Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / WrappedReader.cs / 1 / WrappedReader.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel { using System.IO; using System.Xml; using System.Text; using System.Diagnostics; using HexBinary = System.Runtime.Remoting.Metadata.W3cXsd2001.SoapHexBinary; sealed class WrappedReader : XmlDictionaryReader, IXmlLineInfo { readonly XmlDictionaryReader reader; readonly XmlTokenStream xmlTokens; MemoryStream contentStream; TextReader contentReader; bool recordDone; int depth; public WrappedReader(XmlDictionaryReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("reader")); } if (!reader.IsStartElement()) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.InnerReaderMustBeAtElement))); } this.xmlTokens = new XmlTokenStream(32); this.reader = reader; Record(); } public override string this[int index] { get { return this.reader[index]; } } public override string this[string name] { get { return this.reader[name]; } } public override string this[string name, string ns] { get { return this.reader[name, ns]; } } public override int AttributeCount { get { return this.reader.AttributeCount; } } public override string BaseURI { get { return this.reader.BaseURI; } } public override int Depth { get { return this.reader.Depth; } } public override bool EOF { get { return this.reader.EOF; } } public override bool HasValue { get { return this.reader.HasValue; } } public override bool IsDefault { get { return this.reader.IsDefault; } } public override bool IsEmptyElement { get { return this.reader.IsEmptyElement; } } public int LineNumber { get { IXmlLineInfo lineInfo = this.reader as IXmlLineInfo; if (lineInfo == null) { return 1; } return lineInfo.LineNumber; } } public int LinePosition { get { IXmlLineInfo lineInfo = this.reader as IXmlLineInfo; if (lineInfo == null) { return 1; } return lineInfo.LinePosition; } } public override string LocalName { get { return this.reader.LocalName; } } public override string Name { get { return this.reader.Name; } } public override string NamespaceURI { get { return this.reader.NamespaceURI; } } public override XmlNameTable NameTable { get { return this.reader.NameTable; } } public override XmlNodeType NodeType { get { return this.reader.NodeType; } } public override string Prefix { get { return this.reader.Prefix; } } public override char QuoteChar { get { return this.reader.QuoteChar; } } public override ReadState ReadState { get { return this.reader.ReadState; } } public override string Value { get { return this.reader.Value; } } public override Type ValueType { get { return this.reader.ValueType; } } public override string XmlLang { get { return this.reader.XmlLang; } } public override XmlSpace XmlSpace { get { return this.reader.XmlSpace; } } public XmlTokenStream XmlTokens { get { return this.xmlTokens; } } public override void Close() { OnEndOfContent(); this.reader.Close(); } public override string GetAttribute(int index) { return this.reader.GetAttribute(index); } public override string GetAttribute(string name) { return this.reader.GetAttribute(name); } public override string GetAttribute(string name, string ns) { return this.reader.GetAttribute(name, ns); } public bool HasLineInfo() { IXmlLineInfo lineInfo = this.reader as IXmlLineInfo; return lineInfo != null && lineInfo.HasLineInfo(); } public override string LookupNamespace(string ns) { return this.reader.LookupNamespace(ns); } public override void MoveToAttribute(int index) { OnEndOfContent(); this.reader.MoveToAttribute(index); } public override bool MoveToAttribute(string name) { OnEndOfContent(); return this.reader.MoveToAttribute(name); } public override bool MoveToAttribute(string name, string ns) { OnEndOfContent(); return this.reader.MoveToAttribute(name, ns); } public override bool MoveToElement() { OnEndOfContent(); return this.reader.MoveToElement(); } public override bool MoveToFirstAttribute() { OnEndOfContent(); return this.reader.MoveToFirstAttribute(); } public override bool MoveToNextAttribute() { OnEndOfContent(); return this.reader.MoveToNextAttribute(); } void OnEndOfContent() { if (this.contentReader != null) { this.contentReader.Close(); this.contentReader = null; } if (this.contentStream != null) { this.contentStream.Close(); this.contentStream = null; } } public override bool Read() { OnEndOfContent(); if (!this.reader.Read()) { return false; } if (!this.recordDone) { Record(); } return true; } public override bool ReadAttributeValue() { return this.reader.ReadAttributeValue(); } int ReadBinaryContent(byte[] buffer, int offset, int count, bool isBase64) { CryptoHelper.ValidateBufferBounds(buffer, offset, count); int read = 0; while (count > 0 && this.NodeType != XmlNodeType.Element && this.NodeType != XmlNodeType.EndElement) { if (this.contentStream == null) { byte[] value = isBase64 ? Convert.FromBase64String(this.Value) : HexBinary.Parse(this.Value).Value; this.contentStream = new MemoryStream(value); } int actual = this.contentStream.Read(buffer, offset, count); if (actual == 0) { if (this.NodeType == XmlNodeType.Attribute) { break; } if (!Read()) { break; } } read += actual; offset += actual; count -= actual; } return read; } public override int ReadContentAsBase64(byte[] buffer, int offset, int count) { return ReadBinaryContent(buffer, offset, count, true); } public override int ReadContentAsBinHex(byte[] buffer, int offset, int count) { return ReadBinaryContent(buffer, offset, count, false); } public override int ReadValueChunk(char[] chars, int offset, int count) { if (this.contentReader == null) { this.contentReader = new StringReader(this.Value); } return this.contentReader.Read(chars, offset, count); } void Record() { switch (this.NodeType) { case XmlNodeType.Element: bool isEmpty = this.reader.IsEmptyElement; this.xmlTokens.AddElement(this.reader.Prefix, this.reader.LocalName, this.reader.NamespaceURI, isEmpty); if (this.reader.MoveToFirstAttribute()) { do { this.xmlTokens.AddAttribute(this.reader.Prefix, this.reader.LocalName, this.reader.NamespaceURI, this.reader.Value); } while (this.reader.MoveToNextAttribute()); this.reader.MoveToElement(); } if (!isEmpty) { this.depth++; } else if (this.depth == 0) { this.recordDone = true; } break; case XmlNodeType.CDATA: case XmlNodeType.Comment: case XmlNodeType.Text: case XmlNodeType.EntityReference: case XmlNodeType.EndEntity: case XmlNodeType.SignificantWhitespace: case XmlNodeType.Whitespace: this.xmlTokens.Add(this.NodeType, this.Value); break; case XmlNodeType.EndElement: this.xmlTokens.Add(this.NodeType, this.Value); if (--this.depth == 0) { this.recordDone = true; } break; case XmlNodeType.DocumentType: case XmlNodeType.XmlDeclaration: break; default: throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR.GetString(SR.UnsupportedNodeTypeInReader, this.reader.NodeType, this.reader.Name))); } } public override void ResolveEntity() { this.reader.ResolveEntity(); } } sealed class XmlTokenStream : ISecurityElement { int position; int count; XmlTokenEntry[] entries; string excludedElement; string excludedElementNamespace; public XmlTokenStream(int initialSize) { if (initialSize < 1) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("initialSize", SR.GetString(SR.ValueMustBeGreaterThanZero))); } this.entries = new XmlTokenEntry[initialSize]; } public int Count { get { return this.count; } } public int Position { get { return this.position; } } public XmlNodeType NodeType { get { return this.entries[this.position].nodeType;} } public bool IsEmptyElement { get { return this.entries[this.position].IsEmptyElement;} } public string Prefix { get { return this.entries[this.position].prefix;} } public string LocalName { get { return this.entries[this.position].localName;} } public string NamespaceUri { get { return this.entries[this.position].namespaceUri;} } public string Value { get { return this.entries[this.position].Value;} } public string ExcludedElement { get { return this.excludedElement; } } public string ExcludedElementNamespace { get { return this.excludedElementNamespace; } } public void Add(XmlNodeType type, string value) { EnsureCapacityToAdd(); this.entries[this.count++].Set(type, value); } public void AddAttribute(string prefix, string localName, string namespaceUri, string value) { EnsureCapacityToAdd(); this.entries[this.count++].SetAttribute(prefix, localName, namespaceUri, value); } public void AddElement(string prefix, string localName, string namespaceUri, bool isEmptyElement) { EnsureCapacityToAdd(); this.entries[this.count++].SetElement(prefix, localName, namespaceUri, isEmptyElement); } void EnsureCapacityToAdd() { if (this.count == this.entries.Length) { XmlTokenEntry[] newBuffer = new XmlTokenEntry[this.entries.Length * 2]; Array.Copy(this.entries, 0, newBuffer, 0, this.count); this.entries = newBuffer; } } public bool MoveToFirst() { this.position = 0; return this.count > 0; } public bool MoveToFirstAttribute() { DiagnosticUtility.DebugAssert(this.NodeType == XmlNodeType.Element, ""); if (this.position < this.count - 1 && this.entries[this.position + 1].nodeType == XmlNodeType.Attribute) { this.position++; return true; } else { return false; } } public bool MoveToNext() { if (this.position < this.count - 1) { this.position++; return true; } return false; } public bool MoveToNextAttribute() { DiagnosticUtility.DebugAssert(this.NodeType == XmlNodeType.Attribute, ""); if (this.position < this.count - 1 && this.entries[this.position + 1].nodeType == XmlNodeType.Attribute) { this.position++; return true; } else { return false; } } public void SetElementExclusion(string excludedElement, string excludedElementNamespace) { this.excludedElement = excludedElement; this.excludedElementNamespace = excludedElementNamespace; } public void WriteTo(XmlDictionaryWriter writer, DictionaryManager dictionaryManager) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("writer")); } if (!MoveToFirst()) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.XmlTokenBufferIsEmpty))); } int depth = 0; int recordedDepth = -1; bool include = true; do { switch (this.NodeType) { case XmlNodeType.Element: bool isEmpty = this.IsEmptyElement; depth++; if (include && this.LocalName == this.excludedElement && this.NamespaceUri == this.excludedElementNamespace) { include = false; recordedDepth = depth; } if (include) { writer.WriteStartElement(this.Prefix, this.LocalName, this.NamespaceUri); } if (MoveToFirstAttribute()) { do { if (include) { writer.WriteAttributeString(this.Prefix, this.LocalName, this.NamespaceUri, this.Value); } } while (MoveToNextAttribute()); } if (isEmpty) { goto case XmlNodeType.EndElement; } break; case XmlNodeType.EndElement: if (include) { writer.WriteEndElement(); } else if (recordedDepth == depth) { include = true; recordedDepth = -1; } depth--; break; case XmlNodeType.CDATA: if (include) { writer.WriteCData(this.Value); } break; case XmlNodeType.Comment: if (include) { writer.WriteComment(this.Value); } break; case XmlNodeType.Text: if (include) { writer.WriteString(this.Value); } break; case XmlNodeType.SignificantWhitespace: case XmlNodeType.Whitespace: if (include) { writer.WriteWhitespace(this.Value); } break; case XmlNodeType.DocumentType: case XmlNodeType.XmlDeclaration: break; } } while (MoveToNext()); } bool ISecurityElement.HasId { get { return false; } } string ISecurityElement.Id { get { return null; } } struct XmlTokenEntry { internal XmlNodeType nodeType; internal string prefix; internal string localName; internal string namespaceUri; string value; public bool IsEmptyElement { get { return this.value == null; } set { this.value = value ? null : ""; } } public string Value { get { return this.value; } } public void Set(XmlNodeType nodeType, string value) { this.nodeType = nodeType; this.value = value; } public void SetAttribute(string prefix, string localName, string namespaceUri, string value) { this.nodeType = XmlNodeType.Attribute; this.prefix = prefix; this.localName = localName; this.namespaceUri = namespaceUri; this.value = value; } public void SetElement(string prefix, string localName, string namespaceUri, bool isEmptyElement) { this.nodeType = XmlNodeType.Element; this.prefix = prefix; this.localName = localName; this.namespaceUri = namespaceUri; this.IsEmptyElement = isEmptyElement; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
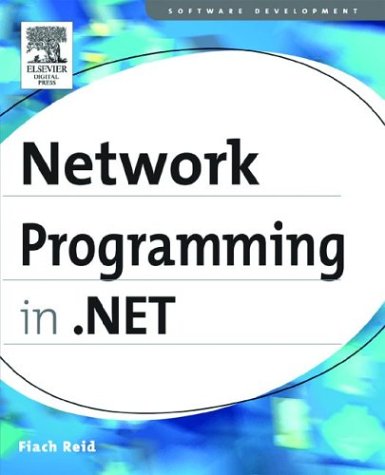
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BinaryMethodMessage.cs
- FixedTextPointer.cs
- Vertex.cs
- ColumnMap.cs
- MimeBasePart.cs
- FileDataSource.cs
- LinqDataSourceInsertEventArgs.cs
- ZoneIdentityPermission.cs
- TreeNodeStyleCollectionEditor.cs
- PolyQuadraticBezierSegment.cs
- DataObjectMethodAttribute.cs
- WaitHandle.cs
- TimeoutException.cs
- PersonalizationStateInfo.cs
- XmlNotation.cs
- HttpModulesSection.cs
- ModuleElement.cs
- OdbcHandle.cs
- CodeParameterDeclarationExpressionCollection.cs
- TrackingServices.cs
- WorkflowServiceHostFactory.cs
- WebPartConnectionsCloseVerb.cs
- ToolTip.cs
- ListBindableAttribute.cs
- UniqueEventHelper.cs
- DataGridViewComboBoxCell.cs
- FloatAverageAggregationOperator.cs
- RoutedUICommand.cs
- AuthenticationService.cs
- TraceInternal.cs
- DictionaryMarkupSerializer.cs
- HttpRawResponse.cs
- HostUtils.cs
- DataGridItemEventArgs.cs
- LoadGrammarCompletedEventArgs.cs
- OleDbSchemaGuid.cs
- PropertyNames.cs
- InputLanguageCollection.cs
- PropertyValueUIItem.cs
- _ProxyChain.cs
- Column.cs
- SQLStringStorage.cs
- XmlReader.cs
- PassportAuthenticationModule.cs
- FontStretch.cs
- ClosableStream.cs
- GeneralTransform3DTo2DTo3D.cs
- SchemaImporterExtension.cs
- AutoGeneratedFieldProperties.cs
- WindowInteropHelper.cs
- XsltLoader.cs
- SectionRecord.cs
- BehaviorDragDropEventArgs.cs
- DataServiceRequestArgs.cs
- HttpPostedFileWrapper.cs
- PolicyManager.cs
- DBNull.cs
- ListControlConvertEventArgs.cs
- HttpCookie.cs
- WebPartCollection.cs
- FrameworkElement.cs
- Slider.cs
- ActiveDocumentEvent.cs
- OracleFactory.cs
- PrintControllerWithStatusDialog.cs
- OracleMonthSpan.cs
- OrderedHashRepartitionStream.cs
- Graphics.cs
- PageResolution.cs
- WasAdminWrapper.cs
- BufferManager.cs
- RandomNumberGenerator.cs
- XmlQueryRuntime.cs
- StylusEventArgs.cs
- ErasingStroke.cs
- SharedDp.cs
- SupportsEventValidationAttribute.cs
- ColorTranslator.cs
- PathGeometry.cs
- CryptoApi.cs
- SmiGettersStream.cs
- Property.cs
- TextRenderer.cs
- TransformerInfoCollection.cs
- RowType.cs
- PointConverter.cs
- UnitySerializationHolder.cs
- SqlGenericUtil.cs
- GridEntry.cs
- WindowsUpDown.cs
- DiscoveryClientRequestChannel.cs
- ViewLoader.cs
- XPathPatternBuilder.cs
- ControlDesignerState.cs
- ListViewItem.cs
- UIElementPropertyUndoUnit.cs
- objectresult_tresulttype.cs
- CellPartitioner.cs
- SimpleHandlerBuildProvider.cs
- RSACryptoServiceProvider.cs