Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / Globalization / BamlTreeNode.cs / 1 / BamlTreeNode.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: BamlTreeNode structures // // History: // 3/30/2005 garyyang - created it // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using System.Windows.Markup; namespace MS.Internal.Globalization { #region BamlTree ////// This reprenents a BamlTree. /// It contains two views of the tree. /// 1) the tree itself through BamlTreeNode. It maintains the Baml /// file structure for serialization /// 2) flat baml through in depth first order /// it will be used when creating flat baml resources /// internal sealed class BamlTree { //---------------------------------- // Constructor //---------------------------------- ////// create an emtpy baml tree /// internal BamlTree(){} ////// Create a baml tree on a root node. /// internal BamlTree(BamlTreeNode root, int size) { Debug.Assert(root != null, "Baml tree root is null!"); Debug.Assert(size > 0, "Baml tree size is less than 1"); _root = root; _nodeList = new List(size); CreateInternalIndex(ref _root, ref _nodeList, false); } internal BamlTreeNode Root { get { return _root;} } internal int Size { get { return _nodeList.Count;} } // index into the flattened baml tree internal BamlTreeNode this[int i] { get{ return _nodeList[i]; } } // create a deep copy of the tree internal BamlTree Copy() { // create new root and new list BamlTreeNode newTreeRoot = _root; List newNodeList = new List (Size); // create a new copy of the tree. CreateInternalIndex(ref newTreeRoot, ref newNodeList, true); BamlTree newTree = new BamlTree(); newTree._root = newTreeRoot; newTree._nodeList = newNodeList; return newTree; } // adds a node into the flattened tree node list. internal void AddTreeNode(BamlTreeNode node) { _nodeList.Add(node); } // travese the tree and store the node in the arraylist in the same order as travesals. // it can also create a a new tree by give true to "toCopy" private void CreateInternalIndex(ref BamlTreeNode parent, ref List nodeList, bool toCopy) { // gets the old children List children = parent.Children; if (toCopy) { // creates a copy of the current node parent = parent.Copy(); if (children != null) { // create an new list if there are children. parent.Children = new List (children.Count); } } // add the node to the flattened list nodeList.Add(parent); // return if this is the leaf if (children == null) return; // for each child for (int i = 0; i < children.Count; i++) { // get to the child BamlTreeNode child = children[i]; // recursively create index CreateInternalIndex(ref child, ref nodeList, toCopy); if (toCopy) { // if toCopy, we link the new child and new parent. child.Parent = parent; parent.Children.Add(child); } } } private BamlTreeNode _root; // the root of the tree private List _nodeList; // stores flattened baml tree in depth first order } #endregion #region ILocalizabilityInheritable inteface /// /// Nodes in the Baml tree can inherit localizability from parent nodes. Nodes that /// implements this interface will be part of the localizabilty inheritance tree. /// internal interface ILocalizabilityInheritable { ////// The ancestor node to inherit localizability from /// ILocalizabilityInheritable LocalizabilityAncestor {get;} ////// The inheritable attributs to child nodes. /// LocalizabilityAttribute InheritableAttribute { get; set; } bool IsIgnored { get; set; } } #endregion #region BamlTreeNode and sub-classes ////// The node for the internal baml tree /// internal abstract class BamlTreeNode { //--------------------------- // Constructor //--------------------------- internal BamlTreeNode(BamlNodeType type) { NodeType = type; } //---------------------------- // internal methods //---------------------------- ////// Add a child to this node. /// /// child node to add internal void AddChild(BamlTreeNode child) { if (_children == null) { _children = new List(); } _children.Add(child); // Add the children child.Parent = this; // Link to its parent } /// /// Create a copy of the BamlTreeNode /// internal abstract BamlTreeNode Copy(); ////// Serialize the node through BamlWriter /// internal abstract void Serialize(BamlWriter writer); //---------------------------- // internal properties. //---------------------------- ////// NodeType /// ///BamlNodeType internal BamlNodeType NodeType { get { return _nodeType; } set { _nodeType = value; } } ////// List of children nodes. /// internal ListChildren { get { return _children; } set { _children = value; } } /// /// Parent node. /// ///BamlTreeNode internal BamlTreeNode Parent { get { return _parent; } set { _parent = value; } } ////// Whether the content of this node has been formatted to be part of /// parent node's content. /// internal bool Formatted { get { return (_state & BamlTreeNodeState.ContentFormatted) != 0; } set { if (value) { _state |= BamlTreeNodeState.ContentFormatted; } else { _state &= (~BamlTreeNodeState.ContentFormatted); } } } ////// Whether the node has been visited. Used to detect loop in the tree. /// internal bool Visited { get { return (_state & BamlTreeNodeState.NodeVisited) != 0; } set { if (value) { _state |= BamlTreeNodeState.NodeVisited; } else { _state &= (~BamlTreeNodeState.NodeVisited); } } } ////// Indicate if the node is not uniquely identifiable in the tree. Its value /// is true when the node or its parent doesn't have unique Uid. /// internal bool Unidentifiable { get { return (_state & BamlTreeNodeState.Unidentifiable) != 0; } set { if (value) { _state |= BamlTreeNodeState.Unidentifiable; } else { _state &= (~BamlTreeNodeState.Unidentifiable); } } } //---------------------------- // protected members //---------------------------- protected BamlNodeType _nodeType; // node type protected List_children; // the children list. protected BamlTreeNode _parent ; // the tree parent of this node private BamlTreeNodeState _state; // the state of this tree node [Flags] private enum BamlTreeNodeState : byte { /// /// Default state /// None = 0, ////// Indicate that the content of this node has been formatted inline. We don't need to /// produce an individual localizable resource for it. /// ContentFormatted = 1, ////// Indicate that this node has already been visited once in the tree traversal (such as serialization). /// It is used to prevent the Baml tree contains multiple references to one node. /// NodeVisited = 2, ////// Indicate that this node cannot be uniquely identify in the Baml tree. It happens when /// it or its parent element has a duplicate Uid. /// Unidentifiable = 4, } } ////// Baml StartDocument node /// internal sealed class BamlStartDocumentNode : BamlTreeNode, ILocalizabilityInheritable { internal BamlStartDocumentNode() : base(BamlNodeType.StartDocument){} internal override void Serialize(BamlWriter writer) { writer.WriteStartDocument(); } internal override BamlTreeNode Copy() { return new BamlStartDocumentNode(); } public ILocalizabilityInheritable LocalizabilityAncestor { // return null as StartDocument is the root of the inheritance tree get { return null; } } public LocalizabilityAttribute InheritableAttribute { get { // return the default attribute as it is at the root of the inheritance LocalizabilityAttribute defaultAttribute = new LocalizabilityAttribute(LocalizationCategory.None); defaultAttribute.Readability = Readability.Readable; defaultAttribute.Modifiability = Modifiability.Modifiable; return defaultAttribute; } set {} } public bool IsIgnored { get { return false; } set {} } } ////// Baml EndDocument node /// internal sealed class BamlEndDocumentNode : BamlTreeNode { internal BamlEndDocumentNode() : base(BamlNodeType.EndDocument) {} internal override void Serialize(BamlWriter writer) { writer.WriteEndDocument(); } internal override BamlTreeNode Copy() { return new BamlEndDocumentNode(); } } ////// Baml ConnectionId node /// internal sealed class BamlConnectionIdNode : BamlTreeNode { internal BamlConnectionIdNode(Int32 connectionId) : base(BamlNodeType.ConnectionId) { _connectionId = connectionId; } internal override void Serialize(BamlWriter writer) { writer.WriteConnectionId(_connectionId); } internal override BamlTreeNode Copy() { return new BamlConnectionIdNode(_connectionId); } private Int32 _connectionId; } ////// Baml StartElement node, it can also inherit localizability attributes from parent nodes. /// internal sealed class BamlStartElementNode : BamlTreeNode, ILocalizabilityInheritable { internal BamlStartElementNode( string assemblyName, string typeFullName, bool isInjected, bool useTypeConverter ): base (BamlNodeType.StartElement) { _assemblyName = assemblyName; _typeFullName = typeFullName; _isInjected = isInjected; _useTypeConverter = useTypeConverter; } internal override void Serialize(BamlWriter writer) { writer.WriteStartElement(_assemblyName, _typeFullName, _isInjected, _useTypeConverter); } internal override BamlTreeNode Copy() { BamlStartElementNode node = new BamlStartElementNode(_assemblyName, _typeFullName, _isInjected, _useTypeConverter); node._content = _content; node._uid = _uid; node._inheritableAttribute = _inheritableAttribute; return node; } ////// insert property node /// ////// Property node needs to be group in front of /// child elements. This method is called when creating a /// new property node. /// internal void InsertProperty(BamlTreeNode child) { if (_children == null) { AddChild(child); } else { int lastProperty = 0; for (int i = 0; i < _children.Count; i++) { if (_children[i].NodeType == BamlNodeType.Property) { lastProperty = i; } } _children.Insert(lastProperty, child); child.Parent = this; } } //------------------------------- // Internal properties //------------------------------- internal string AssemblyName { get { return _assemblyName; } } internal string TypeFullName { get { return _typeFullName; } } internal string Content { get { return _content; } set { _content = value; } } internal string Uid { get { return _uid; } set { _uid = value;} } public ILocalizabilityInheritable LocalizabilityAncestor { // Baml element node inherit from parent element get { if (_localizabilityAncestor == null) { // walk up the tree to find a parent node that is ILocalizabilityInheritable for (BamlTreeNode parentNode = Parent; _localizabilityAncestor == null && parentNode != null; parentNode = parentNode.Parent) { _localizabilityAncestor = (parentNode as ILocalizabilityInheritable); } } return _localizabilityAncestor; } } public LocalizabilityAttribute InheritableAttribute { get { return _inheritableAttribute; } set { Debug.Assert(value.Category != LocalizationCategory.Ignore && value.Category != LocalizationCategory.Inherit); _inheritableAttribute = value; } } public bool IsIgnored { get { return _isIgnored; } set { _isIgnored = value; } } //---------------------- // Private members //---------------------- private string _assemblyName; private string _typeFullName; private string _content; private string _uid; private LocalizabilityAttribute _inheritableAttribute; private ILocalizabilityInheritable _localizabilityAncestor; private bool _isIgnored; private bool _isInjected; private bool _useTypeConverter; } ////// Baml EndElement node /// internal sealed class BamlEndElementNode : BamlTreeNode { internal BamlEndElementNode() : base(BamlNodeType.EndElement) { } internal override void Serialize(BamlWriter writer) { writer.WriteEndElement(); } internal override BamlTreeNode Copy() { return new BamlEndElementNode(); } } ////// Baml XmlnsProperty node /// internal sealed class BamlXmlnsPropertyNode : BamlTreeNode { internal BamlXmlnsPropertyNode( string prefix, string xmlns ): base(BamlNodeType.XmlnsProperty) { _prefix = prefix; _xmlns = xmlns; } internal override void Serialize(BamlWriter writer) { writer.WriteXmlnsProperty(_prefix, _xmlns); } internal override BamlTreeNode Copy() { return new BamlXmlnsPropertyNode(_prefix, _xmlns); } private string _prefix; private string _xmlns; } ////// StartComplexProperty node /// internal class BamlStartComplexPropertyNode : BamlTreeNode, ILocalizabilityInheritable { internal BamlStartComplexPropertyNode( string assemblyName, string ownerTypeFullName, string propertyName ) : base(BamlNodeType.StartComplexProperty) { _assemblyName = assemblyName; _ownerTypeFullName = ownerTypeFullName; _propertyName = propertyName; } internal override void Serialize(BamlWriter writer) { writer.WriteStartComplexProperty( _assemblyName, _ownerTypeFullName, _propertyName ); } internal override BamlTreeNode Copy() { return new BamlStartComplexPropertyNode( _assemblyName, _ownerTypeFullName, _propertyName ); } internal string AssemblyName { get { return _assemblyName; } } internal string PropertyName { get { return _propertyName; } } internal string OwnerTypeFullName { get { return _ownerTypeFullName; } } public ILocalizabilityInheritable LocalizabilityAncestor { get { return _localizabilityAncestor; } set { _localizabilityAncestor = value; } } public LocalizabilityAttribute InheritableAttribute { get { return _inheritableAttribute; } set { Debug.Assert(value.Category != LocalizationCategory.Ignore && value.Category != LocalizationCategory.Inherit); _inheritableAttribute = value; } } public bool IsIgnored { get { return _isIgnored; } set { _isIgnored = value; } } protected string _assemblyName; protected string _ownerTypeFullName; protected string _propertyName; private ILocalizabilityInheritable _localizabilityAncestor; private LocalizabilityAttribute _inheritableAttribute; private bool _isIgnored; } ////// EndComplexProperty node /// internal sealed class BamlEndComplexPropertyNode : BamlTreeNode { internal BamlEndComplexPropertyNode() : base(BamlNodeType.EndComplexProperty) { } internal override void Serialize(BamlWriter writer) { writer.WriteEndComplexProperty(); } internal override BamlTreeNode Copy() { return new BamlEndComplexPropertyNode(); } } ////// Baml Property node, it can inherit localizable attributes from parent nodes. /// internal sealed class BamlPropertyNode : BamlStartComplexPropertyNode { internal BamlPropertyNode( string assemblyName, string ownerTypeFullName, string propertyName, string value, BamlAttributeUsage usage ): base(assemblyName, ownerTypeFullName, propertyName) { _value = value; _attributeUsage = usage; _nodeType = BamlNodeType.Property; } internal override void Serialize(BamlWriter writer) { // skip seralizing Localization.Comments and Localization.Attributes properties if (!LocComments.IsLocCommentsProperty(_ownerTypeFullName, _propertyName) && !LocComments.IsLocLocalizabilityProperty(_ownerTypeFullName, _propertyName)) { writer.WriteProperty( _assemblyName, _ownerTypeFullName, _propertyName, _value, _attributeUsage ); } } internal override BamlTreeNode Copy() { return new BamlPropertyNode( _assemblyName, _ownerTypeFullName, _propertyName, _value, _attributeUsage ); } internal string Value { get { return _value; } set { _value = value; } } internal int Index { get { return _index; } set { _index = value; } } private string _value; private BamlAttributeUsage _attributeUsage; private int _index = 0; // used for auto-numbering repeated properties within an element } ////// LiteralContent node /// internal sealed class BamlLiteralContentNode : BamlTreeNode { internal BamlLiteralContentNode(string literalContent) : base(BamlNodeType.LiteralContent) { _literalContent = literalContent; } internal override void Serialize(BamlWriter writer) { writer.WriteLiteralContent(_literalContent); } internal override BamlTreeNode Copy() { return new BamlLiteralContentNode(_literalContent); } internal string Content { get { return _literalContent; } set { _literalContent = value; } } private string _literalContent; } ////// Text node /// internal sealed class BamlTextNode : BamlTreeNode { internal BamlTextNode(string text) : this (text, null, null) { } internal BamlTextNode( string text, string typeConverterAssemblyName, string typeConverterName ) : base(BamlNodeType.Text) { _content = text; _typeConverterAssemblyName = typeConverterAssemblyName; _typeConverterName = typeConverterName; } internal override void Serialize(BamlWriter writer) { writer.WriteText(_content, _typeConverterAssemblyName, _typeConverterName); } internal override BamlTreeNode Copy() { return new BamlTextNode(_content, _typeConverterAssemblyName, _typeConverterName); } internal string Content { get { return _content; } } private string _content; private string _typeConverterAssemblyName; private string _typeConverterName; } ////// Routed event node /// internal sealed class BamlRoutedEventNode : BamlTreeNode { internal BamlRoutedEventNode( string assemblyName, string ownerTypeFullName, string eventIdName, string handlerName ) : base(BamlNodeType.RoutedEvent) { _assemblyName = assemblyName; _ownerTypeFullName = ownerTypeFullName; _eventIdName = eventIdName; _handlerName = handlerName; } internal override void Serialize(BamlWriter writer) { writer.WriteRoutedEvent( _assemblyName, _ownerTypeFullName, _eventIdName, _handlerName ); } internal override BamlTreeNode Copy() { return new BamlRoutedEventNode( _assemblyName, _ownerTypeFullName, _eventIdName, _handlerName ); } private string _assemblyName; private string _ownerTypeFullName; private string _eventIdName; private string _handlerName; } ////// event node /// internal sealed class BamlEventNode : BamlTreeNode { internal BamlEventNode( string eventName, string handlerName ) : base(BamlNodeType.Event) { _eventName = eventName; _handlerName = handlerName; } internal override void Serialize(BamlWriter writer) { writer.WriteEvent( _eventName, _handlerName ); } internal override BamlTreeNode Copy() { return new BamlEventNode(_eventName, _handlerName); } private string _eventName; private string _handlerName; } ////// DefAttribute node /// internal sealed class BamlDefAttributeNode : BamlTreeNode { internal BamlDefAttributeNode(string name, string value) : base(BamlNodeType.DefAttribute) { _name = name; _value = value; } internal override void Serialize(BamlWriter writer) { writer.WriteDefAttribute(_name, _value); } internal override BamlTreeNode Copy() { return new BamlDefAttributeNode(_name, _value); } private string _name; private string _value; } ////// PIMapping node /// internal sealed class BamlPIMappingNode : BamlTreeNode { internal BamlPIMappingNode( string xmlNamespace, string clrNamespace, string assemblyName ) : base(BamlNodeType.PIMapping) { _xmlNamespace = xmlNamespace; _clrNamespace = clrNamespace; _assemblyName = assemblyName; } internal override void Serialize(BamlWriter writer) { writer.WritePIMapping( _xmlNamespace, _clrNamespace, _assemblyName ); } internal override BamlTreeNode Copy() { return new BamlPIMappingNode( _xmlNamespace, _clrNamespace, _assemblyName ); } private string _xmlNamespace; private string _clrNamespace; private string _assemblyName; } ////// StartConstructor node /// internal sealed class BamlStartConstructorNode : BamlTreeNode { internal BamlStartConstructorNode(): base(BamlNodeType.StartConstructor) { } internal override void Serialize(BamlWriter writer) { writer.WriteStartConstructor(); } internal override BamlTreeNode Copy() { return new BamlStartConstructorNode(); } } ////// EndConstructor node /// internal sealed class BamlEndConstructorNode : BamlTreeNode { internal BamlEndConstructorNode(): base(BamlNodeType.EndConstructor) { } internal override void Serialize(BamlWriter writer) { writer.WriteEndConstructor(); } internal override BamlTreeNode Copy() { return new BamlEndConstructorNode(); } } ////////// ContentProperty node ///// internal sealed class BamlContentPropertyNode : BamlTreeNode { internal BamlContentPropertyNode( string assemblyName, string typeFullName, string propertyName ) : base(BamlNodeType.ContentProperty) { _assemblyName = assemblyName; _typeFullName = typeFullName; _propertyName = propertyName; } internal override void Serialize(BamlWriter writer) { writer.WriteContentProperty( _assemblyName, _typeFullName, _propertyName ); } internal override BamlTreeNode Copy() { return new BamlContentPropertyNode( _assemblyName, _typeFullName, _propertyName ); } private string _assemblyName; private string _typeFullName; private string _propertyName; } internal sealed class BamlPresentationOptionsAttributeNode : BamlTreeNode { internal BamlPresentationOptionsAttributeNode(string name, string value) : base(BamlNodeType.PresentationOptionsAttribute) { _name = name; _value = value; } internal override void Serialize(BamlWriter writer) { writer.WritePresentationOptionsAttribute(_name, _value); } internal override BamlTreeNode Copy() { return new BamlPresentationOptionsAttributeNode(_name, _value); } private string _name; private string _value; } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: BamlTreeNode structures // // History: // 3/30/2005 garyyang - created it // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using System.Windows.Markup; namespace MS.Internal.Globalization { #region BamlTree ////// This reprenents a BamlTree. /// It contains two views of the tree. /// 1) the tree itself through BamlTreeNode. It maintains the Baml /// file structure for serialization /// 2) flat baml through in depth first order /// it will be used when creating flat baml resources /// internal sealed class BamlTree { //---------------------------------- // Constructor //---------------------------------- ////// create an emtpy baml tree /// internal BamlTree(){} ////// Create a baml tree on a root node. /// internal BamlTree(BamlTreeNode root, int size) { Debug.Assert(root != null, "Baml tree root is null!"); Debug.Assert(size > 0, "Baml tree size is less than 1"); _root = root; _nodeList = new List(size); CreateInternalIndex(ref _root, ref _nodeList, false); } internal BamlTreeNode Root { get { return _root;} } internal int Size { get { return _nodeList.Count;} } // index into the flattened baml tree internal BamlTreeNode this[int i] { get{ return _nodeList[i]; } } // create a deep copy of the tree internal BamlTree Copy() { // create new root and new list BamlTreeNode newTreeRoot = _root; List newNodeList = new List (Size); // create a new copy of the tree. CreateInternalIndex(ref newTreeRoot, ref newNodeList, true); BamlTree newTree = new BamlTree(); newTree._root = newTreeRoot; newTree._nodeList = newNodeList; return newTree; } // adds a node into the flattened tree node list. internal void AddTreeNode(BamlTreeNode node) { _nodeList.Add(node); } // travese the tree and store the node in the arraylist in the same order as travesals. // it can also create a a new tree by give true to "toCopy" private void CreateInternalIndex(ref BamlTreeNode parent, ref List nodeList, bool toCopy) { // gets the old children List children = parent.Children; if (toCopy) { // creates a copy of the current node parent = parent.Copy(); if (children != null) { // create an new list if there are children. parent.Children = new List (children.Count); } } // add the node to the flattened list nodeList.Add(parent); // return if this is the leaf if (children == null) return; // for each child for (int i = 0; i < children.Count; i++) { // get to the child BamlTreeNode child = children[i]; // recursively create index CreateInternalIndex(ref child, ref nodeList, toCopy); if (toCopy) { // if toCopy, we link the new child and new parent. child.Parent = parent; parent.Children.Add(child); } } } private BamlTreeNode _root; // the root of the tree private List _nodeList; // stores flattened baml tree in depth first order } #endregion #region ILocalizabilityInheritable inteface /// /// Nodes in the Baml tree can inherit localizability from parent nodes. Nodes that /// implements this interface will be part of the localizabilty inheritance tree. /// internal interface ILocalizabilityInheritable { ////// The ancestor node to inherit localizability from /// ILocalizabilityInheritable LocalizabilityAncestor {get;} ////// The inheritable attributs to child nodes. /// LocalizabilityAttribute InheritableAttribute { get; set; } bool IsIgnored { get; set; } } #endregion #region BamlTreeNode and sub-classes ////// The node for the internal baml tree /// internal abstract class BamlTreeNode { //--------------------------- // Constructor //--------------------------- internal BamlTreeNode(BamlNodeType type) { NodeType = type; } //---------------------------- // internal methods //---------------------------- ////// Add a child to this node. /// /// child node to add internal void AddChild(BamlTreeNode child) { if (_children == null) { _children = new List(); } _children.Add(child); // Add the children child.Parent = this; // Link to its parent } /// /// Create a copy of the BamlTreeNode /// internal abstract BamlTreeNode Copy(); ////// Serialize the node through BamlWriter /// internal abstract void Serialize(BamlWriter writer); //---------------------------- // internal properties. //---------------------------- ////// NodeType /// ///BamlNodeType internal BamlNodeType NodeType { get { return _nodeType; } set { _nodeType = value; } } ////// List of children nodes. /// internal ListChildren { get { return _children; } set { _children = value; } } /// /// Parent node. /// ///BamlTreeNode internal BamlTreeNode Parent { get { return _parent; } set { _parent = value; } } ////// Whether the content of this node has been formatted to be part of /// parent node's content. /// internal bool Formatted { get { return (_state & BamlTreeNodeState.ContentFormatted) != 0; } set { if (value) { _state |= BamlTreeNodeState.ContentFormatted; } else { _state &= (~BamlTreeNodeState.ContentFormatted); } } } ////// Whether the node has been visited. Used to detect loop in the tree. /// internal bool Visited { get { return (_state & BamlTreeNodeState.NodeVisited) != 0; } set { if (value) { _state |= BamlTreeNodeState.NodeVisited; } else { _state &= (~BamlTreeNodeState.NodeVisited); } } } ////// Indicate if the node is not uniquely identifiable in the tree. Its value /// is true when the node or its parent doesn't have unique Uid. /// internal bool Unidentifiable { get { return (_state & BamlTreeNodeState.Unidentifiable) != 0; } set { if (value) { _state |= BamlTreeNodeState.Unidentifiable; } else { _state &= (~BamlTreeNodeState.Unidentifiable); } } } //---------------------------- // protected members //---------------------------- protected BamlNodeType _nodeType; // node type protected List_children; // the children list. protected BamlTreeNode _parent ; // the tree parent of this node private BamlTreeNodeState _state; // the state of this tree node [Flags] private enum BamlTreeNodeState : byte { /// /// Default state /// None = 0, ////// Indicate that the content of this node has been formatted inline. We don't need to /// produce an individual localizable resource for it. /// ContentFormatted = 1, ////// Indicate that this node has already been visited once in the tree traversal (such as serialization). /// It is used to prevent the Baml tree contains multiple references to one node. /// NodeVisited = 2, ////// Indicate that this node cannot be uniquely identify in the Baml tree. It happens when /// it or its parent element has a duplicate Uid. /// Unidentifiable = 4, } } ////// Baml StartDocument node /// internal sealed class BamlStartDocumentNode : BamlTreeNode, ILocalizabilityInheritable { internal BamlStartDocumentNode() : base(BamlNodeType.StartDocument){} internal override void Serialize(BamlWriter writer) { writer.WriteStartDocument(); } internal override BamlTreeNode Copy() { return new BamlStartDocumentNode(); } public ILocalizabilityInheritable LocalizabilityAncestor { // return null as StartDocument is the root of the inheritance tree get { return null; } } public LocalizabilityAttribute InheritableAttribute { get { // return the default attribute as it is at the root of the inheritance LocalizabilityAttribute defaultAttribute = new LocalizabilityAttribute(LocalizationCategory.None); defaultAttribute.Readability = Readability.Readable; defaultAttribute.Modifiability = Modifiability.Modifiable; return defaultAttribute; } set {} } public bool IsIgnored { get { return false; } set {} } } ////// Baml EndDocument node /// internal sealed class BamlEndDocumentNode : BamlTreeNode { internal BamlEndDocumentNode() : base(BamlNodeType.EndDocument) {} internal override void Serialize(BamlWriter writer) { writer.WriteEndDocument(); } internal override BamlTreeNode Copy() { return new BamlEndDocumentNode(); } } ////// Baml ConnectionId node /// internal sealed class BamlConnectionIdNode : BamlTreeNode { internal BamlConnectionIdNode(Int32 connectionId) : base(BamlNodeType.ConnectionId) { _connectionId = connectionId; } internal override void Serialize(BamlWriter writer) { writer.WriteConnectionId(_connectionId); } internal override BamlTreeNode Copy() { return new BamlConnectionIdNode(_connectionId); } private Int32 _connectionId; } ////// Baml StartElement node, it can also inherit localizability attributes from parent nodes. /// internal sealed class BamlStartElementNode : BamlTreeNode, ILocalizabilityInheritable { internal BamlStartElementNode( string assemblyName, string typeFullName, bool isInjected, bool useTypeConverter ): base (BamlNodeType.StartElement) { _assemblyName = assemblyName; _typeFullName = typeFullName; _isInjected = isInjected; _useTypeConverter = useTypeConverter; } internal override void Serialize(BamlWriter writer) { writer.WriteStartElement(_assemblyName, _typeFullName, _isInjected, _useTypeConverter); } internal override BamlTreeNode Copy() { BamlStartElementNode node = new BamlStartElementNode(_assemblyName, _typeFullName, _isInjected, _useTypeConverter); node._content = _content; node._uid = _uid; node._inheritableAttribute = _inheritableAttribute; return node; } ////// insert property node /// ////// Property node needs to be group in front of /// child elements. This method is called when creating a /// new property node. /// internal void InsertProperty(BamlTreeNode child) { if (_children == null) { AddChild(child); } else { int lastProperty = 0; for (int i = 0; i < _children.Count; i++) { if (_children[i].NodeType == BamlNodeType.Property) { lastProperty = i; } } _children.Insert(lastProperty, child); child.Parent = this; } } //------------------------------- // Internal properties //------------------------------- internal string AssemblyName { get { return _assemblyName; } } internal string TypeFullName { get { return _typeFullName; } } internal string Content { get { return _content; } set { _content = value; } } internal string Uid { get { return _uid; } set { _uid = value;} } public ILocalizabilityInheritable LocalizabilityAncestor { // Baml element node inherit from parent element get { if (_localizabilityAncestor == null) { // walk up the tree to find a parent node that is ILocalizabilityInheritable for (BamlTreeNode parentNode = Parent; _localizabilityAncestor == null && parentNode != null; parentNode = parentNode.Parent) { _localizabilityAncestor = (parentNode as ILocalizabilityInheritable); } } return _localizabilityAncestor; } } public LocalizabilityAttribute InheritableAttribute { get { return _inheritableAttribute; } set { Debug.Assert(value.Category != LocalizationCategory.Ignore && value.Category != LocalizationCategory.Inherit); _inheritableAttribute = value; } } public bool IsIgnored { get { return _isIgnored; } set { _isIgnored = value; } } //---------------------- // Private members //---------------------- private string _assemblyName; private string _typeFullName; private string _content; private string _uid; private LocalizabilityAttribute _inheritableAttribute; private ILocalizabilityInheritable _localizabilityAncestor; private bool _isIgnored; private bool _isInjected; private bool _useTypeConverter; } ////// Baml EndElement node /// internal sealed class BamlEndElementNode : BamlTreeNode { internal BamlEndElementNode() : base(BamlNodeType.EndElement) { } internal override void Serialize(BamlWriter writer) { writer.WriteEndElement(); } internal override BamlTreeNode Copy() { return new BamlEndElementNode(); } } ////// Baml XmlnsProperty node /// internal sealed class BamlXmlnsPropertyNode : BamlTreeNode { internal BamlXmlnsPropertyNode( string prefix, string xmlns ): base(BamlNodeType.XmlnsProperty) { _prefix = prefix; _xmlns = xmlns; } internal override void Serialize(BamlWriter writer) { writer.WriteXmlnsProperty(_prefix, _xmlns); } internal override BamlTreeNode Copy() { return new BamlXmlnsPropertyNode(_prefix, _xmlns); } private string _prefix; private string _xmlns; } ////// StartComplexProperty node /// internal class BamlStartComplexPropertyNode : BamlTreeNode, ILocalizabilityInheritable { internal BamlStartComplexPropertyNode( string assemblyName, string ownerTypeFullName, string propertyName ) : base(BamlNodeType.StartComplexProperty) { _assemblyName = assemblyName; _ownerTypeFullName = ownerTypeFullName; _propertyName = propertyName; } internal override void Serialize(BamlWriter writer) { writer.WriteStartComplexProperty( _assemblyName, _ownerTypeFullName, _propertyName ); } internal override BamlTreeNode Copy() { return new BamlStartComplexPropertyNode( _assemblyName, _ownerTypeFullName, _propertyName ); } internal string AssemblyName { get { return _assemblyName; } } internal string PropertyName { get { return _propertyName; } } internal string OwnerTypeFullName { get { return _ownerTypeFullName; } } public ILocalizabilityInheritable LocalizabilityAncestor { get { return _localizabilityAncestor; } set { _localizabilityAncestor = value; } } public LocalizabilityAttribute InheritableAttribute { get { return _inheritableAttribute; } set { Debug.Assert(value.Category != LocalizationCategory.Ignore && value.Category != LocalizationCategory.Inherit); _inheritableAttribute = value; } } public bool IsIgnored { get { return _isIgnored; } set { _isIgnored = value; } } protected string _assemblyName; protected string _ownerTypeFullName; protected string _propertyName; private ILocalizabilityInheritable _localizabilityAncestor; private LocalizabilityAttribute _inheritableAttribute; private bool _isIgnored; } ////// EndComplexProperty node /// internal sealed class BamlEndComplexPropertyNode : BamlTreeNode { internal BamlEndComplexPropertyNode() : base(BamlNodeType.EndComplexProperty) { } internal override void Serialize(BamlWriter writer) { writer.WriteEndComplexProperty(); } internal override BamlTreeNode Copy() { return new BamlEndComplexPropertyNode(); } } ////// Baml Property node, it can inherit localizable attributes from parent nodes. /// internal sealed class BamlPropertyNode : BamlStartComplexPropertyNode { internal BamlPropertyNode( string assemblyName, string ownerTypeFullName, string propertyName, string value, BamlAttributeUsage usage ): base(assemblyName, ownerTypeFullName, propertyName) { _value = value; _attributeUsage = usage; _nodeType = BamlNodeType.Property; } internal override void Serialize(BamlWriter writer) { // skip seralizing Localization.Comments and Localization.Attributes properties if (!LocComments.IsLocCommentsProperty(_ownerTypeFullName, _propertyName) && !LocComments.IsLocLocalizabilityProperty(_ownerTypeFullName, _propertyName)) { writer.WriteProperty( _assemblyName, _ownerTypeFullName, _propertyName, _value, _attributeUsage ); } } internal override BamlTreeNode Copy() { return new BamlPropertyNode( _assemblyName, _ownerTypeFullName, _propertyName, _value, _attributeUsage ); } internal string Value { get { return _value; } set { _value = value; } } internal int Index { get { return _index; } set { _index = value; } } private string _value; private BamlAttributeUsage _attributeUsage; private int _index = 0; // used for auto-numbering repeated properties within an element } ////// LiteralContent node /// internal sealed class BamlLiteralContentNode : BamlTreeNode { internal BamlLiteralContentNode(string literalContent) : base(BamlNodeType.LiteralContent) { _literalContent = literalContent; } internal override void Serialize(BamlWriter writer) { writer.WriteLiteralContent(_literalContent); } internal override BamlTreeNode Copy() { return new BamlLiteralContentNode(_literalContent); } internal string Content { get { return _literalContent; } set { _literalContent = value; } } private string _literalContent; } ////// Text node /// internal sealed class BamlTextNode : BamlTreeNode { internal BamlTextNode(string text) : this (text, null, null) { } internal BamlTextNode( string text, string typeConverterAssemblyName, string typeConverterName ) : base(BamlNodeType.Text) { _content = text; _typeConverterAssemblyName = typeConverterAssemblyName; _typeConverterName = typeConverterName; } internal override void Serialize(BamlWriter writer) { writer.WriteText(_content, _typeConverterAssemblyName, _typeConverterName); } internal override BamlTreeNode Copy() { return new BamlTextNode(_content, _typeConverterAssemblyName, _typeConverterName); } internal string Content { get { return _content; } } private string _content; private string _typeConverterAssemblyName; private string _typeConverterName; } ////// Routed event node /// internal sealed class BamlRoutedEventNode : BamlTreeNode { internal BamlRoutedEventNode( string assemblyName, string ownerTypeFullName, string eventIdName, string handlerName ) : base(BamlNodeType.RoutedEvent) { _assemblyName = assemblyName; _ownerTypeFullName = ownerTypeFullName; _eventIdName = eventIdName; _handlerName = handlerName; } internal override void Serialize(BamlWriter writer) { writer.WriteRoutedEvent( _assemblyName, _ownerTypeFullName, _eventIdName, _handlerName ); } internal override BamlTreeNode Copy() { return new BamlRoutedEventNode( _assemblyName, _ownerTypeFullName, _eventIdName, _handlerName ); } private string _assemblyName; private string _ownerTypeFullName; private string _eventIdName; private string _handlerName; } ////// event node /// internal sealed class BamlEventNode : BamlTreeNode { internal BamlEventNode( string eventName, string handlerName ) : base(BamlNodeType.Event) { _eventName = eventName; _handlerName = handlerName; } internal override void Serialize(BamlWriter writer) { writer.WriteEvent( _eventName, _handlerName ); } internal override BamlTreeNode Copy() { return new BamlEventNode(_eventName, _handlerName); } private string _eventName; private string _handlerName; } ////// DefAttribute node /// internal sealed class BamlDefAttributeNode : BamlTreeNode { internal BamlDefAttributeNode(string name, string value) : base(BamlNodeType.DefAttribute) { _name = name; _value = value; } internal override void Serialize(BamlWriter writer) { writer.WriteDefAttribute(_name, _value); } internal override BamlTreeNode Copy() { return new BamlDefAttributeNode(_name, _value); } private string _name; private string _value; } ////// PIMapping node /// internal sealed class BamlPIMappingNode : BamlTreeNode { internal BamlPIMappingNode( string xmlNamespace, string clrNamespace, string assemblyName ) : base(BamlNodeType.PIMapping) { _xmlNamespace = xmlNamespace; _clrNamespace = clrNamespace; _assemblyName = assemblyName; } internal override void Serialize(BamlWriter writer) { writer.WritePIMapping( _xmlNamespace, _clrNamespace, _assemblyName ); } internal override BamlTreeNode Copy() { return new BamlPIMappingNode( _xmlNamespace, _clrNamespace, _assemblyName ); } private string _xmlNamespace; private string _clrNamespace; private string _assemblyName; } ////// StartConstructor node /// internal sealed class BamlStartConstructorNode : BamlTreeNode { internal BamlStartConstructorNode(): base(BamlNodeType.StartConstructor) { } internal override void Serialize(BamlWriter writer) { writer.WriteStartConstructor(); } internal override BamlTreeNode Copy() { return new BamlStartConstructorNode(); } } ////// EndConstructor node /// internal sealed class BamlEndConstructorNode : BamlTreeNode { internal BamlEndConstructorNode(): base(BamlNodeType.EndConstructor) { } internal override void Serialize(BamlWriter writer) { writer.WriteEndConstructor(); } internal override BamlTreeNode Copy() { return new BamlEndConstructorNode(); } } ////////// ContentProperty node ///// internal sealed class BamlContentPropertyNode : BamlTreeNode { internal BamlContentPropertyNode( string assemblyName, string typeFullName, string propertyName ) : base(BamlNodeType.ContentProperty) { _assemblyName = assemblyName; _typeFullName = typeFullName; _propertyName = propertyName; } internal override void Serialize(BamlWriter writer) { writer.WriteContentProperty( _assemblyName, _typeFullName, _propertyName ); } internal override BamlTreeNode Copy() { return new BamlContentPropertyNode( _assemblyName, _typeFullName, _propertyName ); } private string _assemblyName; private string _typeFullName; private string _propertyName; } internal sealed class BamlPresentationOptionsAttributeNode : BamlTreeNode { internal BamlPresentationOptionsAttributeNode(string name, string value) : base(BamlNodeType.PresentationOptionsAttribute) { _name = name; _value = value; } internal override void Serialize(BamlWriter writer) { writer.WritePresentationOptionsAttribute(_name, _value); } internal override BamlTreeNode Copy() { return new BamlPresentationOptionsAttributeNode(_name, _value); } private string _name; private string _value; } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
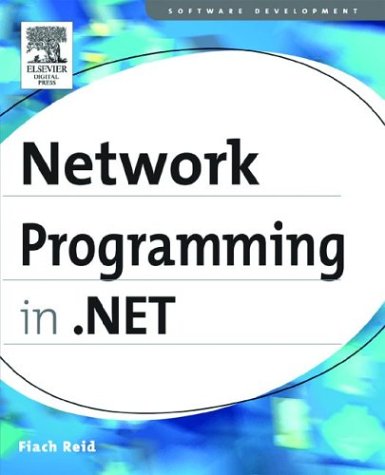
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BmpBitmapEncoder.cs
- WindowsProgressbar.cs
- AuthorizationSection.cs
- QualifierSet.cs
- SQLBytesStorage.cs
- PropertyMetadata.cs
- GridViewPageEventArgs.cs
- ProgressChangedEventArgs.cs
- ImageIndexConverter.cs
- ProfileBuildProvider.cs
- CodeExporter.cs
- FileLoadException.cs
- MessageContractMemberAttribute.cs
- JsonReaderDelegator.cs
- DrawListViewItemEventArgs.cs
- FlowDocumentReader.cs
- TextEditorSpelling.cs
- UserPersonalizationStateInfo.cs
- filewebrequest.cs
- DataGridViewCellMouseEventArgs.cs
- EventWaitHandleSecurity.cs
- XmlAttributeCollection.cs
- FormViewInsertedEventArgs.cs
- WindowsFormsSectionHandler.cs
- TableLayoutStyleCollection.cs
- FileLevelControlBuilderAttribute.cs
- EncodingDataItem.cs
- X509ChainPolicy.cs
- DesigntimeLicenseContext.cs
- TrustSection.cs
- ISFTagAndGuidCache.cs
- ChangeNode.cs
- HatchBrush.cs
- NameObjectCollectionBase.cs
- ObfuscationAttribute.cs
- RotateTransform3D.cs
- HandlerFactoryCache.cs
- MulticastDelegate.cs
- ButtonStandardAdapter.cs
- ConditionValidator.cs
- CollectionTypeElement.cs
- XmlWrappingReader.cs
- InstanceNotReadyException.cs
- DecimalFormatter.cs
- DocumentApplicationJournalEntry.cs
- DataTableExtensions.cs
- NavigationPropertyEmitter.cs
- NotifyParentPropertyAttribute.cs
- Pair.cs
- ToolboxBitmapAttribute.cs
- OutputScopeManager.cs
- CommonProperties.cs
- Int16Converter.cs
- ClientScriptItem.cs
- EmissiveMaterial.cs
- XmlSchemaCollection.cs
- MeshGeometry3D.cs
- DataGridLinkButton.cs
- MultiView.cs
- DbParameterCollectionHelper.cs
- PropertyInfoSet.cs
- BaseResourcesBuildProvider.cs
- XamlGridLengthSerializer.cs
- EnterpriseServicesHelper.cs
- WindowsGrip.cs
- BindingObserver.cs
- Roles.cs
- Int16Converter.cs
- HtmlTextBoxAdapter.cs
- SqlDeflator.cs
- SourceFileBuildProvider.cs
- HttpContextServiceHost.cs
- TimeEnumHelper.cs
- RequestQueue.cs
- TextElement.cs
- HtmlSelect.cs
- ModelPerspective.cs
- AbsoluteQuery.cs
- CapabilitiesPattern.cs
- CollectionChangedEventManager.cs
- WindowsFont.cs
- ListViewItemSelectionChangedEvent.cs
- Win32Exception.cs
- Cell.cs
- ListControlConvertEventArgs.cs
- Win32Interop.cs
- SelectionPattern.cs
- CellParagraph.cs
- SqlClientWrapperSmiStream.cs
- Instrumentation.cs
- LinkConverter.cs
- XmlWriter.cs
- TrackingExtract.cs
- ScalarType.cs
- EntityProviderFactory.cs
- DependencyObjectCodeDomSerializer.cs
- CacheForPrimitiveTypes.cs
- ReflectionUtil.cs
- precedingquery.cs
- MetadataUtilsSmi.cs