Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / LinkConverter.cs / 1 / LinkConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Drawing; using System.Diagnostics; using System.Globalization; using System.Reflection; ////// /// public class LinkConverter : TypeConverter { ///A TypeConverter for LinkLabel.Link objects. Access this /// class through the TypeDescriptor. ////// /// Determines if this converter can convert an object in the given source /// type to the native type of the converter. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// /// Converts the given object to the converter's native type. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { string text = ((string)value).Trim(); if (text.Length == 0) { return null; } else { // Parse 2 integer values - Start & Length of the Link. // if (culture == null) { culture = CultureInfo.CurrentCulture; } char sep = culture.TextInfo.ListSeparator[0]; string[] tokens = text.Split(new char[] {sep}); int[] values = new int[tokens.Length]; TypeConverter intConverter = TypeDescriptor.GetConverter(typeof(int)); for (int i = 0; i < values.Length; i++) { values[i] = (int)intConverter.ConvertFromString(context, culture, tokens[i]); } if (values.Length == 2) { return new LinkLabel.Link(values[0], values[1]); } else { throw new ArgumentException(SR.GetString(SR.TextParseFailedFormat, text, "Start, Length")); } } } return base.ConvertFrom(context, culture, value); } ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (value is LinkLabel.Link) { if (destinationType == typeof(string)) { LinkLabel.Link link = (LinkLabel.Link)value; if (culture == null) { culture = CultureInfo.CurrentCulture; } string sep = culture.TextInfo.ListSeparator + " "; TypeConverter intConverter = TypeDescriptor.GetConverter(typeof(int)); string[] args = new string[2]; int nArg = 0; args[nArg++] = intConverter.ConvertToString(context, culture, link.Start); args[nArg++] = intConverter.ConvertToString(context, culture, link.Length); return string.Join(sep, args); } if (destinationType == typeof(InstanceDescriptor)) { LinkLabel.Link link = (LinkLabel.Link)value; MemberInfo info; if (link.LinkData == null) { info = typeof(LinkLabel.Link).GetConstructor(new Type[] {typeof(int), typeof(int)}); if (info != null) { return new InstanceDescriptor(info, new object[] {link.Start, link.Length}, true); } } else { info = typeof(LinkLabel.Link).GetConstructor(new Type[] {typeof(int), typeof(int), typeof(object)}); if (info != null) { return new InstanceDescriptor(info, new object[] {link.Start, link.Length, link.LinkData}, true); } } } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
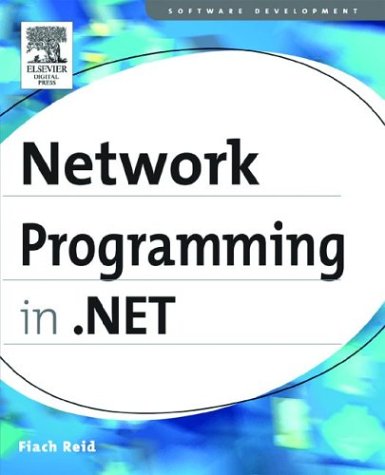
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FunctionUpdateCommand.cs
- AuthenticationServiceManager.cs
- HMACMD5.cs
- PersonalizationProvider.cs
- ServerIdentity.cs
- SyndicationItem.cs
- WindowsComboBox.cs
- UpdateCommandGenerator.cs
- PowerModeChangedEventArgs.cs
- TemplatePropertyEntry.cs
- ThreadStateException.cs
- StructuredTypeEmitter.cs
- ParameterCollection.cs
- WorkflowDurableInstance.cs
- BitmapEffectDrawing.cs
- HashSetDebugView.cs
- TreeNodeEventArgs.cs
- TrackingServices.cs
- DBSchemaTable.cs
- ResizeBehavior.cs
- CompositeDataBoundControl.cs
- ObjectDataSourceStatusEventArgs.cs
- FlowDocumentScrollViewer.cs
- BamlTreeUpdater.cs
- XmlDataFileEditor.cs
- ApplicationSecurityManager.cs
- StrokeCollection2.cs
- ScaleTransform.cs
- SplayTreeNode.cs
- SizeConverter.cs
- DataFormats.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- QuaternionRotation3D.cs
- RayMeshGeometry3DHitTestResult.cs
- ImageInfo.cs
- AspNetSynchronizationContext.cs
- Column.cs
- WorkflowPersistenceContext.cs
- SqlDataSourceSelectingEventArgs.cs
- XsltArgumentList.cs
- ExpandCollapsePattern.cs
- GenericUriParser.cs
- XmlAtomicValue.cs
- DoubleConverter.cs
- PreloadHost.cs
- RoleBoolean.cs
- DataSourceHelper.cs
- PrefixQName.cs
- HostedImpersonationContext.cs
- DecimalAnimationBase.cs
- ParsedAttributeCollection.cs
- DbTransaction.cs
- _SafeNetHandles.cs
- DerivedKeySecurityTokenStub.cs
- DecimalKeyFrameCollection.cs
- TypeListConverter.cs
- Metadata.cs
- AttributeCollection.cs
- ObjectDataSource.cs
- WebPartCollection.cs
- CultureSpecificStringDictionary.cs
- RNGCryptoServiceProvider.cs
- VirtualPathProvider.cs
- ClientSettingsProvider.cs
- SpellerStatusTable.cs
- UTF32Encoding.cs
- ProfileInfo.cs
- TextEditorParagraphs.cs
- Propagator.ExtentPlaceholderCreator.cs
- HTTPNotFoundHandler.cs
- SwitchCase.cs
- DataRecord.cs
- DefaultAutoFieldGenerator.cs
- DataGridBoolColumn.cs
- OracleBoolean.cs
- HtmlCalendarAdapter.cs
- CollectionContainer.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- Crypto.cs
- BitmapEncoder.cs
- DataMisalignedException.cs
- RowSpanVector.cs
- thaishape.cs
- TransactionFlowElement.cs
- AnnotationResource.cs
- AlphaSortedEnumConverter.cs
- TimeStampChecker.cs
- Symbol.cs
- NestPullup.cs
- XPathDocument.cs
- CodeDesigner.cs
- Win32PrintDialog.cs
- MultiBinding.cs
- OleStrCAMarshaler.cs
- Listbox.cs
- StackOverflowException.cs
- Evidence.cs
- ProfileEventArgs.cs
- FileAuthorizationModule.cs
- VisualBasicSettings.cs