Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / Generated / Rect.cs / 1305600 / Rect.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.WindowsBase; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; using System.Windows.Converters; using System.Windows; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows { [Serializable] [TypeConverter(typeof(RectConverter))] [ValueSerializer(typeof(RectValueSerializer))] // Used by MarkupWriter partial struct Rect : IFormattable { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Compares two Rect instances for exact equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Rect instances are exactly equal, false otherwise /// /// The first Rect to compare /// The second Rect to compare public static bool operator == (Rect rect1, Rect rect2) { return rect1.X == rect2.X && rect1.Y == rect2.Y && rect1.Width == rect2.Width && rect1.Height == rect2.Height; } ////// Compares two Rect instances for exact inequality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Rect instances are exactly unequal, false otherwise /// /// The first Rect to compare /// The second Rect to compare public static bool operator != (Rect rect1, Rect rect2) { return !(rect1 == rect2); } ////// Compares two Rect instances for object equality. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the two Rect instances are exactly equal, false otherwise /// /// The first Rect to compare /// The second Rect to compare public static bool Equals (Rect rect1, Rect rect2) { if (rect1.IsEmpty) { return rect2.IsEmpty; } else { return rect1.X.Equals(rect2.X) && rect1.Y.Equals(rect2.Y) && rect1.Width.Equals(rect2.Width) && rect1.Height.Equals(rect2.Height); } } ////// Equals - compares this Rect with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the object is an instance of Rect and if it's equal to "this". /// /// The object to compare to "this" public override bool Equals(object o) { if ((null == o) || !(o is Rect)) { return false; } Rect value = (Rect)o; return Rect.Equals(this,value); } ////// Equals - compares this Rect with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if "value" is equal to "this". /// /// The Rect to compare to "this" public bool Equals(Rect value) { return Rect.Equals(this, value); } ////// Returns the HashCode for this Rect /// ////// int - the HashCode for this Rect /// public override int GetHashCode() { if (IsEmpty) { return 0; } else { // Perform field-by-field XOR of HashCodes return X.GetHashCode() ^ Y.GetHashCode() ^ Width.GetHashCode() ^ Height.GetHashCode(); } } ////// Parse - returns an instance converted from the provided string using /// the culture "en-US" /// string with Rect data /// public static Rect Parse(string source) { IFormatProvider formatProvider = System.Windows.Markup.TypeConverterHelper.InvariantEnglishUS; TokenizerHelper th = new TokenizerHelper(source, formatProvider); Rect value; String firstToken = th.NextTokenRequired(); // The token will already have had whitespace trimmed so we can do a // simple string compare. if (firstToken == "Empty") { value = Empty; } else { value = new Rect( Convert.ToDouble(firstToken, formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider)); } // There should be no more tokens in this string. th.LastTokenRequired(); return value; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal string ConvertToString(string format, IFormatProvider provider) { if (IsEmpty) { return "Empty"; } // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "{1:" + format + "}{0}{2:" + format + "}{0}{3:" + format + "}{0}{4:" + format + "}", separator, _x, _y, _width, _height); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal double _x; internal double _y; internal double _width; internal double _height; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.WindowsBase; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; using System.Windows.Converters; using System.Windows; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows { [Serializable] [TypeConverter(typeof(RectConverter))] [ValueSerializer(typeof(RectValueSerializer))] // Used by MarkupWriter partial struct Rect : IFormattable { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Compares two Rect instances for exact equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Rect instances are exactly equal, false otherwise /// /// The first Rect to compare /// The second Rect to compare public static bool operator == (Rect rect1, Rect rect2) { return rect1.X == rect2.X && rect1.Y == rect2.Y && rect1.Width == rect2.Width && rect1.Height == rect2.Height; } ////// Compares two Rect instances for exact inequality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Rect instances are exactly unequal, false otherwise /// /// The first Rect to compare /// The second Rect to compare public static bool operator != (Rect rect1, Rect rect2) { return !(rect1 == rect2); } ////// Compares two Rect instances for object equality. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the two Rect instances are exactly equal, false otherwise /// /// The first Rect to compare /// The second Rect to compare public static bool Equals (Rect rect1, Rect rect2) { if (rect1.IsEmpty) { return rect2.IsEmpty; } else { return rect1.X.Equals(rect2.X) && rect1.Y.Equals(rect2.Y) && rect1.Width.Equals(rect2.Width) && rect1.Height.Equals(rect2.Height); } } ////// Equals - compares this Rect with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the object is an instance of Rect and if it's equal to "this". /// /// The object to compare to "this" public override bool Equals(object o) { if ((null == o) || !(o is Rect)) { return false; } Rect value = (Rect)o; return Rect.Equals(this,value); } ////// Equals - compares this Rect with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if "value" is equal to "this". /// /// The Rect to compare to "this" public bool Equals(Rect value) { return Rect.Equals(this, value); } ////// Returns the HashCode for this Rect /// ////// int - the HashCode for this Rect /// public override int GetHashCode() { if (IsEmpty) { return 0; } else { // Perform field-by-field XOR of HashCodes return X.GetHashCode() ^ Y.GetHashCode() ^ Width.GetHashCode() ^ Height.GetHashCode(); } } ////// Parse - returns an instance converted from the provided string using /// the culture "en-US" /// string with Rect data /// public static Rect Parse(string source) { IFormatProvider formatProvider = System.Windows.Markup.TypeConverterHelper.InvariantEnglishUS; TokenizerHelper th = new TokenizerHelper(source, formatProvider); Rect value; String firstToken = th.NextTokenRequired(); // The token will already have had whitespace trimmed so we can do a // simple string compare. if (firstToken == "Empty") { value = Empty; } else { value = new Rect( Convert.ToDouble(firstToken, formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider)); } // There should be no more tokens in this string. th.LastTokenRequired(); return value; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal string ConvertToString(string format, IFormatProvider provider) { if (IsEmpty) { return "Empty"; } // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "{1:" + format + "}{0}{2:" + format + "}{0}{3:" + format + "}{0}{4:" + format + "}", separator, _x, _y, _width, _height); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal double _x; internal double _y; internal double _width; internal double _height; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
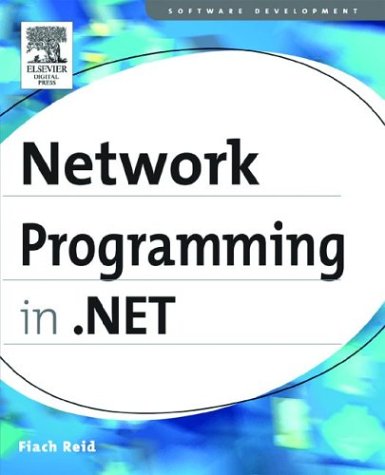
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RunClient.cs
- WebPartVerbCollection.cs
- SqlXmlStorage.cs
- XmlBufferReader.cs
- SoapIgnoreAttribute.cs
- odbcmetadatacollectionnames.cs
- SerializationUtility.cs
- PartialArray.cs
- XPathMessageFilter.cs
- LayoutInformation.cs
- OverrideMode.cs
- DeflateInput.cs
- updatecommandorderer.cs
- RangeValuePatternIdentifiers.cs
- AndMessageFilter.cs
- Site.cs
- XPathConvert.cs
- SqlFunctions.cs
- UdpDiscoveryEndpointElement.cs
- TextTreeUndoUnit.cs
- ScrollProperties.cs
- externdll.cs
- InvalidWorkflowException.cs
- WindowsFormsHostAutomationPeer.cs
- ResetableIterator.cs
- BitmapEffectInput.cs
- TypeExtension.cs
- TableCellAutomationPeer.cs
- ClusterRegistryConfigurationProvider.cs
- Vector3DCollection.cs
- UpdateManifestForBrowserApplication.cs
- TextBoxBase.cs
- MenuBindingsEditor.cs
- AncestorChangedEventArgs.cs
- SqlProviderManifest.cs
- StreamUpdate.cs
- EncryptedXml.cs
- Int64AnimationBase.cs
- TileBrush.cs
- QuadraticBezierSegment.cs
- Matrix.cs
- ScrollData.cs
- ListItemsCollectionEditor.cs
- XmlDataFileEditor.cs
- BindingMAnagerBase.cs
- DirectoryRedirect.cs
- LocatorPart.cs
- Main.cs
- OracleParameterBinding.cs
- HelpHtmlBuilder.cs
- WebPartCancelEventArgs.cs
- shaperfactory.cs
- GeneralTransform3DCollection.cs
- _SSPIWrapper.cs
- ItemCheckedEvent.cs
- SqlRewriteScalarSubqueries.cs
- HTMLTagNameToTypeMapper.cs
- EventManager.cs
- XmlSubtreeReader.cs
- DrawingCollection.cs
- TraceSource.cs
- BamlResourceDeserializer.cs
- PageAsyncTaskManager.cs
- ThaiBuddhistCalendar.cs
- ComNativeDescriptor.cs
- TableHeaderCell.cs
- CollaborationHelperFunctions.cs
- ScrollEventArgs.cs
- Lasso.cs
- Filter.cs
- FieldTemplateFactory.cs
- RangeValuePattern.cs
- NotificationContext.cs
- DefaultTypeArgumentAttribute.cs
- ConfigXmlText.cs
- SafeCryptContextHandle.cs
- OutputWindow.cs
- ToolStripTemplateNode.cs
- KeyBinding.cs
- JournalEntryListConverter.cs
- RegexBoyerMoore.cs
- TwoPhaseCommitProxy.cs
- SqlConnectionPoolProviderInfo.cs
- ProcessModelSection.cs
- OperationDescriptionCollection.cs
- WindowsFormsHost.cs
- SerializationStore.cs
- SelectionHighlightInfo.cs
- PenThreadPool.cs
- CommandField.cs
- XMLSchema.cs
- SplitContainerDesigner.cs
- BackgroundFormatInfo.cs
- DocumentViewer.cs
- StandardOleMarshalObject.cs
- TextTreeInsertElementUndoUnit.cs
- BaseParser.cs
- GraphicsState.cs
- CompressedStack.cs
- SimpleHandlerBuildProvider.cs