Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CompMod / System / Collections / Specialized / StringCollection.cs / 1 / StringCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Collections.Specialized { using System.Diagnostics; using System.Collections; ////// [ Serializable, ] public class StringCollection : IList { private ArrayList data = new ArrayList(); ///Represents a collection of strings. ////// public string this[int index] { get { return ((string)data[index]); } set { data[index] = value; } } ///Represents the entry at the specified index of the ///. /// public int Count { get { return data.Count; } } bool IList.IsReadOnly { get { return false; } } bool IList.IsFixedSize { get { return false; } } ///Gets the number of strings in the /// ///. /// public int Add(string value) { return data.Add(value); } ///Adds a string with the specified value to the /// ///. /// public void AddRange(string[] value) { if (value == null) { throw new ArgumentNullException("value"); } data.AddRange(value); } ///Copies the elements of a string array to the end of the ///. /// public void Clear() { data.Clear(); } ///Removes all the strings from the /// ///. /// public bool Contains(string value) { return data.Contains(value); } ///Gets a value indicating whether the /// ///contains a string with the specified /// value. /// public void CopyTo(string[] array, int index) { data.CopyTo(array, index); } ///Copies the ///values to a one-dimensional instance at the /// specified index. /// public StringEnumerator GetEnumerator() { return new StringEnumerator(this); } ///Returns an enumerator that can iterate through /// the ///. /// public int IndexOf(string value) { return data.IndexOf(value); } ///Returns the index of the first occurrence of a string in /// the ///. /// public void Insert(int index, string value) { data.Insert(index, value); } ///Inserts a string into the ///at the specified /// index. /// public bool IsReadOnly { get { return false; } } ///Gets a value indicating whether the ///is read-only. /// public bool IsSynchronized { get { return false; } } ///Gets a value indicating whether access to the /// ////// is synchronized (thread-safe). /// public void Remove(string value) { data.Remove(value); } ///Removes a specific string from the /// ///. /// public void RemoveAt(int index) { data.RemoveAt(index); } ///Removes the string at the specified index of the ///. /// public object SyncRoot { get { return data.SyncRoot; } } object IList.this[int index] { get { return this[index]; } set { this[index] = (string)value; } } int IList.Add(object value) { return Add((string)value); } bool IList.Contains(object value) { return Contains((string) value); } int IList.IndexOf(object value) { return IndexOf((string)value); } void IList.Insert(int index, object value) { Insert(index, (string)value); } void IList.Remove(object value) { Remove((string)value); } void ICollection.CopyTo(Array array, int index) { data.CopyTo(array, index); } IEnumerator IEnumerable.GetEnumerator() { return data.GetEnumerator(); } } ///Gets an object that can be used to synchronize access to the ///. /// public class StringEnumerator { private System.Collections.IEnumerator baseEnumerator; private System.Collections.IEnumerable temp; internal StringEnumerator(StringCollection mappings) { this.temp = (IEnumerable)(mappings); this.baseEnumerator = temp.GetEnumerator(); } ///[To be supplied.] ////// public string Current { get { return (string)(baseEnumerator.Current); } } ///[To be supplied.] ////// public bool MoveNext() { return baseEnumerator.MoveNext(); } ///[To be supplied.] ////// public void Reset() { baseEnumerator.Reset(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Collections.Specialized { using System.Diagnostics; using System.Collections; ////// [ Serializable, ] public class StringCollection : IList { private ArrayList data = new ArrayList(); ///Represents a collection of strings. ////// public string this[int index] { get { return ((string)data[index]); } set { data[index] = value; } } ///Represents the entry at the specified index of the ///. /// public int Count { get { return data.Count; } } bool IList.IsReadOnly { get { return false; } } bool IList.IsFixedSize { get { return false; } } ///Gets the number of strings in the /// ///. /// public int Add(string value) { return data.Add(value); } ///Adds a string with the specified value to the /// ///. /// public void AddRange(string[] value) { if (value == null) { throw new ArgumentNullException("value"); } data.AddRange(value); } ///Copies the elements of a string array to the end of the ///. /// public void Clear() { data.Clear(); } ///Removes all the strings from the /// ///. /// public bool Contains(string value) { return data.Contains(value); } ///Gets a value indicating whether the /// ///contains a string with the specified /// value. /// public void CopyTo(string[] array, int index) { data.CopyTo(array, index); } ///Copies the ///values to a one-dimensional instance at the /// specified index. /// public StringEnumerator GetEnumerator() { return new StringEnumerator(this); } ///Returns an enumerator that can iterate through /// the ///. /// public int IndexOf(string value) { return data.IndexOf(value); } ///Returns the index of the first occurrence of a string in /// the ///. /// public void Insert(int index, string value) { data.Insert(index, value); } ///Inserts a string into the ///at the specified /// index. /// public bool IsReadOnly { get { return false; } } ///Gets a value indicating whether the ///is read-only. /// public bool IsSynchronized { get { return false; } } ///Gets a value indicating whether access to the /// ////// is synchronized (thread-safe). /// public void Remove(string value) { data.Remove(value); } ///Removes a specific string from the /// ///. /// public void RemoveAt(int index) { data.RemoveAt(index); } ///Removes the string at the specified index of the ///. /// public object SyncRoot { get { return data.SyncRoot; } } object IList.this[int index] { get { return this[index]; } set { this[index] = (string)value; } } int IList.Add(object value) { return Add((string)value); } bool IList.Contains(object value) { return Contains((string) value); } int IList.IndexOf(object value) { return IndexOf((string)value); } void IList.Insert(int index, object value) { Insert(index, (string)value); } void IList.Remove(object value) { Remove((string)value); } void ICollection.CopyTo(Array array, int index) { data.CopyTo(array, index); } IEnumerator IEnumerable.GetEnumerator() { return data.GetEnumerator(); } } ///Gets an object that can be used to synchronize access to the ///. /// public class StringEnumerator { private System.Collections.IEnumerator baseEnumerator; private System.Collections.IEnumerable temp; internal StringEnumerator(StringCollection mappings) { this.temp = (IEnumerable)(mappings); this.baseEnumerator = temp.GetEnumerator(); } ///[To be supplied.] ////// public string Current { get { return (string)(baseEnumerator.Current); } } ///[To be supplied.] ////// public bool MoveNext() { return baseEnumerator.MoveNext(); } ///[To be supplied.] ////// public void Reset() { baseEnumerator.Reset(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
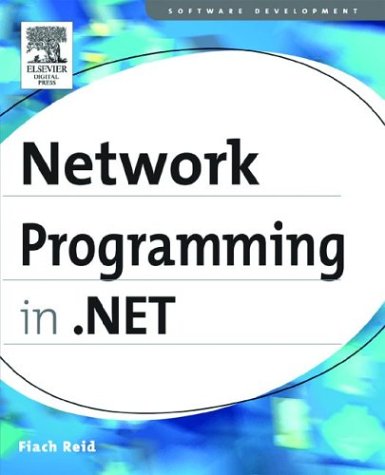
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WMICapabilities.cs
- Pointer.cs
- CompositeFontInfo.cs
- ClientSettingsProvider.cs
- DocumentPageHost.cs
- EntityClientCacheEntry.cs
- WebResourceUtil.cs
- MatrixStack.cs
- XsdCachingReader.cs
- Matrix3D.cs
- ParseNumbers.cs
- CacheModeValueSerializer.cs
- SoapFault.cs
- CapabilitiesPattern.cs
- XmlSchemaComplexContent.cs
- DataGridViewTextBoxEditingControl.cs
- OleDbParameter.cs
- TransactionFlowAttribute.cs
- FrameDimension.cs
- Rect3D.cs
- DebugView.cs
- TreeViewEvent.cs
- AdCreatedEventArgs.cs
- DataListItemCollection.cs
- EncoderExceptionFallback.cs
- GacUtil.cs
- TextDataBindingHandler.cs
- LinqDataSourceContextData.cs
- MILUtilities.cs
- WMICapabilities.cs
- ObjectQueryProvider.cs
- NetworkInterface.cs
- XmlSchemaProviderAttribute.cs
- CacheOutputQuery.cs
- DropShadowEffect.cs
- GroupStyle.cs
- ManifestResourceInfo.cs
- BooleanFacetDescriptionElement.cs
- TypeLibConverter.cs
- StructuralObject.cs
- ImageIndexConverter.cs
- PriorityItem.cs
- recordstate.cs
- CompleteWizardStep.cs
- HttpProfileGroupBase.cs
- WpfWebRequestHelper.cs
- Geometry3D.cs
- SwitchCase.cs
- ObjectHandle.cs
- AnimationStorage.cs
- TreeViewDesigner.cs
- SharedUtils.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- StylusButtonCollection.cs
- NativeRightsManagementAPIsStructures.cs
- DetailsViewDeleteEventArgs.cs
- OleDbInfoMessageEvent.cs
- FeatureSupport.cs
- CodeCommentStatementCollection.cs
- HyperLinkColumn.cs
- SinglePageViewer.cs
- Block.cs
- VirtualPathUtility.cs
- List.cs
- SmiRequestExecutor.cs
- GridLengthConverter.cs
- precedingquery.cs
- CatalogPartChrome.cs
- FilterElement.cs
- MethodImplAttribute.cs
- BaseParser.cs
- Vector3DKeyFrameCollection.cs
- TypedTableBase.cs
- TileBrush.cs
- DES.cs
- SynchronizedInputAdaptor.cs
- Debug.cs
- DecimalFormatter.cs
- WorkflowItemsPresenter.cs
- FilterException.cs
- TableCellsCollectionEditor.cs
- ConfigurationElementCollection.cs
- LinqExpressionNormalizer.cs
- ExtendedPropertyDescriptor.cs
- WebPartEditorCancelVerb.cs
- EntityRecordInfo.cs
- QueryExpression.cs
- WebHttpBehavior.cs
- NavigateEvent.cs
- ClickablePoint.cs
- HtmlContainerControl.cs
- _ProxyChain.cs
- BitmapPalettes.cs
- HebrewNumber.cs
- QilExpression.cs
- MessageDecoder.cs
- SafeRightsManagementPubHandle.cs
- DataGridRelationshipRow.cs
- DataObject.cs
- SubqueryTrackingVisitor.cs