Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / Host / Serialization / CodeDomLocalizationProvider.cs / 1 / CodeDomLocalizationProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Globalization; using System.Resources; using System.Windows.Forms; using System.Windows.Forms.Design; ////// /// This is a serialization provider that provides a localization feature. This provider /// adds two properties to the root component: Language and Localizable. If Localizable /// is set to true this provider will change the way that component properties are generated /// and will route their values to a resource file. Two localization models are /// supported. /// public sealed class CodeDomLocalizationProvider: IDisposable, IDesignerSerializationProvider { private IExtenderProviderService _providerService; private CodeDomLocalizationModel _model; private CultureInfo[] _supportedCultures; private LanguageExtenders _extender; private Hashtable _memberSerializers; private Hashtable _nopMemberSerializers; ////// /// Creates a new adapter and attaches it to the serialization manager. This /// will add itself as a serializer for resources into the serialization manager, and, /// if not already added, will add itself as an extender provider to the roost component /// and provide the Language and Localizable properties. The latter piece is only /// supplied if CodeDomLocalizationModel is not �none�. /// public CodeDomLocalizationProvider(IServiceProvider provider, CodeDomLocalizationModel model) { if (provider == null) throw new ArgumentNullException("provider"); _model = model; Initialize(provider); } ////// /// Creates a new adapter and attaches it to the serialization manager. This /// will add itself as a serializer for resources into the serialization manager, and, /// if not already added, will add itself as an extender provider to the roost component /// and provide the Language and Localizable properties. The latter piece is only /// supplied if CodeDomLocalizationModel is not �none�. /// public CodeDomLocalizationProvider(IServiceProvider provider, CodeDomLocalizationModel model, CultureInfo[] supportedCultures) { if (provider == null) throw new ArgumentNullException("provider"); if (supportedCultures == null) throw new ArgumentNullException("supportedCultures"); _model = model; _supportedCultures = (CultureInfo[])supportedCultures.Clone(); Initialize(provider); } ////// /// Disposes this object. /// public void Dispose() { if (_providerService != null && _extender != null) { _providerService.RemoveExtenderProvider(_extender); _providerService = null; _extender = null; } } ////// Adds our extended properties. /// private void Initialize(IServiceProvider provider) { _providerService = provider.GetService(typeof(IExtenderProviderService)) as IExtenderProviderService; if (_providerService == null) { throw new NotSupportedException(SR.GetString(SR.LocalizationProviderMissingService, typeof(IExtenderProviderService).Name)); } _extender = new LanguageExtenders(provider, _supportedCultures); _providerService.AddExtenderProvider(_extender); } #region IDesignerSerializationProvider Members ////// Returns a code dom serializer /// private object GetCodeDomSerializer(IDesignerSerializationManager manager, object currentSerializer, Type objectType, Type serializerType) { if (currentSerializer == null) { return null; } // Always do default processing for the resource manager. if (typeof(ResourceManager).IsAssignableFrom(objectType)) { return null; } // Here's how this works. We have two different types of serializers to offer : a // serializer that writes out code like this: // // this.Button1.Text = rm.GetString("Button1_Text"); // // And one that writes out like this: // // rm.ApplyResources(Button1, "Button1"); // // The first serializer is used for serializable objects that have no serializer of their // own, and for localizable properties when the CodeDomLocalizationModel is set to PropertyAssignment. // The second serializer is used only for localizaing properties when the CodeDomLocalizationModel // is set to PropertyReflection // // Compute a localization model based on the property, localization mode, // and what (if any) serializer already exists CodeDomLocalizationModel model = CodeDomLocalizationModel.None; object modelObj = manager.Context[typeof(CodeDomLocalizationModel)]; if (modelObj != null) model = (CodeDomLocalizationModel)modelObj; /* Nifty, but this causes everything to be loc'd because our provider comes in before the default one if (model == CodeDomLocalizationModel.None && currentSerializer == null) { model = CodeDomLocalizationModel.PropertyAssignment; } */ if (model != CodeDomLocalizationModel.None) { return new LocalizationCodeDomSerializer(model, currentSerializer); } return null; } ////// Returns a code dom serializer for members. /// private object GetMemberCodeDomSerializer(IDesignerSerializationManager manager, object currentSerializer, Type objectType, Type serializerType) { CodeDomLocalizationModel model = _model; if (!typeof(PropertyDescriptor).IsAssignableFrom(objectType)) { return null; } // Ok, we got a property descriptor. If we're being localized // we provide a different type of serializer. But, we only // do this if we were given a current serializer. Otherwise // we don't know how to perform the serialization. // We can only provide a custom serializer if we have an existing one // to base off of. if (currentSerializer == null) { return null; } // If we've already provided this serializer, don't do it again if (currentSerializer is ResourcePropertyMemberCodeDomSerializer) { return null; } // We only care if we're localizable if (_extender == null || !_extender.GetLocalizable(null)) { return null; } // Fish the property out of the context to see if the property is // localizable. PropertyDescriptor serializingProperty = manager.Context[typeof(PropertyDescriptor)] as PropertyDescriptor; if (serializingProperty == null || !serializingProperty.IsLocalizable) { model = CodeDomLocalizationModel.None; } if (_memberSerializers == null) { _memberSerializers = new Hashtable(); } if (_nopMemberSerializers == null) { _nopMemberSerializers = new Hashtable(); } object newSerializer = null; if (model == CodeDomLocalizationModel.None) { newSerializer = _nopMemberSerializers[currentSerializer]; } else { newSerializer = _memberSerializers[currentSerializer]; } if (newSerializer == null) { newSerializer = new ResourcePropertyMemberCodeDomSerializer((MemberCodeDomSerializer)currentSerializer, _extender, model); if (model == CodeDomLocalizationModel.None) { _nopMemberSerializers[currentSerializer] = newSerializer; } else { _memberSerializers[currentSerializer] = newSerializer; } } return newSerializer; } ////// /// Returns an appropriate serializer for the object. /// object IDesignerSerializationProvider.GetSerializer(IDesignerSerializationManager manager, object currentSerializer, Type objectType, Type serializerType) { if (serializerType == typeof(CodeDomSerializer)) { return GetCodeDomSerializer(manager, currentSerializer, objectType, serializerType); } else if (serializerType == typeof(MemberCodeDomSerializer)) { return GetMemberCodeDomSerializer(manager, currentSerializer, objectType, serializerType); } return null; // don't understand this type of serializer. } #endregion #region LanguageExtenders class ////// The design time language and localizable properties. /// [ProvideProperty("Language", typeof(IComponent))] [ProvideProperty("LoadLanguage", typeof(IComponent))] [ProvideProperty("Localizable", typeof(IComponent))] internal class LanguageExtenders: IExtenderProvider { private IServiceProvider _serviceProvider; private IDesignerHost _host; private IComponent _lastRoot; private TypeConverter.StandardValuesCollection _supportedCultures; private bool _localizable; private CultureInfo _language; private CultureInfo _loadLanguage; private CultureInfo _defaultLanguage; ////// public LanguageExtenders(IServiceProvider serviceProvider, CultureInfo[] supportedCultures) { _serviceProvider = serviceProvider; _host = serviceProvider.GetService(typeof(IDesignerHost)) as IDesignerHost; _language = CultureInfo.InvariantCulture; if (supportedCultures != null) { _supportedCultures = new TypeConverter.StandardValuesCollection(supportedCultures); } } ////// A collection of custom supported cultures. This can be null, indicating that the /// type converter should use the default set of supported cultures. /// ///internal TypeConverter.StandardValuesCollection SupportedCultures { get { return _supportedCultures; } } /// /// Returns the current default language for the thread. /// private CultureInfo ThreadDefaultLanguage { get { if (_defaultLanguage == null) { _defaultLanguage = Application.CurrentCulture; } return _defaultLanguage; } } ////// Broadcasts a global change, indicating that all /// objects on the designer have changed. /// private void BroadcastGlobalChange(IComponent comp) { ISite site = comp.Site; if (site != null) { IComponentChangeService cs = site.GetService(typeof(IComponentChangeService)) as IComponentChangeService; IContainer container = site.GetService(typeof(IContainer)) as IContainer; if (cs != null && container != null) { foreach(IComponent c in container.Components) { cs.OnComponentChanging(c, null); cs.OnComponentChanged(c, null, null, null); } } } } ////// This method compares the current root component /// with the last one we saw. If they don't match, /// that means the designer has reloaded and we /// should set all of our properties back to their /// defaults. This is more efficient than syncing /// an event. /// private void CheckRoot() { if (_host != null && _host.RootComponent != _lastRoot) { _lastRoot = _host.RootComponent; _language = CultureInfo.InvariantCulture; _loadLanguage = null; _localizable = false; } } ////// Gets the language set for the specified object. /// [DesignOnly(true)] [TypeConverter(typeof(LanguageCultureInfoConverter))] [Category("Design")] [SRDescriptionAttribute("LocalizationProviderLanguageDescr")] public CultureInfo GetLanguage(IComponent o) { CheckRoot(); return _language; } ////// Gets the language we'll use when re-loading the designer. /// [DesignOnly(true)] [Browsable(false)] [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public CultureInfo GetLoadLanguage(IComponent o) { CheckRoot(); // If we never configured the load language, we're always invariant. if (_loadLanguage == null) { _loadLanguage = CultureInfo.InvariantCulture; } return _loadLanguage; } ////// Gets a value indicating whether the specified object supports design-time localization /// support. /// [DesignOnly(true)] [Category("Design")] [SRDescriptionAttribute("LocalizationProviderLocalizableDescr")] public bool GetLocalizable(IComponent o) { CheckRoot(); return _localizable; } ////// Sets the language to use. When the language is set the designer will be /// reloaded. /// public void SetLanguage(IComponent o, CultureInfo language) { CheckRoot(); if (language == null) { language = CultureInfo.InvariantCulture; } bool isInvariantCulture = (language.Equals(CultureInfo.InvariantCulture)); CultureInfo defaultUICulture = this.ThreadDefaultLanguage; if (_language.Equals(language)) { return; } _language = language; if (!isInvariantCulture) { SetLocalizable(o, true); } if (_serviceProvider != null && _host != null) { IDesignerLoaderService ls = _serviceProvider.GetService(typeof(IDesignerLoaderService)) as IDesignerLoaderService; // Only reload if we're not in the process of loading! // if (_host.Loading) { _loadLanguage = language; } else { bool reloadSuccessful = false; if (ls != null) { reloadSuccessful = ls.Reload(); } if (!reloadSuccessful) { IUIService uis = (IUIService)_serviceProvider.GetService(typeof(IUIService)); if (uis != null) { uis.ShowMessage(SR.GetString(SR.LocalizationProviderManualReload)); } } } } } ////// Sets a value indicating whether or not the specified object has design-time /// localization support. /// public void SetLocalizable(IComponent o, bool localizable) { CheckRoot(); if (localizable != _localizable) { _localizable = localizable; if (!localizable) { SetLanguage(o, CultureInfo.InvariantCulture); } if (_host != null && !_host.Loading) { BroadcastGlobalChange(o); } } } ////// Gets a value indicating whether the specified object should have its design-time localization support persisted. /// private bool ShouldSerializeLanguage(IComponent o) { return (_language != null && _language != CultureInfo.InvariantCulture); } ////// Gets a value indicating whether the specified object should have its design-time localization support persisted. /// private bool ShouldSerializeLocalizable(IComponent o) { return (_localizable); } ////// Resets the localizable property to the 'defaultLocalizable' value. /// private void ResetLocalizable(IComponent o) { SetLocalizable(o, false); } ////// Resets the language for the specified object. /// private void ResetLanguage(IComponent o) { SetLanguage(o, CultureInfo.InvariantCulture); } ////// We only extend the root component. /// public bool CanExtend(object o) { CheckRoot(); return (_host != null && o == _host.RootComponent); } } #region LanguageCultureInfoConverter ////// This is a culture info converter that knows how to provide /// a restricted list of cultures based on the SupportedCultures /// property of the extender. If the extender can't be found /// or the SupportedCultures property returns null, this /// defaults to the stock implementation. /// internal sealed class LanguageCultureInfoConverter: CultureInfoConverter { ////// Gets a collection of standard values collection for a System.Globalization.CultureInfo /// object using the specified context. /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { StandardValuesCollection values = null; if (context.PropertyDescriptor != null) { ExtenderProvidedPropertyAttribute attr = context.PropertyDescriptor.Attributes[typeof(ExtenderProvidedPropertyAttribute)] as ExtenderProvidedPropertyAttribute; if (attr != null) { LanguageExtenders provider = attr.Provider as LanguageExtenders; if (provider != null) { values = provider.SupportedCultures; } } } if (values == null) { values = base.GetStandardValues(context); } return values; } } #endregion #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
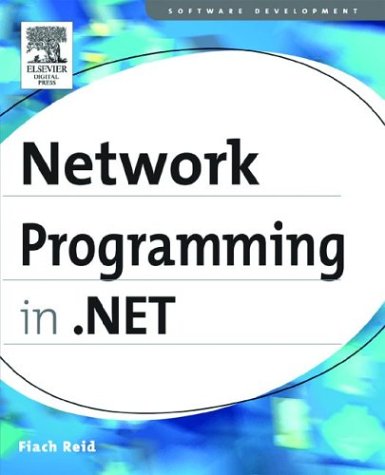
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SchemaNames.cs
- EdmScalarPropertyAttribute.cs
- HttpApplicationStateBase.cs
- WebCategoryAttribute.cs
- ReadOnlyNameValueCollection.cs
- WindowsRegion.cs
- DictionaryContent.cs
- OleDbInfoMessageEvent.cs
- SynthesizerStateChangedEventArgs.cs
- XmlWriterSettings.cs
- SendingRequestEventArgs.cs
- GenericAuthenticationEventArgs.cs
- SafeProcessHandle.cs
- OdbcConnection.cs
- MemoryRecordBuffer.cs
- ArraySortHelper.cs
- BrowserTree.cs
- NativeMethods.cs
- SessionConnectionReader.cs
- TabItemAutomationPeer.cs
- ApplicationFileCodeDomTreeGenerator.cs
- IndexOutOfRangeException.cs
- XmlHierarchicalDataSourceView.cs
- XmlSerializerNamespaces.cs
- TemplateBuilder.cs
- MemoryFailPoint.cs
- FusionWrap.cs
- BitmapPalette.cs
- NativeCompoundFileAPIs.cs
- MobilePage.cs
- DetailsView.cs
- FixedSOMLineRanges.cs
- ImageSource.cs
- ReadOnlyTernaryTree.cs
- FileInfo.cs
- ChineseLunisolarCalendar.cs
- Rotation3DAnimationUsingKeyFrames.cs
- RowSpanVector.cs
- SmiEventStream.cs
- ZoneLinkButton.cs
- TreeView.cs
- AttributeInfo.cs
- XmlQueryOutput.cs
- DataDocumentXPathNavigator.cs
- XmlSecureResolver.cs
- PointLightBase.cs
- TimeSpanOrInfiniteValidator.cs
- SafeRightsManagementSessionHandle.cs
- VirtualizingPanel.cs
- Typeface.cs
- Calendar.cs
- ProfileEventArgs.cs
- XsdValidatingReader.cs
- Soap12ProtocolImporter.cs
- OleDbConnectionInternal.cs
- SafeNativeMethodsOther.cs
- XmlEntity.cs
- EventLogEntry.cs
- CatalogZone.cs
- LineGeometry.cs
- SqlTrackingWorkflowInstance.cs
- XmlSchemaSimpleTypeList.cs
- XPathDocumentIterator.cs
- AvTraceDetails.cs
- FileDataSourceCache.cs
- SystemIPv6InterfaceProperties.cs
- SystemTcpStatistics.cs
- EnumMemberAttribute.cs
- FileDetails.cs
- SecurityCredentialsManager.cs
- DoubleAnimation.cs
- OlePropertyStructs.cs
- PathStreamGeometryContext.cs
- PageWrapper.cs
- EditorZone.cs
- DaylightTime.cs
- HTTPNotFoundHandler.cs
- WorkflowFileItem.cs
- UrlPath.cs
- Floater.cs
- ClientClassGenerator.cs
- ProvidePropertyAttribute.cs
- HttpCacheParams.cs
- WorkItem.cs
- ReceiveCompletedEventArgs.cs
- HttpServerVarsCollection.cs
- GPStream.cs
- TypeForwardedFromAttribute.cs
- ExpressionNode.cs
- Constants.cs
- TextFindEngine.cs
- RunClient.cs
- RootProfilePropertySettingsCollection.cs
- webproxy.cs
- GridViewCommandEventArgs.cs
- Hex.cs
- CustomErrorCollection.cs
- NetworkInformationException.cs
- EdmSchemaError.cs
- WCFModelStrings.Designer.cs