Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / Evaluator.cs / 3 / Evaluator.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides funcletization of expression tree prior to resource binding. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Linq; using System.Linq.Expressions; using System.Diagnostics; #if ASTORIA_LIGHT // Hashset not available ///hack until silver light client is updated internal class HashSet: Dictionary , IEnumerable where T : class { /// hack until silver light client is updated public HashSet() { } ///hack until silver light client is updated public HashSet(IEqualityComparercomparer) : base(comparer) { } /// hack until silver light client is updated public bool Add(T value) { if (!base.ContainsKey(value)) { base.Add(value, value); return true; } return false; } ///hack until silver light client is updated public bool Contains(T value) { return base.ContainsKey(value); } ///hack until silver light client is updated new public bool Remove(T value) { return base.Remove(value); } ///hack until silver light client is updated new public IEnumeratorGetEnumerator() { return base.Keys.GetEnumerator(); } } #endif /// /// performs funcletization on an expression tree /// internal static class Evaluator { ////// Performs evaluation and replacement of independent sub-trees /// /// The root of the expression tree. /// A function that decides whether a given expression node can be part of the local function. ///A new tree with sub-trees evaluated and replaced. internal static Expression PartialEval(Expression expression, FunccanBeEvaluated) { return new SubtreeEvaluator(new Nominator(canBeEvaluated).Nominate(expression)).Eval(expression); } /// /// Performs evaluation and replacement of independent sub-trees /// /// The root of the expression tree. ///A new tree with sub-trees evaluated and replaced. internal static Expression PartialEval(Expression expression) { return PartialEval(expression, Evaluator.CanBeEvaluatedLocally); } ////// Evaluates if an expression can be evaluated locally. /// /// the expression. ///true/ false if can be evaluated locally private static bool CanBeEvaluatedLocally(Expression expression) { return expression.NodeType != ExpressionType.Parameter && expression.NodeType != ExpressionType.Lambda && expression.NodeType != (ExpressionType) ResourceExpressionType.RootResourceSet; } ////// Evaluates and replaces sub-trees when first candidate is reached (top-down) /// internal class SubtreeEvaluator : DataServiceExpressionVisitor { ///list of candidates private HashSetcandidates; /// /// constructs an expression evaluator with a list of candidates /// /// List of expressions to evaluate internal SubtreeEvaluator(HashSetcandidates) { this.candidates = candidates; } /// /// Evaluates an expression sub-tree /// /// The expression to evaluate. ///The evaluated expression. internal Expression Eval(Expression exp) { return this.Visit(exp); } ////// Visit method for visitor /// /// the expression to visit ///visited expression internal override Expression Visit(Expression exp) { if (exp == null) { return null; } if (this.candidates.Contains(exp)) { return Evaluate(exp); } return base.Visit(exp); } ////// Evaluates expression /// /// the expression to evaluate ///constant expression with return value of evaluation private static Expression Evaluate(Expression e) { if (e.NodeType == ExpressionType.Constant) { return e; } LambdaExpression lambda = Expression.Lambda(e); Delegate fn = lambda.Compile(); object value = fn.DynamicInvoke(null); Debug.Assert(!(value is Expression), "!(value is Expression)"); return Expression.Constant(value, e.Type); } } ////// Performs bottom-up analysis to determine which nodes can possibly /// be part of an evaluated sub-tree. /// internal class Nominator : DataServiceExpressionVisitor { ///func to determine whether expression can be evaluated private FuncfunctionCanBeEvaluated; /// candidate expressions for evaluation private HashSetcandidates; /// flag for when sub tree cannot be evaluated private bool cannotBeEvaluated; ////// Creates the Nominator based on the function passed. /// /// /// A Func speficying whether an expression can be evaluated or not. /// ///visited expression internal Nominator(FuncfunctionCanBeEvaluated) { this.functionCanBeEvaluated = functionCanBeEvaluated; } /// /// Nominates an expression to see if it can be evaluated /// /// /// Expression to check /// ///a list of expression sub trees that can be evaluated internal HashSetNominate(Expression expression) { this.candidates = new HashSet (EqualityComparer .Default); this.Visit(expression); return this.candidates; } /// /// Visit method for walking expression tree bottom up. /// /// /// root expression to visit /// ///visited expression internal override Expression Visit(Expression expression) { if (expression != null) { bool saveCannotBeEvaluated = this.cannotBeEvaluated; this.cannotBeEvaluated = false; base.Visit(expression); if (!this.cannotBeEvaluated) { if (this.functionCanBeEvaluated(expression)) { this.candidates.Add(expression); } else { this.cannotBeEvaluated = true; } } this.cannotBeEvaluated |= saveCannotBeEvaluated; } return expression; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides funcletization of expression tree prior to resource binding. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Linq; using System.Linq.Expressions; using System.Diagnostics; #if ASTORIA_LIGHT // Hashset not available ///hack until silver light client is updated internal class HashSet: Dictionary , IEnumerable where T : class { /// hack until silver light client is updated public HashSet() { } ///hack until silver light client is updated public HashSet(IEqualityComparercomparer) : base(comparer) { } /// hack until silver light client is updated public bool Add(T value) { if (!base.ContainsKey(value)) { base.Add(value, value); return true; } return false; } ///hack until silver light client is updated public bool Contains(T value) { return base.ContainsKey(value); } ///hack until silver light client is updated new public bool Remove(T value) { return base.Remove(value); } ///hack until silver light client is updated new public IEnumeratorGetEnumerator() { return base.Keys.GetEnumerator(); } } #endif /// /// performs funcletization on an expression tree /// internal static class Evaluator { ////// Performs evaluation and replacement of independent sub-trees /// /// The root of the expression tree. /// A function that decides whether a given expression node can be part of the local function. ///A new tree with sub-trees evaluated and replaced. internal static Expression PartialEval(Expression expression, FunccanBeEvaluated) { return new SubtreeEvaluator(new Nominator(canBeEvaluated).Nominate(expression)).Eval(expression); } /// /// Performs evaluation and replacement of independent sub-trees /// /// The root of the expression tree. ///A new tree with sub-trees evaluated and replaced. internal static Expression PartialEval(Expression expression) { return PartialEval(expression, Evaluator.CanBeEvaluatedLocally); } ////// Evaluates if an expression can be evaluated locally. /// /// the expression. ///true/ false if can be evaluated locally private static bool CanBeEvaluatedLocally(Expression expression) { return expression.NodeType != ExpressionType.Parameter && expression.NodeType != ExpressionType.Lambda && expression.NodeType != (ExpressionType) ResourceExpressionType.RootResourceSet; } ////// Evaluates and replaces sub-trees when first candidate is reached (top-down) /// internal class SubtreeEvaluator : DataServiceExpressionVisitor { ///list of candidates private HashSetcandidates; /// /// constructs an expression evaluator with a list of candidates /// /// List of expressions to evaluate internal SubtreeEvaluator(HashSetcandidates) { this.candidates = candidates; } /// /// Evaluates an expression sub-tree /// /// The expression to evaluate. ///The evaluated expression. internal Expression Eval(Expression exp) { return this.Visit(exp); } ////// Visit method for visitor /// /// the expression to visit ///visited expression internal override Expression Visit(Expression exp) { if (exp == null) { return null; } if (this.candidates.Contains(exp)) { return Evaluate(exp); } return base.Visit(exp); } ////// Evaluates expression /// /// the expression to evaluate ///constant expression with return value of evaluation private static Expression Evaluate(Expression e) { if (e.NodeType == ExpressionType.Constant) { return e; } LambdaExpression lambda = Expression.Lambda(e); Delegate fn = lambda.Compile(); object value = fn.DynamicInvoke(null); Debug.Assert(!(value is Expression), "!(value is Expression)"); return Expression.Constant(value, e.Type); } } ////// Performs bottom-up analysis to determine which nodes can possibly /// be part of an evaluated sub-tree. /// internal class Nominator : DataServiceExpressionVisitor { ///func to determine whether expression can be evaluated private FuncfunctionCanBeEvaluated; /// candidate expressions for evaluation private HashSetcandidates; /// flag for when sub tree cannot be evaluated private bool cannotBeEvaluated; ////// Creates the Nominator based on the function passed. /// /// /// A Func speficying whether an expression can be evaluated or not. /// ///visited expression internal Nominator(FuncfunctionCanBeEvaluated) { this.functionCanBeEvaluated = functionCanBeEvaluated; } /// /// Nominates an expression to see if it can be evaluated /// /// /// Expression to check /// ///a list of expression sub trees that can be evaluated internal HashSetNominate(Expression expression) { this.candidates = new HashSet (EqualityComparer .Default); this.Visit(expression); return this.candidates; } /// /// Visit method for walking expression tree bottom up. /// /// /// root expression to visit /// ///visited expression internal override Expression Visit(Expression expression) { if (expression != null) { bool saveCannotBeEvaluated = this.cannotBeEvaluated; this.cannotBeEvaluated = false; base.Visit(expression); if (!this.cannotBeEvaluated) { if (this.functionCanBeEvaluated(expression)) { this.candidates.Add(expression); } else { this.cannotBeEvaluated = true; } } this.cannotBeEvaluated |= saveCannotBeEvaluated; } return expression; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
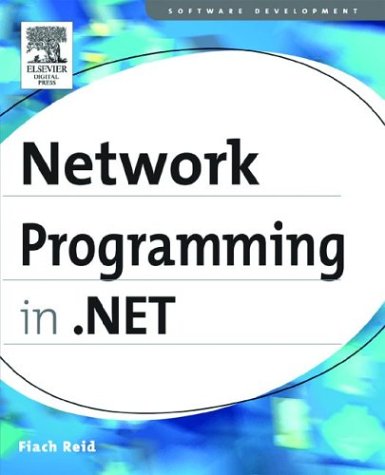
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Button.cs
- DetailsViewRowCollection.cs
- XmlSerializableWriter.cs
- FusionWrap.cs
- RangeValidator.cs
- HttpMethodConstraint.cs
- EnumerableRowCollection.cs
- XmlWriterDelegator.cs
- MeshGeometry3D.cs
- AuthenticationServiceManager.cs
- AttachmentService.cs
- HttpProfileBase.cs
- FontWeights.cs
- SimplePropertyEntry.cs
- OracleSqlParser.cs
- TraceHandler.cs
- RenamedEventArgs.cs
- VirtualDirectoryMapping.cs
- FilteredAttributeCollection.cs
- RoleManagerSection.cs
- InkPresenterAutomationPeer.cs
- SimpleType.cs
- FilteredReadOnlyMetadataCollection.cs
- HttpProxyTransportBindingElement.cs
- CallbackValidator.cs
- OleDbDataAdapter.cs
- ProfileManager.cs
- SQLRoleProvider.cs
- Container.cs
- SkinBuilder.cs
- _HeaderInfo.cs
- EUCJPEncoding.cs
- DBSqlParserColumnCollection.cs
- WebPartCancelEventArgs.cs
- CreateUserWizardStep.cs
- ByteStorage.cs
- ModulesEntry.cs
- SR.cs
- ToolBarPanel.cs
- HealthMonitoringSectionHelper.cs
- EntityContainerEntitySet.cs
- RewritingProcessor.cs
- BitmapEffectRenderDataResource.cs
- EntityContainer.cs
- TreeNode.cs
- TailCallAnalyzer.cs
- MemberAccessException.cs
- CheckBoxRenderer.cs
- XmlUtf8RawTextWriter.cs
- ObjectNotFoundException.cs
- OrderedDictionary.cs
- LinqDataSourceSelectEventArgs.cs
- UpdatePanelTriggerCollection.cs
- CommentEmitter.cs
- EntityCollection.cs
- PropertyContainer.cs
- EventLogEntry.cs
- ValidatingCollection.cs
- SchemaDeclBase.cs
- TableLayoutSettingsTypeConverter.cs
- SmtpAuthenticationManager.cs
- ControlValuePropertyAttribute.cs
- Dispatcher.cs
- Canvas.cs
- BaseTreeIterator.cs
- AsnEncodedData.cs
- WSSecurityTokenSerializer.cs
- ImagingCache.cs
- SqlUDTStorage.cs
- CommandHelper.cs
- ValidatedControlConverter.cs
- FilterableAttribute.cs
- FrameworkTextComposition.cs
- StringDictionary.cs
- TextElementEnumerator.cs
- TaskFileService.cs
- Regex.cs
- SafeFileMapViewHandle.cs
- BaseCodePageEncoding.cs
- Point.cs
- MetadataExchangeBindings.cs
- UnsignedPublishLicense.cs
- AcceptorSessionSymmetricTransportSecurityProtocol.cs
- OrthographicCamera.cs
- XmlConverter.cs
- DashStyle.cs
- LayoutDump.cs
- PasswordPropertyTextAttribute.cs
- OleDbPermission.cs
- HtmlMeta.cs
- FixedSOMTextRun.cs
- ClientSettings.cs
- InstallerTypeAttribute.cs
- DirectionalLight.cs
- ToggleProviderWrapper.cs
- ClientFormsIdentity.cs
- XmlSchemaImporter.cs
- SqlUnionizer.cs
- CollectionViewGroup.cs
- RegexRunner.cs