Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / SMDiagnostics / System / ServiceModel / Diagnostics / ExceptionUtility.cs / 1305376 / ExceptionUtility.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Diagnostics { using System; using System.Diagnostics; using System.Runtime; using System.Runtime.ConstrainedExecution; using System.Runtime.Diagnostics; using System.Runtime.InteropServices; using System.Threading; using System.Runtime.Serialization; using System.Reflection; using System.Runtime.CompilerServices; using System.Collections; class ExceptionUtility { const string ExceptionStackAsStringKey = "System.ServiceModel.Diagnostics.ExceptionUtility.ExceptionStackAsString"; // This field should be only used for debug build. internal static ExceptionUtility mainInstance; DiagnosticTrace diagnosticTrace; string name; string eventSourceName; [ThreadStatic] static Guid activityId; [ThreadStatic] static bool useStaticActivityId; [Obsolete("For SMDiagnostics.dll use only. Call DiagnosticUtility.ExceptionUtility instead")] internal ExceptionUtility(string name, string eventSourceName, object diagnosticTrace) { this.diagnosticTrace = (DiagnosticTrace)diagnosticTrace; this.name = name; this.eventSourceName = eventSourceName; } [Obsolete("For SMDiagnostics.dll use only. Call DiagnosticUtility.ExceptionUtility instead")] [MethodImpl(MethodImplOptions.NoInlining)] #pragma warning disable 56500 internal void TraceFailFast(string message) { EventLogger logger = null; try { #pragma warning disable 618 logger = new EventLogger(this.eventSourceName, this.diagnosticTrace); #pragma warning restore 618 } finally { #pragma warning disable 618 TraceFailFast(message, logger); #pragma warning restore 618 } } // Fail-- Event Log entry will be generated. // To force a Watson on a dev machine, do the following: // 1. Set \HKLM\SOFTWARE\Microsoft\PCHealth\ErrorReporting ForceQueueMode = 0 // 2. In the command environment, set COMPLUS_DbgJitDebugLaunchSetting=0 [Obsolete("For SMDiagnostics.dll use only. Call DiagnosticUtility.ExceptionUtility instead")] [MethodImpl(MethodImplOptions.NoInlining)] internal static void TraceFailFast(string message, EventLogger logger) { try { if (logger != null) { string stackTrace = null; try { stackTrace = new StackTrace().ToString(); } catch (Exception exception) { stackTrace = exception.Message; } finally { logger.LogEvent(TraceEventType.Critical, EventLogCategory.FailFast, EventLogEventId.FailFast, message, stackTrace); } } } catch (Exception e) { if (logger != null) { logger.LogEvent(TraceEventType.Critical, EventLogCategory.FailFast, EventLogEventId.FailFastException, e.ToString()); } throw; } } #pragma warning restore 56500 [Obsolete("For SMDiagnostics.dll use only. Call DiagnosticUtility.ExceptionUtility instead")] internal void TraceFailFastException(Exception exception) { TraceFailFast(exception == null ? null : exception.ToString()); } internal Exception ThrowHelper(Exception exception, TraceEventType eventType, TraceRecord extendedData) { #pragma warning disable 618 bool shouldTrace = (this.diagnosticTrace != null && this.diagnosticTrace.ShouldTrace(eventType)); #pragma warning restore 618 if (shouldTrace) { using (ExceptionUtility.useStaticActivityId ? Activity.CreateActivity(ExceptionUtility.activityId) : null) { this.diagnosticTrace.TraceEvent(eventType, DiagnosticsTraceCode.ThrowingException, DiagnosticTrace.GenerateMsdnTraceCode("System.ServiceModel.Diagnostics", "ThrowingException"), TraceSR.GetString(TraceSR.ThrowingException), extendedData, exception, null); } } #if DEBUG string stack = exception.StackTrace; #else // Avoid doing this for perf reasons unless we're already taking the hit for tracing. string stack = (shouldTrace ? exception.StackTrace : null); #endif if (!string.IsNullOrEmpty(stack)) { IDictionary data = exception.Data; if (data != null && !data.IsReadOnly && !data.IsFixedSize) { object existingString = data[ExceptionStackAsStringKey]; string stackString = existingString == null ? "" : existingString as string; if (stackString != null) { stackString = string.Concat(stackString, stackString.Length == 0 ? "" : Environment.NewLine, "throw", Environment.NewLine, stack, Environment.NewLine, "catch", Environment.NewLine); data[ExceptionStackAsStringKey] = stackString; } } } return exception; } internal Exception ThrowHelper(Exception exception, TraceEventType eventType) { return this.ThrowHelper(exception, eventType, null); } internal ArgumentException ThrowHelperArgument(string message) { return (ArgumentException)this.ThrowHelperError(new ArgumentException(message)); } internal ArgumentException ThrowHelperArgument(string paramName, string message) { return (ArgumentException)this.ThrowHelperError(new ArgumentException(message, paramName)); } internal ArgumentNullException ThrowHelperArgumentNull(string paramName) { return (ArgumentNullException)this.ThrowHelperError(new ArgumentNullException(paramName)); } internal ArgumentNullException ThrowHelperArgumentNull(string paramName, string message) { return (ArgumentNullException)this.ThrowHelperError(new ArgumentNullException(paramName, message)); } internal Exception ThrowHelperFatal(string message, Exception innerException) { return this.ThrowHelperError(new FatalException(message, innerException)); } internal Exception ThrowHelperInternal(bool fatal) { return fatal ? Fx.AssertAndThrowFatal("Fatal InternalException should never be thrown.") : Fx.AssertAndThrow("InternalException should never be thrown."); } internal Exception ThrowHelperCallback(string message, Exception innerException) { return this.ThrowHelperCritical(new CallbackException(message, innerException)); } internal Exception ThrowHelperCallback(Exception innerException) { return this.ThrowHelperCallback(TraceSR.GetString(TraceSR.GenericCallbackException), innerException); } internal Exception ThrowHelperCritical(Exception exception) { return this.ThrowHelper(exception, TraceEventType.Critical); } internal Exception ThrowHelperError(Exception exception) { return this.ThrowHelper(exception, TraceEventType.Error); } internal Exception ThrowHelperWarning(Exception exception) { return this.ThrowHelper(exception, TraceEventType.Warning); } internal void TraceHandledException(Exception exception, TraceEventType eventType) { #pragma warning disable 618 bool shouldTrace = (this.diagnosticTrace != null && this.diagnosticTrace.ShouldTrace(eventType)); #pragma warning restore 618 if (shouldTrace) { using (ExceptionUtility.useStaticActivityId ? Activity.CreateActivity(ExceptionUtility.activityId) : null) { this.diagnosticTrace.TraceEvent(eventType, DiagnosticsTraceCode.TraceHandledException, DiagnosticTrace.GenerateMsdnTraceCode("System.ServiceModel.Diagnostics", "TraceHandledException"), TraceSR.GetString(TraceSR.TraceHandledException), null, exception, null); } } } // On a single thread, these functions will complete just fine // and don't need to worry about locking issues because the effected // variables are ThreadStatic. internal static void UseActivityId(Guid activityId) { ExceptionUtility.activityId = activityId; ExceptionUtility.useStaticActivityId = true; } internal static void ClearActivityId() { ExceptionUtility.useStaticActivityId = false; ExceptionUtility.activityId = Guid.Empty; } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static bool IsInfrastructureException(Exception exception) { return exception != null && (exception is ThreadAbortException || exception is AppDomainUnloadedException); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Diagnostics { using System; using System.Diagnostics; using System.Runtime; using System.Runtime.ConstrainedExecution; using System.Runtime.Diagnostics; using System.Runtime.InteropServices; using System.Threading; using System.Runtime.Serialization; using System.Reflection; using System.Runtime.CompilerServices; using System.Collections; class ExceptionUtility { const string ExceptionStackAsStringKey = "System.ServiceModel.Diagnostics.ExceptionUtility.ExceptionStackAsString"; // This field should be only used for debug build. internal static ExceptionUtility mainInstance; DiagnosticTrace diagnosticTrace; string name; string eventSourceName; [ThreadStatic] static Guid activityId; [ThreadStatic] static bool useStaticActivityId; [Obsolete("For SMDiagnostics.dll use only. Call DiagnosticUtility.ExceptionUtility instead")] internal ExceptionUtility(string name, string eventSourceName, object diagnosticTrace) { this.diagnosticTrace = (DiagnosticTrace)diagnosticTrace; this.name = name; this.eventSourceName = eventSourceName; } [Obsolete("For SMDiagnostics.dll use only. Call DiagnosticUtility.ExceptionUtility instead")] [MethodImpl(MethodImplOptions.NoInlining)] #pragma warning disable 56500 internal void TraceFailFast(string message) { EventLogger logger = null; try { #pragma warning disable 618 logger = new EventLogger(this.eventSourceName, this.diagnosticTrace); #pragma warning restore 618 } finally { #pragma warning disable 618 TraceFailFast(message, logger); #pragma warning restore 618 } } // Fail-- Event Log entry will be generated. // To force a Watson on a dev machine, do the following: // 1. Set \HKLM\SOFTWARE\Microsoft\PCHealth\ErrorReporting ForceQueueMode = 0 // 2. In the command environment, set COMPLUS_DbgJitDebugLaunchSetting=0 [Obsolete("For SMDiagnostics.dll use only. Call DiagnosticUtility.ExceptionUtility instead")] [MethodImpl(MethodImplOptions.NoInlining)] internal static void TraceFailFast(string message, EventLogger logger) { try { if (logger != null) { string stackTrace = null; try { stackTrace = new StackTrace().ToString(); } catch (Exception exception) { stackTrace = exception.Message; } finally { logger.LogEvent(TraceEventType.Critical, EventLogCategory.FailFast, EventLogEventId.FailFast, message, stackTrace); } } } catch (Exception e) { if (logger != null) { logger.LogEvent(TraceEventType.Critical, EventLogCategory.FailFast, EventLogEventId.FailFastException, e.ToString()); } throw; } } #pragma warning restore 56500 [Obsolete("For SMDiagnostics.dll use only. Call DiagnosticUtility.ExceptionUtility instead")] internal void TraceFailFastException(Exception exception) { TraceFailFast(exception == null ? null : exception.ToString()); } internal Exception ThrowHelper(Exception exception, TraceEventType eventType, TraceRecord extendedData) { #pragma warning disable 618 bool shouldTrace = (this.diagnosticTrace != null && this.diagnosticTrace.ShouldTrace(eventType)); #pragma warning restore 618 if (shouldTrace) { using (ExceptionUtility.useStaticActivityId ? Activity.CreateActivity(ExceptionUtility.activityId) : null) { this.diagnosticTrace.TraceEvent(eventType, DiagnosticsTraceCode.ThrowingException, DiagnosticTrace.GenerateMsdnTraceCode("System.ServiceModel.Diagnostics", "ThrowingException"), TraceSR.GetString(TraceSR.ThrowingException), extendedData, exception, null); } } #if DEBUG string stack = exception.StackTrace; #else // Avoid doing this for perf reasons unless we're already taking the hit for tracing. string stack = (shouldTrace ? exception.StackTrace : null); #endif if (!string.IsNullOrEmpty(stack)) { IDictionary data = exception.Data; if (data != null && !data.IsReadOnly && !data.IsFixedSize) { object existingString = data[ExceptionStackAsStringKey]; string stackString = existingString == null ? "" : existingString as string; if (stackString != null) { stackString = string.Concat(stackString, stackString.Length == 0 ? "" : Environment.NewLine, "throw", Environment.NewLine, stack, Environment.NewLine, "catch", Environment.NewLine); data[ExceptionStackAsStringKey] = stackString; } } } return exception; } internal Exception ThrowHelper(Exception exception, TraceEventType eventType) { return this.ThrowHelper(exception, eventType, null); } internal ArgumentException ThrowHelperArgument(string message) { return (ArgumentException)this.ThrowHelperError(new ArgumentException(message)); } internal ArgumentException ThrowHelperArgument(string paramName, string message) { return (ArgumentException)this.ThrowHelperError(new ArgumentException(message, paramName)); } internal ArgumentNullException ThrowHelperArgumentNull(string paramName) { return (ArgumentNullException)this.ThrowHelperError(new ArgumentNullException(paramName)); } internal ArgumentNullException ThrowHelperArgumentNull(string paramName, string message) { return (ArgumentNullException)this.ThrowHelperError(new ArgumentNullException(paramName, message)); } internal Exception ThrowHelperFatal(string message, Exception innerException) { return this.ThrowHelperError(new FatalException(message, innerException)); } internal Exception ThrowHelperInternal(bool fatal) { return fatal ? Fx.AssertAndThrowFatal("Fatal InternalException should never be thrown.") : Fx.AssertAndThrow("InternalException should never be thrown."); } internal Exception ThrowHelperCallback(string message, Exception innerException) { return this.ThrowHelperCritical(new CallbackException(message, innerException)); } internal Exception ThrowHelperCallback(Exception innerException) { return this.ThrowHelperCallback(TraceSR.GetString(TraceSR.GenericCallbackException), innerException); } internal Exception ThrowHelperCritical(Exception exception) { return this.ThrowHelper(exception, TraceEventType.Critical); } internal Exception ThrowHelperError(Exception exception) { return this.ThrowHelper(exception, TraceEventType.Error); } internal Exception ThrowHelperWarning(Exception exception) { return this.ThrowHelper(exception, TraceEventType.Warning); } internal void TraceHandledException(Exception exception, TraceEventType eventType) { #pragma warning disable 618 bool shouldTrace = (this.diagnosticTrace != null && this.diagnosticTrace.ShouldTrace(eventType)); #pragma warning restore 618 if (shouldTrace) { using (ExceptionUtility.useStaticActivityId ? Activity.CreateActivity(ExceptionUtility.activityId) : null) { this.diagnosticTrace.TraceEvent(eventType, DiagnosticsTraceCode.TraceHandledException, DiagnosticTrace.GenerateMsdnTraceCode("System.ServiceModel.Diagnostics", "TraceHandledException"), TraceSR.GetString(TraceSR.TraceHandledException), null, exception, null); } } } // On a single thread, these functions will complete just fine // and don't need to worry about locking issues because the effected // variables are ThreadStatic. internal static void UseActivityId(Guid activityId) { ExceptionUtility.activityId = activityId; ExceptionUtility.useStaticActivityId = true; } internal static void ClearActivityId() { ExceptionUtility.useStaticActivityId = false; ExceptionUtility.activityId = Guid.Empty; } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static bool IsInfrastructureException(Exception exception) { return exception != null && (exception is ThreadAbortException || exception is AppDomainUnloadedException); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
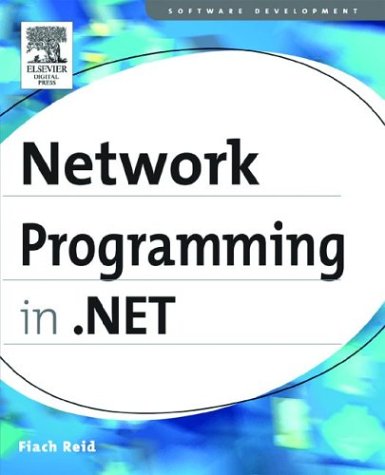
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ItemChangedEventArgs.cs
- CustomAttributeBuilder.cs
- PackagePartCollection.cs
- SQLBytes.cs
- DisplayMemberTemplateSelector.cs
- PatternMatcher.cs
- DragEventArgs.cs
- WindowsListViewGroup.cs
- TextFormatterContext.cs
- DataGridViewColumnTypePicker.cs
- NegotiationTokenAuthenticatorStateCache.cs
- MemoryMappedViewStream.cs
- XmlTypeAttribute.cs
- SystemDropShadowChrome.cs
- StringResourceManager.cs
- EncodingDataItem.cs
- MessageBox.cs
- metadatamappinghashervisitor.cs
- ObjectListDesigner.cs
- WebControl.cs
- ViewSimplifier.cs
- Relationship.cs
- SessionStateContainer.cs
- HttpHandlersSection.cs
- FixedSOMTable.cs
- ConfigurationSectionHelper.cs
- SQLInt16.cs
- InvalidCardException.cs
- Attributes.cs
- TrackingProvider.cs
- Assembly.cs
- EditCommandColumn.cs
- XamlToRtfParser.cs
- ClientTargetSection.cs
- XmlSchemaGroupRef.cs
- SecurityProtocolCorrelationState.cs
- ProfileSection.cs
- CryptoApi.cs
- QueryExpression.cs
- ReadingWritingEntityEventArgs.cs
- RequestCachePolicy.cs
- RectangleGeometry.cs
- DesignerTransactionCloseEvent.cs
- Validator.cs
- CustomErrorsSectionWrapper.cs
- EdmProperty.cs
- KnownColorTable.cs
- SafeArrayRankMismatchException.cs
- ConnectivityStatus.cs
- MsmqBindingElementBase.cs
- httpstaticobjectscollection.cs
- InfoCardConstants.cs
- TemplateControlParser.cs
- PrinterUnitConvert.cs
- ClientBuildManagerCallback.cs
- FastEncoderWindow.cs
- Utils.cs
- RSACryptoServiceProvider.cs
- TableStyle.cs
- WpfSharedXamlSchemaContext.cs
- DoWorkEventArgs.cs
- InternalSafeNativeMethods.cs
- TextProperties.cs
- CodeThrowExceptionStatement.cs
- AdornerDecorator.cs
- ProviderConnectionPointCollection.cs
- CheckoutException.cs
- httpapplicationstate.cs
- TraceUtility.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- TextServicesHost.cs
- DecodeHelper.cs
- XmlSchemaAttributeGroupRef.cs
- LZCodec.cs
- WebPartTransformerCollection.cs
- AssemblyResolver.cs
- RangeValuePatternIdentifiers.cs
- FixedBufferAttribute.cs
- ToolStripSeparator.cs
- ConstraintManager.cs
- ValidationService.cs
- PackWebRequest.cs
- BindingCollection.cs
- TextTreeText.cs
- DataObjectSettingDataEventArgs.cs
- WorkflowMarkupSerializationManager.cs
- SqlError.cs
- QilXmlWriter.cs
- PartialToken.cs
- PointAnimationUsingKeyFrames.cs
- DesignerDataConnection.cs
- UIElement.cs
- Base64Decoder.cs
- CodeEventReferenceExpression.cs
- Comparer.cs
- DetailsView.cs
- PersistChildrenAttribute.cs
- BindingGroup.cs
- securitycriticaldata.cs
- StreamWithDictionary.cs