Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewSelectedColumnCollection.cs / 1305376 / DataGridViewSelectedColumnCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Collections; using System.Windows.Forms; using System.ComponentModel; using System.Globalization; using System.Diagnostics.CodeAnalysis; ///[ ListBindable(false), SuppressMessage("Microsoft.Design", "CA1010:CollectionsShouldImplementGenericInterface") // Consider adding an IList implementation ] public class DataGridViewSelectedColumnCollection : BaseCollection, IList { ArrayList items = new ArrayList(); /// /// int IList.Add(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.Contains(object value) { return this.items.Contains(value); } /// /// int IList.IndexOf(object value) { return this.items.IndexOf(value); } /// /// void IList.Insert(int index, object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Remove(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.RemoveAt(int index) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.IsFixedSize { get { return true; } } /// /// bool IList.IsReadOnly { get { return true; } } /// /// object IList.this[int index] { get { return this.items[index]; } set { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } /// /// void ICollection.CopyTo(Array array, int index) { this.items.CopyTo(array, index); } /// /// int ICollection.Count { get { return this.items.Count; } } /// /// bool ICollection.IsSynchronized { get { return false; } } /// /// object ICollection.SyncRoot { get { return this; } } /// /// IEnumerator IEnumerable.GetEnumerator() { return this.items.GetEnumerator(); } internal DataGridViewSelectedColumnCollection() { } /// protected override ArrayList List { get { return this.items; } } /// public DataGridViewColumn this[int index] { get { return (DataGridViewColumn) this.items[index]; } } /// /// /// internal int Add(DataGridViewColumn dataGridViewColumn) { return this.items.Add(dataGridViewColumn); } /* Unused at this point internal void AddRange(DataGridViewColumn[] dataGridViewColumns) { Debug.Assert(dataGridViewColumns != null); foreach(DataGridViewColumn dataGridViewColumn in dataGridViewColumns) { this.items.Add(dataGridViewColumn); } } */ /* Unused at this point internal void AddColumnCollection(DataGridViewColumnCollection dataGridViewColumns) { Debug.Assert(dataGridViewColumns != null); foreach(DataGridViewColumn dataGridViewColumn in dataGridViewColumns) { this.items.Add(dataGridViewColumn); } } */ ///Adds a ///to this collection. [ EditorBrowsable(EditorBrowsableState.Never) ] public void Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// /// Checks to see if a DataGridViewCell is contained in this collection. /// public bool Contains(DataGridViewColumn dataGridViewColumn) { return this.items.IndexOf(dataGridViewColumn) != -1; } ///public void CopyTo(DataGridViewColumn[] array, int index) { this.items.CopyTo(array, index); } /// [ EditorBrowsable(EditorBrowsableState.Never), SuppressMessage("Microsoft.Performance", "CA1801:AvoidUnusedParameters") ] public void Insert(int index, DataGridViewColumn dataGridViewColumn) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Collections; using System.Windows.Forms; using System.ComponentModel; using System.Globalization; using System.Diagnostics.CodeAnalysis; ///[ ListBindable(false), SuppressMessage("Microsoft.Design", "CA1010:CollectionsShouldImplementGenericInterface") // Consider adding an IList implementation ] public class DataGridViewSelectedColumnCollection : BaseCollection, IList { ArrayList items = new ArrayList(); /// /// int IList.Add(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.Contains(object value) { return this.items.Contains(value); } /// /// int IList.IndexOf(object value) { return this.items.IndexOf(value); } /// /// void IList.Insert(int index, object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.Remove(object value) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// void IList.RemoveAt(int index) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// bool IList.IsFixedSize { get { return true; } } /// /// bool IList.IsReadOnly { get { return true; } } /// /// object IList.this[int index] { get { return this.items[index]; } set { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } /// /// void ICollection.CopyTo(Array array, int index) { this.items.CopyTo(array, index); } /// /// int ICollection.Count { get { return this.items.Count; } } /// /// bool ICollection.IsSynchronized { get { return false; } } /// /// object ICollection.SyncRoot { get { return this; } } /// /// IEnumerator IEnumerable.GetEnumerator() { return this.items.GetEnumerator(); } internal DataGridViewSelectedColumnCollection() { } /// protected override ArrayList List { get { return this.items; } } /// public DataGridViewColumn this[int index] { get { return (DataGridViewColumn) this.items[index]; } } /// /// /// internal int Add(DataGridViewColumn dataGridViewColumn) { return this.items.Add(dataGridViewColumn); } /* Unused at this point internal void AddRange(DataGridViewColumn[] dataGridViewColumns) { Debug.Assert(dataGridViewColumns != null); foreach(DataGridViewColumn dataGridViewColumn in dataGridViewColumns) { this.items.Add(dataGridViewColumn); } } */ /* Unused at this point internal void AddColumnCollection(DataGridViewColumnCollection dataGridViewColumns) { Debug.Assert(dataGridViewColumns != null); foreach(DataGridViewColumn dataGridViewColumn in dataGridViewColumns) { this.items.Add(dataGridViewColumn); } } */ ///Adds a ///to this collection. [ EditorBrowsable(EditorBrowsableState.Never) ] public void Clear() { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } /// /// /// Checks to see if a DataGridViewCell is contained in this collection. /// public bool Contains(DataGridViewColumn dataGridViewColumn) { return this.items.IndexOf(dataGridViewColumn) != -1; } ///public void CopyTo(DataGridViewColumn[] array, int index) { this.items.CopyTo(array, index); } /// [ EditorBrowsable(EditorBrowsableState.Never), SuppressMessage("Microsoft.Performance", "CA1801:AvoidUnusedParameters") ] public void Insert(int index, DataGridViewColumn dataGridViewColumn) { throw new NotSupportedException(SR.GetString(SR.DataGridView_ReadOnlyCollection)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
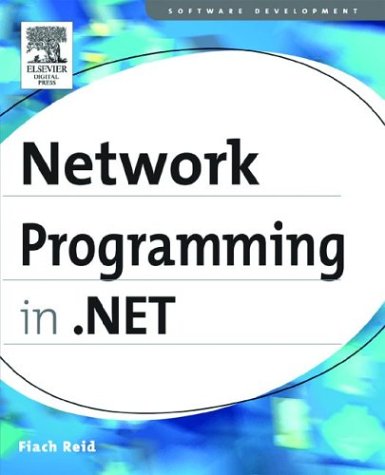
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InstanceDataCollection.cs
- DrawToolTipEventArgs.cs
- MouseDevice.cs
- HotSpotCollection.cs
- XmlLinkedNode.cs
- NativeMethods.cs
- CryptoConfig.cs
- CultureInfoConverter.cs
- SaveWorkflowCommand.cs
- SpeechSynthesizer.cs
- RoleService.cs
- TableLayoutStyle.cs
- TypedTableGenerator.cs
- CFGGrammar.cs
- SecuritySessionSecurityTokenAuthenticator.cs
- Error.cs
- StatusBar.cs
- CheckPair.cs
- CqlParserHelpers.cs
- RootProfilePropertySettingsCollection.cs
- RadioButtonBaseAdapter.cs
- EnumValidator.cs
- QilCloneVisitor.cs
- VirtualDirectoryMappingCollection.cs
- PageMediaType.cs
- DefaultValidator.cs
- ThicknessKeyFrameCollection.cs
- PerfCounterSection.cs
- Msec.cs
- OleDbMetaDataFactory.cs
- SymmetricAlgorithm.cs
- CqlGenerator.cs
- XmlBoundElement.cs
- ComplexTypeEmitter.cs
- ChoiceConverter.cs
- DataKey.cs
- ExceptionUtility.cs
- smtppermission.cs
- updatecommandorderer.cs
- _DigestClient.cs
- SettingsAttributes.cs
- DrawingAttributeSerializer.cs
- DataGridrowEditEndingEventArgs.cs
- LiteralControl.cs
- TextEffectCollection.cs
- EntityStoreSchemaFilterEntry.cs
- ProfileSection.cs
- ShapeTypeface.cs
- shaper.cs
- ProfileElement.cs
- EntityDataSourceMemberPath.cs
- arabicshape.cs
- MetadataSource.cs
- MapPathBasedVirtualPathProvider.cs
- StrokeCollection2.cs
- CrossContextChannel.cs
- TextDecorationCollection.cs
- ScriptingWebServicesSectionGroup.cs
- QueryableDataSource.cs
- AdornerDecorator.cs
- AssertUtility.cs
- WindowsFormsSynchronizationContext.cs
- LinqDataSourceDisposeEventArgs.cs
- Semaphore.cs
- CollectionBuilder.cs
- ExceptionHandler.cs
- Identity.cs
- CommandManager.cs
- AttachedPropertyMethodSelector.cs
- SerializationFieldInfo.cs
- EventManager.cs
- mda.cs
- MaterialGroup.cs
- OrderedDictionaryStateHelper.cs
- Token.cs
- XmlLanguage.cs
- TypeBuilder.cs
- ReaderOutput.cs
- DockPanel.cs
- TagPrefixCollection.cs
- SettingsSection.cs
- AnnotationService.cs
- HuffCodec.cs
- EncoderFallback.cs
- Scripts.cs
- MailBnfHelper.cs
- SoapAttributes.cs
- ConnectionManagementSection.cs
- Int32RectConverter.cs
- AlternateViewCollection.cs
- HttpModuleAction.cs
- DataGridViewColumnConverter.cs
- HandlerBase.cs
- MDIControlStrip.cs
- rsa.cs
- SafeNativeMethods.cs
- ResourceManager.cs
- GraphicsPath.cs
- FontStyle.cs
- CompilerCollection.cs