Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / MDIWindowDialog.cs / 1 / MDIWindowDialog.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.ComponentModel; using System.Diagnostics; using System; using System.Drawing; using System.Windows.Forms; using Microsoft.Win32; ////// /// ///[ System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode) ] internal sealed class MdiWindowDialog : Form { private System.Windows.Forms.ListBox itemList; private System.Windows.Forms.Button okButton; private System.Windows.Forms.Button cancelButton; private System.Windows.Forms.TableLayoutPanel okCancelTableLayoutPanel; Form active; public MdiWindowDialog() : base() { InitializeComponent(); } public Form ActiveChildForm { get { #if DEBUG ListItem item = (ListItem)itemList.SelectedItem; Debug.Assert(item != null, "No item selected!"); #endif return active; } } /// /// /// private class ListItem { public Form form; public ListItem(Form f) { form = f; } public override string ToString() { return form.Text; } } public void SetItems(Form active, Form[] all) { int selIndex = 0; for (int i=0; i/// /// NOTE: The following code is required by the Windows Forms /// designer. It can be modified using the form editor. Do not /// modify it using the code editor. /// private void InitializeComponent() { System.ComponentModel.ComponentResourceManager resources = new System.ComponentModel.ComponentResourceManager(typeof(MdiWindowDialog)); this.itemList = new System.Windows.Forms.ListBox(); this.okButton = new System.Windows.Forms.Button(); this.cancelButton = new System.Windows.Forms.Button(); this.okCancelTableLayoutPanel = new System.Windows.Forms.TableLayoutPanel(); this.okCancelTableLayoutPanel.SuspendLayout(); this.itemList.DoubleClick += new System.EventHandler(this.ItemList_doubleClick); this.itemList.SelectedIndexChanged += new EventHandler(this.ItemList_selectedIndexChanged); this.SuspendLayout(); // // itemList // resources.ApplyResources(this.itemList, "itemList"); this.itemList.FormattingEnabled = true; this.itemList.Name = "itemList"; // // okButton // resources.ApplyResources(this.okButton, "okButton"); this.okButton.DialogResult = System.Windows.Forms.DialogResult.OK; this.okButton.Margin = new System.Windows.Forms.Padding(0, 0, 3, 0); this.okButton.Name = "okButton"; // // cancelButton // resources.ApplyResources(this.cancelButton, "cancelButton"); this.cancelButton.DialogResult = System.Windows.Forms.DialogResult.Cancel; this.cancelButton.Margin = new System.Windows.Forms.Padding(3, 0, 0, 0); this.cancelButton.Name = "cancelButton"; // // okCancelTableLayoutPanel // resources.ApplyResources(this.okCancelTableLayoutPanel, "okCancelTableLayoutPanel"); this.okCancelTableLayoutPanel.ColumnCount = 2; this.okCancelTableLayoutPanel.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Percent, 50F)); this.okCancelTableLayoutPanel.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Percent, 50F)); this.okCancelTableLayoutPanel.Controls.Add(this.okButton, 0, 0); this.okCancelTableLayoutPanel.Controls.Add(this.cancelButton, 1, 0); this.okCancelTableLayoutPanel.Name = "okCancelTableLayoutPanel"; this.okCancelTableLayoutPanel.RowCount = 1; this.okCancelTableLayoutPanel.RowStyles.Add(new System.Windows.Forms.RowStyle()); // // MdiWindowDialog // resources.ApplyResources(this, "$this"); this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font; this.Controls.Add(this.okCancelTableLayoutPanel); this.Controls.Add(this.itemList); this.MaximizeBox = false; this.MinimizeBox = false; this.Name = "MdiWindowDialog"; this.ShowIcon = false; this.okCancelTableLayoutPanel.ResumeLayout(false); this.okCancelTableLayoutPanel.PerformLayout(); this.AcceptButton = this.okButton; this.CancelButton = this.cancelButton; this.ResumeLayout(false); this.PerformLayout(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.ComponentModel; using System.Diagnostics; using System; using System.Drawing; using System.Windows.Forms; using Microsoft.Win32; ////// /// ///[ System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode) ] internal sealed class MdiWindowDialog : Form { private System.Windows.Forms.ListBox itemList; private System.Windows.Forms.Button okButton; private System.Windows.Forms.Button cancelButton; private System.Windows.Forms.TableLayoutPanel okCancelTableLayoutPanel; Form active; public MdiWindowDialog() : base() { InitializeComponent(); } public Form ActiveChildForm { get { #if DEBUG ListItem item = (ListItem)itemList.SelectedItem; Debug.Assert(item != null, "No item selected!"); #endif return active; } } /// /// /// private class ListItem { public Form form; public ListItem(Form f) { form = f; } public override string ToString() { return form.Text; } } public void SetItems(Form active, Form[] all) { int selIndex = 0; for (int i=0; i/// /// NOTE: The following code is required by the Windows Forms /// designer. It can be modified using the form editor. Do not /// modify it using the code editor. /// private void InitializeComponent() { System.ComponentModel.ComponentResourceManager resources = new System.ComponentModel.ComponentResourceManager(typeof(MdiWindowDialog)); this.itemList = new System.Windows.Forms.ListBox(); this.okButton = new System.Windows.Forms.Button(); this.cancelButton = new System.Windows.Forms.Button(); this.okCancelTableLayoutPanel = new System.Windows.Forms.TableLayoutPanel(); this.okCancelTableLayoutPanel.SuspendLayout(); this.itemList.DoubleClick += new System.EventHandler(this.ItemList_doubleClick); this.itemList.SelectedIndexChanged += new EventHandler(this.ItemList_selectedIndexChanged); this.SuspendLayout(); // // itemList // resources.ApplyResources(this.itemList, "itemList"); this.itemList.FormattingEnabled = true; this.itemList.Name = "itemList"; // // okButton // resources.ApplyResources(this.okButton, "okButton"); this.okButton.DialogResult = System.Windows.Forms.DialogResult.OK; this.okButton.Margin = new System.Windows.Forms.Padding(0, 0, 3, 0); this.okButton.Name = "okButton"; // // cancelButton // resources.ApplyResources(this.cancelButton, "cancelButton"); this.cancelButton.DialogResult = System.Windows.Forms.DialogResult.Cancel; this.cancelButton.Margin = new System.Windows.Forms.Padding(3, 0, 0, 0); this.cancelButton.Name = "cancelButton"; // // okCancelTableLayoutPanel // resources.ApplyResources(this.okCancelTableLayoutPanel, "okCancelTableLayoutPanel"); this.okCancelTableLayoutPanel.ColumnCount = 2; this.okCancelTableLayoutPanel.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Percent, 50F)); this.okCancelTableLayoutPanel.ColumnStyles.Add(new System.Windows.Forms.ColumnStyle(System.Windows.Forms.SizeType.Percent, 50F)); this.okCancelTableLayoutPanel.Controls.Add(this.okButton, 0, 0); this.okCancelTableLayoutPanel.Controls.Add(this.cancelButton, 1, 0); this.okCancelTableLayoutPanel.Name = "okCancelTableLayoutPanel"; this.okCancelTableLayoutPanel.RowCount = 1; this.okCancelTableLayoutPanel.RowStyles.Add(new System.Windows.Forms.RowStyle()); // // MdiWindowDialog // resources.ApplyResources(this, "$this"); this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font; this.Controls.Add(this.okCancelTableLayoutPanel); this.Controls.Add(this.itemList); this.MaximizeBox = false; this.MinimizeBox = false; this.Name = "MdiWindowDialog"; this.ShowIcon = false; this.okCancelTableLayoutPanel.ResumeLayout(false); this.okCancelTableLayoutPanel.PerformLayout(); this.AcceptButton = this.okButton; this.CancelButton = this.cancelButton; this.ResumeLayout(false); this.PerformLayout(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
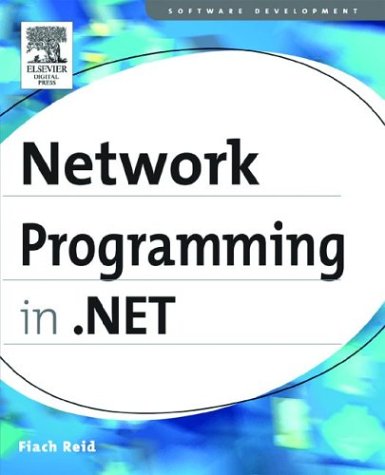
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThreadSafeList.cs
- OracleTransaction.cs
- SqlUserDefinedTypeAttribute.cs
- XmlText.cs
- ConnectionProviderAttribute.cs
- SelectionProcessor.cs
- AutoCompleteStringCollection.cs
- SafeNativeMethodsOther.cs
- TextDecoration.cs
- PieceNameHelper.cs
- DefaultMemberAttribute.cs
- ConstraintManager.cs
- SetterBase.cs
- ErasingStroke.cs
- ProfileGroupSettings.cs
- CustomWebEventKey.cs
- Ticks.cs
- HeaderedContentControl.cs
- RegexParser.cs
- ParagraphVisual.cs
- ZoneButton.cs
- Wizard.cs
- TimeSpanStorage.cs
- DuplicateWaitObjectException.cs
- BulletChrome.cs
- IDQuery.cs
- SiteMapNodeCollection.cs
- MarshalDirectiveException.cs
- UnknownWrapper.cs
- CodeCompiler.cs
- ListViewItemEventArgs.cs
- ScriptControl.cs
- GlobalProxySelection.cs
- RTLAwareMessageBox.cs
- ExceptionUtil.cs
- CollectionChangeEventArgs.cs
- Light.cs
- _FtpDataStream.cs
- TraceUtils.cs
- EntityType.cs
- GenericsNotImplementedException.cs
- PermissionToken.cs
- TextCollapsingProperties.cs
- PipelineModuleStepContainer.cs
- BinaryReader.cs
- ConnectionManagementElementCollection.cs
- StrokeCollectionConverter.cs
- FieldToken.cs
- TextCharacters.cs
- GenerateHelper.cs
- StaticFileHandler.cs
- CreateParams.cs
- ChtmlCalendarAdapter.cs
- XsltContext.cs
- DirectoryNotFoundException.cs
- StorageEndPropertyMapping.cs
- InputLangChangeRequestEvent.cs
- DebuggerAttributes.cs
- Subtree.cs
- DataControlReferenceCollection.cs
- InlineCollection.cs
- ClientRuntimeConfig.cs
- MediaCommands.cs
- ExpressionContext.cs
- XamlPoint3DCollectionSerializer.cs
- SafeCoTaskMem.cs
- ConfigurationSettings.cs
- StringValidator.cs
- DiscoveryOperationContextExtension.cs
- TabPageDesigner.cs
- CellRelation.cs
- StackOverflowException.cs
- ContentElementAutomationPeer.cs
- HotSpotCollectionEditor.cs
- WindowsFormsSynchronizationContext.cs
- EntityProxyFactory.cs
- SqlBulkCopyColumnMappingCollection.cs
- SqlDataSourceTableQuery.cs
- ValidationResult.cs
- XmlSchemaImporter.cs
- SendActivity.cs
- CompilerTypeWithParams.cs
- Error.cs
- AuthorizationRuleCollection.cs
- TimeEnumHelper.cs
- UnsignedPublishLicense.cs
- Point3DConverter.cs
- DropShadowBitmapEffect.cs
- CodeDomSerializer.cs
- ProtocolsConfiguration.cs
- ThreadInterruptedException.cs
- OptimisticConcurrencyException.cs
- EditCommandColumn.cs
- safelink.cs
- InvalidCastException.cs
- SqlPersonalizationProvider.cs
- PackagePartCollection.cs
- CachingHintValidation.cs
- WebHeaderCollection.cs
- MatrixTransform3D.cs