Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / Mail / SevenBitStream.cs / 1305376 / SevenBitStream.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Mime { using System; using System.IO; using System.Text; ////// This stream validates outgoing bytes to be within the /// acceptible range of 0 - 127. Writes will throw if a /// value > 127 is found. /// internal class SevenBitStream : DelegatedStream, IEncodableStream { ////// ctor. /// /// Underlying stream internal SevenBitStream(Stream stream) : base(stream) { } ////// Writes the specified content to the underlying stream /// /// Buffer to write /// Offset within buffer to start writing /// Count of bytes to write /// Callback to call when write completes /// State to pass to callback public override IAsyncResult BeginWrite(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { if (buffer == null) throw new ArgumentNullException("buffer"); if (offset < 0 || offset >= buffer.Length) throw new ArgumentOutOfRangeException("offset"); if (offset + count > buffer.Length) throw new ArgumentOutOfRangeException("count"); CheckBytes(buffer, offset, count); IAsyncResult result = base.BeginWrite(buffer, offset, count, callback, state); return result; } ////// Writes the specified content to the underlying stream /// /// Buffer to write /// Offset within buffer to start writing /// Count of bytes to write public override void Write(byte[] buffer, int offset, int count) { if (buffer == null) throw new ArgumentNullException("buffer"); if (offset < 0 || offset >= buffer.Length) throw new ArgumentOutOfRangeException("offset"); if (offset + count > buffer.Length) throw new ArgumentOutOfRangeException("count"); CheckBytes(buffer, offset, count); base.Write(buffer, offset, count); } // helper methods ////// Checks the data in the buffer for bytes > 127. /// /// Buffer containing data /// Offset within buffer to start checking /// Count of bytes to check void CheckBytes(byte[] buffer, int offset, int count) { for (int i = count; i < offset + count; i++) { if (buffer[i] > 127) throw new FormatException(SR.GetString(SR.Mail7BitStreamInvalidCharacter)); } } public int DecodeBytes(byte[] buffer, int offset, int count) { throw new NotImplementedException(); } //nothing to "encode" here so all we're actually doing is folding public int EncodeBytes(byte[] buffer, int offset, int count) { throw new NotImplementedException(); } public Stream GetStream() { return this; } public string GetEncodedString() { throw new NotImplementedException(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Mime { using System; using System.IO; using System.Text; ////// This stream validates outgoing bytes to be within the /// acceptible range of 0 - 127. Writes will throw if a /// value > 127 is found. /// internal class SevenBitStream : DelegatedStream, IEncodableStream { ////// ctor. /// /// Underlying stream internal SevenBitStream(Stream stream) : base(stream) { } ////// Writes the specified content to the underlying stream /// /// Buffer to write /// Offset within buffer to start writing /// Count of bytes to write /// Callback to call when write completes /// State to pass to callback public override IAsyncResult BeginWrite(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { if (buffer == null) throw new ArgumentNullException("buffer"); if (offset < 0 || offset >= buffer.Length) throw new ArgumentOutOfRangeException("offset"); if (offset + count > buffer.Length) throw new ArgumentOutOfRangeException("count"); CheckBytes(buffer, offset, count); IAsyncResult result = base.BeginWrite(buffer, offset, count, callback, state); return result; } ////// Writes the specified content to the underlying stream /// /// Buffer to write /// Offset within buffer to start writing /// Count of bytes to write public override void Write(byte[] buffer, int offset, int count) { if (buffer == null) throw new ArgumentNullException("buffer"); if (offset < 0 || offset >= buffer.Length) throw new ArgumentOutOfRangeException("offset"); if (offset + count > buffer.Length) throw new ArgumentOutOfRangeException("count"); CheckBytes(buffer, offset, count); base.Write(buffer, offset, count); } // helper methods ////// Checks the data in the buffer for bytes > 127. /// /// Buffer containing data /// Offset within buffer to start checking /// Count of bytes to check void CheckBytes(byte[] buffer, int offset, int count) { for (int i = count; i < offset + count; i++) { if (buffer[i] > 127) throw new FormatException(SR.GetString(SR.Mail7BitStreamInvalidCharacter)); } } public int DecodeBytes(byte[] buffer, int offset, int count) { throw new NotImplementedException(); } //nothing to "encode" here so all we're actually doing is folding public int EncodeBytes(byte[] buffer, int offset, int count) { throw new NotImplementedException(); } public Stream GetStream() { return this; } public string GetEncodedString() { throw new NotImplementedException(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
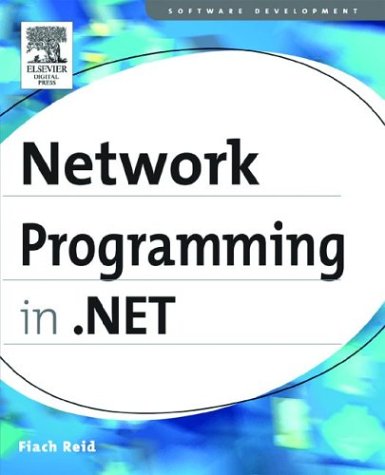
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStripPanelRow.cs
- InternalControlCollection.cs
- unsafenativemethodsother.cs
- RadioButtonPopupAdapter.cs
- ParameterReplacerVisitor.cs
- GenerateTemporaryAssemblyTask.cs
- FindCriteriaElement.cs
- Style.cs
- ClientOptions.cs
- TrackingMemoryStreamFactory.cs
- BulletDecorator.cs
- ListViewContainer.cs
- ReadOnlyHierarchicalDataSourceView.cs
- Cursors.cs
- Transaction.cs
- ColumnMapTranslator.cs
- CatalogPart.cs
- InputEventArgs.cs
- CacheMemory.cs
- CalendarDateChangedEventArgs.cs
- HttpContext.cs
- CheckBoxList.cs
- LinkedResource.cs
- handlecollector.cs
- ByteAnimationUsingKeyFrames.cs
- PerformanceCounterLib.cs
- CustomAttribute.cs
- WebPartManager.cs
- RuleAction.cs
- HtmlAnchor.cs
- DynamicILGenerator.cs
- VariableModifiersHelper.cs
- TransactionContext.cs
- ApplicationFileParser.cs
- basecomparevalidator.cs
- PersonalizationProviderHelper.cs
- Geometry3D.cs
- RectAnimation.cs
- CurrencyWrapper.cs
- DataTablePropertyDescriptor.cs
- CrossAppDomainChannel.cs
- RemotingException.cs
- DnsPermission.cs
- Highlights.cs
- ADMembershipProvider.cs
- NavigationEventArgs.cs
- BidirectionalDictionary.cs
- Type.cs
- HtmlElementErrorEventArgs.cs
- LastQueryOperator.cs
- DeflateEmulationStream.cs
- ImageMapEventArgs.cs
- Formatter.cs
- FileUpload.cs
- WebPartEditVerb.cs
- PageThemeCodeDomTreeGenerator.cs
- ModelTreeEnumerator.cs
- JsonFormatGeneratorStatics.cs
- ValidationEventArgs.cs
- TaskFileService.cs
- Figure.cs
- ArrayList.cs
- HMACSHA1.cs
- DeobfuscatingStream.cs
- IListConverters.cs
- ObjectMaterializedEventArgs.cs
- XmlQualifiedName.cs
- NameValueCollection.cs
- PolyLineSegmentFigureLogic.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- NeutralResourcesLanguageAttribute.cs
- ButtonBaseAutomationPeer.cs
- StreamGeometry.cs
- RightsManagementInformation.cs
- TextBoxBase.cs
- ListItemConverter.cs
- ContainerFilterService.cs
- OwnerDrawPropertyBag.cs
- ObjectManager.cs
- DocumentPaginator.cs
- ClaimTypeElement.cs
- MouseEvent.cs
- ToolBarButtonClickEvent.cs
- ProcessStartInfo.cs
- ApplicationManager.cs
- XmlCountingReader.cs
- BaseTemplateParser.cs
- HtmlImageAdapter.cs
- ListItemCollection.cs
- SoapElementAttribute.cs
- UrlAuthFailureHandler.cs
- GeneralTransform3DTo2D.cs
- XmlQualifiedName.cs
- ConnectionStringsSection.cs
- SingleConverter.cs
- Utils.cs
- ContainerSelectorGlyph.cs
- AsymmetricSignatureFormatter.cs
- FileSystemWatcher.cs
- SqlException.cs