Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / figurelengthconverter.cs / 1305600 / figurelengthconverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Figure length converter implementation // // History: // 06/23/2005 : ghermann - Created (Adapted from GridLengthConverter) // //--------------------------------------------------------------------------- using MS.Internal; using MS.Utility; using System.ComponentModel; using System.Windows; using System; using System.Security; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Windows.Markup; namespace System.Windows { ////// FigureLengthConverter - Converter class for converting /// instances of other types to and from FigureLength instances. /// public class FigureLengthConverter: TypeConverter { //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Checks whether or not this class can convert from a given type. /// /// The ITypeDescriptorContext /// for this call. /// The Type being queried for support. ////// public override bool CanConvertFrom( ITypeDescriptorContext typeDescriptorContext, Type sourceType) { // We can only handle strings, integral and floating types TypeCode tc = Type.GetTypeCode(sourceType); switch (tc) { case TypeCode.String: case TypeCode.Decimal: case TypeCode.Single: case TypeCode.Double: case TypeCode.Int16: case TypeCode.Int32: case TypeCode.Int64: case TypeCode.UInt16: case TypeCode.UInt32: case TypeCode.UInt64: return true; default: return false; } } ///true if thie converter can convert from the provided type, ///false otherwise. ////// Checks whether or not this class can convert to a given type. /// /// The ITypeDescriptorContext /// for this call. /// The Type being queried for support. ////// public override bool CanConvertTo( ITypeDescriptorContext typeDescriptorContext, Type destinationType) { return ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string) ); } ///true if this converter can convert to the provided type, ///false otherwise. ////// Attempts to convert to a FigureLength from the given object. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to a FigureLength. ////// The FigureLength instance which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null /// and is not a valid type which can be converted to a FigureLength. /// public override object ConvertFrom( ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object source) { if (source != null) { if (source is string) { return (FromString((string)source, cultureInfo)); } else { return new FigureLength(Convert.ToDouble(source, cultureInfo)); //conversion from numeric type } } throw GetConvertFromException(source); } ////// Attempts to convert a FigureLength instance to the given type. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The FigureLength to convert. /// The type to which to convert the FigureLength instance. ////// The object which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the object is not null and is not a FigureLength, /// or if the destinationType isn't one of the valid destination types. /// ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for FigureLength, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if ( value != null && value is FigureLength ) { FigureLength fl = (FigureLength)value; if (destinationType == typeof(string)) { return (ToString(fl, cultureInfo)); } if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(FigureLength).GetConstructor(new Type[] { typeof(double), typeof(FigureUnitType) }); return (new InstanceDescriptor(ci, new object[] { fl.Value, fl.FigureUnitType })); } } throw GetConvertToException(value, destinationType); } #endregion Public Methods //-------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods ////// Converts a FigureLength instance to a String given the CultureInfo. /// /// FigureLength instance to convert. /// Culture Info. ///String representation of the object. static internal string ToString(FigureLength fl, CultureInfo cultureInfo) { switch (fl.FigureUnitType) { // for Auto print out "Auto". value is always "1.0" case FigureUnitType.Auto: return ("Auto"); case FigureUnitType.Pixel: return Convert.ToString(fl.Value, cultureInfo); default: return Convert.ToString(fl.Value, cultureInfo) + " " + fl.FigureUnitType.ToString(); } } ////// Parses a FigureLength from a string given the CultureInfo. /// /// String to parse from. /// Culture Info. ///Newly created FigureLength instance. ////// Formats: /// "[value][unit]" /// [value] is a double /// [unit] is a string in FigureLength._unitTypes connected to a FigureUnitType /// "[value]" /// As above, but the FigureUnitType is assumed to be FigureUnitType.Pixel /// "[unit]" /// As above, but the value is assumed to be 1.0 /// This is only acceptable for a subset of FigureUnitType: Auto /// static internal FigureLength FromString(string s, CultureInfo cultureInfo) { double value; FigureUnitType unit; XamlFigureLengthSerializer.FromString(s, cultureInfo, out value, out unit); return (new FigureLength(value, unit)); } #endregion Internal Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
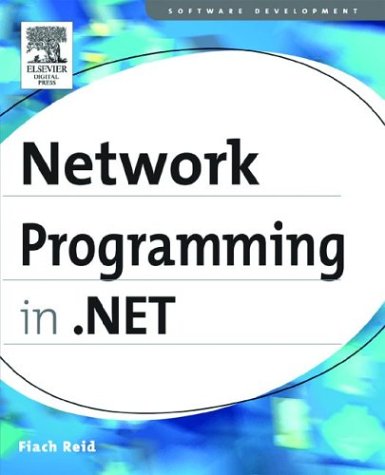
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlLiteralTextAdapter.cs
- DBDataPermission.cs
- InputLangChangeRequestEvent.cs
- Help.cs
- OrderPreservingPipeliningSpoolingTask.cs
- DbConnectionPoolGroupProviderInfo.cs
- ContextMenuStripGroupCollection.cs
- FrameworkElementFactoryMarkupObject.cs
- Expressions.cs
- PreloadHost.cs
- SetIterators.cs
- TextCompositionEventArgs.cs
- SqlConnectionPoolProviderInfo.cs
- TextEditorSelection.cs
- Flattener.cs
- StringFormat.cs
- ISFTagAndGuidCache.cs
- SettingsSection.cs
- FontFamilyIdentifier.cs
- TextChangedEventArgs.cs
- DisplayInformation.cs
- DataObject.cs
- OperationPerformanceCounters.cs
- XPathBinder.cs
- FixedSOMImage.cs
- TypeConverterHelper.cs
- GridViewUpdatedEventArgs.cs
- BamlCollectionHolder.cs
- SimpleBitVector32.cs
- NamespaceMapping.cs
- WebBrowserBase.cs
- Latin1Encoding.cs
- HtmlInputText.cs
- ArrayExtension.cs
- WindowsTooltip.cs
- Crc32.cs
- DiscoveryCallbackBehavior.cs
- SamlSecurityTokenAuthenticator.cs
- StringFreezingAttribute.cs
- EUCJPEncoding.cs
- FormClosingEvent.cs
- DataControlImageButton.cs
- SafeFindHandle.cs
- TraceEventCache.cs
- ExtractedStateEntry.cs
- MultilineStringConverter.cs
- ImageFormatConverter.cs
- CompiledXpathExpr.cs
- XpsSerializationManager.cs
- ColorAnimationUsingKeyFrames.cs
- Viewport3DVisual.cs
- PermissionAttributes.cs
- ServiceHost.cs
- WorkflowDefinitionDispenser.cs
- AccessControlList.cs
- NegotiateStream.cs
- UnsafeNativeMethods.cs
- XPathNavigatorReader.cs
- PrintPreviewGraphics.cs
- CodeTypeParameter.cs
- Inline.cs
- TagPrefixCollection.cs
- WebMethodAttribute.cs
- ProxyWebPartConnectionCollection.cs
- IPCCacheManager.cs
- FromRequest.cs
- DiagnosticTrace.cs
- BaseCollection.cs
- dataobject.cs
- WebPartZone.cs
- FragmentNavigationEventArgs.cs
- CryptoApi.cs
- MetadataArtifactLoaderCompositeFile.cs
- ColorConverter.cs
- DesignerAttribute.cs
- DispatcherSynchronizationContext.cs
- BindingContext.cs
- CustomWebEventKey.cs
- CodeVariableDeclarationStatement.cs
- _AcceptOverlappedAsyncResult.cs
- XsdCachingReader.cs
- X509PeerCertificateAuthenticationElement.cs
- MonitoringDescriptionAttribute.cs
- StringCollectionEditor.cs
- XmlSchemaElement.cs
- FileAuthorizationModule.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- externdll.cs
- ThicknessAnimation.cs
- CLRBindingWorker.cs
- _NestedSingleAsyncResult.cs
- XmlSerializationReader.cs
- SelectionUIService.cs
- FormViewInsertedEventArgs.cs
- ErrorHandlingAcceptor.cs
- MobileControlsSectionHelper.cs
- CutCopyPasteHelper.cs
- EncodingDataItem.cs
- ArgIterator.cs
- AnnotationMap.cs