-dereference can occur here. return String.Format( cultureInfo, "{0}{4}{1}{4}{2}{4}{3}", keySpline.ControlPoint1.X, keySpline.ControlPoint1.Y, keySpline.ControlPoint2.X, keySpline.ControlPoint2.Y, cultureInfo != null ? cultureInfo.TextInfo.ListSeparator : CultureInfo.InvariantCulture.TextInfo.ListSeparator); #pragma warning restore 56506 } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, cultureInfo, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001-2003 // // File: KeySplineConverter.cs //----------------------------------------------------------------------------- // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using MS.Internal; using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Windows.Media.Animation; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// PointConverter - Converter class for converting instances of other types to Point instances /// ///public class KeySplineConverter : TypeConverter { /// /// CanConvertFrom - Returns whether or not this class can convert from a given type /// ///public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptor, Type destinationType) { if (destinationType == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible ///public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } /// /// ConvertFrom /// public override object ConvertFrom( ITypeDescriptorContext context, CultureInfo cultureInfo, object value) { string stringValue = value as string; if (value == null) { throw new NotSupportedException(SR.Get(SRID.Converter_ConvertFromNotSupported)); } TokenizerHelper th = new TokenizerHelper(stringValue, cultureInfo); return new KeySpline( Convert.ToDouble(th.NextTokenRequired(), cultureInfo), Convert.ToDouble(th.NextTokenRequired(), cultureInfo), Convert.ToDouble(th.NextTokenRequired(), cultureInfo), Convert.ToDouble(th.NextTokenRequired(), cultureInfo)); } ////// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs), null is a valid value /// value to convert from /// Type to convert to ///converted value ////// /// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for KeySpline, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext context, CultureInfo cultureInfo, object value, Type destinationType) { KeySpline keySpline = value as KeySpline; if (keySpline != null && destinationType != null) { if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(KeySpline).GetConstructor(new Type[] { typeof(double), typeof(double), typeof(double), typeof(double) }); return new InstanceDescriptor(ci, new object[] { keySpline.ControlPoint1.X, keySpline.ControlPoint1.Y, keySpline.ControlPoint2.X, keySpline.ControlPoint2.Y }); } else if (destinationType == typeof(string)) { #pragma warning disable 56506 // Suppress presharp warning: Parameter 'cultureInfo.TextInfo' to this public method must be validated: A null-dereference can occur here. return String.Format( cultureInfo, "{0}{4}{1}{4}{2}{4}{3}", keySpline.ControlPoint1.X, keySpline.ControlPoint1.Y, keySpline.ControlPoint2.X, keySpline.ControlPoint2.Y, cultureInfo != null ? cultureInfo.TextInfo.ListSeparator : CultureInfo.InvariantCulture.TextInfo.ListSeparator); #pragma warning restore 56506 } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, cultureInfo, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
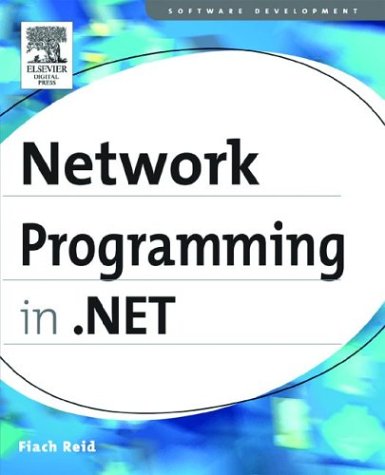
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsComboBox.cs
- Sentence.cs
- TypeInitializationException.cs
- Query.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- ProcessHostMapPath.cs
- NotifyIcon.cs
- ValuePattern.cs
- MultitargetingHelpers.cs
- CatalogPartCollection.cs
- BlurBitmapEffect.cs
- FlowLayoutSettings.cs
- XmlSchemaObjectCollection.cs
- SoapFormatterSinks.cs
- CodeCommentStatement.cs
- PlainXmlDeserializer.cs
- isolationinterop.cs
- HttpPostedFile.cs
- ReflectTypeDescriptionProvider.cs
- GatewayIPAddressInformationCollection.cs
- SpellerStatusTable.cs
- TimeIntervalCollection.cs
- ZipFileInfo.cs
- CfgParser.cs
- HtmlTableRow.cs
- BaseAddressElement.cs
- Int32Rect.cs
- OutputWindow.cs
- WindowCollection.cs
- WindowsListViewGroupHelper.cs
- WindowsListViewItem.cs
- TemplateControl.cs
- DataGridViewRowsRemovedEventArgs.cs
- DataKey.cs
- TimeSpanStorage.cs
- Int64KeyFrameCollection.cs
- MouseGestureConverter.cs
- SemanticTag.cs
- SafeEventLogWriteHandle.cs
- TrustManager.cs
- Solver.cs
- OutOfProcStateClientManager.cs
- GeneralTransform3DTo2DTo3D.cs
- OdbcDataAdapter.cs
- CryptoApi.cs
- VisualStateChangedEventArgs.cs
- IDispatchConstantAttribute.cs
- ItemsControl.cs
- DefaultMemberAttribute.cs
- FileClassifier.cs
- SafeEventLogWriteHandle.cs
- SafeHandle.cs
- DataGridCommandEventArgs.cs
- SingleKeyFrameCollection.cs
- StartUpEventArgs.cs
- ThemeDictionaryExtension.cs
- WebServiceFault.cs
- HostedBindingBehavior.cs
- TableFieldsEditor.cs
- TransformerConfigurationWizardBase.cs
- RegexBoyerMoore.cs
- Win32KeyboardDevice.cs
- TextTreeNode.cs
- TrustManagerMoreInformation.cs
- SuppressIldasmAttribute.cs
- StylusPointPropertyUnit.cs
- DoubleAnimationUsingKeyFrames.cs
- relpropertyhelper.cs
- QilScopedVisitor.cs
- HierarchicalDataSourceControl.cs
- Enum.cs
- ModelItem.cs
- LicenseProviderAttribute.cs
- FontStretchConverter.cs
- FloaterParagraph.cs
- Visual3D.cs
- TimeZoneInfo.cs
- EvidenceTypeDescriptor.cs
- CompositeFontFamily.cs
- ConsoleTraceListener.cs
- EventRecord.cs
- LineServicesRun.cs
- DiagnosticTrace.cs
- ModuleBuilderData.cs
- KeySplineConverter.cs
- TableCellCollection.cs
- InternalPermissions.cs
- DbConnectionPoolGroupProviderInfo.cs
- NotifyParentPropertyAttribute.cs
- SqlDataSourceFilteringEventArgs.cs
- EventLogPermissionEntryCollection.cs
- PersonalizationStateQuery.cs
- MenuRenderer.cs
- MetadataProperty.cs
- CommentEmitter.cs
- ExeContext.cs
- Application.cs
- MultiView.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- EventItfInfo.cs