Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Shared / MS / Win32 / unsafenativemethodsother.cs / 2 / unsafenativemethodsother.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Threading; using System.ComponentModel; using System.Diagnostics; namespace MS.Win32 { using Accessibility; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System; using System.Security.Permissions; using System.Collections; using System.IO; using System.Text; using System.Security; using Microsoft.Win32.SafeHandles; #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif DRT using MS.Internal.Drt; #else #error Attempt to use FriendAccessAllowedAttribute from an unknown assembly. using MS.Internal.YourAssemblyName; #endif using IComDataObject = System.Runtime.InteropServices.ComTypes.IDataObject; //[SuppressUnmanagedCodeSecurity()] [FriendAccessAllowed] internal partial class UnsafeNativeMethods { ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport("kernel32.dll", CharSet=CharSet.Auto, SetLastError=true, EntryPoint="GetTempFileName")] internal static extern uint _GetTempFileName(string tmpPath, string prefix, uint uniqueIdOrZero, StringBuilder tmpFileName); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical] internal static uint GetTempFileName(string tmpPath, string prefix, uint uniqueIdOrZero, StringBuilder tmpFileName) { uint result = _GetTempFileName(tmpPath, prefix, uniqueIdOrZero, tmpFileName); if (result == 0) { throw new Win32Exception(); } return result; } ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.NaturalLanguage6, PreserveSig = false, EntryPoint = "DllGetClassObject")] internal static extern void NaturalLanguageDllGetClassObject(ref Guid clsid, ref Guid iid, [MarshalAs(UnmanagedType.Interface)] out object classObject); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Shell32, CharSet = System.Runtime.InteropServices.CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern int ExtractIconEx( string stExeFileName, int nIconIndex, ref NativeMethods.IconHandle phiconLarge, ref NativeMethods.IconHandle phiconSmall, int nIcons); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError=true)] internal static extern NativeMethods.IconHandle CreateIcon(IntPtr hInstance, int nWidth, int nHeight, byte cPlanes, byte cBitsPixel, byte[] lpbANDbits, byte[] lpbXORbits); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = CharSet.Auto)] public static extern bool CreateCaret(HandleRef hwnd, NativeMethods.BitmapHandle hbitmap, int width, int height); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = CharSet.Auto)] public static extern bool ShowCaret(HandleRef hwnd); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern bool ShowWindowAsync(HandleRef hWnd, int nCmdShow); [DllImport(ExternDll.User32, EntryPoint="LoadImage", CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern NativeMethods.IconHandle LoadImageIcon(IntPtr hinst, string stName, int nType, int cxDesired, int cyDesired, int nFlags); [DllImport(ExternDll.User32, EntryPoint="LoadImage", CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern NativeMethods.CursorHandle LoadImageCursor(IntPtr hinst, string stName, int nType, int cxDesired, int cyDesired, int nFlags); // uncomment this if you plan to use LoadImage to load anything other than Icons/Cursors. /* [DllImport(ExternDll.User32, CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern SafeHandle LoadImage( IntPtr hinst, string stName, int nType, int cxDesired, int cyDesired, int nFlags); */ /* [DllImport(ExternDll.User32, CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern NativeMethods.IconHandle LoadImage( IntPtr hinst, string stName, int nType, int cxDesired, int cyDesired, int nFlags); */ ////// Critical - performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Urlmon, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Ansi, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern int ObtainUserAgentString(int dwOption, StringBuilder userAgent, ref int length); ////// Critical - performs an elevation. /// /// Could be a candidate for safe - as the only information disclosed is whether /// a certain security measure is on or off. /// Likely this determination could be made by trying certain actions and failing. /// [SecurityCritical, SuppressUnmanagedCodeSecurity ] [DllImport( ExternDll.Urlmon, ExactSpelling=true)] internal static extern int CoInternetIsFeatureEnabled( int featureEntry , int dwFlags ); ////// Critical - performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity ] [DllImport( ExternDll.Urlmon, ExactSpelling=true)] internal static extern int CoInternetSetFeatureEnabled( int featureEntry , int dwFlags, bool fEnable ); ////// Critical - performs an elevation. /// /// Could be a candidate for safe - as the only information disclosed is whether /// a certain security measure is on or off. /// Likely this determination could be made by trying certain actions and failing. /// [SecurityCritical, SuppressUnmanagedCodeSecurity ] [DllImport( ExternDll.Urlmon, ExactSpelling=true)] internal static extern int CoInternetIsFeatureZoneElevationEnabled( [MarshalAs(UnmanagedType.LPWStr)] string szFromURL, [MarshalAs(UnmanagedType.LPWStr)] string szToURL, UnsafeNativeMethods.IInternetSecurityManager secMgr, int dwFlags ); ////// Critical - call is SUC'ed /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.PresentationHostDll, EntryPoint = "ProcessUnhandledException")] internal static extern void ProcessUnhandledException_DLL([MarshalAs(UnmanagedType.BStr)] string errMsg); ////// Critical - performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity ] [DllImport(ExternDll.Kernel32, CharSet=CharSet.Unicode)] internal static extern bool GetVersionEx([In, Out] NativeMethods.OSVERSIONINFOEX ver); ////// Critical - performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity ] [DllImport( ExternDll.Urlmon, ExactSpelling=true)] internal static extern int CoInternetCreateSecurityManager( [MarshalAs(UnmanagedType.Interface)] object pIServiceProvider, [MarshalAs(UnmanagedType.Interface)] out object ppISecurityManager , int dwReserved ) ; [ComImport, ComVisible(false), Guid("79eac9ee-baf9-11ce-8c82-00aa004ba90b"), System.Runtime.InteropServices.InterfaceType(ComInterfaceType.InterfaceIsIUnknown)] internal interface IInternetSecurityManager { void SetSecuritySite( NativeMethods.IInternetSecurityMgrSite pSite); unsafe void GetSecuritySite( /* [out] */ void **ppSite); ////// Critical - performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] void MapUrlToZone( [In, MarshalAs(UnmanagedType.BStr)] string pwszUrl, [Out] out int pdwZone, [In] int dwFlags); unsafe void GetSecurityId( /* [in] */ string pwszUrl, /* [size_is][out] */ byte *pbSecurityId, /* [out][in] */ int *pcbSecurityId, /* [in] */ int dwReserved); unsafe void ProcessUrlAction( /* [in] */ string pwszUrl, /* [in] */ int dwAction, /* [size_is][out] */ byte *pPolicy, /* [in] */ int cbPolicy, /* [in] */ byte *pContext, /* [in] */ int cbContext, /* [in] */ int dwFlags, /* [in] */ int dwReserved); unsafe void QueryCustomPolicy( /* [in] */ string pwszUrl, /* [in] */ /*REFGUID*/ void *guidKey, /* [size_is][size_is][out] */ byte **ppPolicy, /* [out] */ int *pcbPolicy, /* [in] */ byte *pContext, /* [in] */ int cbContext, /* [in] */ int dwReserved); unsafe void SetZoneMapping( /* [in] */ int dwZone, /* [in] */ string lpszPattern, /* [in] */ int dwFlags); unsafe void GetZoneMappings( /* [in] */ int dwZone, /* [out] */ /*IEnumString*/ void **ppenumString, /* [in] */ int dwFlags); } ////// /// /// ///[DllImport("kernel32.dll", SetLastError = true), ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static extern IntPtr LocalFree(IntPtr hMem); #if BASE_NATIVEMETHODS /// /// SecurityCritical: This code returns a critical resource obtained under an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal unsafe static extern SafeFileHandle CreateFile( string lpFileName, uint dwDesiredAccess, uint dwShareMode, [In] NativeMethods.SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile); #endif #if BASE_NATIVEMETHODS ////// Critical: This code is critical because it can be used to /// pass and force arbitrary data into the tree. We should /// consider yanking it out all the way /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet = CharSet.Auto)] internal static extern IntPtr GetMessageExtraInfo(); #endif #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, EntryPoint="WaitForMultipleObjectsEx", SetLastError = true, CharSet = CharSet.Auto)] private static extern int IntWaitForMultipleObjectsEx(int nCount, IntPtr[] pHandles, bool bWaitAll, int dwMilliseconds, bool bAlertable); public const int WAIT_FAILED = unchecked((int)0xFFFFFFFF); ////// Critical - calls IntWaitForMultipleObjectsEx (the real PInvoke method) /// [SecurityCritical] internal static int WaitForMultipleObjectsEx(int nCount, IntPtr[] pHandles, bool bWaitAll, int dwMilliseconds, bool bAlertable) { int result = IntWaitForMultipleObjectsEx(nCount, pHandles, bWaitAll, dwMilliseconds, bAlertable); if(result == UnsafeNativeMethods.WAIT_FAILED) { throw new Win32Exception(); } return result; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="MsgWaitForMultipleObjectsEx", SetLastError=true, ExactSpelling = true, CharSet = CharSet.Auto)] private static extern int IntMsgWaitForMultipleObjectsEx(int nCount, IntPtr[] pHandles, int dwMilliseconds, int dwWakeMask, int dwFlags); ////// Critical - calls IntMsgWaitForMultipleObjectsEx (the real PInvoke method) /// [SecurityCritical] internal static int MsgWaitForMultipleObjectsEx(int nCount, IntPtr[] pHandles, int dwMilliseconds, int dwWakeMask, int dwFlags) { int result = IntMsgWaitForMultipleObjectsEx(nCount, pHandles, dwMilliseconds, dwWakeMask, dwFlags); if(result == -1) { throw new Win32Exception(); } return result; } #endif ////// Critical: This code elevates to unmanaged code permission /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="RegisterClassEx", CharSet=CharSet.Auto, SetLastError=true, BestFitMapping=false)] internal static extern UInt16 IntRegisterClassEx(NativeMethods.WNDCLASSEX_D wc_d); ////// Critical - calls IntRegisterClassEx (the real PInvoke method) /// [SecurityCritical] internal static UInt16 RegisterClassEx(NativeMethods.WNDCLASSEX_D wc_d) { UInt16 result = IntRegisterClassEx(wc_d); if(result == 0) { throw new Win32Exception(); } return result; } ////// Critical: This code elevates to unmanaged code permission /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="UnregisterClass",CharSet = CharSet.Auto, SetLastError = true, BestFitMapping=false)] internal static extern int IntUnregisterClass(IntPtr atomString /*lpClassName*/ , IntPtr hInstance); ////// Critical - calls IntUnregisterClass (the real PInvoke method) /// [SecurityCritical] internal static void UnregisterClass(IntPtr atomString /*lpClassName*/ , IntPtr hInstance) { int result = IntUnregisterClass(atomString, hInstance); if (result == 0) { throw new Win32Exception(); } } // note that this method exists in UnsafeNativeMethodsCLR.cs but with a different signature // using a HandleRef for the hWnd instead of an IntPtr, and not using an IntPtr for lParam [DllImport(ExternDll.User32, CharSet = CharSet.Auto)] internal static extern IntPtr SendMessage(IntPtr hWnd, int msg, IntPtr wParam, IntPtr lParam); // note that this method exists in UnsafeNativeMethodsCLR.cs but with a different signature // using a HandleRef for the hWnd instead of an IntPtr, and not using an IntPtr for lParam ////// Critical: This code has the ability to send a message to the wndproc. It exists purely for /// the secure close scenario. For any other scenario please use the SendMessage call /// [DllImport(ExternDll.User32,EntryPoint="SendMessage", CharSet = CharSet.Auto)] [SecurityCritical,SuppressUnmanagedCodeSecurity] internal static extern IntPtr UnsafeSendMessage(IntPtr hWnd, int msg, IntPtr wParam, IntPtr lParam); /* // // SendMessage taking a SafeHandle for wParam. Needed by some Win32 messages. e.g. WM_PRINT // [DllImport(ExternDll.User32, CharSet = CharSet.Auto)] internal static extern IntPtr SendMessage(HandleRef hWnd, int msg, SafeHandle wParam, IntPtr lParam); */ // private DllImport - that takes an IconHandle. ////// Critical: This code causes elevation to unmanaged code /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet = CharSet.Auto, SetLastError = true)] internal static extern IntPtr SendMessage( HandleRef hWnd, int msg, IntPtr wParam, NativeMethods.IconHandle iconHandle ); ////// Critical: This code causes elevation to unmanaged code /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto)] internal static extern void SetLastError(int dwErrorCode); #if BASE_NATIVEMETHODS || CORE_NATIVEMETHODS || FRAMEWORK_NATIVEMETHODS ////// Win32 GetLayeredWindowAttributes. /// /// /// /// /// ////// /// Critical: This code calls into unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport("user32.dll")] public static extern bool GetLayeredWindowAttributes( HandleRef hwnd, IntPtr pcrKey, IntPtr pbAlpha, IntPtr pdwFlags); internal sealed class SafeFileMappingHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Critical: base class enforces link demand and inheritance demand /// TreatAsSafe: Creating this is ok, accessing the pointer is bad /// [SecurityCritical,SecurityTreatAsSafe] internal SafeFileMappingHandle() : base(true) { } ////// Critical: base class enforces link demand and inheritance demand /// TreatAsSafe: This call is safe /// public override bool IsInvalid { [SecurityCritical,SecurityTreatAsSafe] get { return handle == IntPtr.Zero; } } ////// Critical - as this function does an elevation to close a handle. /// TreatAsSafe - as this can at best be used to destabilize one's own app. /// [SecurityCritical, SecurityTreatAsSafe] protected override bool ReleaseHandle() { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); try { return CloseHandleNoThrow(new HandleRef(null, handle)); } finally { SecurityPermission.RevertAssert(); } } } internal sealed class SafeViewOfFileHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Critical: This code calls into a base class which link demands for unmanaged code /// TreatAsSafe:Creating this is ok it is acessing the pointers in it that can be risky /// [SecurityCritical,SecurityTreatAsSafe] internal SafeViewOfFileHandle() : base(true) { } ////// Critical: This code accesses an unsafe object (pointer) and returns it as a pointer /// internal unsafe void* Memory { [SecurityCritical] get { Debug.Assert(handle != IntPtr.Zero); return (void*)handle; } } ////// Critical - as this function does an elevation to close a handle. /// TreatAsSafe - as this can at best be used to destabilize one's own app. /// [SecurityCritical, SecurityTreatAsSafe] override protected bool ReleaseHandle() { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); try { return UnsafeNativeMethods.UnmapViewOfFileNoThrow(new HandleRef(null, handle)); } finally { SecurityPermission.RevertAssert(); } } } ////// SecurityCritical: This code returns critical resource obtained under an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport("kernel32.dll", SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal unsafe static extern SafeFileMappingHandle CreateFileMapping(SafeFileHandle hFile, NativeMethods.SECURITY_ATTRIBUTES lpFileMappingAttributes, int flProtect, uint dwMaximumSizeHigh, uint dwMaximumSizeLow, string lpName); ////// SecurityCritical: This code returns a critical resource obtained under an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, SetLastError = true)] internal static extern SafeViewOfFileHandle MapViewOfFileEx(SafeFileMappingHandle hFileMappingObject, int dwDesiredAccess, int dwFileOffsetHigh, int dwFileOffsetLow, IntPtr dwNumberOfBytesToMap, IntPtr lpBaseAddress); #endif // BASE_NATIVEMETHODS [DllImport(ExternDll.User32, EntryPoint="SetWindowLongPtr", CharSet=CharSet.Auto)] private static extern IntPtr IntSetWindowLongPtr(HandleRef hWnd, int nIndex, IntPtr dwNewLong); [DllImport(ExternDll.User32, EntryPoint="SetWindowLong", CharSet=CharSet.Auto)] private static extern Int32 IntSetWindowLong(HandleRef hWnd, int nIndex, Int32 dwNewLong); ////// Critical: LinkDemand on Marshal.GetLastWin32Error /// TreatAsSafe: Getting an error code isn't unsafe /// Note: If a SupressUnmanagedCodeSecurity attribute is ever added to IntsetWindowLong(Ptr), we'd need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] internal static IntPtr SetWindowLong(HandleRef hWnd, int nIndex, IntPtr dwNewLong) { IntPtr result = IntPtr.Zero; if (IntPtr.Size == 4) { // use SetWindowLong Int32 tempResult = IntSetWindowLong(hWnd, nIndex, NativeMethods.IntPtrToInt32(dwNewLong)); result = new IntPtr(tempResult); } else { // use SetWindowLongPtr result = IntSetWindowLongPtr(hWnd, nIndex, dwNewLong); } return result; } ////// Critical - link demands for UnmanagedCode permissions /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="SetWindowLongPtr", CharSet=CharSet.Auto)] private static extern IntPtr IntCriticalSetWindowLongPtr(HandleRef hWnd, int nIndex, IntPtr dwNewLong); [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="SetWindowLong", CharSet=CharSet.Auto)] private static extern Int32 IntCriticalSetWindowLong(HandleRef hWnd, int nIndex, Int32 dwNewLong); ////// Critical - it calls IntCriticalSetWindowLongPtr() / IntCriticalSetWindowLong(), which are Critical /// [SecurityCritical] internal static IntPtr CriticalSetWindowLong(HandleRef hWnd, int nIndex, IntPtr dwNewLong) { IntPtr result = IntPtr.Zero; if (IntPtr.Size == 4) { // use SetWindowLong Int32 tempResult = IntCriticalSetWindowLong(hWnd, nIndex, NativeMethods.IntPtrToInt32(dwNewLong)); result = new IntPtr(tempResult); } else { // use SetWindowLongPtr result = IntCriticalSetWindowLongPtr(hWnd, nIndex, dwNewLong); } return result; } ////// Critical - This link demands for UnmanagedCode permissions /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="SetWindowLongPtr", CharSet=CharSet.Auto, SetLastError=true)] private static extern IntPtr IntCriticalSetWindowLongPtr(HandleRef hWnd, int nIndex, NativeMethods.WndProc dwNewLong); [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="SetWindowLong", CharSet=CharSet.Auto, SetLastError=true)] private static extern Int32 IntCriticalSetWindowLong(HandleRef hWnd, int nIndex, NativeMethods.WndProc dwNewLong); ////// Critical - This calls SetLatError() and IntCriticalSetWindowLongPtr() / IntCriticalSetWindowLong(), which are Critical /// [SecurityCritical] internal static IntPtr CriticalSetWindowLong(HandleRef hWnd, int nIndex, NativeMethods.WndProc dwNewLong) { int errorCode; IntPtr retVal; SetLastError(0); // Win32 SetWindowLong doesn't clear error on success if (IntPtr.Size == 4) { Int32 tempRetVal = IntCriticalSetWindowLong(hWnd, nIndex, dwNewLong); errorCode = Marshal.GetLastWin32Error(); retVal = new IntPtr(tempRetVal); } else { retVal = IntCriticalSetWindowLongPtr(hWnd, nIndex, dwNewLong); errorCode = Marshal.GetLastWin32Error(); } if (retVal == IntPtr.Zero) { if (errorCode != 0) { throw new System.ComponentModel.Win32Exception(errorCode); } } return retVal; } ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="GetWindowLongPtr", CharSet=CharSet.Auto, SetLastError=true)] private static extern IntPtr IntGetWindowLongPtr(HandleRef hWnd, int nIndex); ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="GetWindowLong", CharSet=CharSet.Auto, SetLastError=true)] private static extern Int32 IntGetWindowLong(HandleRef hWnd, int nIndex ); ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical] internal static IntPtr GetWindowLongPtr(HandleRef hWnd, int nIndex ) { IntPtr result = IntPtr.Zero; int error = 0; SetLastError(0); // Win32 GetWindowLong doesn't clear error on success if (IntPtr.Size == 4) { // use getWindowLong Int32 tempResult = IntGetWindowLong(hWnd, nIndex); error = Marshal.GetLastWin32Error(); result = new IntPtr(tempResult); } else { // use GetWindowLongPtr result = IntGetWindowLongPtr( hWnd, nIndex); error = Marshal.GetLastWin32Error(); } if ((result == IntPtr.Zero) && (error != 0)) { // To be consistent with out other PInvoke wrappers // we should "throw" here. But we don't want to // introduce new "throws" w/o time to follow up on any // new problems that causes. Debug.WriteLine("GetWindowLongPtr failed. Error = " + error); // throw new System.ComponentModel.Win32Exception(error); } return result; } ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical] internal static Int32 GetWindowLong(HandleRef hWnd, int nIndex ) { int iResult = 0; IntPtr result = IntPtr.Zero; int error = 0; SetLastError(0); // Win32 GetWindowLong doesn't clear error on success if (IntPtr.Size == 4) { // use GetWindowLong iResult = IntGetWindowLong(hWnd, nIndex); error = Marshal.GetLastWin32Error(); result = new IntPtr(iResult); } else { // use GetWindowLongPtr result = IntGetWindowLongPtr(hWnd, nIndex); error = Marshal.GetLastWin32Error(); iResult = NativeMethods.IntPtrToInt32(result); } if ((result == IntPtr.Zero) && (error != 0)) { // To be consistent with out other PInvoke wrappers // we should "throw" here. But we don't want to // introduce new "throws" w/o time to follow up on any // new problems that causes. Debug.WriteLine("GetWindowLong failed. Error = " + error); // throw new System.ComponentModel.Win32Exception(error); } return iResult; } ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="GetWindowLongPtr", CharSet=CharSet.Auto, SetLastError=true)] public static extern NativeMethods.WndProc IntGetWindowLongWndProcPtr(HandleRef hWnd, int nIndex); ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="GetWindowLong", CharSet=CharSet.Auto, SetLastError=true)] public static extern NativeMethods.WndProc IntGetWindowLongWndProc(HandleRef hWnd, int nIndex); ////// Critical: Call critical method IntGetWindowLongWndProc and IntGetWindowLongWndProcPtr that causes unmanaged code elevation. /// LinkDemand on Win32Exception constructor but throwing an exception isn't unsafe /// [SecurityCritical] internal static NativeMethods.WndProc GetWindowLongWndProc(HandleRef hWnd) { NativeMethods.WndProc returnValue = null; int error = 0; SetLastError(0); // Win32 GetWindowLong doesn't clear error on success if (IntPtr.Size == 4) { // use getWindowLong returnValue = IntGetWindowLongWndProc (hWnd, NativeMethods.GWL_WNDPROC); error = Marshal.GetLastWin32Error(); } else { // use GetWindowLongPtr returnValue = IntGetWindowLongWndProcPtr (hWnd, NativeMethods.GWL_WNDPROC); error = Marshal.GetLastWin32Error(); } if (null == returnValue) { throw new Win32Exception(error); } return returnValue; } ////// Critical - Unmanaged code permission is supressed. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport("winmm.dll", CharSet = CharSet.Unicode)] internal static extern bool PlaySound([In]string soundName, IntPtr hmod, SafeNativeMethods.PlaySoundFlags soundFlags); internal const uint INTERNET_COOKIE_THIRD_PARTY = 0x10, INTERNET_COOKIE_EVALUATE_P3P = 0x40, INTERNET_COOKIE_IS_RESTRICTED = 0x200, COOKIE_STATE_REJECT = 5; //!!! CAUTION // PresentationHost intercepts calls to InternetGetCookieEx & InternetSetCookieEx and delegates them // to the browser. It doesn't do this for InternetGetCookie & InternetSetCookie. // See also Application.Get/SetCookie(). //!!! ////// SecurityCritical - calls unmanaged code. /// [DllImport(ExternDll.Wininet, SetLastError=true, ExactSpelling=true, EntryPoint="InternetGetCookieExW", CharSet=CharSet.Unicode)] [SuppressUnmanagedCodeSecurity, SecurityCritical] internal static extern bool InternetGetCookieEx([In]string Url, [In]string cookieName, [Out] StringBuilder cookieData, [In, Out] ref UInt32 pchCookieData, uint flags, IntPtr reserved); ////// SecurityCritical - calls unmanaged code. /// [DllImport(ExternDll.Wininet, SetLastError = true, ExactSpelling = true, EntryPoint = "InternetSetCookieExW", CharSet = CharSet.Unicode)] [SuppressUnmanagedCodeSecurity] [SecurityCritical] internal static extern uint InternetSetCookieEx([In]string Url, [In]string CookieName, [In]string cookieData, uint flags, [In] string p3pHeader); #if DRT_NATIVEMETHODS [DllImport(ExternDll.User32, ExactSpelling = true, EntryPoint = "mouse_event", CharSet = CharSet.Auto)] internal static extern void Mouse_event(int flags, int dx, int dy, int dwData, IntPtr extrainfo); #endif ///////////////////////////// // needed by Framework ////// Critical - calls unmanaged code /// [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Unicode)] [SuppressUnmanagedCodeSecurity] [SecurityCritical] internal static extern int GetLocaleInfoW(int locale, int type, string data, int dataSize); ////// Critical - calls unmanaged code /// [DllImport(ExternDll.Kernel32, ExactSpelling = true, SetLastError = true)] [SuppressUnmanagedCodeSecurity] [SecurityCritical] internal static extern int FindNLSString(int locale, uint flags, [MarshalAs(UnmanagedType.LPWStr)]string sourceString, int sourceCount, [MarshalAs(UnmanagedType.LPWStr)]string findString, int findCount, out int found); //[DllImport(ExternDll.Psapi, SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false)] //public static extern int GetModuleFileNameEx(IntPtr hProcess, IntPtr hModule, StringBuilder buffer, int length); // // OpenProcess // public const int PROCESS_VM_READ = 0x0010; public const int PROCESS_QUERY_INFORMATION = 0x0400; //[DllImport(ExternDll.Kernel32, SetLastError = true, CharSet = CharSet.Auto)] //public static extern IntPtr OpenProcess(int dwDesiredAccess, bool fInherit, int dwProcessId); [DllImport(ExternDll.User32, EntryPoint = "SetWindowText", CharSet = CharSet.Auto, SetLastError = true, BestFitMapping = false)] private static extern bool IntSetWindowText(HandleRef hWnd, string text); ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// Note: If a SupressUnmanagedCodeSecurity attribute is ever added to IntSetWindowText, we'd need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] internal static void SetWindowText(HandleRef hWnd, string text) { if (IntSetWindowText(hWnd, text) == false) { throw new Win32Exception(); } } ////// Critical: This code calls into unmanaged code /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "GetIconInfo", CharSet = CharSet.Auto, SetLastError = true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] private static extern bool GetIconInfoImpl(HandleRef hIcon, [Out] ICONINFO_IMPL piconinfo); [StructLayout(LayoutKind.Sequential)] internal class ICONINFO_IMPL { public bool fIcon = false; public int xHotspot = 0; public int yHotspot = 0; public IntPtr hbmMask = IntPtr.Zero; public IntPtr hbmColor = IntPtr.Zero; } // FOR [SecurityCritical] internal static void GetIconInfo(HandleRef hIcon, out NativeMethods.ICONINFO piconinfo) { bool success = false; int error = 0; piconinfo = new NativeMethods.ICONINFO(); ICONINFO_IMPL iconInfoImpl = new ICONINFO_IMPL(); RuntimeHelpers.PrepareConstrainedRegions(); // Mark the following as special try { // Intentionally empty } finally { // This block won't be interrupted by certain runtime induced failures or thread abort success = GetIconInfoImpl(hIcon, iconInfoImpl); error = Marshal.GetLastWin32Error(); if (success) { piconinfo.hbmMask = new NativeMethods.BitmapHandle(iconInfoImpl.hbmMask, true /*own*/); piconinfo.hbmColor = new NativeMethods.BitmapHandle(iconInfoImpl.hbmColor, true /*own*/); piconinfo.fIcon = iconInfoImpl.fIcon; piconinfo.xHotspot = iconInfoImpl.xHotspot; piconinfo.yHotspot = iconInfoImpl.yHotspot; } } if(!success) { Debug.WriteLine("GetIconInfo failed. Error = " + error); throw new Win32Exception(); } } #if never [DllImport(ExternDll.User32, #if WIN64 EntryPoint="GetClassLongPtr", #endif CharSet = CharSet.Auto, SetLastError = true) ] internal static extern UInt32 GetClassLong(IntPtr hwnd, int nIndex); #endif [DllImport(ExternDll.User32, EntryPoint = "GetWindowPlacement", ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] private static extern bool IntGetWindowPlacement(HandleRef hWnd, ref NativeMethods.WINDOWPLACEMENT placement); // note: this method exists in UnsafeNativeMethodsCLR.cs, but that method does not have the if/throw implemntation ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// Note: If a SupressUnmanagedCodeSecurity attribute is ever added to IntGetWindowPlacement, we'd need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] internal static void GetWindowPlacement(HandleRef hWnd, ref NativeMethods.WINDOWPLACEMENT placement) { if (IntGetWindowPlacement(hWnd, ref placement) == false) { throw new Win32Exception(); } } [DllImport(ExternDll.User32, EntryPoint = "SetWindowPlacement", ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] private static extern bool IntSetWindowPlacement(HandleRef hWnd, [In] ref NativeMethods.WINDOWPLACEMENT placement); // note: this method appears in UnsafeNativeMethodsCLR.cs but does not have the if/throw block ////// Critical: LinkDemand on Win32Exception constructor /// Note: If a SupressUnmanagedCodeSecurity attribute is ever added to IntSetWindowPlacement, we'd need to be Critical /// TreatAsSafe: Throwing an exception isn't unsafe /// [SecurityCritical, SecurityTreatAsSafe] internal static void SetWindowPlacement(HandleRef hWnd, [In] ref NativeMethods.WINDOWPLACEMENT placement) { if (IntSetWindowPlacement(hWnd, ref placement) == false) { throw new Win32Exception(); } } //[DllImport("secur32.dll", CharSet = CharSet.Unicode, SetLastError = true, ExactSpelling = true)] //internal static extern bool GetUserNameExW( // [In] EXTENDED_NAME_FORMAT nameFormat, // [MarshalAs(UnmanagedType.LPWStr)] StringBuilder lpNameBuffer, // [In, Out] ref ulong nSize); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet = CharSet.Auto, BestFitMapping = false)] internal static extern bool SystemParametersInfo(int nAction, int nParam, [In, Out] NativeMethods.ANIMATIONINFO anim, int nUpdate); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern bool SystemParametersInfo(int nAction, int nParam, [In, Out] NativeMethods.ICONMETRICS metrics, int nUpdate); //--------------------------------------------------------------------------- // SetWindowThemeAttribute() // - set attributes to control how themes are applied to // windows. // // hwnd - the handle of the window (cannot be NULL) // // eAttribute - one of the following: // // WTA_NONCLIENT: // pvAttribute must be a WINDOWTHEMEATTRIBUTE pointer with a valid WTNCA flag // the default is all flags set to 0 // // pvAttribute - pointer to data relevant to property being set size // is cbAttribute see each property for details. // // cbAttribute - size in bytes of the data pointed to by pvAttribute // //--------------------------------------------------------------------------- #if WCP_SYSTEM_THEMES_ENABLED [DllImport(ExternDll.Uxtheme, CharSet = CharSet.Unicode)] public static extern uint SetWindowThemeAttribute(HandleRef hwnd, NativeMethods.WINDOWTHEMEATTRIBUTETYPE eAttribute, [In, MarshalAs(UnmanagedType.LPStruct)] NativeMethods.WTA_OPTIONS pvAttribute, int cbAttribute); public static uint SetWindowThemeAttribute(HandleRef hwnd, NativeMethods.WINDOWTHEMEATTRIBUTETYPE eAttribute, NativeMethods.WTA_OPTIONS pvAttribute) { return SetWindowThemeAttribute(hwnd, eAttribute, pvAttribute, Marshal.SizeOf(typeof(NativeMethods.WTA_OPTIONS))); } #endif ////// /// [DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)] public static extern bool SetEvent(IntPtr hEvent); [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern int SetEvent([In] SafeWaitHandle hHandle); [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern int WaitForSingleObject([In] SafeWaitHandle hHandle, [In] int dwMilliseconds); #if never //"STDAPI GetCORSystemDirectory(LPWSTR pbuffer, DWORD cchBuffer, DWORD* dwLength);" [System.Runtime.InteropServices.DllImport("mscoree.dll", CharSet = System.Runtime.InteropServices.CharSet.Unicode, ExactSpelling = true, SetLastError = false, PreserveSig = false)] //since PreserveSig is false, it will return number of bytes filled in sb on success and throw on fail internal static extern int GetCORSystemDirectory(System.Text.StringBuilder sb, int sbSize); #endif //[DllImport(ExternDll.Kernel32, SetLastError = true)] //internal static extern int GetFileSize(SafeFileHandle hFile, ref int lpFileSizeHigh); ////////////////////////////////////// // Needed by BASE #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] internal static extern int GetMouseMovePointsEx( uint cbSize, [In] ref NativeMethods.MOUSEMOVEPOINT pointsIn, [Out] NativeMethods.MOUSEMOVEPOINT[] pointsBufferOut, int nBufPoints, uint resolution ); [StructLayout(LayoutKind.Explicit)] internal unsafe struct ULARGE_INTEGER { [FieldOffset(0)] internal uint LowPart; [FieldOffset(4)] internal uint HighPart; [FieldOffset(0)] internal ulong QuadPart; } [StructLayout(LayoutKind.Explicit)] internal unsafe struct LARGE_INTEGER { [FieldOffset(0)] internal int LowPart; [FieldOffset(4)] internal int HighPart; [FieldOffset(0)] internal long QuadPart; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport("kernel32.dll", SetLastError = true)] internal static extern bool GetFileSizeEx( SafeFileHandle hFile, ref LARGE_INTEGER lpFileSize ); ///Win32 constants internal static readonly IntPtr INVALID_HANDLE_VALUE = new IntPtr(-1); ///Win32 constants internal const int PAGE_NOACCESS = 0x01; ///Win32 constants internal const int PAGE_READONLY = 0x02; ///Win32 constants internal const int PAGE_READWRITE = 0x04; ///Win32 constants internal const int PAGE_WRITECOPY = 0x08; ///Win32 constants internal const int PAGE_EXECUTE = 0x10; ///Win32 constants internal const int PAGE_EXECUTE_READ = 0x20; ///Win32 constants internal const int PAGE_EXECUTE_READWRITE = 0x40; ///Win32 constants internal const int PAGE_EXECUTE_WRITECOPY = 0x80; ///Win32 constants internal const int PAGE_GUARD = 0x100; ///Win32 constants internal const int PAGE_NOCACHE = 0x200; ///Win32 constants internal const int PAGE_WRITECOMBINE = 0x400; ///Win32 constants internal const int MEM_COMMIT = 0x1000; ///Win32 constants internal const int MEM_RESERVE = 0x2000; ///Win32 constants internal const int MEM_DECOMMIT = 0x4000; ///Win32 constants internal const int MEM_RELEASE = 0x8000; ///Win32 constants internal const int MEM_FREE = 0x10000; ///Win32 constants internal const int MEM_PRIVATE = 0x20000; ///Win32 constants internal const int MEM_MAPPED = 0x40000; ///Win32 constants internal const int MEM_RESET = 0x80000; ///Win32 constants internal const int MEM_TOP_DOWN = 0x100000; ///Win32 constants internal const int MEM_WRITE_WATCH = 0x200000; ///Win32 constants internal const int MEM_PHYSICAL = 0x400000; ///Win32 constants internal const int MEM_4MB_PAGES = unchecked((int)0x80000000); ///Win32 constants internal const int SEC_FILE = 0x800000; ///Win32 constants internal const int SEC_IMAGE = 0x1000000; ///Win32 constants internal const int SEC_RESERVE = 0x4000000; ///Win32 constants internal const int SEC_COMMIT = 0x8000000; ///Win32 constants internal const int SEC_NOCACHE = 0x10000000; ///Win32 constants internal const int MEM_IMAGE = SEC_IMAGE; ///Win32 constants internal const int WRITE_WATCH_FLAG_RESET = 0x01; ///Win32 constants internal const int SECTION_ALL_ACCESS = STANDARD_RIGHTS_REQUIRED | SECTION_QUERY | SECTION_MAP_WRITE | SECTION_MAP_READ | SECTION_MAP_EXECUTE | SECTION_EXTEND_SIZE; ///Win32 constants internal const int STANDARD_RIGHTS_REQUIRED = 0x000F0000; ///Win32 constants internal const int SECTION_QUERY = 0x0001; ///Win32 constants internal const int SECTION_MAP_WRITE = 0x0002; ///Win32 constants internal const int SECTION_MAP_READ = 0x0004; ///Win32 constants internal const int SECTION_MAP_EXECUTE = 0x0008; ///Win32 constants internal const int SECTION_EXTEND_SIZE = 0x0010; ///Win32 constants internal const int FILE_MAP_COPY = SECTION_QUERY; ///Win32 constants internal const int FILE_MAP_WRITE = SECTION_MAP_WRITE; ///Win32 constants internal const int FILE_MAP_READ = SECTION_MAP_READ; ///Win32 constants internal const int FILE_MAP_ALL_ACCESS = SECTION_ALL_ACCESS; ////// /// /// /// /// /// ///[DllImport("advapi32.dll", SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern bool ConvertStringSecurityDescriptorToSecurityDescriptor( string stringSecurityDescriptor, // security descriptor string int stringSDRevision, // revision level ref IntPtr securityDescriptor, // SD IntPtr securityDescriptorSize // SD size ); /// Win32 constants internal const int SDDL_REVISION_1 = 1; ///Win32 constants internal const int SDDL_REVISION = SDDL_REVISION_1; ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport("kernel32.dll", SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern SafeFileMappingHandle OpenFileMapping( int dwDesiredAccess, bool bInheritHandle, string lpName ); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport("kernel32.dll", SetLastError = true)] internal static extern IntPtr VirtualAlloc( IntPtr lpAddress, UIntPtr dwSize, int flAllocationType, int flProtect ); // // RIT WM_MOUSEQUERY structure for DWM WM_MOUSEQUERY (see HwndMouseInputSource.cs) // [StructLayout(LayoutKind.Sequential, Pack = 1)] // For DWM WM_MOUSEQUERY internal unsafe struct MOUSEQUERY { internal UInt32 uMsg; internal IntPtr wParam; internal IntPtr lParam; internal Int32 ptX; internal Int32 ptY; internal IntPtr hwnd; } ////// Critical as this code performs an elevation (via SuppressUnmanagedCodeSecurity) /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern int OleIsCurrentClipboard(IComDataObject pDataObj); [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto)] internal static extern int GetOEMCP(); #if never [DllImport("user32.dll", CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern int ToUnicode(int nVirtKey, int nScanCode, byte[] keystate, StringBuilder text, int cch, int flags); #endif // WinEvent fired when new Avalon UI is created public const int EventObjectUIFragmentCreate = 0x6FFFFFFF; ////////////////////////////////// // Needed by FontCache [DllImport("ntdll.dll")] internal static extern int RtlNtStatusToDosError(int Status); internal static bool NtSuccess(int err) { return err >= STATUS_SUCCESS; } ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// [SecurityCritical, SecurityTreatAsSafe] internal static void NtCheck(int err) { if (!NtSuccess(err)) { int win32error = RtlNtStatusToDosError(err); throw new System.ComponentModel.Win32Exception(win32error); } } internal const int STATUS_SUCCESS = 0; internal const int STATUS_TIMEOUT = 0x00000102; internal const int STATUS_BUFFER_TOO_SMALL = unchecked((int)0xC0000023); #endif // BASE_NATIVEMETHODS // // ETW Tracing native methods // ////// Critical as this code performs an elevation. /// [SecurityCritical(SecurityCriticalScope.Everything)] [SuppressUnmanagedCodeSecurity] internal sealed class EtwTrace { // Ensure class cannot be instantiated by making the constructor private private EtwTrace() { } internal unsafe delegate uint EtwProc(uint requestCode, System.IntPtr requestContext, System.IntPtr bufferSize, byte* buffer); // // Structures // [StructLayout(LayoutKind.Sequential)] internal struct CSTRACE_GUID_REGISTRATION { internal unsafe System.Guid* Guid; internal uint RegHandle; } [StructLayout(LayoutKind.Sequential)] internal struct TraceGuidRegistration { internal unsafe System.Guid* Guid; internal unsafe void* RegHandle; } // // Function Signatures // [DllImport("advapi32", ExactSpelling = true, EntryPoint = "GetTraceEnableFlags", CharSet = System.Runtime.InteropServices.CharSet.Unicode)] internal static extern int GetTraceEnableFlags(ulong traceHandle); [DllImport("advapi32", ExactSpelling = true, EntryPoint = "GetTraceEnableLevel", CharSet = System.Runtime.InteropServices.CharSet.Unicode)] internal static extern byte GetTraceEnableLevel(ulong traceHandle); [DllImport("advapi32", ExactSpelling = true, EntryPoint = "RegisterTraceGuidsW", CharSet = System.Runtime.InteropServices.CharSet.Unicode)] internal static extern unsafe uint RegisterTraceGuids([In]EtwProc cbFunc, [In]void* context, [In] ref System.Guid controlGuid, [In] uint guidCount, ref TraceGuidRegistration guidReg, [In]string mofImagePath, [In] string mofResourceName, [Out] out ulong regHandle); [DllImport("advapi32", ExactSpelling = true, EntryPoint = "UnregisterTraceGuids", CharSet = System.Runtime.InteropServices.CharSet.Unicode)] internal static extern int UnregisterTraceGuids(ulong regHandle); [DllImport("advapi32", ExactSpelling = true, EntryPoint = "TraceEvent", CharSet = System.Runtime.InteropServices.CharSet.Unicode)] internal static extern unsafe uint TraceEvent(ulong traceHandle, char* header); } // // COM Helper Methods // ////// Critical: Satisfies a LinkDemand on releasecom call. /// [SecurityCritical] internal static int SafeReleaseComObject(object o) { int refCount = 0; // Validate if (o != null) { if (Marshal.IsComObject(o)) { refCount = Marshal.ReleaseComObject(o); } } return refCount; } #if WINDOWS_BASE ////// Critical as this code performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(Microsoft.Internal.DllImport.Wininet, EntryPoint = "GetUrlCacheConfigInfoW", SetLastError=true)] internal static extern bool GetUrlCacheConfigInfo( ref NativeMethods.InternetCacheConfigInfo pInternetCacheConfigInfo, ref UInt32 cbCacheConfigInfo, UInt32 /* DWORD */ fieldControl ); #endif ////// Critical: takes an hwnd, calls unmanaged code /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport("WtsApi32.dll")] [return: MarshalAs(UnmanagedType.Bool)] public static extern bool WTSRegisterSessionNotification(IntPtr hwnd, uint dwFlags); ////// Critical: takes an hwnd, calls unmanaged code /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport("WtsApi32.dll")] [return: MarshalAs(UnmanagedType.Bool)] public static extern bool WTSUnRegisterSessionNotification(IntPtr hwnd); [SecurityCritical] [DllImport(ExternDll.Kernel32, SetLastError = true)] public static extern IntPtr GetCurrentProcess(); public const int DUPLICATE_CLOSE_SOURCE = 1; public const int DUPLICATE_SAME_ACCESS = 2; [SecurityCritical] [DllImport(ExternDll.Kernel32, SetLastError = true)] public static extern bool DuplicateHandle( IntPtr hSourceProcess, SafeWaitHandle hSourceHandle, IntPtr hTargetProcessHandle, out IntPtr hTargetHandle, uint dwDesiredAccess, bool fInheritHandle, uint dwOptions ); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Threading; using System.ComponentModel; using System.Diagnostics; namespace MS.Win32 { using Accessibility; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System; using System.Security.Permissions; using System.Collections; using System.IO; using System.Text; using System.Security; using Microsoft.Win32.SafeHandles; #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif DRT using MS.Internal.Drt; #else #error Attempt to use FriendAccessAllowedAttribute from an unknown assembly. using MS.Internal.YourAssemblyName; #endif using IComDataObject = System.Runtime.InteropServices.ComTypes.IDataObject; //[SuppressUnmanagedCodeSecurity()] [FriendAccessAllowed] internal partial class UnsafeNativeMethods { ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport("kernel32.dll", CharSet=CharSet.Auto, SetLastError=true, EntryPoint="GetTempFileName")] internal static extern uint _GetTempFileName(string tmpPath, string prefix, uint uniqueIdOrZero, StringBuilder tmpFileName); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical] internal static uint GetTempFileName(string tmpPath, string prefix, uint uniqueIdOrZero, StringBuilder tmpFileName) { uint result = _GetTempFileName(tmpPath, prefix, uniqueIdOrZero, tmpFileName); if (result == 0) { throw new Win32Exception(); } return result; } ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.NaturalLanguage6, PreserveSig = false, EntryPoint = "DllGetClassObject")] internal static extern void NaturalLanguageDllGetClassObject(ref Guid clsid, ref Guid iid, [MarshalAs(UnmanagedType.Interface)] out object classObject); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Shell32, CharSet = System.Runtime.InteropServices.CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern int ExtractIconEx( string stExeFileName, int nIconIndex, ref NativeMethods.IconHandle phiconLarge, ref NativeMethods.IconHandle phiconSmall, int nIcons); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError=true)] internal static extern NativeMethods.IconHandle CreateIcon(IntPtr hInstance, int nWidth, int nHeight, byte cPlanes, byte cBitsPixel, byte[] lpbANDbits, byte[] lpbXORbits); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = CharSet.Auto)] public static extern bool CreateCaret(HandleRef hwnd, NativeMethods.BitmapHandle hbitmap, int width, int height); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, SetLastError = true, CharSet = CharSet.Auto)] public static extern bool ShowCaret(HandleRef hwnd); ////// Critical: This elevates to unmanaged code permission /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Auto)] public static extern bool ShowWindowAsync(HandleRef hWnd, int nCmdShow); [DllImport(ExternDll.User32, EntryPoint="LoadImage", CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern NativeMethods.IconHandle LoadImageIcon(IntPtr hinst, string stName, int nType, int cxDesired, int cyDesired, int nFlags); [DllImport(ExternDll.User32, EntryPoint="LoadImage", CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern NativeMethods.CursorHandle LoadImageCursor(IntPtr hinst, string stName, int nType, int cxDesired, int cyDesired, int nFlags); // uncomment this if you plan to use LoadImage to load anything other than Icons/Cursors. /* [DllImport(ExternDll.User32, CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern SafeHandle LoadImage( IntPtr hinst, string stName, int nType, int cxDesired, int cyDesired, int nFlags); */ /* [DllImport(ExternDll.User32, CharSet = System.Runtime.InteropServices.CharSet.Auto, SetLastError = true, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern NativeMethods.IconHandle LoadImage( IntPtr hinst, string stName, int nType, int cxDesired, int cyDesired, int nFlags); */ ////// Critical - performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Urlmon, ExactSpelling = true, CharSet = System.Runtime.InteropServices.CharSet.Ansi, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern int ObtainUserAgentString(int dwOption, StringBuilder userAgent, ref int length); ////// Critical - performs an elevation. /// /// Could be a candidate for safe - as the only information disclosed is whether /// a certain security measure is on or off. /// Likely this determination could be made by trying certain actions and failing. /// [SecurityCritical, SuppressUnmanagedCodeSecurity ] [DllImport( ExternDll.Urlmon, ExactSpelling=true)] internal static extern int CoInternetIsFeatureEnabled( int featureEntry , int dwFlags ); ////// Critical - performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity ] [DllImport( ExternDll.Urlmon, ExactSpelling=true)] internal static extern int CoInternetSetFeatureEnabled( int featureEntry , int dwFlags, bool fEnable ); ////// Critical - performs an elevation. /// /// Could be a candidate for safe - as the only information disclosed is whether /// a certain security measure is on or off. /// Likely this determination could be made by trying certain actions and failing. /// [SecurityCritical, SuppressUnmanagedCodeSecurity ] [DllImport( ExternDll.Urlmon, ExactSpelling=true)] internal static extern int CoInternetIsFeatureZoneElevationEnabled( [MarshalAs(UnmanagedType.LPWStr)] string szFromURL, [MarshalAs(UnmanagedType.LPWStr)] string szToURL, UnsafeNativeMethods.IInternetSecurityManager secMgr, int dwFlags ); ////// Critical - call is SUC'ed /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.PresentationHostDll, EntryPoint = "ProcessUnhandledException")] internal static extern void ProcessUnhandledException_DLL([MarshalAs(UnmanagedType.BStr)] string errMsg); ////// Critical - performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity ] [DllImport(ExternDll.Kernel32, CharSet=CharSet.Unicode)] internal static extern bool GetVersionEx([In, Out] NativeMethods.OSVERSIONINFOEX ver); ////// Critical - performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity ] [DllImport( ExternDll.Urlmon, ExactSpelling=true)] internal static extern int CoInternetCreateSecurityManager( [MarshalAs(UnmanagedType.Interface)] object pIServiceProvider, [MarshalAs(UnmanagedType.Interface)] out object ppISecurityManager , int dwReserved ) ; [ComImport, ComVisible(false), Guid("79eac9ee-baf9-11ce-8c82-00aa004ba90b"), System.Runtime.InteropServices.InterfaceType(ComInterfaceType.InterfaceIsIUnknown)] internal interface IInternetSecurityManager { void SetSecuritySite( NativeMethods.IInternetSecurityMgrSite pSite); unsafe void GetSecuritySite( /* [out] */ void **ppSite); ////// Critical - performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] void MapUrlToZone( [In, MarshalAs(UnmanagedType.BStr)] string pwszUrl, [Out] out int pdwZone, [In] int dwFlags); unsafe void GetSecurityId( /* [in] */ string pwszUrl, /* [size_is][out] */ byte *pbSecurityId, /* [out][in] */ int *pcbSecurityId, /* [in] */ int dwReserved); unsafe void ProcessUrlAction( /* [in] */ string pwszUrl, /* [in] */ int dwAction, /* [size_is][out] */ byte *pPolicy, /* [in] */ int cbPolicy, /* [in] */ byte *pContext, /* [in] */ int cbContext, /* [in] */ int dwFlags, /* [in] */ int dwReserved); unsafe void QueryCustomPolicy( /* [in] */ string pwszUrl, /* [in] */ /*REFGUID*/ void *guidKey, /* [size_is][size_is][out] */ byte **ppPolicy, /* [out] */ int *pcbPolicy, /* [in] */ byte *pContext, /* [in] */ int cbContext, /* [in] */ int dwReserved); unsafe void SetZoneMapping( /* [in] */ int dwZone, /* [in] */ string lpszPattern, /* [in] */ int dwFlags); unsafe void GetZoneMappings( /* [in] */ int dwZone, /* [out] */ /*IEnumString*/ void **ppenumString, /* [in] */ int dwFlags); } ////// /// /// ///[DllImport("kernel32.dll", SetLastError = true), ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static extern IntPtr LocalFree(IntPtr hMem); #if BASE_NATIVEMETHODS /// /// SecurityCritical: This code returns a critical resource obtained under an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal unsafe static extern SafeFileHandle CreateFile( string lpFileName, uint dwDesiredAccess, uint dwShareMode, [In] NativeMethods.SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile); #endif #if BASE_NATIVEMETHODS ////// Critical: This code is critical because it can be used to /// pass and force arbitrary data into the tree. We should /// consider yanking it out all the way /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet = CharSet.Auto)] internal static extern IntPtr GetMessageExtraInfo(); #endif #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, EntryPoint="WaitForMultipleObjectsEx", SetLastError = true, CharSet = CharSet.Auto)] private static extern int IntWaitForMultipleObjectsEx(int nCount, IntPtr[] pHandles, bool bWaitAll, int dwMilliseconds, bool bAlertable); public const int WAIT_FAILED = unchecked((int)0xFFFFFFFF); ////// Critical - calls IntWaitForMultipleObjectsEx (the real PInvoke method) /// [SecurityCritical] internal static int WaitForMultipleObjectsEx(int nCount, IntPtr[] pHandles, bool bWaitAll, int dwMilliseconds, bool bAlertable) { int result = IntWaitForMultipleObjectsEx(nCount, pHandles, bWaitAll, dwMilliseconds, bAlertable); if(result == UnsafeNativeMethods.WAIT_FAILED) { throw new Win32Exception(); } return result; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="MsgWaitForMultipleObjectsEx", SetLastError=true, ExactSpelling = true, CharSet = CharSet.Auto)] private static extern int IntMsgWaitForMultipleObjectsEx(int nCount, IntPtr[] pHandles, int dwMilliseconds, int dwWakeMask, int dwFlags); ////// Critical - calls IntMsgWaitForMultipleObjectsEx (the real PInvoke method) /// [SecurityCritical] internal static int MsgWaitForMultipleObjectsEx(int nCount, IntPtr[] pHandles, int dwMilliseconds, int dwWakeMask, int dwFlags) { int result = IntMsgWaitForMultipleObjectsEx(nCount, pHandles, dwMilliseconds, dwWakeMask, dwFlags); if(result == -1) { throw new Win32Exception(); } return result; } #endif ////// Critical: This code elevates to unmanaged code permission /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="RegisterClassEx", CharSet=CharSet.Auto, SetLastError=true, BestFitMapping=false)] internal static extern UInt16 IntRegisterClassEx(NativeMethods.WNDCLASSEX_D wc_d); ////// Critical - calls IntRegisterClassEx (the real PInvoke method) /// [SecurityCritical] internal static UInt16 RegisterClassEx(NativeMethods.WNDCLASSEX_D wc_d) { UInt16 result = IntRegisterClassEx(wc_d); if(result == 0) { throw new Win32Exception(); } return result; } ////// Critical: This code elevates to unmanaged code permission /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="UnregisterClass",CharSet = CharSet.Auto, SetLastError = true, BestFitMapping=false)] internal static extern int IntUnregisterClass(IntPtr atomString /*lpClassName*/ , IntPtr hInstance); ////// Critical - calls IntUnregisterClass (the real PInvoke method) /// [SecurityCritical] internal static void UnregisterClass(IntPtr atomString /*lpClassName*/ , IntPtr hInstance) { int result = IntUnregisterClass(atomString, hInstance); if (result == 0) { throw new Win32Exception(); } } // note that this method exists in UnsafeNativeMethodsCLR.cs but with a different signature // using a HandleRef for the hWnd instead of an IntPtr, and not using an IntPtr for lParam [DllImport(ExternDll.User32, CharSet = CharSet.Auto)] internal static extern IntPtr SendMessage(IntPtr hWnd, int msg, IntPtr wParam, IntPtr lParam); // note that this method exists in UnsafeNativeMethodsCLR.cs but with a different signature // using a HandleRef for the hWnd instead of an IntPtr, and not using an IntPtr for lParam ////// Critical: This code has the ability to send a message to the wndproc. It exists purely for /// the secure close scenario. For any other scenario please use the SendMessage call /// [DllImport(ExternDll.User32,EntryPoint="SendMessage", CharSet = CharSet.Auto)] [SecurityCritical,SuppressUnmanagedCodeSecurity] internal static extern IntPtr UnsafeSendMessage(IntPtr hWnd, int msg, IntPtr wParam, IntPtr lParam); /* // // SendMessage taking a SafeHandle for wParam. Needed by some Win32 messages. e.g. WM_PRINT // [DllImport(ExternDll.User32, CharSet = CharSet.Auto)] internal static extern IntPtr SendMessage(HandleRef hWnd, int msg, SafeHandle wParam, IntPtr lParam); */ // private DllImport - that takes an IconHandle. ////// Critical: This code causes elevation to unmanaged code /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet = CharSet.Auto, SetLastError = true)] internal static extern IntPtr SendMessage( HandleRef hWnd, int msg, IntPtr wParam, NativeMethods.IconHandle iconHandle ); ////// Critical: This code causes elevation to unmanaged code /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto)] internal static extern void SetLastError(int dwErrorCode); #if BASE_NATIVEMETHODS || CORE_NATIVEMETHODS || FRAMEWORK_NATIVEMETHODS ////// Win32 GetLayeredWindowAttributes. /// /// /// /// /// ////// /// Critical: This code calls into unmanaged code /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport("user32.dll")] public static extern bool GetLayeredWindowAttributes( HandleRef hwnd, IntPtr pcrKey, IntPtr pbAlpha, IntPtr pdwFlags); internal sealed class SafeFileMappingHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Critical: base class enforces link demand and inheritance demand /// TreatAsSafe: Creating this is ok, accessing the pointer is bad /// [SecurityCritical,SecurityTreatAsSafe] internal SafeFileMappingHandle() : base(true) { } ////// Critical: base class enforces link demand and inheritance demand /// TreatAsSafe: This call is safe /// public override bool IsInvalid { [SecurityCritical,SecurityTreatAsSafe] get { return handle == IntPtr.Zero; } } ////// Critical - as this function does an elevation to close a handle. /// TreatAsSafe - as this can at best be used to destabilize one's own app. /// [SecurityCritical, SecurityTreatAsSafe] protected override bool ReleaseHandle() { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); try { return CloseHandleNoThrow(new HandleRef(null, handle)); } finally { SecurityPermission.RevertAssert(); } } } internal sealed class SafeViewOfFileHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Critical: This code calls into a base class which link demands for unmanaged code /// TreatAsSafe:Creating this is ok it is acessing the pointers in it that can be risky /// [SecurityCritical,SecurityTreatAsSafe] internal SafeViewOfFileHandle() : base(true) { } ////// Critical: This code accesses an unsafe object (pointer) and returns it as a pointer /// internal unsafe void* Memory { [SecurityCritical] get { Debug.Assert(handle != IntPtr.Zero); return (void*)handle; } } ////// Critical - as this function does an elevation to close a handle. /// TreatAsSafe - as this can at best be used to destabilize one's own app. /// [SecurityCritical, SecurityTreatAsSafe] override protected bool ReleaseHandle() { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); try { return UnsafeNativeMethods.UnmapViewOfFileNoThrow(new HandleRef(null, handle)); } finally { SecurityPermission.RevertAssert(); } } } ////// SecurityCritical: This code returns critical resource obtained under an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport("kernel32.dll", SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal unsafe static extern SafeFileMappingHandle CreateFileMapping(SafeFileHandle hFile, NativeMethods.SECURITY_ATTRIBUTES lpFileMappingAttributes, int flProtect, uint dwMaximumSizeHigh, uint dwMaximumSizeLow, string lpName); ////// SecurityCritical: This code returns a critical resource obtained under an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, SetLastError = true)] internal static extern SafeViewOfFileHandle MapViewOfFileEx(SafeFileMappingHandle hFileMappingObject, int dwDesiredAccess, int dwFileOffsetHigh, int dwFileOffsetLow, IntPtr dwNumberOfBytesToMap, IntPtr lpBaseAddress); #endif // BASE_NATIVEMETHODS [DllImport(ExternDll.User32, EntryPoint="SetWindowLongPtr", CharSet=CharSet.Auto)] private static extern IntPtr IntSetWindowLongPtr(HandleRef hWnd, int nIndex, IntPtr dwNewLong); [DllImport(ExternDll.User32, EntryPoint="SetWindowLong", CharSet=CharSet.Auto)] private static extern Int32 IntSetWindowLong(HandleRef hWnd, int nIndex, Int32 dwNewLong); ////// Critical: LinkDemand on Marshal.GetLastWin32Error /// TreatAsSafe: Getting an error code isn't unsafe /// Note: If a SupressUnmanagedCodeSecurity attribute is ever added to IntsetWindowLong(Ptr), we'd need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] internal static IntPtr SetWindowLong(HandleRef hWnd, int nIndex, IntPtr dwNewLong) { IntPtr result = IntPtr.Zero; if (IntPtr.Size == 4) { // use SetWindowLong Int32 tempResult = IntSetWindowLong(hWnd, nIndex, NativeMethods.IntPtrToInt32(dwNewLong)); result = new IntPtr(tempResult); } else { // use SetWindowLongPtr result = IntSetWindowLongPtr(hWnd, nIndex, dwNewLong); } return result; } ////// Critical - link demands for UnmanagedCode permissions /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="SetWindowLongPtr", CharSet=CharSet.Auto)] private static extern IntPtr IntCriticalSetWindowLongPtr(HandleRef hWnd, int nIndex, IntPtr dwNewLong); [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="SetWindowLong", CharSet=CharSet.Auto)] private static extern Int32 IntCriticalSetWindowLong(HandleRef hWnd, int nIndex, Int32 dwNewLong); ////// Critical - it calls IntCriticalSetWindowLongPtr() / IntCriticalSetWindowLong(), which are Critical /// [SecurityCritical] internal static IntPtr CriticalSetWindowLong(HandleRef hWnd, int nIndex, IntPtr dwNewLong) { IntPtr result = IntPtr.Zero; if (IntPtr.Size == 4) { // use SetWindowLong Int32 tempResult = IntCriticalSetWindowLong(hWnd, nIndex, NativeMethods.IntPtrToInt32(dwNewLong)); result = new IntPtr(tempResult); } else { // use SetWindowLongPtr result = IntCriticalSetWindowLongPtr(hWnd, nIndex, dwNewLong); } return result; } ////// Critical - This link demands for UnmanagedCode permissions /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="SetWindowLongPtr", CharSet=CharSet.Auto, SetLastError=true)] private static extern IntPtr IntCriticalSetWindowLongPtr(HandleRef hWnd, int nIndex, NativeMethods.WndProc dwNewLong); [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="SetWindowLong", CharSet=CharSet.Auto, SetLastError=true)] private static extern Int32 IntCriticalSetWindowLong(HandleRef hWnd, int nIndex, NativeMethods.WndProc dwNewLong); ////// Critical - This calls SetLatError() and IntCriticalSetWindowLongPtr() / IntCriticalSetWindowLong(), which are Critical /// [SecurityCritical] internal static IntPtr CriticalSetWindowLong(HandleRef hWnd, int nIndex, NativeMethods.WndProc dwNewLong) { int errorCode; IntPtr retVal; SetLastError(0); // Win32 SetWindowLong doesn't clear error on success if (IntPtr.Size == 4) { Int32 tempRetVal = IntCriticalSetWindowLong(hWnd, nIndex, dwNewLong); errorCode = Marshal.GetLastWin32Error(); retVal = new IntPtr(tempRetVal); } else { retVal = IntCriticalSetWindowLongPtr(hWnd, nIndex, dwNewLong); errorCode = Marshal.GetLastWin32Error(); } if (retVal == IntPtr.Zero) { if (errorCode != 0) { throw new System.ComponentModel.Win32Exception(errorCode); } } return retVal; } ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="GetWindowLongPtr", CharSet=CharSet.Auto, SetLastError=true)] private static extern IntPtr IntGetWindowLongPtr(HandleRef hWnd, int nIndex); ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="GetWindowLong", CharSet=CharSet.Auto, SetLastError=true)] private static extern Int32 IntGetWindowLong(HandleRef hWnd, int nIndex ); ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical] internal static IntPtr GetWindowLongPtr(HandleRef hWnd, int nIndex ) { IntPtr result = IntPtr.Zero; int error = 0; SetLastError(0); // Win32 GetWindowLong doesn't clear error on success if (IntPtr.Size == 4) { // use getWindowLong Int32 tempResult = IntGetWindowLong(hWnd, nIndex); error = Marshal.GetLastWin32Error(); result = new IntPtr(tempResult); } else { // use GetWindowLongPtr result = IntGetWindowLongPtr( hWnd, nIndex); error = Marshal.GetLastWin32Error(); } if ((result == IntPtr.Zero) && (error != 0)) { // To be consistent with out other PInvoke wrappers // we should "throw" here. But we don't want to // introduce new "throws" w/o time to follow up on any // new problems that causes. Debug.WriteLine("GetWindowLongPtr failed. Error = " + error); // throw new System.ComponentModel.Win32Exception(error); } return result; } ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical] internal static Int32 GetWindowLong(HandleRef hWnd, int nIndex ) { int iResult = 0; IntPtr result = IntPtr.Zero; int error = 0; SetLastError(0); // Win32 GetWindowLong doesn't clear error on success if (IntPtr.Size == 4) { // use GetWindowLong iResult = IntGetWindowLong(hWnd, nIndex); error = Marshal.GetLastWin32Error(); result = new IntPtr(iResult); } else { // use GetWindowLongPtr result = IntGetWindowLongPtr(hWnd, nIndex); error = Marshal.GetLastWin32Error(); iResult = NativeMethods.IntPtrToInt32(result); } if ((result == IntPtr.Zero) && (error != 0)) { // To be consistent with out other PInvoke wrappers // we should "throw" here. But we don't want to // introduce new "throws" w/o time to follow up on any // new problems that causes. Debug.WriteLine("GetWindowLong failed. Error = " + error); // throw new System.ComponentModel.Win32Exception(error); } return iResult; } ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="GetWindowLongPtr", CharSet=CharSet.Auto, SetLastError=true)] public static extern NativeMethods.WndProc IntGetWindowLongWndProcPtr(HandleRef hWnd, int nIndex); ////// SecurityCritical: This code happens to return a critical resource and causes unmanaged code elevation /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint="GetWindowLong", CharSet=CharSet.Auto, SetLastError=true)] public static extern NativeMethods.WndProc IntGetWindowLongWndProc(HandleRef hWnd, int nIndex); ////// Critical: Call critical method IntGetWindowLongWndProc and IntGetWindowLongWndProcPtr that causes unmanaged code elevation. /// LinkDemand on Win32Exception constructor but throwing an exception isn't unsafe /// [SecurityCritical] internal static NativeMethods.WndProc GetWindowLongWndProc(HandleRef hWnd) { NativeMethods.WndProc returnValue = null; int error = 0; SetLastError(0); // Win32 GetWindowLong doesn't clear error on success if (IntPtr.Size == 4) { // use getWindowLong returnValue = IntGetWindowLongWndProc (hWnd, NativeMethods.GWL_WNDPROC); error = Marshal.GetLastWin32Error(); } else { // use GetWindowLongPtr returnValue = IntGetWindowLongWndProcPtr (hWnd, NativeMethods.GWL_WNDPROC); error = Marshal.GetLastWin32Error(); } if (null == returnValue) { throw new Win32Exception(error); } return returnValue; } ////// Critical - Unmanaged code permission is supressed. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] [DllImport("winmm.dll", CharSet = CharSet.Unicode)] internal static extern bool PlaySound([In]string soundName, IntPtr hmod, SafeNativeMethods.PlaySoundFlags soundFlags); internal const uint INTERNET_COOKIE_THIRD_PARTY = 0x10, INTERNET_COOKIE_EVALUATE_P3P = 0x40, INTERNET_COOKIE_IS_RESTRICTED = 0x200, COOKIE_STATE_REJECT = 5; //!!! CAUTION // PresentationHost intercepts calls to InternetGetCookieEx & InternetSetCookieEx and delegates them // to the browser. It doesn't do this for InternetGetCookie & InternetSetCookie. // See also Application.Get/SetCookie(). //!!! ////// SecurityCritical - calls unmanaged code. /// [DllImport(ExternDll.Wininet, SetLastError=true, ExactSpelling=true, EntryPoint="InternetGetCookieExW", CharSet=CharSet.Unicode)] [SuppressUnmanagedCodeSecurity, SecurityCritical] internal static extern bool InternetGetCookieEx([In]string Url, [In]string cookieName, [Out] StringBuilder cookieData, [In, Out] ref UInt32 pchCookieData, uint flags, IntPtr reserved); ////// SecurityCritical - calls unmanaged code. /// [DllImport(ExternDll.Wininet, SetLastError = true, ExactSpelling = true, EntryPoint = "InternetSetCookieExW", CharSet = CharSet.Unicode)] [SuppressUnmanagedCodeSecurity] [SecurityCritical] internal static extern uint InternetSetCookieEx([In]string Url, [In]string CookieName, [In]string cookieData, uint flags, [In] string p3pHeader); #if DRT_NATIVEMETHODS [DllImport(ExternDll.User32, ExactSpelling = true, EntryPoint = "mouse_event", CharSet = CharSet.Auto)] internal static extern void Mouse_event(int flags, int dx, int dy, int dwData, IntPtr extrainfo); #endif ///////////////////////////// // needed by Framework ////// Critical - calls unmanaged code /// [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Unicode)] [SuppressUnmanagedCodeSecurity] [SecurityCritical] internal static extern int GetLocaleInfoW(int locale, int type, string data, int dataSize); ////// Critical - calls unmanaged code /// [DllImport(ExternDll.Kernel32, ExactSpelling = true, SetLastError = true)] [SuppressUnmanagedCodeSecurity] [SecurityCritical] internal static extern int FindNLSString(int locale, uint flags, [MarshalAs(UnmanagedType.LPWStr)]string sourceString, int sourceCount, [MarshalAs(UnmanagedType.LPWStr)]string findString, int findCount, out int found); //[DllImport(ExternDll.Psapi, SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false)] //public static extern int GetModuleFileNameEx(IntPtr hProcess, IntPtr hModule, StringBuilder buffer, int length); // // OpenProcess // public const int PROCESS_VM_READ = 0x0010; public const int PROCESS_QUERY_INFORMATION = 0x0400; //[DllImport(ExternDll.Kernel32, SetLastError = true, CharSet = CharSet.Auto)] //public static extern IntPtr OpenProcess(int dwDesiredAccess, bool fInherit, int dwProcessId); [DllImport(ExternDll.User32, EntryPoint = "SetWindowText", CharSet = CharSet.Auto, SetLastError = true, BestFitMapping = false)] private static extern bool IntSetWindowText(HandleRef hWnd, string text); ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// Note: If a SupressUnmanagedCodeSecurity attribute is ever added to IntSetWindowText, we'd need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] internal static void SetWindowText(HandleRef hWnd, string text) { if (IntSetWindowText(hWnd, text) == false) { throw new Win32Exception(); } } ////// Critical: This code calls into unmanaged code /// [SecurityCritical,SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, EntryPoint = "GetIconInfo", CharSet = CharSet.Auto, SetLastError = true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] private static extern bool GetIconInfoImpl(HandleRef hIcon, [Out] ICONINFO_IMPL piconinfo); [StructLayout(LayoutKind.Sequential)] internal class ICONINFO_IMPL { public bool fIcon = false; public int xHotspot = 0; public int yHotspot = 0; public IntPtr hbmMask = IntPtr.Zero; public IntPtr hbmColor = IntPtr.Zero; } // FOR [SecurityCritical] internal static void GetIconInfo(HandleRef hIcon, out NativeMethods.ICONINFO piconinfo) { bool success = false; int error = 0; piconinfo = new NativeMethods.ICONINFO(); ICONINFO_IMPL iconInfoImpl = new ICONINFO_IMPL(); RuntimeHelpers.PrepareConstrainedRegions(); // Mark the following as special try { // Intentionally empty } finally { // This block won't be interrupted by certain runtime induced failures or thread abort success = GetIconInfoImpl(hIcon, iconInfoImpl); error = Marshal.GetLastWin32Error(); if (success) { piconinfo.hbmMask = new NativeMethods.BitmapHandle(iconInfoImpl.hbmMask, true /*own*/); piconinfo.hbmColor = new NativeMethods.BitmapHandle(iconInfoImpl.hbmColor, true /*own*/); piconinfo.fIcon = iconInfoImpl.fIcon; piconinfo.xHotspot = iconInfoImpl.xHotspot; piconinfo.yHotspot = iconInfoImpl.yHotspot; } } if(!success) { Debug.WriteLine("GetIconInfo failed. Error = " + error); throw new Win32Exception(); } } #if never [DllImport(ExternDll.User32, #if WIN64 EntryPoint="GetClassLongPtr", #endif CharSet = CharSet.Auto, SetLastError = true) ] internal static extern UInt32 GetClassLong(IntPtr hwnd, int nIndex); #endif [DllImport(ExternDll.User32, EntryPoint = "GetWindowPlacement", ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] private static extern bool IntGetWindowPlacement(HandleRef hWnd, ref NativeMethods.WINDOWPLACEMENT placement); // note: this method exists in UnsafeNativeMethodsCLR.cs, but that method does not have the if/throw implemntation ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// Note: If a SupressUnmanagedCodeSecurity attribute is ever added to IntGetWindowPlacement, we'd need to be Critical /// [SecurityCritical, SecurityTreatAsSafe] internal static void GetWindowPlacement(HandleRef hWnd, ref NativeMethods.WINDOWPLACEMENT placement) { if (IntGetWindowPlacement(hWnd, ref placement) == false) { throw new Win32Exception(); } } [DllImport(ExternDll.User32, EntryPoint = "SetWindowPlacement", ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] private static extern bool IntSetWindowPlacement(HandleRef hWnd, [In] ref NativeMethods.WINDOWPLACEMENT placement); // note: this method appears in UnsafeNativeMethodsCLR.cs but does not have the if/throw block ////// Critical: LinkDemand on Win32Exception constructor /// Note: If a SupressUnmanagedCodeSecurity attribute is ever added to IntSetWindowPlacement, we'd need to be Critical /// TreatAsSafe: Throwing an exception isn't unsafe /// [SecurityCritical, SecurityTreatAsSafe] internal static void SetWindowPlacement(HandleRef hWnd, [In] ref NativeMethods.WINDOWPLACEMENT placement) { if (IntSetWindowPlacement(hWnd, ref placement) == false) { throw new Win32Exception(); } } //[DllImport("secur32.dll", CharSet = CharSet.Unicode, SetLastError = true, ExactSpelling = true)] //internal static extern bool GetUserNameExW( // [In] EXTENDED_NAME_FORMAT nameFormat, // [MarshalAs(UnmanagedType.LPWStr)] StringBuilder lpNameBuffer, // [In, Out] ref ulong nSize); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet = CharSet.Auto, BestFitMapping = false)] internal static extern bool SystemParametersInfo(int nAction, int nParam, [In, Out] NativeMethods.ANIMATIONINFO anim, int nUpdate); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern bool SystemParametersInfo(int nAction, int nParam, [In, Out] NativeMethods.ICONMETRICS metrics, int nUpdate); //--------------------------------------------------------------------------- // SetWindowThemeAttribute() // - set attributes to control how themes are applied to // windows. // // hwnd - the handle of the window (cannot be NULL) // // eAttribute - one of the following: // // WTA_NONCLIENT: // pvAttribute must be a WINDOWTHEMEATTRIBUTE pointer with a valid WTNCA flag // the default is all flags set to 0 // // pvAttribute - pointer to data relevant to property being set size // is cbAttribute see each property for details. // // cbAttribute - size in bytes of the data pointed to by pvAttribute // //--------------------------------------------------------------------------- #if WCP_SYSTEM_THEMES_ENABLED [DllImport(ExternDll.Uxtheme, CharSet = CharSet.Unicode)] public static extern uint SetWindowThemeAttribute(HandleRef hwnd, NativeMethods.WINDOWTHEMEATTRIBUTETYPE eAttribute, [In, MarshalAs(UnmanagedType.LPStruct)] NativeMethods.WTA_OPTIONS pvAttribute, int cbAttribute); public static uint SetWindowThemeAttribute(HandleRef hwnd, NativeMethods.WINDOWTHEMEATTRIBUTETYPE eAttribute, NativeMethods.WTA_OPTIONS pvAttribute) { return SetWindowThemeAttribute(hwnd, eAttribute, pvAttribute, Marshal.SizeOf(typeof(NativeMethods.WTA_OPTIONS))); } #endif ////// /// [DllImport("kernel32.dll", CharSet = CharSet.Auto, SetLastError = true)] public static extern bool SetEvent(IntPtr hEvent); [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern int SetEvent([In] SafeWaitHandle hHandle); [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Kernel32, SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern int WaitForSingleObject([In] SafeWaitHandle hHandle, [In] int dwMilliseconds); #if never //"STDAPI GetCORSystemDirectory(LPWSTR pbuffer, DWORD cchBuffer, DWORD* dwLength);" [System.Runtime.InteropServices.DllImport("mscoree.dll", CharSet = System.Runtime.InteropServices.CharSet.Unicode, ExactSpelling = true, SetLastError = false, PreserveSig = false)] //since PreserveSig is false, it will return number of bytes filled in sb on success and throw on fail internal static extern int GetCORSystemDirectory(System.Text.StringBuilder sb, int sbSize); #endif //[DllImport(ExternDll.Kernel32, SetLastError = true)] //internal static extern int GetFileSize(SafeFileHandle hFile, ref int lpFileSizeHigh); ////////////////////////////////////// // Needed by BASE #if BASE_NATIVEMETHODS ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.User32, ExactSpelling = true, CharSet = CharSet.Auto, SetLastError = true)] internal static extern int GetMouseMovePointsEx( uint cbSize, [In] ref NativeMethods.MOUSEMOVEPOINT pointsIn, [Out] NativeMethods.MOUSEMOVEPOINT[] pointsBufferOut, int nBufPoints, uint resolution ); [StructLayout(LayoutKind.Explicit)] internal unsafe struct ULARGE_INTEGER { [FieldOffset(0)] internal uint LowPart; [FieldOffset(4)] internal uint HighPart; [FieldOffset(0)] internal ulong QuadPart; } [StructLayout(LayoutKind.Explicit)] internal unsafe struct LARGE_INTEGER { [FieldOffset(0)] internal int LowPart; [FieldOffset(4)] internal int HighPart; [FieldOffset(0)] internal long QuadPart; } ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport("kernel32.dll", SetLastError = true)] internal static extern bool GetFileSizeEx( SafeFileHandle hFile, ref LARGE_INTEGER lpFileSize ); ///Win32 constants internal static readonly IntPtr INVALID_HANDLE_VALUE = new IntPtr(-1); ///Win32 constants internal const int PAGE_NOACCESS = 0x01; ///Win32 constants internal const int PAGE_READONLY = 0x02; ///Win32 constants internal const int PAGE_READWRITE = 0x04; ///Win32 constants internal const int PAGE_WRITECOPY = 0x08; ///Win32 constants internal const int PAGE_EXECUTE = 0x10; ///Win32 constants internal const int PAGE_EXECUTE_READ = 0x20; ///Win32 constants internal const int PAGE_EXECUTE_READWRITE = 0x40; ///Win32 constants internal const int PAGE_EXECUTE_WRITECOPY = 0x80; ///Win32 constants internal const int PAGE_GUARD = 0x100; ///Win32 constants internal const int PAGE_NOCACHE = 0x200; ///Win32 constants internal const int PAGE_WRITECOMBINE = 0x400; ///Win32 constants internal const int MEM_COMMIT = 0x1000; ///Win32 constants internal const int MEM_RESERVE = 0x2000; ///Win32 constants internal const int MEM_DECOMMIT = 0x4000; ///Win32 constants internal const int MEM_RELEASE = 0x8000; ///Win32 constants internal const int MEM_FREE = 0x10000; ///Win32 constants internal const int MEM_PRIVATE = 0x20000; ///Win32 constants internal const int MEM_MAPPED = 0x40000; ///Win32 constants internal const int MEM_RESET = 0x80000; ///Win32 constants internal const int MEM_TOP_DOWN = 0x100000; ///Win32 constants internal const int MEM_WRITE_WATCH = 0x200000; ///Win32 constants internal const int MEM_PHYSICAL = 0x400000; ///Win32 constants internal const int MEM_4MB_PAGES = unchecked((int)0x80000000); ///Win32 constants internal const int SEC_FILE = 0x800000; ///Win32 constants internal const int SEC_IMAGE = 0x1000000; ///Win32 constants internal const int SEC_RESERVE = 0x4000000; ///Win32 constants internal const int SEC_COMMIT = 0x8000000; ///Win32 constants internal const int SEC_NOCACHE = 0x10000000; ///Win32 constants internal const int MEM_IMAGE = SEC_IMAGE; ///Win32 constants internal const int WRITE_WATCH_FLAG_RESET = 0x01; ///Win32 constants internal const int SECTION_ALL_ACCESS = STANDARD_RIGHTS_REQUIRED | SECTION_QUERY | SECTION_MAP_WRITE | SECTION_MAP_READ | SECTION_MAP_EXECUTE | SECTION_EXTEND_SIZE; ///Win32 constants internal const int STANDARD_RIGHTS_REQUIRED = 0x000F0000; ///Win32 constants internal const int SECTION_QUERY = 0x0001; ///Win32 constants internal const int SECTION_MAP_WRITE = 0x0002; ///Win32 constants internal const int SECTION_MAP_READ = 0x0004; ///Win32 constants internal const int SECTION_MAP_EXECUTE = 0x0008; ///Win32 constants internal const int SECTION_EXTEND_SIZE = 0x0010; ///Win32 constants internal const int FILE_MAP_COPY = SECTION_QUERY; ///Win32 constants internal const int FILE_MAP_WRITE = SECTION_MAP_WRITE; ///Win32 constants internal const int FILE_MAP_READ = SECTION_MAP_READ; ///Win32 constants internal const int FILE_MAP_ALL_ACCESS = SECTION_ALL_ACCESS; ////// /// /// /// /// /// ///[DllImport("advapi32.dll", SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern bool ConvertStringSecurityDescriptorToSecurityDescriptor( string stringSecurityDescriptor, // security descriptor string int stringSDRevision, // revision level ref IntPtr securityDescriptor, // SD IntPtr securityDescriptorSize // SD size ); /// Win32 constants internal const int SDDL_REVISION_1 = 1; ///Win32 constants internal const int SDDL_REVISION = SDDL_REVISION_1; ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport("kernel32.dll", SetLastError = true, CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern SafeFileMappingHandle OpenFileMapping( int dwDesiredAccess, bool bInheritHandle, string lpName ); ////// Critical as this code performs an elevation. /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport("kernel32.dll", SetLastError = true)] internal static extern IntPtr VirtualAlloc( IntPtr lpAddress, UIntPtr dwSize, int flAllocationType, int flProtect ); // // RIT WM_MOUSEQUERY structure for DWM WM_MOUSEQUERY (see HwndMouseInputSource.cs) // [StructLayout(LayoutKind.Sequential, Pack = 1)] // For DWM WM_MOUSEQUERY internal unsafe struct MOUSEQUERY { internal UInt32 uMsg; internal IntPtr wParam; internal IntPtr lParam; internal Int32 ptX; internal Int32 ptY; internal IntPtr hwnd; } ////// Critical as this code performs an elevation (via SuppressUnmanagedCodeSecurity) /// [SecurityCritical] [SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Ole32, ExactSpelling = true, CharSet = CharSet.Auto)] public static extern int OleIsCurrentClipboard(IComDataObject pDataObj); [DllImport(ExternDll.Kernel32, ExactSpelling = true, CharSet = CharSet.Auto)] internal static extern int GetOEMCP(); #if never [DllImport("user32.dll", CharSet = CharSet.Auto, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern int ToUnicode(int nVirtKey, int nScanCode, byte[] keystate, StringBuilder text, int cch, int flags); #endif // WinEvent fired when new Avalon UI is created public const int EventObjectUIFragmentCreate = 0x6FFFFFFF; ////////////////////////////////// // Needed by FontCache [DllImport("ntdll.dll")] internal static extern int RtlNtStatusToDosError(int Status); internal static bool NtSuccess(int err) { return err >= STATUS_SUCCESS; } ////// Critical: LinkDemand on Win32Exception constructor /// TreatAsSafe: Throwing an exception isn't unsafe /// [SecurityCritical, SecurityTreatAsSafe] internal static void NtCheck(int err) { if (!NtSuccess(err)) { int win32error = RtlNtStatusToDosError(err); throw new System.ComponentModel.Win32Exception(win32error); } } internal const int STATUS_SUCCESS = 0; internal const int STATUS_TIMEOUT = 0x00000102; internal const int STATUS_BUFFER_TOO_SMALL = unchecked((int)0xC0000023); #endif // BASE_NATIVEMETHODS // // ETW Tracing native methods // ////// Critical as this code performs an elevation. /// [SecurityCritical(SecurityCriticalScope.Everything)] [SuppressUnmanagedCodeSecurity] internal sealed class EtwTrace { // Ensure class cannot be instantiated by making the constructor private private EtwTrace() { } internal unsafe delegate uint EtwProc(uint requestCode, System.IntPtr requestContext, System.IntPtr bufferSize, byte* buffer); // // Structures // [StructLayout(LayoutKind.Sequential)] internal struct CSTRACE_GUID_REGISTRATION { internal unsafe System.Guid* Guid; internal uint RegHandle; } [StructLayout(LayoutKind.Sequential)] internal struct TraceGuidRegistration { internal unsafe System.Guid* Guid; internal unsafe void* RegHandle; } // // Function Signatures // [DllImport("advapi32", ExactSpelling = true, EntryPoint = "GetTraceEnableFlags", CharSet = System.Runtime.InteropServices.CharSet.Unicode)] internal static extern int GetTraceEnableFlags(ulong traceHandle); [DllImport("advapi32", ExactSpelling = true, EntryPoint = "GetTraceEnableLevel", CharSet = System.Runtime.InteropServices.CharSet.Unicode)] internal static extern byte GetTraceEnableLevel(ulong traceHandle); [DllImport("advapi32", ExactSpelling = true, EntryPoint = "RegisterTraceGuidsW", CharSet = System.Runtime.InteropServices.CharSet.Unicode)] internal static extern unsafe uint RegisterTraceGuids([In]EtwProc cbFunc, [In]void* context, [In] ref System.Guid controlGuid, [In] uint guidCount, ref TraceGuidRegistration guidReg, [In]string mofImagePath, [In] string mofResourceName, [Out] out ulong regHandle); [DllImport("advapi32", ExactSpelling = true, EntryPoint = "UnregisterTraceGuids", CharSet = System.Runtime.InteropServices.CharSet.Unicode)] internal static extern int UnregisterTraceGuids(ulong regHandle); [DllImport("advapi32", ExactSpelling = true, EntryPoint = "TraceEvent", CharSet = System.Runtime.InteropServices.CharSet.Unicode)] internal static extern unsafe uint TraceEvent(ulong traceHandle, char* header); } // // COM Helper Methods // ////// Critical: Satisfies a LinkDemand on releasecom call. /// [SecurityCritical] internal static int SafeReleaseComObject(object o) { int refCount = 0; // Validate if (o != null) { if (Marshal.IsComObject(o)) { refCount = Marshal.ReleaseComObject(o); } } return refCount; } #if WINDOWS_BASE ////// Critical as this code performs an elevation. /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(Microsoft.Internal.DllImport.Wininet, EntryPoint = "GetUrlCacheConfigInfoW", SetLastError=true)] internal static extern bool GetUrlCacheConfigInfo( ref NativeMethods.InternetCacheConfigInfo pInternetCacheConfigInfo, ref UInt32 cbCacheConfigInfo, UInt32 /* DWORD */ fieldControl ); #endif ////// Critical: takes an hwnd, calls unmanaged code /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport("WtsApi32.dll")] [return: MarshalAs(UnmanagedType.Bool)] public static extern bool WTSRegisterSessionNotification(IntPtr hwnd, uint dwFlags); ////// Critical: takes an hwnd, calls unmanaged code /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport("WtsApi32.dll")] [return: MarshalAs(UnmanagedType.Bool)] public static extern bool WTSUnRegisterSessionNotification(IntPtr hwnd); [SecurityCritical] [DllImport(ExternDll.Kernel32, SetLastError = true)] public static extern IntPtr GetCurrentProcess(); public const int DUPLICATE_CLOSE_SOURCE = 1; public const int DUPLICATE_SAME_ACCESS = 2; [SecurityCritical] [DllImport(ExternDll.Kernel32, SetLastError = true)] public static extern bool DuplicateHandle( IntPtr hSourceProcess, SafeWaitHandle hSourceHandle, IntPtr hTargetProcessHandle, out IntPtr hTargetHandle, uint dwDesiredAccess, bool fInheritHandle, uint dwOptions ); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
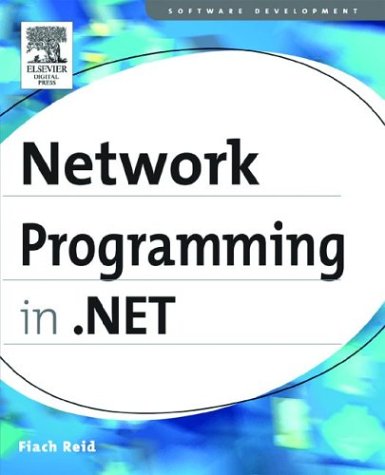
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlNamespaceDeclarationsAttribute.cs
- AssemblyBuilder.cs
- Timer.cs
- DescendantBaseQuery.cs
- OrderablePartitioner.cs
- TypeDependencyAttribute.cs
- ArraySubsetEnumerator.cs
- SemanticAnalyzer.cs
- XmlSchemaSimpleContent.cs
- DataGridViewRowPrePaintEventArgs.cs
- NavigationWindow.cs
- BoundsDrawingContextWalker.cs
- Substitution.cs
- TreeView.cs
- ObjectComplexPropertyMapping.cs
- ActivityDefaults.cs
- ScrollableControl.cs
- ThreadAttributes.cs
- DataGridPagingPage.cs
- NativeRightsManagementAPIsStructures.cs
- MenuItem.cs
- OpCodes.cs
- AnnouncementEndpointElement.cs
- DataGridViewRowPrePaintEventArgs.cs
- _HelperAsyncResults.cs
- FilterException.cs
- ThreadSafeList.cs
- ServiceOperation.cs
- SingleKeyFrameCollection.cs
- EventsTab.cs
- XMLSyntaxException.cs
- DurableDispatcherAddressingFault.cs
- DateRangeEvent.cs
- Attributes.cs
- DataColumnChangeEvent.cs
- LockRecursionException.cs
- ChtmlFormAdapter.cs
- CodeIdentifier.cs
- DataSvcMapFileSerializer.cs
- DurationConverter.cs
- SafeNativeMethods.cs
- ListViewDeleteEventArgs.cs
- StringValidator.cs
- XsltException.cs
- BinaryMethodMessage.cs
- RegexRunnerFactory.cs
- SQLSingle.cs
- WindowsNonControl.cs
- SqlClientWrapperSmiStreamChars.cs
- SqlTypeConverter.cs
- RichTextBox.cs
- GifBitmapDecoder.cs
- WorkflowMarkupSerializationProvider.cs
- TextBounds.cs
- KeyValuePair.cs
- InternalConfigConfigurationFactory.cs
- Pool.cs
- XmlUtil.cs
- DataSvcMapFile.cs
- Visitors.cs
- IDispatchConstantAttribute.cs
- SqlFlattener.cs
- __Filters.cs
- IntPtr.cs
- ModelEditingScope.cs
- InputLanguageEventArgs.cs
- Helper.cs
- DefaultCompensation.cs
- ManagedWndProcTracker.cs
- StorageAssociationSetMapping.cs
- ParameterReplacerVisitor.cs
- FormViewUpdateEventArgs.cs
- XmlDeclaration.cs
- ControlBuilder.cs
- DbParameterCollectionHelper.cs
- sortedlist.cs
- NumberFunctions.cs
- CultureInfoConverter.cs
- PtsHost.cs
- InputEventArgs.cs
- PathGeometry.cs
- NameValuePair.cs
- OutKeywords.cs
- ScriptHandlerFactory.cs
- PackageFilter.cs
- RuleSetBrowserDialog.cs
- GiveFeedbackEvent.cs
- COAUTHINFO.cs
- PackageRelationshipCollection.cs
- StorageAssociationSetMapping.cs
- PersistenceMetadataNamespace.cs
- EpmSyndicationContentDeSerializer.cs
- ResXResourceWriter.cs
- RangeBaseAutomationPeer.cs
- QueryStringHandler.cs
- ScrollData.cs
- Vector3D.cs
- MessageContractExporter.cs
- ForeignKeyConstraint.cs
- HttpListenerResponse.cs