Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CommonUI / System / Drawing / Size.cs / 2 / Size.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System; using System.IO; using Microsoft.Win32; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Globalization; /** * Represents a dimension in 2D coordinate space */ ////// /// Represents the size of a rectangular region /// with an ordered pair of width and height. /// [ TypeConverterAttribute(typeof(SizeConverter)), ] [Serializable] [System.Runtime.InteropServices.ComVisible(true)] [SuppressMessage("Microsoft.Usage", "CA2225:OperatorOverloadsHaveNamedAlternates")] public struct Size { ////// /// Initializes a new instance of the public static readonly Size Empty = new Size(); private int width; private int height; /** * Create a new Size object from a point */ ///class. /// /// /// public Size(Point pt) { width = pt.X; height = pt.Y; } /** * Create a new Size object of the specified dimension */ ////// Initializes a new instance of the ///class from /// the specified . /// /// /// Initializes a new instance of the public Size(int width, int height) { this.width = width; this.height = height; } ///class from /// the specified dimensions. /// /// /// Converts the specified public static implicit operator SizeF(Size p) { return new SizeF(p.Width, p.Height); } ///to a /// . /// /// /// public static Size operator +(Size sz1, Size sz2) { return Add(sz1, sz2); } ////// Performs vector addition of two ///objects. /// /// /// public static Size operator -(Size sz1, Size sz2) { return Subtract(sz1, sz2); } ////// Contracts a ///by another /// . /// /// /// Tests whether two public static bool operator ==(Size sz1, Size sz2) { return sz1.Width == sz2.Width && sz1.Height == sz2.Height; } ///objects /// are identical. /// /// /// public static bool operator !=(Size sz1, Size sz2) { return !(sz1 == sz2); } ////// Tests whether two ///objects are different. /// /// /// Converts the specified public static explicit operator Point(Size size) { return new Point(size.Width, size.Height); } ///to a /// . /// /// /// Tests whether this [Browsable(false)] public bool IsEmpty { get { return width == 0 && height == 0; } } /** * Horizontal dimension */ ///has zero /// width and height. /// /// /// public int Width { get { return width; } set { width = value; } } /** * Vertical dimension */ ////// Represents the horizontal component of this /// ///. /// /// /// Represents the vertical component of this /// public int Height { get { return height; } set { height = value; } } ///. /// /// public static Size Add(Size sz1, Size sz2) { return new Size(sz1.Width + sz2.Width, sz1.Height + sz2.Height); } ////// Performs vector addition of two ///objects. /// /// /// Converts a SizeF to a Size by performing a ceiling operation on /// all the coordinates. /// public static Size Ceiling(SizeF value) { return new Size((int)Math.Ceiling(value.Width), (int)Math.Ceiling(value.Height)); } ////// public static Size Subtract(Size sz1, Size sz2) { return new Size(sz1.Width - sz2.Width, sz1.Height - sz2.Height); } ////// Contracts a ///by another . /// /// /// Converts a SizeF to a Size by performing a truncate operation on /// all the coordinates. /// public static Size Truncate(SizeF value) { return new Size((int)value.Width, (int)value.Height); } ////// /// Converts a SizeF to a Size by performing a round operation on /// all the coordinates. /// public static Size Round(SizeF value) { return new Size((int)Math.Round(value.Width), (int)Math.Round(value.Height)); } ////// /// public override bool Equals(object obj) { if (!(obj is Size)) return false; Size comp = (Size)obj; // Note value types can't have derived classes, so we don't need to // check the types of the objects here. -- [....], 2/21/2001 return (comp.width == this.width) && (comp.height == this.height); } ////// Tests to see whether the specified object is a /// ////// with the same dimensions as this . /// /// /// public override int GetHashCode() { return width ^ height; } ////// Returns a hash code. /// ////// /// public override string ToString() { return "{Width=" + width.ToString(CultureInfo.CurrentCulture) + ", Height=" + height.ToString(CultureInfo.CurrentCulture) + "}"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Creates a human-readable string that represents this /// ///. /// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System; using System.IO; using Microsoft.Win32; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Globalization; /** * Represents a dimension in 2D coordinate space */ ////// /// Represents the size of a rectangular region /// with an ordered pair of width and height. /// [ TypeConverterAttribute(typeof(SizeConverter)), ] [Serializable] [System.Runtime.InteropServices.ComVisible(true)] [SuppressMessage("Microsoft.Usage", "CA2225:OperatorOverloadsHaveNamedAlternates")] public struct Size { ////// /// Initializes a new instance of the public static readonly Size Empty = new Size(); private int width; private int height; /** * Create a new Size object from a point */ ///class. /// /// /// public Size(Point pt) { width = pt.X; height = pt.Y; } /** * Create a new Size object of the specified dimension */ ////// Initializes a new instance of the ///class from /// the specified . /// /// /// Initializes a new instance of the public Size(int width, int height) { this.width = width; this.height = height; } ///class from /// the specified dimensions. /// /// /// Converts the specified public static implicit operator SizeF(Size p) { return new SizeF(p.Width, p.Height); } ///to a /// . /// /// /// public static Size operator +(Size sz1, Size sz2) { return Add(sz1, sz2); } ////// Performs vector addition of two ///objects. /// /// /// public static Size operator -(Size sz1, Size sz2) { return Subtract(sz1, sz2); } ////// Contracts a ///by another /// . /// /// /// Tests whether two public static bool operator ==(Size sz1, Size sz2) { return sz1.Width == sz2.Width && sz1.Height == sz2.Height; } ///objects /// are identical. /// /// /// public static bool operator !=(Size sz1, Size sz2) { return !(sz1 == sz2); } ////// Tests whether two ///objects are different. /// /// /// Converts the specified public static explicit operator Point(Size size) { return new Point(size.Width, size.Height); } ///to a /// . /// /// /// Tests whether this [Browsable(false)] public bool IsEmpty { get { return width == 0 && height == 0; } } /** * Horizontal dimension */ ///has zero /// width and height. /// /// /// public int Width { get { return width; } set { width = value; } } /** * Vertical dimension */ ////// Represents the horizontal component of this /// ///. /// /// /// Represents the vertical component of this /// public int Height { get { return height; } set { height = value; } } ///. /// /// public static Size Add(Size sz1, Size sz2) { return new Size(sz1.Width + sz2.Width, sz1.Height + sz2.Height); } ////// Performs vector addition of two ///objects. /// /// /// Converts a SizeF to a Size by performing a ceiling operation on /// all the coordinates. /// public static Size Ceiling(SizeF value) { return new Size((int)Math.Ceiling(value.Width), (int)Math.Ceiling(value.Height)); } ////// public static Size Subtract(Size sz1, Size sz2) { return new Size(sz1.Width - sz2.Width, sz1.Height - sz2.Height); } ////// Contracts a ///by another . /// /// /// Converts a SizeF to a Size by performing a truncate operation on /// all the coordinates. /// public static Size Truncate(SizeF value) { return new Size((int)value.Width, (int)value.Height); } ////// /// Converts a SizeF to a Size by performing a round operation on /// all the coordinates. /// public static Size Round(SizeF value) { return new Size((int)Math.Round(value.Width), (int)Math.Round(value.Height)); } ////// /// public override bool Equals(object obj) { if (!(obj is Size)) return false; Size comp = (Size)obj; // Note value types can't have derived classes, so we don't need to // check the types of the objects here. -- [....], 2/21/2001 return (comp.width == this.width) && (comp.height == this.height); } ////// Tests to see whether the specified object is a /// ////// with the same dimensions as this . /// /// /// public override int GetHashCode() { return width ^ height; } ////// Returns a hash code. /// ////// /// public override string ToString() { return "{Width=" + width.ToString(CultureInfo.CurrentCulture) + ", Height=" + height.ToString(CultureInfo.CurrentCulture) + "}"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Creates a human-readable string that represents this /// ///. ///
Link Menu
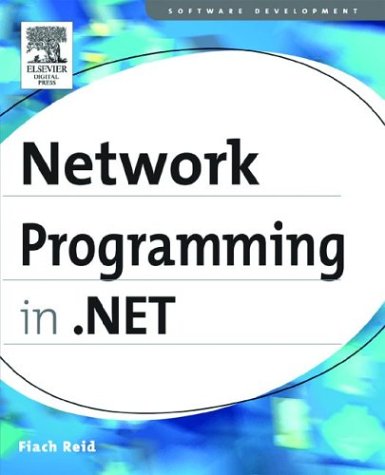
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- __TransparentProxy.cs
- ResponseStream.cs
- Context.cs
- HttpContextServiceHost.cs
- Timer.cs
- ClientBuildManager.cs
- RouteValueExpressionBuilder.cs
- MonitoringDescriptionAttribute.cs
- InputMethodStateChangeEventArgs.cs
- mongolianshape.cs
- SimpleFieldTemplateFactory.cs
- WriteTimeStream.cs
- MultiAsyncResult.cs
- Function.cs
- AuthorizationSection.cs
- SignatureToken.cs
- ZoneLinkButton.cs
- recordstatescratchpad.cs
- SchemaNamespaceManager.cs
- UTF8Encoding.cs
- HelpKeywordAttribute.cs
- WebServiceReceive.cs
- MappingModelBuildProvider.cs
- ExtensibleClassFactory.cs
- KeyedCollection.cs
- PackWebRequestFactory.cs
- CommandEventArgs.cs
- regiisutil.cs
- RequestContext.cs
- Transform.cs
- Evidence.cs
- KeyFrames.cs
- DesignerMetadata.cs
- OutputCacheSection.cs
- CodePageEncoding.cs
- ColumnResult.cs
- File.cs
- Compress.cs
- AuthenticationModuleElement.cs
- DesignerDataSchemaClass.cs
- ExpressionsCollectionConverter.cs
- Application.cs
- ConfigurationManagerInternalFactory.cs
- translator.cs
- BitmapEffectState.cs
- XmlConvert.cs
- SoapIncludeAttribute.cs
- CurrencyManager.cs
- DataControlButton.cs
- DataGridSortingEventArgs.cs
- wgx_commands.cs
- SqlUDTStorage.cs
- COM2AboutBoxPropertyDescriptor.cs
- FixedSOMTextRun.cs
- XmlSchemaCollection.cs
- httpserverutility.cs
- RoutedEventValueSerializer.cs
- UnsafeNetInfoNativeMethods.cs
- DifferencingCollection.cs
- NumericPagerField.cs
- base64Transforms.cs
- Mapping.cs
- Stackframe.cs
- HttpModulesSection.cs
- SecureStringHasher.cs
- BehaviorService.cs
- DataGridColumn.cs
- WebBrowserDocumentCompletedEventHandler.cs
- GridViewHeaderRowPresenter.cs
- DataRecordInfo.cs
- codemethodreferenceexpression.cs
- Thickness.cs
- SafeNativeMethods.cs
- KernelTypeValidation.cs
- WorkerRequest.cs
- WsatConfiguration.cs
- PowerStatus.cs
- LogRecordSequence.cs
- TcpClientSocketManager.cs
- CompiledRegexRunnerFactory.cs
- Binding.cs
- XsdBuilder.cs
- AuthorizationRule.cs
- ConnectionManagementElement.cs
- NonParentingControl.cs
- ImpersonationContext.cs
- AssemblyCache.cs
- Stack.cs
- WindowsGrip.cs
- templategroup.cs
- Set.cs
- TypeTypeConverter.cs
- CacheMode.cs
- Pkcs7Recipient.cs
- OleDbTransaction.cs
- ObjectKeyFrameCollection.cs
- RawStylusInputCustomDataList.cs
- QilGeneratorEnv.cs
- UniqueConstraint.cs
- ContextStaticAttribute.cs