Code:
/ DotNET / DotNET / 8.0 / untmp / Orcas / RTM / ndp / fx / src / xsp / System / Web / Extensions / Script / Services / WCFServiceClientProxyGenerator.cs / 1 / WCFServiceClientProxyGenerator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Script.Services { using System; using System.Globalization; using System.ServiceModel.Description; using System.Text; internal class WCFServiceClientProxyGenerator : ClientProxyGenerator { const int MaxIdentifierLength = 511; const string DataContractXsdBaseNamespace = @"http://schemas.datacontract.org/2004/07/"; string _path; // Similar to proxy generation code in WCF System.Runtime.Serialization.CodeExporter // to generate CLR namespace from DataContract namespace private static void AddToNamespace(StringBuilder builder, string fragment) { if (fragment == null) { return; } bool isStart = true; for (int i = 0; i < fragment.Length && builder.Length < MaxIdentifierLength; i++) { char c = fragment[i]; if (IsValid(c)) { if (isStart && !IsValidStart(c)) { builder.Append("_"); } builder.Append(c); isStart = false; } else if ((c == '.' || c == '/' || c == ':') && (builder.Length == 1 || (builder.Length > 1 && builder[builder.Length - 1] != '.'))) { builder.Append('.'); isStart = true; } } } protected override string GetProxyPath() { return _path; } internal static string GetClientProxyScript(Type contractType, string path, bool debugMode) { ContractDescription contract = ContractDescription.GetContract(contractType); WebServiceData webServiceData = WebServiceData.GetWebServiceData(contract); WCFServiceClientProxyGenerator proxyGenerator = new WCFServiceClientProxyGenerator(path, debugMode); return proxyGenerator.GetClientProxyScript(webServiceData); } // Similar to proxy generation code in WCF System.Runtime.Serialization.CodeExporter // to generate CLR namespace from DataContract namespace protected override string GetClientTypeNamespace(string ns) { if (string.IsNullOrEmpty(ns)) { return String.Empty; } Uri uri = null; StringBuilder builder = new StringBuilder(); if (Uri.TryCreate(ns, UriKind.RelativeOrAbsolute, out uri)) { if (!uri.IsAbsoluteUri) { AddToNamespace(builder, uri.OriginalString); } else { string uriString = uri.AbsoluteUri; if (uriString.StartsWith(DataContractXsdBaseNamespace, StringComparison.Ordinal)) { AddToNamespace(builder, uriString.Substring(DataContractXsdBaseNamespace.Length)); } else { string host = uri.Host; if (host != null) { AddToNamespace(builder, host); } string path = uri.PathAndQuery; if (path != null) { AddToNamespace(builder, path); } } } } if (builder.Length == 0) { return String.Empty; } int length = builder.Length; if (builder[builder.Length - 1] == '.') { length--; } length = Math.Min(MaxIdentifierLength, length); return builder.ToString(0, length); } protected override string GetProxyTypeName(WebServiceData data) { return GetClientTypeNamespace(data.TypeData.TypeName); } // Similar to proxy generation code in WCF System.Runtime.Serialization.CodeExporter // to generate CLR namespace from DataContract namespace private static bool IsValid(char c) { UnicodeCategory uc = Char.GetUnicodeCategory(c); // each char must be Lu, Ll, Lt, Lm, Lo, Nd, Mn, Mc, Pc switch (uc) { case UnicodeCategory.UppercaseLetter: // Lu case UnicodeCategory.LowercaseLetter: // Ll case UnicodeCategory.TitlecaseLetter: // Lt case UnicodeCategory.ModifierLetter: // Lm case UnicodeCategory.OtherLetter: // Lo case UnicodeCategory.DecimalDigitNumber: // Nd case UnicodeCategory.NonSpacingMark: // Mn case UnicodeCategory.SpacingCombiningMark: // Mc case UnicodeCategory.ConnectorPunctuation: // Pc return true; default: return false; } } // Similar to proxy generation code in WCF System.Runtime.Serialization.CodeExporter // to generate CLR namespace from DataContract namespace private static bool IsValidStart(char c) { return (Char.GetUnicodeCategory(c) != UnicodeCategory.DecimalDigitNumber); } internal WCFServiceClientProxyGenerator(string path, bool debugMode) { this._path = path; this._debugMode = debugMode; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
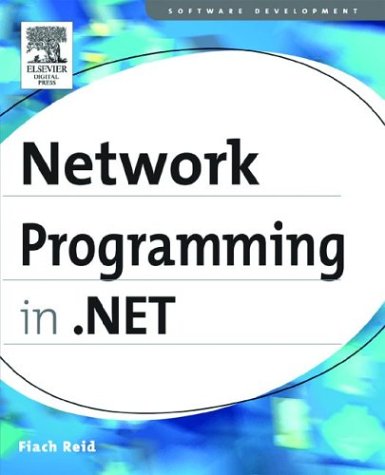
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LexicalChunk.cs
- ElementProxy.cs
- TreeNodeBindingCollection.cs
- SmuggledIUnknown.cs
- StatusBarItem.cs
- _Semaphore.cs
- EncodingFallbackAwareXmlTextWriter.cs
- RtfNavigator.cs
- Tracking.cs
- Encoder.cs
- DesignerSerializationOptionsAttribute.cs
- UIPropertyMetadata.cs
- PerfCounters.cs
- GridSplitter.cs
- ReflectEventDescriptor.cs
- XmlnsDictionary.cs
- ImageDrawing.cs
- EntityDataReader.cs
- RawKeyboardInputReport.cs
- X509Utils.cs
- WindowsTitleBar.cs
- WinEventWrap.cs
- FixedSOMFixedBlock.cs
- BrowserTree.cs
- RestHandlerFactory.cs
- XmlWriter.cs
- processwaithandle.cs
- FilterableAttribute.cs
- Decimal.cs
- BezierSegment.cs
- TargetInvocationException.cs
- SqlFlattener.cs
- SqlCachedBuffer.cs
- LogEntrySerializationException.cs
- Canvas.cs
- PageWrapper.cs
- PropertyValueEditor.cs
- TimeStampChecker.cs
- Misc.cs
- InnerItemCollectionView.cs
- WebPartPersonalization.cs
- SemanticResultKey.cs
- RadioButtonBaseAdapter.cs
- EventSinkActivity.cs
- ParameterCollection.cs
- PropertyEntry.cs
- PointHitTestResult.cs
- DecimalAnimationBase.cs
- ServicePoint.cs
- SystemInfo.cs
- DataBoundLiteralControl.cs
- GeneralTransform3DTo2DTo3D.cs
- TableItemStyle.cs
- StyleModeStack.cs
- DataTemplateKey.cs
- printdlgexmarshaler.cs
- Privilege.cs
- MenuItem.cs
- InputReport.cs
- RijndaelManagedTransform.cs
- DelimitedListTraceListener.cs
- BindingExpressionUncommonField.cs
- ThemeInfoAttribute.cs
- DataObjectFieldAttribute.cs
- BreadCrumbTextConverter.cs
- WebPartZoneDesigner.cs
- IteratorFilter.cs
- TableAdapterManagerHelper.cs
- PageContentCollection.cs
- AssertFilter.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- ListBox.cs
- SqlDataSourceConfigureSelectPanel.cs
- DocumentDesigner.cs
- WorkflowValidationFailedException.cs
- WindowsFormsLinkLabel.cs
- HttpWebResponse.cs
- coordinatorscratchpad.cs
- KeySplineConverter.cs
- MultiTouchSystemGestureLogic.cs
- PropertyPathConverter.cs
- ApplicationDirectoryMembershipCondition.cs
- ReturnValue.cs
- FrameworkEventSource.cs
- AsyncDataRequest.cs
- TreeSet.cs
- Error.cs
- CodeConditionStatement.cs
- CharacterHit.cs
- FixUpCollection.cs
- EffectiveValueEntry.cs
- SessionEndingCancelEventArgs.cs
- SqlDependency.cs
- ColorMap.cs
- FilterException.cs
- XPathArrayIterator.cs
- CodeComment.cs
- PeerResolverElement.cs
- ProfileSettingsCollection.cs
- NullToBooleanConverter.cs