Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / ComponentModel / COM2Interop / COM2FontConverter.cs / 1 / COM2FontConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.Drawing; using System.Collections; using Microsoft.Win32; ////// /// This class maps an OLE_COLOR to a managed Color editor. /// internal class Com2FontConverter : Com2DataTypeToManagedDataTypeConverter { private IntPtr lastHandle = IntPtr.Zero; private Font lastFont = null; public override bool AllowExpand { get { return true; } } ////// /// Returns the managed type that this editor maps the property type to. /// public override Type ManagedType { get { return typeof(Font); } } ////// /// Converts the native value into a managed value /// public override object ConvertNativeToManaged(object nativeValue, Com2PropertyDescriptor pd) { // we're getting an IFont thing here UnsafeNativeMethods.IFont nativeFont = nativeValue as UnsafeNativeMethods.IFont; if (nativeFont == null) { lastHandle = IntPtr.Zero; lastFont = Control.DefaultFont; return lastFont; } IntPtr fontHandle = nativeFont.GetHFont(); // see if we have this guy cached if (fontHandle == lastHandle && lastFont != null) { return lastFont; } lastHandle = fontHandle; try { // this wasn't working because it was converting everything to // world units. // Font font = Font.FromHfont(lastHandle); try { lastFont = ControlPaint.FontInPoints(font); } finally { font.Dispose(); } } catch(ArgumentException) { // we will fail on non-truetype fonts, so // just use the default font. lastFont = Control.DefaultFont; } return lastFont; } ////// /// Converts the managed value into a native value /// public override object ConvertManagedToNative(object managedValue, Com2PropertyDescriptor pd, ref bool cancelSet) { // we default to black. // if (managedValue == null) { managedValue = Control.DefaultFont; } cancelSet = true; if (lastFont != null && lastFont.Equals(managedValue)) { // don't do anything here. return null; } lastFont = (Font)managedValue; UnsafeNativeMethods.IFont nativeFont = (UnsafeNativeMethods.IFont)pd.GetNativeValue(pd.TargetObject); // now, push all the values into the native side if (nativeFont != null) { bool changed = ControlPaint.FontToIFont(lastFont, nativeFont); if (changed) { // here, we want to pick up a new font from the handle lastFont = null; ConvertNativeToManaged(nativeFont, pd); } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
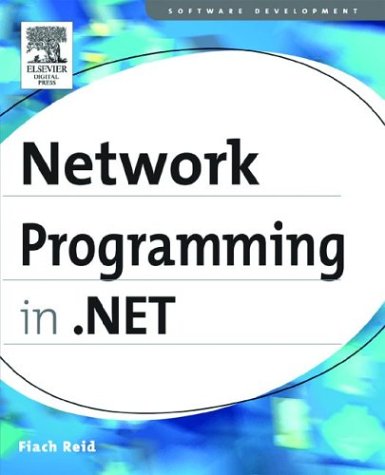
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerialReceived.cs
- SqlFunctionAttribute.cs
- TypeDescriptionProvider.cs
- NonVisualControlAttribute.cs
- XmlNodeChangedEventManager.cs
- XmlLanguage.cs
- UIElementCollection.cs
- CollectionViewGroup.cs
- ParameterModifier.cs
- HtmlTableRowCollection.cs
- TypeUsage.cs
- AddIn.cs
- CodeDirectoryCompiler.cs
- Helper.cs
- AppendHelper.cs
- VariableAction.cs
- LifetimeServices.cs
- CodeParameterDeclarationExpressionCollection.cs
- Canvas.cs
- LicFileLicenseProvider.cs
- DataServiceExpressionVisitor.cs
- DataGridViewColumnCollection.cs
- AutomationPatternInfo.cs
- DrawItemEvent.cs
- ConfigurationValue.cs
- StateWorkerRequest.cs
- KernelTypeValidation.cs
- FlatButtonAppearance.cs
- HtmlHistory.cs
- PropertyInformationCollection.cs
- SqlFunctionAttribute.cs
- CursorConverter.cs
- PasswordDeriveBytes.cs
- FrameworkElement.cs
- MaxValueConverter.cs
- TypeSystemProvider.cs
- ToolStripItemClickedEventArgs.cs
- XXXInfos.cs
- Brush.cs
- WebPart.cs
- HeaderedContentControl.cs
- ToolStripDropDown.cs
- MetricEntry.cs
- OleDbPropertySetGuid.cs
- BamlStream.cs
- DockPanel.cs
- ConstraintStruct.cs
- XmlLinkedNode.cs
- CompleteWizardStep.cs
- TypeExtension.cs
- FontDifferentiator.cs
- COM2FontConverter.cs
- LOSFormatter.cs
- RootBrowserWindowAutomationPeer.cs
- DataTableClearEvent.cs
- PersonalizationAdministration.cs
- TextChangedEventArgs.cs
- BufferAllocator.cs
- nulltextnavigator.cs
- SequenceQuery.cs
- Aes.cs
- NotSupportedException.cs
- DesignerCategoryAttribute.cs
- ApplicationId.cs
- TimeManager.cs
- DesignerLoader.cs
- GetTokenRequest.cs
- XmlExtensionFunction.cs
- Scene3D.cs
- DataControlFieldCell.cs
- TopClause.cs
- CodeNamespaceImport.cs
- StateWorkerRequest.cs
- Opcode.cs
- PrinterUnitConvert.cs
- TextTrailingWordEllipsis.cs
- DataControlField.cs
- XPathExpr.cs
- ClientCultureInfo.cs
- SiteMapDataSourceView.cs
- OperationFormatStyle.cs
- ListChangedEventArgs.cs
- NativeMethods.cs
- RegistryExceptionHelper.cs
- Renderer.cs
- ListItemsPage.cs
- XmlChildNodes.cs
- ModuleBuilder.cs
- EmbeddedMailObjectCollectionEditor.cs
- ValidationService.cs
- SqlMethodCallConverter.cs
- FocusManager.cs
- Crc32.cs
- ContentElement.cs
- ClickablePoint.cs
- DataGridViewCellStateChangedEventArgs.cs
- KeyedByTypeCollection.cs
- UnsafeNetInfoNativeMethods.cs
- AnnotationComponentManager.cs
- ColorTransform.cs