Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / Configuration / HttpModuleAction.cs / 1 / HttpModuleAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Configuration; using System.Web.Configuration.Common; using System.Web.Util; using System.Globalization; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpModuleAction : ConfigurationElement { private static readonly ConfigurationElementProperty s_elemProperty = new ConfigurationElementProperty(new CallbackValidator(typeof(HttpModuleAction), Validate)); private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propName = new ConfigurationProperty("name", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propType = new ConfigurationProperty("type", typeof(string), String.Empty, ConfigurationPropertyOptions.IsRequired); private ModulesEntry _modualEntry; static HttpModuleAction() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propName); _properties.Add(_propType); } internal HttpModuleAction() { } public HttpModuleAction(String name, String type) : this() { Name = name; Type = type; _modualEntry = null; } internal string Key { get { return Name; } } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("name", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Name { get { return (string)base[_propName]; } set { base[_propName] = value; } } [ConfigurationProperty("type", IsRequired = true, DefaultValue = "")] public string Type { get { return (string)base[_propType]; } set { base[_propType] = value; } } internal string FileName { get { return ElementInformation.Properties["name"].Source; } } internal int LineNumber { get { return ElementInformation.Properties["name"].LineNumber; } } internal ModulesEntry Entry { get { try { if (_modualEntry == null) { _modualEntry = new ModulesEntry(Name, Type, _propType.Name, this); } return _modualEntry; } catch (Exception ex) { throw new ConfigurationErrorsException(ex.Message, ElementInformation.Properties[_propType.Name].Source, ElementInformation.Properties[_propType.Name].LineNumber); } } } internal static bool IsSpecialModule(String className) { return ModulesEntry.IsTypeMatch(typeof(System.Web.Security.DefaultAuthenticationModule), className); } internal static bool IsSpecialModuleName(String name) { return (StringUtil.EqualsIgnoreCase(name, "DefaultAuthentication")); } protected override ConfigurationElementProperty ElementProperty { get { return s_elemProperty; } } private static void Validate(object value) { if (value == null) { throw new ArgumentNullException("httpModule"); } HttpModuleAction elem = (HttpModuleAction)value; if (HttpModuleAction.IsSpecialModule(elem.Type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Special_module_cannot_be_added_manually, elem.Type), elem.ElementInformation.Properties["type"].Source, elem.ElementInformation.Properties["type"].LineNumber); } if (HttpModuleAction.IsSpecialModuleName(elem.Name)) { throw new ConfigurationErrorsException( SR.GetString(SR.Special_module_cannot_be_added_manually, elem.Name), elem.ElementInformation.Properties["name"].Source, elem.ElementInformation.Properties["name"].LineNumber); } } } // class HttpModule } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Configuration; using System.Web.Configuration.Common; using System.Web.Util; using System.Globalization; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpModuleAction : ConfigurationElement { private static readonly ConfigurationElementProperty s_elemProperty = new ConfigurationElementProperty(new CallbackValidator(typeof(HttpModuleAction), Validate)); private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propName = new ConfigurationProperty("name", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propType = new ConfigurationProperty("type", typeof(string), String.Empty, ConfigurationPropertyOptions.IsRequired); private ModulesEntry _modualEntry; static HttpModuleAction() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propName); _properties.Add(_propType); } internal HttpModuleAction() { } public HttpModuleAction(String name, String type) : this() { Name = name; Type = type; _modualEntry = null; } internal string Key { get { return Name; } } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("name", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Name { get { return (string)base[_propName]; } set { base[_propName] = value; } } [ConfigurationProperty("type", IsRequired = true, DefaultValue = "")] public string Type { get { return (string)base[_propType]; } set { base[_propType] = value; } } internal string FileName { get { return ElementInformation.Properties["name"].Source; } } internal int LineNumber { get { return ElementInformation.Properties["name"].LineNumber; } } internal ModulesEntry Entry { get { try { if (_modualEntry == null) { _modualEntry = new ModulesEntry(Name, Type, _propType.Name, this); } return _modualEntry; } catch (Exception ex) { throw new ConfigurationErrorsException(ex.Message, ElementInformation.Properties[_propType.Name].Source, ElementInformation.Properties[_propType.Name].LineNumber); } } } internal static bool IsSpecialModule(String className) { return ModulesEntry.IsTypeMatch(typeof(System.Web.Security.DefaultAuthenticationModule), className); } internal static bool IsSpecialModuleName(String name) { return (StringUtil.EqualsIgnoreCase(name, "DefaultAuthentication")); } protected override ConfigurationElementProperty ElementProperty { get { return s_elemProperty; } } private static void Validate(object value) { if (value == null) { throw new ArgumentNullException("httpModule"); } HttpModuleAction elem = (HttpModuleAction)value; if (HttpModuleAction.IsSpecialModule(elem.Type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Special_module_cannot_be_added_manually, elem.Type), elem.ElementInformation.Properties["type"].Source, elem.ElementInformation.Properties["type"].LineNumber); } if (HttpModuleAction.IsSpecialModuleName(elem.Name)) { throw new ConfigurationErrorsException( SR.GetString(SR.Special_module_cannot_be_added_manually, elem.Name), elem.ElementInformation.Properties["name"].Source, elem.ElementInformation.Properties["name"].LineNumber); } } } // class HttpModule } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
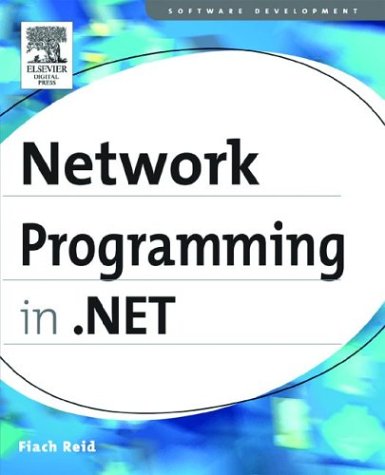
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlMetaData.cs
- FullTextBreakpoint.cs
- SHA384CryptoServiceProvider.cs
- UserPersonalizationStateInfo.cs
- LineProperties.cs
- Directory.cs
- Font.cs
- ArrayExtension.cs
- SoapExtension.cs
- ConstructorBuilder.cs
- TreeView.cs
- ToolStripDropDownItem.cs
- AccessDataSourceView.cs
- EncryptedPackageFilter.cs
- CloudCollection.cs
- XmlFormatExtensionPrefixAttribute.cs
- ParallelTimeline.cs
- ScopelessEnumAttribute.cs
- SourceElementsCollection.cs
- PageHandlerFactory.cs
- NotImplementedException.cs
- HttpMethodConstraint.cs
- ServerValidateEventArgs.cs
- HostingEnvironment.cs
- AuthorizationSection.cs
- WebBrowser.cs
- HtmlInputImage.cs
- SecurityException.cs
- CollectionChangeEventArgs.cs
- CodeMethodReturnStatement.cs
- ContainerUtilities.cs
- XmlStreamStore.cs
- SystemIPInterfaceStatistics.cs
- TypeSystem.cs
- SchemaName.cs
- MarkupObject.cs
- GridViewRowEventArgs.cs
- CodeTypeOfExpression.cs
- TraceEventCache.cs
- HostingEnvironmentSection.cs
- Convert.cs
- EUCJPEncoding.cs
- RightsManagementEncryptedStream.cs
- ByteStream.cs
- ComboBox.cs
- XmlBoundElement.cs
- SqlFacetAttribute.cs
- PointHitTestResult.cs
- BaseComponentEditor.cs
- safemediahandle.cs
- TreeView.cs
- JsonFaultDetail.cs
- _BasicClient.cs
- BitmapFrame.cs
- Filter.cs
- LoopExpression.cs
- ByteRangeDownloader.cs
- AssociationSetEnd.cs
- TemplateBindingExpression.cs
- PasswordRecovery.cs
- KeyInfo.cs
- EdmType.cs
- _SslStream.cs
- LogicalExpressionTypeConverter.cs
- ReachSerializer.cs
- HtmlToClrEventProxy.cs
- XmlQueryOutput.cs
- NumberFormatter.cs
- Formatter.cs
- ValueCollectionParameterReader.cs
- ElementHostAutomationPeer.cs
- InheritanceRules.cs
- ProfilePropertySettingsCollection.cs
- UdpDuplexChannel.cs
- Hash.cs
- ColorContext.cs
- loginstatus.cs
- PointCollection.cs
- ColumnBinding.cs
- ManagedFilter.cs
- HttpSessionStateWrapper.cs
- ControlUtil.cs
- LayoutInformation.cs
- mediapermission.cs
- TraceSource.cs
- ObfuscateAssemblyAttribute.cs
- DesignOnlyAttribute.cs
- handlecollector.cs
- RectIndependentAnimationStorage.cs
- PropertyDescriptor.cs
- ConsumerConnectionPoint.cs
- TypeConverterHelper.cs
- RoutingExtension.cs
- DbConnectionPoolOptions.cs
- RegexTree.cs
- GridViewHeaderRowPresenter.cs
- WebPartUserCapability.cs
- TypedTableGenerator.cs
- DesignerGenericWebPart.cs
- ReadWriteControlDesigner.cs