Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities.DurableInstancing / System / Activities / DurableInstancing / SqlWorkflowInstanceStoreLock.cs / 1305376 / SqlWorkflowInstanceStoreLock.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.DurableInstancing { using System; using System.Collections.Generic; using System.Linq; using System.Runtime; using System.Runtime.DurableInstancing; using System.Threading; class SqlWorkflowInstanceStoreLock { bool isBeingModified; Guid lockOwnerId; SqlWorkflowInstanceStore sqlWorkflowInstanceStore; WeakReference lockOwnerInstanceHandle; object thisLock; public SqlWorkflowInstanceStoreLock(SqlWorkflowInstanceStore sqlWorkflowInstanceStore) { this.sqlWorkflowInstanceStore = sqlWorkflowInstanceStore; this.thisLock = new object(); this.SurrogateLockOwnerId = -1; } public PersistenceTask InstanceDetectionTask { get; set; } public bool IsValid { get { return IsLockOwnerValid(this.SurrogateLockOwnerId); } } public bool IsLockOwnerValid(long surrogateLockOwnerId) { return (this.SurrogateLockOwnerId != -1) && (surrogateLockOwnerId == this.SurrogateLockOwnerId) && (this.sqlWorkflowInstanceStore.InstanceOwnersExist); } public Guid LockOwnerId { get { return this.lockOwnerId; } } public PersistenceTask LockRecoveryTask { get; set; } public PersistenceTask LockRenewalTask { get; set; } public long SurrogateLockOwnerId { get; private set; } object ThisLock { get { return this.thisLock; } } public void MarkInstanceOwnerCreated(Guid lockOwnerId, long surrogateLockOwnerId, InstanceHandle lockOwnerInstanceHandle, bool detectRunnableInstances, bool detectActivatableInstances) { Fx.Assert(this.isBeingModified, "Must have modification lock to mark owner as created"); this.lockOwnerId = lockOwnerId; this.SurrogateLockOwnerId = surrogateLockOwnerId; this.lockOwnerInstanceHandle = new WeakReference(lockOwnerInstanceHandle); TimeSpan hostLockRenewalPeriod = this.sqlWorkflowInstanceStore.HostLockRenewalPeriod; TimeSpan runnableInstancesDetectionPeriod = this.sqlWorkflowInstanceStore.RunnableInstancesDetectionPeriod; if (detectActivatableInstances) { this.InstanceDetectionTask = new DetectActivatableWorkflowsTask(this.sqlWorkflowInstanceStore, this, runnableInstancesDetectionPeriod); } else if (detectRunnableInstances) { this.InstanceDetectionTask = new DetectRunnableInstancesTask(this.sqlWorkflowInstanceStore, this, runnableInstancesDetectionPeriod); } this.LockRenewalTask = new LockRenewalTask(this.sqlWorkflowInstanceStore, this, hostLockRenewalPeriod); this.LockRecoveryTask = new LockRecoveryTask(this.sqlWorkflowInstanceStore, this, hostLockRenewalPeriod); if (this.InstanceDetectionTask != null) { this.InstanceDetectionTask.ResetTimer(true); } this.LockRenewalTask.ResetTimer(true); this.LockRecoveryTask.ResetTimer(true); } public void MarkInstanceOwnerLost(long surrogateLockOwnerId, bool hasModificationLock) { if (hasModificationLock) { this.MarkInstanceOwnerLost(surrogateLockOwnerId); } else { this.TakeModificationLock(); this.MarkInstanceOwnerLost(surrogateLockOwnerId); this.ReturnModificationLock(); } } public void ReturnModificationLock() { Fx.Assert(this.isBeingModified, "Must have modification lock to release it!"); bool lockTaken = false; while (true) { Monitor.Enter(ThisLock, ref lockTaken); if (lockTaken) { this.isBeingModified = false; Monitor.Pulse(ThisLock); Monitor.Exit(ThisLock); return; } } } public void TakeModificationLock() { bool lockTaken = false; while (true) { Monitor.Enter(ThisLock, ref lockTaken); if (lockTaken) { while (this.isBeingModified) { Monitor.Wait(ThisLock); } this.isBeingModified = true; Monitor.Exit(ThisLock); return; } } } void MarkInstanceOwnerLost(long surrogateLockOwnerId) { Fx.Assert(this.isBeingModified, "Must have modification lock to mark owner as lost"); if (this.SurrogateLockOwnerId == surrogateLockOwnerId) { this.SurrogateLockOwnerId = -1; InstanceHandle instanceHandle = this.lockOwnerInstanceHandle.Target as InstanceHandle; if (instanceHandle != null) { instanceHandle.Free(); } if (this.sqlWorkflowInstanceStore.IsLockRetryEnabled()) { this.sqlWorkflowInstanceStore.LoadRetryHandler.AbortPendingRetries(); } if (this.LockRenewalTask != null) { this.LockRenewalTask.CancelTimer(); } if (this.LockRecoveryTask != null) { this.LockRecoveryTask.CancelTimer(); } if (this.InstanceDetectionTask != null) { this.InstanceDetectionTask.CancelTimer(); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
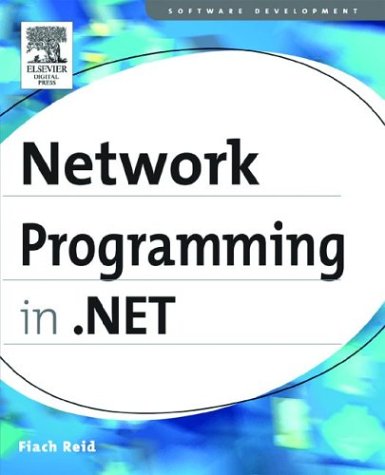
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ElapsedEventArgs.cs
- JobInputBins.cs
- SpeechDetectedEventArgs.cs
- InProcStateClientManager.cs
- DBDataPermissionAttribute.cs
- MemoryStream.cs
- Wizard.cs
- SafeReadContext.cs
- XPathEmptyIterator.cs
- SoapEnumAttribute.cs
- MachineKeySection.cs
- InfoCardMetadataExchangeClient.cs
- Win32Exception.cs
- AtomServiceDocumentSerializer.cs
- Button.cs
- DoubleAverageAggregationOperator.cs
- EventRouteFactory.cs
- ScalarRestriction.cs
- FacetEnabledSchemaElement.cs
- SqlResolver.cs
- Models.cs
- DesignTimeParseData.cs
- StyleReferenceConverter.cs
- ChildDocumentBlock.cs
- ProfilePropertySettingsCollection.cs
- ExpressionNormalizer.cs
- ListManagerBindingsCollection.cs
- LowerCaseStringConverter.cs
- TextContainerHelper.cs
- GlyphingCache.cs
- MeshGeometry3D.cs
- DataGridLinkButton.cs
- StorageInfo.cs
- OperandQuery.cs
- ListParagraph.cs
- Repeater.cs
- CultureTable.cs
- IntSecurity.cs
- TextRangeEditLists.cs
- OracleParameter.cs
- QueryInterceptorAttribute.cs
- AddingNewEventArgs.cs
- StrokeNodeEnumerator.cs
- TypeResolvingOptionsAttribute.cs
- FormViewAutoFormat.cs
- DataGridColumnHeader.cs
- Win32Native.cs
- ResXResourceWriter.cs
- RelatedEnd.cs
- BlockUIContainer.cs
- PackageRelationship.cs
- unsafenativemethodsother.cs
- Adorner.cs
- BitStack.cs
- PropertyMetadata.cs
- PropertyCondition.cs
- ActivityExecutionWorkItem.cs
- SendActivityEventArgs.cs
- SspiWrapper.cs
- NativeMethodsOther.cs
- TreeViewBindingsEditor.cs
- UrlAuthFailedErrorFormatter.cs
- TextDecorationUnitValidation.cs
- ColumnMapTranslator.cs
- BaseCollection.cs
- DataErrorValidationRule.cs
- ListMarkerSourceInfo.cs
- Rect.cs
- WindowsTooltip.cs
- ScrollChrome.cs
- LinqDataSourceUpdateEventArgs.cs
- Version.cs
- HierarchicalDataSourceDesigner.cs
- SmtpAuthenticationManager.cs
- WindowsClaimSet.cs
- GridViewItemAutomationPeer.cs
- SafeEventLogWriteHandle.cs
- CodeValidator.cs
- XmlSchemaType.cs
- PersonalizationStateInfoCollection.cs
- TraceSource.cs
- PageVisual.cs
- TextRangeEditLists.cs
- Keyboard.cs
- Section.cs
- BehaviorEditorPart.cs
- ClientRuntimeConfig.cs
- VectorAnimation.cs
- PipeStream.cs
- SessionPageStateSection.cs
- ReaderWriterLock.cs
- GlyphRunDrawing.cs
- COAUTHIDENTITY.cs
- Base64Stream.cs
- RegexMatch.cs
- NameValuePermission.cs
- ModuleBuilder.cs
- Win32MouseDevice.cs
- TraceHelpers.cs
- PageTrueTypeFont.cs