Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ContextMenu.cs / 1 / ContextMenu.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using Microsoft.Win32; using System; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Security; using System.Security.Permissions; ////// /// This class is used to put context menus on your form and show them for /// controls at runtime. It basically acts like a regular Menu control, /// but can be set for the ContextMenu property that most controls have. /// [ DefaultEvent("Popup"), ] public class ContextMenu : Menu { private EventHandler onPopup; private EventHandler onCollapse; internal Control sourceControl; private RightToLeft rightToLeft = System.Windows.Forms.RightToLeft.Inherit; ////// /// Creates a new ContextMenu object with no items in it by default. /// public ContextMenu() : base(null) { } ////// /// Creates a ContextMenu object with the given MenuItems. /// public ContextMenu(MenuItem[] menuItems) : base(menuItems) { } ////// /// The last control that was acted upon that resulted in this context /// menu being displayed. /// VSWHIDBEY 426099 - add demand for AllWindows. /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ContextMenuSourceControlDescr) ] public Control SourceControl { [UIPermission(SecurityAction.Demand, Window=UIPermissionWindow.AllWindows)] get { return sourceControl; } } ////// /// [SRDescription(SR.MenuItemOnInitDescr)] public event EventHandler Popup { add { onPopup += value; } remove { onPopup -= value; } } ///[To be supplied.] ////// /// Fires when the context menu collapses. /// [SRDescription(SR.ContextMenuCollapseDescr)] public event EventHandler Collapse { add { onCollapse += value; } remove { onCollapse -= value; } } ////// /// This is used for international applications where the language /// is written from RightToLeft. When this property is true, /// text alignment and reading order will be from right to left. /// // VSWhidbey 164244: Add a DefaultValue attribute so that the Reset context menu becomes // available in the Property Grid but the default value remains No. [ Localizable(true), DefaultValue(RightToLeft.No), SRDescription(SR.MenuRightToLeftDescr) ] public virtual RightToLeft RightToLeft { get { if (System.Windows.Forms.RightToLeft.Inherit == rightToLeft) { if (sourceControl != null) { return ((Control)sourceControl).RightToLeft; } else { return RightToLeft.No; } } else { return rightToLeft; } } set { //valid values are 0x0 to 0x2. if (!ClientUtils.IsEnumValid(value, (int)value, (int)RightToLeft.No, (int)RightToLeft.Inherit)){ throw new InvalidEnumArgumentException("RightToLeft", (int)value, typeof(RightToLeft)); } if (RightToLeft != value) { rightToLeft = value; UpdateRtl((value == System.Windows.Forms.RightToLeft.Yes)); } } } internal override bool RenderIsRightToLeft { get { return (rightToLeft == System.Windows.Forms.RightToLeft.Yes); } } ////// /// Fires the popup event /// protected internal virtual void OnPopup(EventArgs e) { if (onPopup != null) { onPopup(this, e); } } ////// /// Fires the collapse event /// protected internal virtual void OnCollapse(EventArgs e) { if (onCollapse != null) { onCollapse(this, e); } } ////// /// ///[ System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode), System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode) ] protected internal virtual bool ProcessCmdKey(ref Message msg, Keys keyData, Control control) { sourceControl = control; return ProcessCmdKey(ref msg, keyData); } private void ResetRightToLeft() { RightToLeft = RightToLeft.No; } /// /// /// Returns true if the RightToLeft should be persisted in code gen. /// internal virtual bool ShouldSerializeRightToLeft() { if (System.Windows.Forms.RightToLeft.Inherit == rightToLeft) { return false; } return true; } ////// /// Displays the context menu at the specified position. This method /// doesn't return until the menu is dismissed. /// public void Show(Control control, Point pos) { Show(control, pos, NativeMethods.TPM_VERTICAL | NativeMethods.TPM_RIGHTBUTTON); } ////// /// Displays the context menu at the specified position. This method /// doesn't return until the menu is dismissed. /// public void Show(Control control, Point pos, LeftRightAlignment alignment) { // This code below looks wrong but it's correct. // WinForms Left alignment means we want the menu to show up left of the point it is invoked from. // We specify TPM_RIGHTALIGN which tells win32 to align the right side of this // menu with the point (which aligns it Left visually) if (alignment == LeftRightAlignment.Left) { Show(control, pos, NativeMethods.TPM_VERTICAL | NativeMethods.TPM_RIGHTBUTTON | NativeMethods.TPM_RIGHTALIGN); } else { Show(control, pos, NativeMethods.TPM_VERTICAL | NativeMethods.TPM_RIGHTBUTTON | NativeMethods.TPM_LEFTALIGN); } } private void Show(Control control, Point pos, int flags) { if (control == null) throw new ArgumentNullException("control"); if (!control.IsHandleCreated || !control.Visible) throw new ArgumentException(SR.GetString(SR.ContextMenuInvalidParent), "control"); sourceControl = control; OnPopup(EventArgs.Empty); pos = control.PointToScreen(pos); SafeNativeMethods.TrackPopupMenuEx(new HandleRef(this, Handle), flags, pos.X, pos.Y, new HandleRef(control, control.Handle), null); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
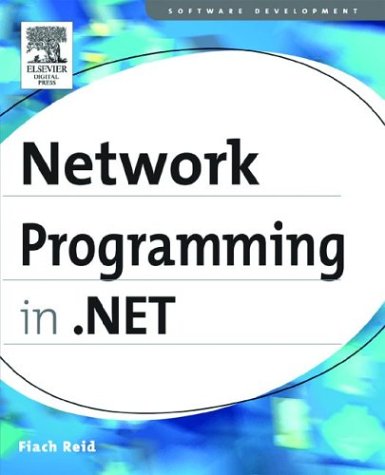
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WarningException.cs
- ElementsClipboardData.cs
- XmlSchemaSearchPattern.cs
- EntityDataSourceDesigner.cs
- SafeProcessHandle.cs
- Transform3D.cs
- HtmlShim.cs
- OdbcConnectionPoolProviderInfo.cs
- Menu.cs
- ExceptionValidationRule.cs
- FixedPageStructure.cs
- MappedMetaModel.cs
- ClipboardData.cs
- ContainerVisual.cs
- PersonalizationProviderCollection.cs
- WaitingCursor.cs
- RadioButton.cs
- StreamReader.cs
- FontDialog.cs
- AnimatedTypeHelpers.cs
- ContentDisposition.cs
- IsolatedStorageFileStream.cs
- InfocardChannelParameter.cs
- ResolveCriteriaApril2005.cs
- WCFServiceClientProxyGenerator.cs
- ContractReference.cs
- CodeTypeDeclarationCollection.cs
- CompilerErrorCollection.cs
- AnimationClockResource.cs
- CatalogPartCollection.cs
- RadioButtonPopupAdapter.cs
- HtmlControlPersistable.cs
- BigInt.cs
- PeerApplication.cs
- Pts.cs
- WorkerRequest.cs
- SqlDataSourceCache.cs
- TreeView.cs
- PropertyContainer.cs
- SignatureToken.cs
- SamlSerializer.cs
- SqlDataRecord.cs
- DbUpdateCommandTree.cs
- AttachedPropertyDescriptor.cs
- CodeSnippetExpression.cs
- Drawing.cs
- RequiredAttributeAttribute.cs
- HtmlPanelAdapter.cs
- ReferenceService.cs
- RowToFieldTransformer.cs
- AnnotationAdorner.cs
- InvalidateEvent.cs
- DockPattern.cs
- ProcessModelSection.cs
- SchemaNotation.cs
- DataGridItem.cs
- WebPartMinimizeVerb.cs
- DefaultValueTypeConverter.cs
- ManagementObject.cs
- Animatable.cs
- DataDocumentXPathNavigator.cs
- WebBrowsableAttribute.cs
- EntityContainerEntitySet.cs
- PenLineJoinValidation.cs
- GPRECTF.cs
- PopupControlService.cs
- FixedSOMContainer.cs
- PathParser.cs
- OutputWindow.cs
- PropertyGridEditorPart.cs
- Utility.cs
- FigureParagraph.cs
- CommandDevice.cs
- SnapLine.cs
- PathFigureCollection.cs
- SupportingTokenProviderSpecification.cs
- GridView.cs
- FileDataSourceCache.cs
- connectionpool.cs
- ValidationError.cs
- DataGridItem.cs
- _HeaderInfoTable.cs
- WebMessageEncodingElement.cs
- HttpRequest.cs
- Wizard.cs
- DataSourceViewSchemaConverter.cs
- MappingSource.cs
- IdentityManager.cs
- ReferencedCollectionType.cs
- BamlTreeUpdater.cs
- DataGridColumnCollection.cs
- TranslateTransform.cs
- FileDialogCustomPlacesCollection.cs
- DatatypeImplementation.cs
- EditorPart.cs
- XmlWrappingReader.cs
- SwitchElementsCollection.cs
- MaskedTextProvider.cs
- SqlNotificationEventArgs.cs
- IntegerValidatorAttribute.cs