Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / ImportRequest.cs / 1 / ImportRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections; using System.Security.Principal; using System.Diagnostics; using System.Threading; //ManualResetEvent using System.ComponentModel; //Win32Exception using System.IO; //Stream using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using Microsoft.InfoCards.Diagnostics; // // This class is an instance of a request to import a card from a file. // It starts up the UI in the 'Import' mode. // class ImportRequest : ClientUIRequest { FileStream m_importFile; //keep a read pointer to the file string m_filename; public ImportRequest( Process callingProcess, WindowsIdentity callingIdentity, InfoCardUIAgent uiAgent, IntPtr rpcHandle, Stream inArgs, Stream outArgs ) : base( callingProcess, callingIdentity, uiAgent, rpcHandle, inArgs, outArgs, InfoCardUIAgent.CallMode.Import, ExceptionList.AllNonFatal ) { } public string ImportedFile { get{ return m_filename; } } // // No input args // protected override void OnMarshalInArgs() { BinaryReader breader = new InfoCardBinaryReader( InArgs, Encoding.Unicode ); string filename = Utility.DeserializeString( breader ); if (String.IsNullOrEmpty(filename) || filename.Length > Constants.Maxima.FileNameLength) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFileName ) ) ); } if( !Path.IsPathRooted( filename ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFileName) ) ); } try { m_filename = filename; m_importFile = new FileStream( m_filename, FileMode.Open, FileAccess.Read, FileShare.Read ); } catch( ArgumentException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.CannotOpenImportFile ), e ) ); } catch( IOException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.CannotOpenImportFile ), e ) ); } catch( NotSupportedException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.CannotOpenImportFile ), e ) ); } catch( UnauthorizedAccessException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.CannotOpenImportFile ), e ) ); } } protected override void OnProcess() { // // ClientUiRequest's member function // StartAndWaitForUIAgent(); } // // No Output args. // protected override void OnMarshalOutArgs() { } protected override void OnDisposeAsUser() { base.OnDisposeAsUser(); if( null != m_importFile ) { m_importFile.Dispose(); m_importFile = null; } } // // Summary // Gets called a) if there was an exception in client processing // or b) if there was an exception in the child's request processing // protected override bool OnHandleException( Exception e, out int errorCode ) { errorCode = 0; bool handled = false; if( e is UserCancelledException ) { errorCode = (e as UserCancelledException).NativeHResult; handled = true; } return handled; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
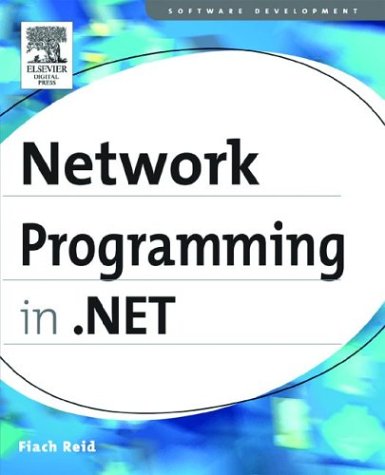
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlStatistics.cs
- NavigateEvent.cs
- RuntimeArgumentHandle.cs
- RadioButton.cs
- TitleStyle.cs
- ModuleConfigurationInfo.cs
- Page.cs
- PointCollection.cs
- ListViewEditEventArgs.cs
- AdjustableArrowCap.cs
- StringUtil.cs
- SystemIcons.cs
- TransactionChannel.cs
- CrossAppDomainChannel.cs
- Menu.cs
- SqlUserDefinedAggregateAttribute.cs
- EmptyQuery.cs
- CodeTypeReferenceExpression.cs
- FileIOPermission.cs
- OverlappedAsyncResult.cs
- SoapEnumAttribute.cs
- HttpModuleAction.cs
- FieldToken.cs
- InertiaExpansionBehavior.cs
- ProxyElement.cs
- BitmapCodecInfoInternal.cs
- PixelFormats.cs
- TypeLoadException.cs
- SecurityHelper.cs
- XmlQualifiedNameTest.cs
- XmlKeywords.cs
- DrawingContext.cs
- StylusButtonEventArgs.cs
- WindowsGraphics.cs
- Model3DGroup.cs
- ParseNumbers.cs
- TextContainerChangedEventArgs.cs
- SqlTriggerAttribute.cs
- SafeFindHandle.cs
- ConditionCollection.cs
- PanelDesigner.cs
- PointKeyFrameCollection.cs
- TableTextElementCollectionInternal.cs
- OutputCacheProfile.cs
- XPathQilFactory.cs
- DbConnectionHelper.cs
- DeviceSpecificChoice.cs
- SecurityElement.cs
- HttpListener.cs
- ParserOptions.cs
- HttpHandlersSection.cs
- CheckBox.cs
- ProofTokenCryptoHandle.cs
- ListComponentEditor.cs
- BasicHttpBinding.cs
- TemplateControlParser.cs
- PointHitTestParameters.cs
- RootContext.cs
- DbProviderManifest.cs
- PackageRelationshipCollection.cs
- SmiSettersStream.cs
- LinqDataSourceView.cs
- XmlCharCheckingWriter.cs
- Base64Stream.cs
- basevalidator.cs
- ListItemConverter.cs
- _SslStream.cs
- XmlWriterSettings.cs
- StylusCaptureWithinProperty.cs
- ObjectTag.cs
- PathSegment.cs
- SQLBytes.cs
- GC.cs
- XComponentModel.cs
- AccessControlEntry.cs
- SlipBehavior.cs
- CodeDirectoryCompiler.cs
- DataGridViewCellStyle.cs
- ContextMenuAutomationPeer.cs
- DataStorage.cs
- SharedStatics.cs
- QueryOpeningEnumerator.cs
- AuthStoreRoleProvider.cs
- ObjectListDataBindEventArgs.cs
- RepeaterCommandEventArgs.cs
- DataGridViewSelectedColumnCollection.cs
- XmlSchemaAnnotation.cs
- FloaterParagraph.cs
- StorageFunctionMapping.cs
- InterleavedZipPartStream.cs
- Utils.cs
- DynamicQueryStringParameter.cs
- BinarySerializer.cs
- TextFindEngine.cs
- DataServiceEntityAttribute.cs
- DialogWindow.cs
- SizeChangedEventArgs.cs
- AmbientProperties.cs
- PinProtectionHelper.cs
- ProxyGenerator.cs