Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / EntitySql / AST / QueryExpr.cs / 1305376 / QueryExpr.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- namespace System.Data.Common.EntitySql.AST { using System; using System.Globalization; using System.Collections; using System.Collections.Generic; ////// Represents select kind (value,row). /// internal enum SelectKind { Value, Row } ////// Represents join kind (cross,inner,leftouter,rightouter). /// internal enum JoinKind { Cross, Inner, LeftOuter, FullOuter, RightOuter } ////// Represents order kind (none=asc,asc,desc). /// internal enum OrderKind { None, Asc, Desc } ////// Represents distinct kind (none=all,all,distinct). /// internal enum DistinctKind { None, All, Distinct } ////// Represents apply kind (cross,outer). /// internal enum ApplyKind { Cross, Outer } ////// Represents a query expression ast node. /// internal sealed class QueryExpr : Node { private readonly SelectClause _selectClause; private readonly FromClause _fromClause; private readonly Node _whereClause; private readonly GroupByClause _groupByClause; private readonly HavingClause _havingClause; private readonly OrderByClause _orderByClause; ////// Initializes a query expression ast node. /// /// select clause /// from clasuse /// optional where clause /// optional group by clause /// optional having clause /// optional order by clause internal QueryExpr(SelectClause selectClause, FromClause fromClause, Node whereClause, GroupByClause groupByClause, HavingClause havingClause, OrderByClause orderByClause) { _selectClause = selectClause; _fromClause = fromClause; _whereClause = whereClause; _groupByClause = groupByClause; _havingClause = havingClause; _orderByClause = orderByClause; } ////// Returns select clause. /// internal SelectClause SelectClause { get { return _selectClause; } } ////// Returns from clause. /// internal FromClause FromClause { get { return _fromClause; } } ////// Returns optional where clause (expr). /// internal Node WhereClause { get { return _whereClause; } } ////// Returns optional group by clause. /// internal GroupByClause GroupByClause { get { return _groupByClause; } } ////// Returns optional having clause (expr). /// internal HavingClause HavingClause { get { return _havingClause; } } ////// Returns optional order by clause. /// internal OrderByClause OrderByClause { get { return _orderByClause; } } ////// Returns true if method calls are present. /// internal bool HasMethodCall { get { return _selectClause.HasMethodCall || (null != _havingClause && _havingClause.HasMethodCall) || (null != _orderByClause && _orderByClause.HasMethodCall); } } } ////// Represents select clause. /// internal sealed class SelectClause : Node { private readonly NodeList_selectClauseItems; private readonly SelectKind _selectKind; private readonly DistinctKind _distinctKind; private readonly Node _topExpr; private readonly uint _methodCallCount; /// /// Initialize SelectKind.SelectRow clause. /// internal SelectClause(NodeListitems, SelectKind selectKind, DistinctKind distinctKind, Node topExpr, uint methodCallCount) { _selectKind = selectKind; _selectClauseItems = items; _distinctKind = distinctKind; _topExpr = topExpr; _methodCallCount = methodCallCount; } /// /// Projection list. /// internal NodeListItems { get { return _selectClauseItems; } } /// /// Select kind (row or value). /// internal SelectKind SelectKind { get { return _selectKind; } } ////// Distinct kind (none,all,distinct). /// internal DistinctKind DistinctKind { get { return _distinctKind; } } ////// Optional top expression. /// internal Node TopExpr { get { return _topExpr; } } ////// True if select list has method calls. /// internal bool HasMethodCall { get { return (_methodCallCount > 0); } } } ////// Represents from clause. /// internal sealed class FromClause : Node { private readonly NodeList_fromClauseItems; /// /// Initializes from clause. /// internal FromClause(NodeListfromClauseItems) { _fromClauseItems = fromClauseItems; } /// /// List of from clause items. /// internal NodeListFromClauseItems { get { return _fromClauseItems; } } } /// /// From clause item kind. /// internal enum FromClauseItemKind { AliasedFromClause, JoinFromClause, ApplyFromClause } ////// Represents single from clause item. /// internal sealed class FromClauseItem : Node { private readonly Node _fromClauseItemExpr; private readonly FromClauseItemKind _fromClauseItemKind; ////// Initializes as 'simple' aliased expression. /// internal FromClauseItem(AliasedExpr aliasExpr) { _fromClauseItemExpr = aliasExpr; _fromClauseItemKind = FromClauseItemKind.AliasedFromClause; } ////// Initializes as join clause item. /// internal FromClauseItem(JoinClauseItem joinClauseItem) { _fromClauseItemExpr = joinClauseItem; _fromClauseItemKind = FromClauseItemKind.JoinFromClause; } ////// Initializes as apply clause item. /// internal FromClauseItem(ApplyClauseItem applyClauseItem) { _fromClauseItemExpr = applyClauseItem; _fromClauseItemKind = FromClauseItemKind.ApplyFromClause; } ////// From clause item expression. /// internal Node FromExpr { get { return _fromClauseItemExpr; } } ////// From clause item kind (alias,join,apply). /// internal FromClauseItemKind FromClauseItemKind { get { return _fromClauseItemKind; } } } ////// Represents group by clause. /// internal sealed class GroupByClause : Node { private readonly NodeList_groupItems; /// /// Initializes GROUP BY clause /// internal GroupByClause(NodeListgroupItems) { _groupItems = groupItems; } /// /// Group items. /// internal NodeListGroupItems { get { return _groupItems; } } } /// /// Represents having clause. /// internal sealed class HavingClause : Node { private readonly Node _havingExpr; private readonly uint _methodCallCount; ////// Initializes having clause. /// internal HavingClause(Node havingExpr, uint methodCallCounter) { _havingExpr = havingExpr; _methodCallCount = methodCallCounter; } ////// Returns having inner expression. /// internal Node HavingPredicate { get { return _havingExpr; } } ////// True if predicate has method calls. /// internal bool HasMethodCall { get { return (_methodCallCount > 0); } } } ////// Represents order by clause. /// internal sealed class OrderByClause : Node { private readonly NodeList_orderByClauseItem; private readonly Node _skipExpr; private readonly Node _limitExpr; private readonly uint _methodCallCount; /// /// Initializes order by clause. /// internal OrderByClause(NodeListorderByClauseItem, Node skipExpr, Node limitExpr, uint methodCallCount) { _orderByClauseItem = orderByClauseItem; _skipExpr = skipExpr; _limitExpr = limitExpr; _methodCallCount = methodCallCount; } /// /// Returns order by clause items. /// internal NodeListOrderByClauseItem { get { return _orderByClauseItem; } } /// /// Returns skip sub clause ast node. /// internal Node SkipSubClause { get { return _skipExpr; } } ////// Returns limit sub-clause ast node. /// internal Node LimitSubClause { get { return _limitExpr; } } ////// True if order by has method calls. /// internal bool HasMethodCall { get { return (_methodCallCount > 0); } } } ////// Represents a order by clause item. /// internal sealed class OrderByClauseItem : Node { private readonly Node _orderExpr; private readonly OrderKind _orderKind; private readonly Identifier _optCollationIdentifier; ////// Initializes non-collated order by clause item. /// internal OrderByClauseItem(Node orderExpr, OrderKind orderKind) : this(orderExpr, orderKind, null) { } ////// Initializes collated order by clause item. /// /// optional Collation identifier internal OrderByClauseItem(Node orderExpr, OrderKind orderKind, Identifier optCollationIdentifier) { _orderExpr = orderExpr; _orderKind = orderKind; _optCollationIdentifier = optCollationIdentifier; } ////// Oeturns order expression. /// internal Node OrderExpr { get { return _orderExpr; } } ////// Returns order kind (none,asc,desc). /// internal OrderKind OrderKind { get { return _orderKind; } } ////// Returns collattion identifier if one exists. /// internal Identifier Collation { get { return _optCollationIdentifier; } } } ////// Represents join clause item. /// internal sealed class JoinClauseItem : Node { private readonly FromClauseItem _joinLeft; private readonly FromClauseItem _joinRight; private JoinKind _joinKind; private readonly Node _onExpr; ////// Initializes join clause item without ON expression. /// internal JoinClauseItem(FromClauseItem joinLeft, FromClauseItem joinRight, JoinKind joinKind) : this(joinLeft, joinRight, joinKind, null) { } ////// Initializes join clause item with ON expression. /// internal JoinClauseItem(FromClauseItem joinLeft, FromClauseItem joinRight, JoinKind joinKind, Node onExpr) { _joinLeft = joinLeft; _joinRight = joinRight; _joinKind = joinKind; _onExpr = onExpr; } ////// Returns join left expression. /// internal FromClauseItem LeftExpr { get { return _joinLeft; } } ////// Returns join right expression. /// internal FromClauseItem RightExpr { get { return _joinRight; } } ////// Join kind (cross, inner, full, left outer,right outer). /// internal JoinKind JoinKind { get { return _joinKind; } set { _joinKind = value; } } ////// Returns join on expression. /// internal Node OnExpr { get { return _onExpr; } } } ////// Represents apply expression. /// internal sealed class ApplyClauseItem : Node { private readonly FromClauseItem _applyLeft; private readonly FromClauseItem _applyRight; private readonly ApplyKind _applyKind; ////// Initializes apply clause item. /// internal ApplyClauseItem(FromClauseItem applyLeft, FromClauseItem applyRight, ApplyKind applyKind) { _applyLeft = applyLeft; _applyRight = applyRight; _applyKind = applyKind; } ////// Returns apply left expression. /// internal FromClauseItem LeftExpr { get { return _applyLeft; } } ////// Returns apply right expression. /// internal FromClauseItem RightExpr { get { return _applyRight; } } ////// Returns apply kind (cross,outer). /// internal ApplyKind ApplyKind { get { return _applyKind; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
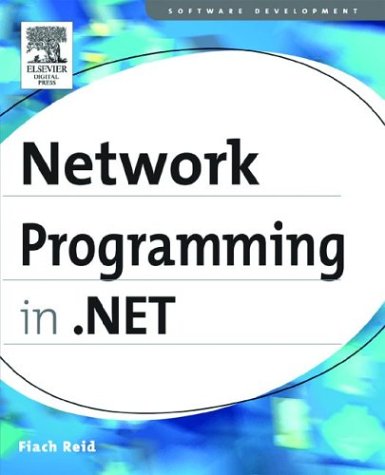
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateBamlRecordReader.cs
- HybridObjectCache.cs
- OleDbConnectionFactory.cs
- RemotingException.cs
- ClockController.cs
- DesignerForm.cs
- WorkflowFormatterBehavior.cs
- ValidatorUtils.cs
- SmtpException.cs
- UIPropertyMetadata.cs
- DelimitedListTraceListener.cs
- ManagementInstaller.cs
- BCryptNative.cs
- EntityDataReader.cs
- WindowsRebar.cs
- NullReferenceException.cs
- CharStorage.cs
- DbConnectionPoolGroupProviderInfo.cs
- FormViewPagerRow.cs
- TrustManagerMoreInformation.cs
- ListDataHelper.cs
- SqlNotificationRequest.cs
- ColumnReorderedEventArgs.cs
- ScrollPattern.cs
- SchemaAttDef.cs
- DecoderFallback.cs
- EntityAdapter.cs
- AssemblyAttributesGoHere.cs
- HtmlInputRadioButton.cs
- LocationSectionRecord.cs
- FixedTextPointer.cs
- DynamicMetaObject.cs
- EnumBuilder.cs
- Parser.cs
- ProtocolsConfiguration.cs
- WorkflowMarkupSerializerMapping.cs
- XmlSerializerAssemblyAttribute.cs
- TraceSwitch.cs
- ValidateNames.cs
- IItemContainerGenerator.cs
- MembershipUser.cs
- PropertyChangeTracker.cs
- Vector.cs
- FormViewPageEventArgs.cs
- UIntPtr.cs
- XmlIlVisitor.cs
- Preprocessor.cs
- ConnectionProviderAttribute.cs
- MsmqHostedTransportManager.cs
- DbConnectionPoolGroupProviderInfo.cs
- ConfigurationSectionCollection.cs
- InputLangChangeEvent.cs
- SrgsElement.cs
- AddInStore.cs
- IBuiltInEvidence.cs
- XComponentModel.cs
- ViewBase.cs
- VariableReference.cs
- DtdParser.cs
- BuilderInfo.cs
- XPathNavigatorKeyComparer.cs
- ZipFileInfoCollection.cs
- Lease.cs
- SlipBehavior.cs
- GlobalProxySelection.cs
- TextLine.cs
- XmlSchemaImporter.cs
- ServiceDesigner.xaml.cs
- RIPEMD160.cs
- SystemColors.cs
- SocketManager.cs
- KeyProperty.cs
- TextTreeObjectNode.cs
- SqlConnectionHelper.cs
- DetailsViewDeletedEventArgs.cs
- ColorConverter.cs
- Emitter.cs
- ZoneIdentityPermission.cs
- TableLayoutStyle.cs
- ZoneButton.cs
- TypedTableHandler.cs
- EntityViewContainer.cs
- TextElementCollection.cs
- ImageMap.cs
- shaperfactoryquerycachekey.cs
- StrongNamePublicKeyBlob.cs
- OrderedDictionaryStateHelper.cs
- RadioButtonList.cs
- RequestDescription.cs
- AssemblyCollection.cs
- XmlBinaryReader.cs
- FileReader.cs
- StringFormat.cs
- GeneralTransform3DCollection.cs
- TextEndOfSegment.cs
- RelationshipType.cs
- DesignerVerbCollection.cs
- XDeferredAxisSource.cs
- AvTraceFormat.cs
- DbgUtil.cs