Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / DoubleAverageAggregationOperator.cs / 1305376 / DoubleAverageAggregationOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // DoubleAverageAggregationOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined average aggregation operator and its enumerator, for doubles. /// internal sealed class DoubleAverageAggregationOperator : InlinedAggregationOperator, double> { //---------------------------------------------------------------------------------------- // Constructs a new instance of an average associative operator. // internal DoubleAverageAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override double InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator > enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // Throw an error for empty results. if (!enumerator.MoveNext()) { singularExceptionToThrow = new InvalidOperationException(SR.GetString(SR.NoElements)); return default(double); } Pair result = enumerator.Current; // Simply add together the sums and totals. while (enumerator.MoveNext()) { checked { result.First += enumerator.Current.First; result.Second += enumerator.Current.Second; } } // And divide the sum by the total to obtain the final result. return result.First / result.Second; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator , int> CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new DoubleAverageAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class DoubleAverageAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator > { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal DoubleAverageAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the average of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref Pair currentElement) { // The temporary result contains the running sum and count, respectively. double sum = 0.0; long count = 0; QueryOperatorEnumerator source = m_source; double current = default(double); TKey keyUnused = default(TKey); if (source.MoveNext(ref current, ref keyUnused)) { int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); checked { sum += current; count++; } } while (source.MoveNext(ref current, ref keyUnused)); currentElement = new Pair (sum, count); return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // DoubleAverageAggregationOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined average aggregation operator and its enumerator, for doubles. /// internal sealed class DoubleAverageAggregationOperator : InlinedAggregationOperator, double> { //---------------------------------------------------------------------------------------- // Constructs a new instance of an average associative operator. // internal DoubleAverageAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override double InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator > enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // Throw an error for empty results. if (!enumerator.MoveNext()) { singularExceptionToThrow = new InvalidOperationException(SR.GetString(SR.NoElements)); return default(double); } Pair result = enumerator.Current; // Simply add together the sums and totals. while (enumerator.MoveNext()) { checked { result.First += enumerator.Current.First; result.Second += enumerator.Current.Second; } } // And divide the sum by the total to obtain the final result. return result.First / result.Second; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator , int> CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new DoubleAverageAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class DoubleAverageAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator > { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal DoubleAverageAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the average of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref Pair currentElement) { // The temporary result contains the running sum and count, respectively. double sum = 0.0; long count = 0; QueryOperatorEnumerator source = m_source; double current = default(double); TKey keyUnused = default(TKey); if (source.MoveNext(ref current, ref keyUnused)) { int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); checked { sum += current; count++; } } while (source.MoveNext(ref current, ref keyUnused)); currentElement = new Pair (sum, count); return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
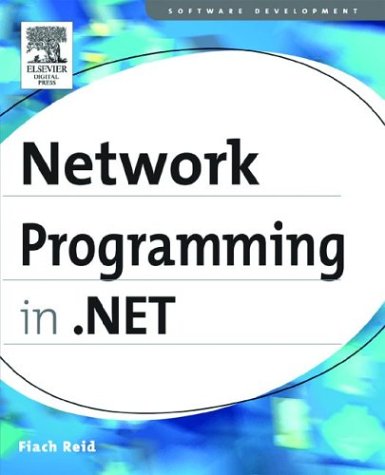
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TerminateDesigner.cs
- SelectionRangeConverter.cs
- HighlightVisual.cs
- BidOverLoads.cs
- HelpKeywordAttribute.cs
- SystemIPInterfaceStatistics.cs
- CompensateDesigner.cs
- WSSecurityPolicy12.cs
- TreeNodeBinding.cs
- HandledEventArgs.cs
- WorkItem.cs
- Point4DConverter.cs
- XmlBoundElement.cs
- RegistrationServices.cs
- SoapClientMessage.cs
- HtmlInputHidden.cs
- WebPartConnectionsCloseVerb.cs
- ComponentEditorPage.cs
- RightNameExpirationInfoPair.cs
- FixedSOMImage.cs
- SqlSelectClauseBuilder.cs
- XamlPathDataSerializer.cs
- EncryptedType.cs
- AnimationClockResource.cs
- NumberFunctions.cs
- FormViewPagerRow.cs
- XPathQilFactory.cs
- DataGridViewCellLinkedList.cs
- AllMembershipCondition.cs
- webproxy.cs
- WasAdminWrapper.cs
- MetadataCache.cs
- ListView.cs
- XmlIlVisitor.cs
- DataColumn.cs
- BlobPersonalizationState.cs
- validationstate.cs
- MultiAsyncResult.cs
- StructuralObject.cs
- Funcletizer.cs
- HijriCalendar.cs
- WmlControlAdapter.cs
- ButtonDesigner.cs
- AttributeSetAction.cs
- DisplayInformation.cs
- Overlapped.cs
- UnsafeNativeMethods.cs
- ChtmlSelectionListAdapter.cs
- SrgsGrammar.cs
- ProgressBar.cs
- SequentialOutput.cs
- ObjectTypeMapping.cs
- PeerConnector.cs
- RadioButtonAutomationPeer.cs
- EtwTrace.cs
- EditorResources.cs
- Int32CollectionConverter.cs
- TracedNativeMethods.cs
- BindingExpressionUncommonField.cs
- RuntimeConfigLKG.cs
- WebBrowserHelper.cs
- BamlRecordWriter.cs
- LightweightCodeGenerator.cs
- Listbox.cs
- Stack.cs
- OrthographicCamera.cs
- SafeWaitHandle.cs
- IntegerValidator.cs
- OutputScopeManager.cs
- BinaryWriter.cs
- RoutedEventValueSerializer.cs
- ActivityStatusChangeEventArgs.cs
- DbXmlEnabledProviderManifest.cs
- BitmapFrame.cs
- PartialCachingControl.cs
- AssemblyBuilder.cs
- DataSourceXmlSerializationAttribute.cs
- MenuEventArgs.cs
- XmlSchemaAnnotation.cs
- EmissiveMaterial.cs
- UrlPath.cs
- EncoderNLS.cs
- Speller.cs
- SqlUserDefinedAggregateAttribute.cs
- ResetableIterator.cs
- WmfPlaceableFileHeader.cs
- WebPartVerbsEventArgs.cs
- SyndicationCategory.cs
- TreeNodeEventArgs.cs
- FlowDocumentReader.cs
- BindingBase.cs
- TextParagraphCache.cs
- ToolStripDropDownButton.cs
- CompositeScriptReferenceEventArgs.cs
- ObjectDataSourceMethodEventArgs.cs
- Base64Decoder.cs
- CommandBindingCollection.cs
- ModelItemKeyValuePair.cs
- CodeNamespaceImportCollection.cs
- TextDecorationCollectionConverter.cs