Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / MS / Internal / IO / Zip / ZipFileInfo.cs / 1 / ZipFileInfo.cs
//------------------------------------------------------------------------------ //------------- *** WARNING *** //------------- This file is part of a legally monitored development project. //------------- Do not check in changes to this project. Do not raid bugs on this //------------- code in the main PS database. Do not contact the owner of this //------------- code directly. Contact the legal team at ‘ZSLegal’ for assistance. //------------- *** WARNING *** //----------------------------------------------------------------------------- //----------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is an internal class that enables interactions with Zip archives // for OPC scenarios // // History: // 11/19/2004: IgorBel: Initial creation. // //----------------------------------------------------------------------------- using System; using System.IO; using System.Diagnostics; namespace MS.Internal.IO.Zip { internal sealed class ZipFileInfo { //------------------------------------------------------ // // Public Members // //----------------------------------------------------- // None //------------------------------------------------------ // // Internal Constructors // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal API Methods (although these methods are marked as // Internal they are part of the internal ZIP IO API surface // //------------------------------------------------------ internal Stream GetStream(FileMode mode, FileAccess access) { CheckDisposed(); return _fileBlock.GetStream(mode, access); } //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- internal string Name { get { CheckDisposed(); return _fileBlock.FileName; } } internal ZipArchive ZipArchive { get { CheckDisposed(); return _zipArchive; } } internal CompressionMethodEnum CompressionMethod { get { CheckDisposed(); return _fileBlock.CompressionMethod; } } internal DateTime LastModFileDateTime { get { CheckDisposed(); return ZipIOBlockManager.FromMsDosDateTime(_fileBlock.LastModFileDateTime); } } #if false internal bool EncryptedFlag { get { CheckDisposed(); return _fileBlock.EncryptedFlag; } } #endif internal DeflateOptionEnum DeflateOption { get { CheckDisposed(); return _fileBlock.DeflateOption; } } #if false internal bool StreamingCreationFlag { get { CheckDisposed(); return _fileBlock.StreamingCreationFlag; } } #endif // This ia Directory flag based on the informtion from the central directory // at the moment we have only provide reliable value for the files authored in MS-DOS // The upper byte of version made by indicating (OS) must be == 0 (MS-DOS) // for the other cases (OSes) we will return false internal bool FolderFlag { get { CheckDisposed(); return _fileBlock.FolderFlag; } } // This ia Directory flag based on the informtion from the central directory // at the moment we have only provide reliable value for the files authored in MS-DOS // The upper byte of version made by indicating (OS) must be == 0 (MS-DOS) // for the other cases (OSes) we will return false internal bool VolumeLabelFlag { get { CheckDisposed(); return _fileBlock.VolumeLabelFlag; } } //----------------------------------------------------- // Internal NON API Constructor (this constructor is marked as internal // and isNOT part of the ZIP IO API surface) // It supposed to be called only by the ZipArchive class //------------------------------------------------------ internal ZipFileInfo(ZipArchive zipArchive, ZipIOLocalFileBlock fileBlock) { Debug.Assert((fileBlock != null) && (zipArchive != null)); _fileBlock = fileBlock; _zipArchive = zipArchive; #if DEBUG // validate that date time is legal DateTime dt = LastModFileDateTime; #endif } //----------------------------------------------------- // Internal NON API property to be used to map FileInfo back to a block that needs to be deleted // (this prperty is marked as internal and isNOT part of the ZIP IO API surface) // It supposed to be called only by the ZipArchive class //------------------------------------------------------ internal ZipIOLocalFileBlock LocalFileBlock { get { return _fileBlock; } } //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- private void CheckDisposed() { _fileBlock.CheckDisposed(); } //------------------------------------------------------ // // Private Properties // //----------------------------------------------------- //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- private ZipIOLocalFileBlock _fileBlock; private ZipArchive _zipArchive; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //------------- *** WARNING *** //------------- This file is part of a legally monitored development project. //------------- Do not check in changes to this project. Do not raid bugs on this //------------- code in the main PS database. Do not contact the owner of this //------------- code directly. Contact the legal team at ‘ZSLegal’ for assistance. //------------- *** WARNING *** //----------------------------------------------------------------------------- //----------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is an internal class that enables interactions with Zip archives // for OPC scenarios // // History: // 11/19/2004: IgorBel: Initial creation. // //----------------------------------------------------------------------------- using System; using System.IO; using System.Diagnostics; namespace MS.Internal.IO.Zip { internal sealed class ZipFileInfo { //------------------------------------------------------ // // Public Members // //----------------------------------------------------- // None //------------------------------------------------------ // // Internal Constructors // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal API Methods (although these methods are marked as // Internal they are part of the internal ZIP IO API surface // //------------------------------------------------------ internal Stream GetStream(FileMode mode, FileAccess access) { CheckDisposed(); return _fileBlock.GetStream(mode, access); } //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- internal string Name { get { CheckDisposed(); return _fileBlock.FileName; } } internal ZipArchive ZipArchive { get { CheckDisposed(); return _zipArchive; } } internal CompressionMethodEnum CompressionMethod { get { CheckDisposed(); return _fileBlock.CompressionMethod; } } internal DateTime LastModFileDateTime { get { CheckDisposed(); return ZipIOBlockManager.FromMsDosDateTime(_fileBlock.LastModFileDateTime); } } #if false internal bool EncryptedFlag { get { CheckDisposed(); return _fileBlock.EncryptedFlag; } } #endif internal DeflateOptionEnum DeflateOption { get { CheckDisposed(); return _fileBlock.DeflateOption; } } #if false internal bool StreamingCreationFlag { get { CheckDisposed(); return _fileBlock.StreamingCreationFlag; } } #endif // This ia Directory flag based on the informtion from the central directory // at the moment we have only provide reliable value for the files authored in MS-DOS // The upper byte of version made by indicating (OS) must be == 0 (MS-DOS) // for the other cases (OSes) we will return false internal bool FolderFlag { get { CheckDisposed(); return _fileBlock.FolderFlag; } } // This ia Directory flag based on the informtion from the central directory // at the moment we have only provide reliable value for the files authored in MS-DOS // The upper byte of version made by indicating (OS) must be == 0 (MS-DOS) // for the other cases (OSes) we will return false internal bool VolumeLabelFlag { get { CheckDisposed(); return _fileBlock.VolumeLabelFlag; } } //----------------------------------------------------- // Internal NON API Constructor (this constructor is marked as internal // and isNOT part of the ZIP IO API surface) // It supposed to be called only by the ZipArchive class //------------------------------------------------------ internal ZipFileInfo(ZipArchive zipArchive, ZipIOLocalFileBlock fileBlock) { Debug.Assert((fileBlock != null) && (zipArchive != null)); _fileBlock = fileBlock; _zipArchive = zipArchive; #if DEBUG // validate that date time is legal DateTime dt = LastModFileDateTime; #endif } //----------------------------------------------------- // Internal NON API property to be used to map FileInfo back to a block that needs to be deleted // (this prperty is marked as internal and isNOT part of the ZIP IO API surface) // It supposed to be called only by the ZipArchive class //------------------------------------------------------ internal ZipIOLocalFileBlock LocalFileBlock { get { return _fileBlock; } } //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- private void CheckDisposed() { _fileBlock.CheckDisposed(); } //------------------------------------------------------ // // Private Properties // //----------------------------------------------------- //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- private ZipIOLocalFileBlock _fileBlock; private ZipArchive _zipArchive; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
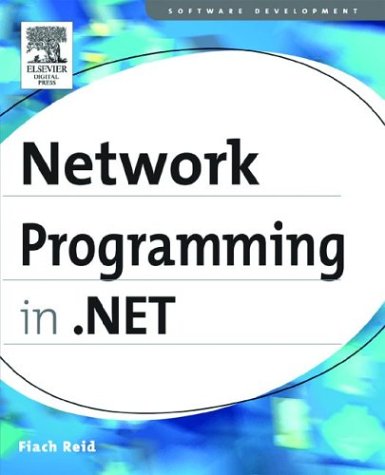
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmptyEnumerator.cs
- PropertyChangingEventArgs.cs
- ListViewGroupConverter.cs
- BlurBitmapEffect.cs
- CompiledRegexRunnerFactory.cs
- TraceHandler.cs
- PlatformCulture.cs
- PropertyMetadata.cs
- DataListAutoFormat.cs
- CallTemplateAction.cs
- Constants.cs
- HandleInitializationContext.cs
- Int16Storage.cs
- Bezier.cs
- Control.cs
- PixelShader.cs
- TextLine.cs
- ActivityTypeDesigner.xaml.cs
- CellParagraph.cs
- XmlSchemaObject.cs
- EndpointBehaviorElementCollection.cs
- BitVector32.cs
- ServiceContractGenerationContext.cs
- ToolStripSplitStackLayout.cs
- XmlNodeComparer.cs
- _SecureChannel.cs
- ArrayWithOffset.cs
- WindowsFormsHostPropertyMap.cs
- XmlSchemaFacet.cs
- ProtocolsSection.cs
- AssemblyBuilder.cs
- LongValidator.cs
- Visual3D.cs
- DateRangeEvent.cs
- BitVector32.cs
- EditorOptionAttribute.cs
- StaticFileHandler.cs
- LicenseException.cs
- XmlSchemaObjectCollection.cs
- DependencyObjectType.cs
- ResolvedKeyFrameEntry.cs
- DefaultPrintController.cs
- TrackingServices.cs
- VisualStyleInformation.cs
- CollectionViewProxy.cs
- ContextMarshalException.cs
- SelectionChangedEventArgs.cs
- SqlDataSourceQueryEditorForm.cs
- Bitmap.cs
- AccessDataSource.cs
- DatasetMethodGenerator.cs
- MemoryStream.cs
- ExtendedProtectionPolicyElement.cs
- ByteAnimationBase.cs
- DataPagerCommandEventArgs.cs
- AutoGeneratedFieldProperties.cs
- TrackingConditionCollection.cs
- MdbDataFileEditor.cs
- LinkedResource.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- Documentation.cs
- ValidationSummary.cs
- ProcessHostFactoryHelper.cs
- ResXResourceReader.cs
- PartitionedStream.cs
- WsatExtendedInformation.cs
- ClientCredentialsSecurityTokenManager.cs
- RolePrincipal.cs
- hresults.cs
- MessageQueuePermissionEntry.cs
- QuerySetOp.cs
- OracleCommandSet.cs
- Int64KeyFrameCollection.cs
- GetResponse.cs
- StorageScalarPropertyMapping.cs
- PointConverter.cs
- AsymmetricKeyExchangeFormatter.cs
- Code.cs
- SplitterCancelEvent.cs
- documentsequencetextcontainer.cs
- PenThreadWorker.cs
- ParseChildrenAsPropertiesAttribute.cs
- TemplatedMailWebEventProvider.cs
- XsdBuildProvider.cs
- SourceExpressionException.cs
- ColumnHeader.cs
- TypeDescriptorFilterService.cs
- SchemaImporter.cs
- ItemCollectionEditor.cs
- TextAutomationPeer.cs
- ListViewInsertionMark.cs
- DocumentPageViewAutomationPeer.cs
- SymbolUsageManager.cs
- XmlSchemaAny.cs
- GuidConverter.cs
- GlyphsSerializer.cs
- ToolStripTextBox.cs
- UpdateProgress.cs
- ModifierKeysConverter.cs
- PageParser.cs