Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / System / Windows / Vector.cs / 1 / Vector.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001, 2002 // // File: Vector.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Text; using System.Collections; using System.Globalization; using System.Windows; using System.Windows.Media; using System.Runtime.InteropServices; namespace System.Windows { ////// Vector - A value type which defined a vector in terms of X and Y /// public partial struct Vector { #region Constructors ////// Constructor which sets the vector's initial values /// /// double - The initial X /// double - THe initial Y public Vector(double x, double y) { _x = x; _y = y; } #endregion Constructors #region Public Methods ////// Length Property - the length of this Vector /// public double Length { get { return Math.Sqrt(_x*_x + _y*_y); } } ////// LengthSquared Property - the squared length of this Vector /// public double LengthSquared { get { return _x*_x + _y*_y; } } ////// Normalize - Updates this Vector to maintain its direction, but to have a length /// of 1. This is equivalent to dividing this Vector by Length /// public void Normalize() { // Avoid overflow this /= Math.Max(Math.Abs(_x),Math.Abs(_y)); this /= Length; } ////// CrossProduct - Returns the cross product: vector1.X*vector2.Y - vector1.Y*vector2.X /// ////// Returns the cross product: vector1.X*vector2.Y - vector1.Y*vector2.X /// /// The first Vector /// The second Vector public static double CrossProduct(Vector vector1, Vector vector2) { return vector1._x * vector2._y - vector1._y * vector2._x; } ////// AngleBetween - the angle between 2 vectors /// ////// Returns the the angle in degrees between vector1 and vector2 /// /// The first Vector /// The second Vector public static double AngleBetween(Vector vector1, Vector vector2) { double sin = vector1._x * vector2._y - vector2._x * vector1._y; double cos = vector1._x * vector2._x + vector1._y * vector2._y; return Math.Atan2(sin, cos) * (180 / Math.PI); } #endregion Public Methods #region Public Operators ////// Operator -Vector (unary negation) /// public static Vector operator - (Vector vector) { return new Vector(-vector._x,-vector._y); } ////// Negates the values of X and Y on this Vector /// public void Negate() { _x = -_x; _y = -_y; } ////// Operator Vector + Vector /// public static Vector operator + (Vector vector1, Vector vector2) { return new Vector(vector1._x + vector2._x, vector1._y + vector2._y); } ////// Add: Vector + Vector /// public static Vector Add(Vector vector1, Vector vector2) { return new Vector(vector1._x + vector2._x, vector1._y + vector2._y); } ////// Operator Vector - Vector /// public static Vector operator - (Vector vector1, Vector vector2) { return new Vector(vector1._x - vector2._x, vector1._y - vector2._y); } ////// Subtract: Vector - Vector /// public static Vector Subtract(Vector vector1, Vector vector2) { return new Vector(vector1._x - vector2._x, vector1._y - vector2._y); } ////// Operator Vector + Point /// public static Point operator + (Vector vector, Point point) { return new Point(point._x + vector._x, point._y + vector._y); } ////// Add: Vector + Point /// public static Point Add(Vector vector, Point point) { return new Point(point._x + vector._x, point._y + vector._y); } ////// Operator Vector * double /// public static Vector operator * (Vector vector, double scalar) { return new Vector(vector._x * scalar, vector._y * scalar); } ////// Multiply: Vector * double /// public static Vector Multiply(Vector vector, double scalar) { return new Vector(vector._x * scalar, vector._y * scalar); } ////// Operator double * Vector /// public static Vector operator * (double scalar, Vector vector) { return new Vector(vector._x * scalar, vector._y * scalar); } ////// Multiply: double * Vector /// public static Vector Multiply(double scalar, Vector vector) { return new Vector(vector._x * scalar, vector._y * scalar); } ////// Operator Vector / double /// public static Vector operator / (Vector vector, double scalar) { return vector * (1.0 / scalar); } ////// Multiply: Vector / double /// public static Vector Divide(Vector vector, double scalar) { return vector * (1.0 / scalar); } ////// Operator Vector * Matrix /// public static Vector operator * (Vector vector, Matrix matrix) { return matrix.Transform(vector); } ////// Multiply: Vector * Matrix /// public static Vector Multiply(Vector vector, Matrix matrix) { return matrix.Transform(vector); } ////// Operator Vector * Vector, interpreted as their dot product /// public static double operator * (Vector vector1, Vector vector2) { return vector1._x * vector2._x + vector1._y * vector2._y; } ////// Multiply - Returns the dot product: vector1.X*vector2.X + vector1.Y*vector2.Y /// ////// Returns the dot product: vector1.X*vector2.X + vector1.Y*vector2.Y /// /// The first Vector /// The second Vector public static double Multiply(Vector vector1, Vector vector2) { return vector1._x * vector2._x + vector1._y * vector2._y; } ////// Determinant - Returns the determinant det(vector1, vector2) /// ////// Returns the determinant: vector1.X*vector2.Y - vector1.Y*vector2.X /// /// The first Vector /// The second Vector public static double Determinant(Vector vector1, Vector vector2) { return vector1._x * vector2._y - vector1._y * vector2._x; } ////// Explicit conversion to Size. Note that since Size cannot contain negative values, /// the resulting size will contains the absolute values of X and Y /// ////// Size - A Size equal to this Vector /// /// Vector - the Vector to convert to a Size public static explicit operator Size(Vector vector) { return new Size(Math.Abs(vector._x), Math.Abs(vector._y)); } ////// Explicit conversion to Point /// ////// Point - A Point equal to this Vector /// /// Vector - the Vector to convert to a Point public static explicit operator Point(Vector vector) { return new Point(vector._x, vector._y); } #endregion Public Operators } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001, 2002 // // File: Vector.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Text; using System.Collections; using System.Globalization; using System.Windows; using System.Windows.Media; using System.Runtime.InteropServices; namespace System.Windows { ////// Vector - A value type which defined a vector in terms of X and Y /// public partial struct Vector { #region Constructors ////// Constructor which sets the vector's initial values /// /// double - The initial X /// double - THe initial Y public Vector(double x, double y) { _x = x; _y = y; } #endregion Constructors #region Public Methods ////// Length Property - the length of this Vector /// public double Length { get { return Math.Sqrt(_x*_x + _y*_y); } } ////// LengthSquared Property - the squared length of this Vector /// public double LengthSquared { get { return _x*_x + _y*_y; } } ////// Normalize - Updates this Vector to maintain its direction, but to have a length /// of 1. This is equivalent to dividing this Vector by Length /// public void Normalize() { // Avoid overflow this /= Math.Max(Math.Abs(_x),Math.Abs(_y)); this /= Length; } ////// CrossProduct - Returns the cross product: vector1.X*vector2.Y - vector1.Y*vector2.X /// ////// Returns the cross product: vector1.X*vector2.Y - vector1.Y*vector2.X /// /// The first Vector /// The second Vector public static double CrossProduct(Vector vector1, Vector vector2) { return vector1._x * vector2._y - vector1._y * vector2._x; } ////// AngleBetween - the angle between 2 vectors /// ////// Returns the the angle in degrees between vector1 and vector2 /// /// The first Vector /// The second Vector public static double AngleBetween(Vector vector1, Vector vector2) { double sin = vector1._x * vector2._y - vector2._x * vector1._y; double cos = vector1._x * vector2._x + vector1._y * vector2._y; return Math.Atan2(sin, cos) * (180 / Math.PI); } #endregion Public Methods #region Public Operators ////// Operator -Vector (unary negation) /// public static Vector operator - (Vector vector) { return new Vector(-vector._x,-vector._y); } ////// Negates the values of X and Y on this Vector /// public void Negate() { _x = -_x; _y = -_y; } ////// Operator Vector + Vector /// public static Vector operator + (Vector vector1, Vector vector2) { return new Vector(vector1._x + vector2._x, vector1._y + vector2._y); } ////// Add: Vector + Vector /// public static Vector Add(Vector vector1, Vector vector2) { return new Vector(vector1._x + vector2._x, vector1._y + vector2._y); } ////// Operator Vector - Vector /// public static Vector operator - (Vector vector1, Vector vector2) { return new Vector(vector1._x - vector2._x, vector1._y - vector2._y); } ////// Subtract: Vector - Vector /// public static Vector Subtract(Vector vector1, Vector vector2) { return new Vector(vector1._x - vector2._x, vector1._y - vector2._y); } ////// Operator Vector + Point /// public static Point operator + (Vector vector, Point point) { return new Point(point._x + vector._x, point._y + vector._y); } ////// Add: Vector + Point /// public static Point Add(Vector vector, Point point) { return new Point(point._x + vector._x, point._y + vector._y); } ////// Operator Vector * double /// public static Vector operator * (Vector vector, double scalar) { return new Vector(vector._x * scalar, vector._y * scalar); } ////// Multiply: Vector * double /// public static Vector Multiply(Vector vector, double scalar) { return new Vector(vector._x * scalar, vector._y * scalar); } ////// Operator double * Vector /// public static Vector operator * (double scalar, Vector vector) { return new Vector(vector._x * scalar, vector._y * scalar); } ////// Multiply: double * Vector /// public static Vector Multiply(double scalar, Vector vector) { return new Vector(vector._x * scalar, vector._y * scalar); } ////// Operator Vector / double /// public static Vector operator / (Vector vector, double scalar) { return vector * (1.0 / scalar); } ////// Multiply: Vector / double /// public static Vector Divide(Vector vector, double scalar) { return vector * (1.0 / scalar); } ////// Operator Vector * Matrix /// public static Vector operator * (Vector vector, Matrix matrix) { return matrix.Transform(vector); } ////// Multiply: Vector * Matrix /// public static Vector Multiply(Vector vector, Matrix matrix) { return matrix.Transform(vector); } ////// Operator Vector * Vector, interpreted as their dot product /// public static double operator * (Vector vector1, Vector vector2) { return vector1._x * vector2._x + vector1._y * vector2._y; } ////// Multiply - Returns the dot product: vector1.X*vector2.X + vector1.Y*vector2.Y /// ////// Returns the dot product: vector1.X*vector2.X + vector1.Y*vector2.Y /// /// The first Vector /// The second Vector public static double Multiply(Vector vector1, Vector vector2) { return vector1._x * vector2._x + vector1._y * vector2._y; } ////// Determinant - Returns the determinant det(vector1, vector2) /// ////// Returns the determinant: vector1.X*vector2.Y - vector1.Y*vector2.X /// /// The first Vector /// The second Vector public static double Determinant(Vector vector1, Vector vector2) { return vector1._x * vector2._y - vector1._y * vector2._x; } ////// Explicit conversion to Size. Note that since Size cannot contain negative values, /// the resulting size will contains the absolute values of X and Y /// ////// Size - A Size equal to this Vector /// /// Vector - the Vector to convert to a Size public static explicit operator Size(Vector vector) { return new Size(Math.Abs(vector._x), Math.Abs(vector._y)); } ////// Explicit conversion to Point /// ////// Point - A Point equal to this Vector /// /// Vector - the Vector to convert to a Point public static explicit operator Point(Vector vector) { return new Point(vector._x, vector._y); } #endregion Public Operators } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
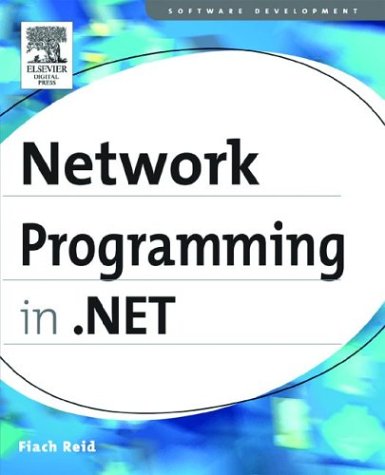
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeRemoveEventStatement.cs
- TargetControlTypeAttribute.cs
- FocusManager.cs
- MinMaxParagraphWidth.cs
- ToolBar.cs
- SQLBinary.cs
- XmlSchemaExporter.cs
- EntityDataSourceWrapperCollection.cs
- WorkflowRuntimeServiceElement.cs
- XmlSchemaSet.cs
- GuidConverter.cs
- AttributeTableBuilder.cs
- DataServiceStreamProviderWrapper.cs
- BitmapEffectInput.cs
- WebEventCodes.cs
- Application.cs
- HttpAsyncResult.cs
- Delegate.cs
- KerberosTicketHashIdentifierClause.cs
- VisualCollection.cs
- DataGridAutomationPeer.cs
- TableLayoutColumnStyleCollection.cs
- RequestCachingSection.cs
- ZipFileInfo.cs
- SessionPageStatePersister.cs
- ChannelToken.cs
- HttpCapabilitiesEvaluator.cs
- DoubleAnimationUsingPath.cs
- CfgSemanticTag.cs
- Descriptor.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- RuntimeHelpers.cs
- MethodBody.cs
- HtmlTitle.cs
- EntityCommandDefinition.cs
- HistoryEventArgs.cs
- ItemMap.cs
- BitmapEditor.cs
- NetSectionGroup.cs
- ArrayElementGridEntry.cs
- SqlGenerator.cs
- AtlasWeb.Designer.cs
- PrintDocument.cs
- RoutedEventHandlerInfo.cs
- CodeEntryPointMethod.cs
- PasswordRecoveryAutoFormat.cs
- TogglePatternIdentifiers.cs
- DXD.cs
- SizeChangedEventArgs.cs
- DataGridColumnCollection.cs
- CodeDomExtensionMethods.cs
- HttpVersion.cs
- ValidationErrorInfo.cs
- SingleResultAttribute.cs
- StaticTextPointer.cs
- ThreadExceptionDialog.cs
- ReflectionPermission.cs
- InputScopeAttribute.cs
- WebServiceClientProxyGenerator.cs
- EntityDataSourceWrapperCollection.cs
- NetCodeGroup.cs
- DecoderFallbackWithFailureFlag.cs
- DataGridPagingPage.cs
- ResXResourceReader.cs
- UnSafeCharBuffer.cs
- ControlBuilderAttribute.cs
- TextComposition.cs
- UnsafeNativeMethodsPenimc.cs
- MailFileEditor.cs
- ItemCollection.cs
- InstanceStore.cs
- WeakHashtable.cs
- CFStream.cs
- Translator.cs
- ResXDataNode.cs
- ImageIndexConverter.cs
- EnumValAlphaComparer.cs
- CodeDOMUtility.cs
- SoapFault.cs
- ComponentFactoryHelpers.cs
- streamingZipPartStream.cs
- FormViewCommandEventArgs.cs
- XmlSchemas.cs
- TimeEnumHelper.cs
- PathFigureCollection.cs
- LongValidator.cs
- BamlResourceSerializer.cs
- ActivityBindForm.Designer.cs
- StandardOleMarshalObject.cs
- EntitySetBaseCollection.cs
- SingleAnimationUsingKeyFrames.cs
- CollectionContainer.cs
- QilParameter.cs
- ProviderUtil.cs
- DbModificationClause.cs
- XmlDeclaration.cs
- ApplicationFileCodeDomTreeGenerator.cs
- Animatable.cs
- SpeechUI.cs
- BuildProviderUtils.cs